Eliminating Duplicate Nodes in Neo4j: A How-To Guide
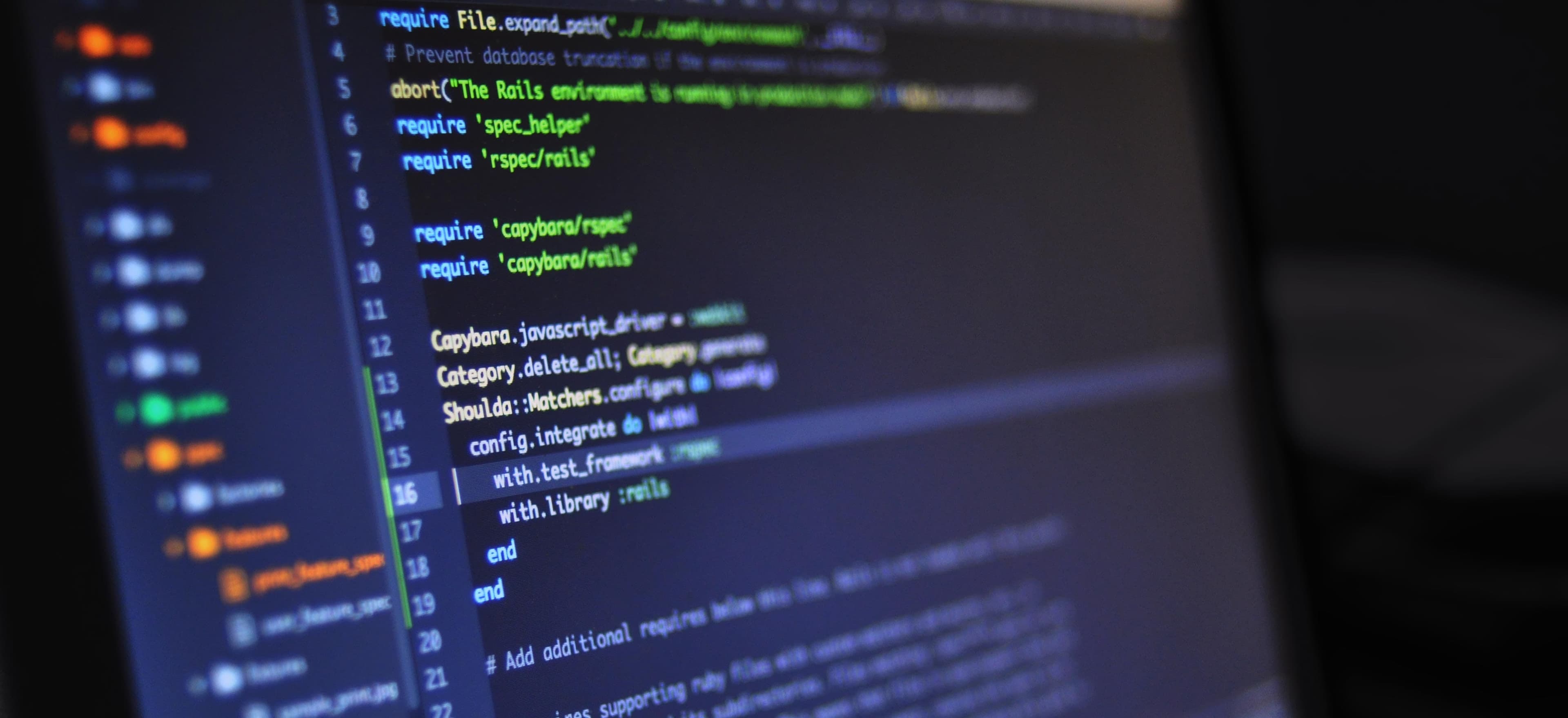
- Published on
Eliminating Duplicate Nodes in Neo4j: A How-To Guide
When managing data in graph databases like Neo4j, duplication can be a notorious issue. Duplicates not only bloat storage but can also lead to inaccuracies in data analysis and querying. In this guide, we will explore how to identify and eliminate duplicate nodes effectively in Neo4j, ensuring your graph database remains efficient and accurate.
Why Duplicate Nodes Occur in Neo4j
Before diving into solutions, it's essential to understand why duplicate nodes might creep into your graph database:
- Data Import Errors: When importing data from multiple sources, inconsistency in identifiers can lead to duplicates.
- Merging Data Sources: If two or more datasets are combined without deduplication efforts, duplicates may arise.
- User Errors: Applications that allow user input might inadvertently create duplicates when users input what they believe to be new data.
Understanding Neo4j's Unique Constraints
Before we address how to eliminate duplicates, it’s worth mentioning Neo4j's unique constraints feature. By defining a property (or properties) as unique for a node label, you prevent duplicates from being created in the first place.
To create a unique constraint:
CREATE CONSTRAINT ON (n:Person) ASSERT n.email IS UNIQUE;
In this example, we are asserting that the email
property for nodes labeled Person
must be unique. This command will help prevent duplicates based on email addresses.
However, existing duplicates must be addressed before applying a unique constraint. Here’s how to find and eliminate them.
Step 1: Identify Duplicate Nodes
To find duplicate nodes in your Neo4j database, you can run a Cypher query. Below is a sample query that finds duplicate nodes based on a specified property; in this case, we'll use the email
property for nodes labeled Person
.
MATCH (p:Person)
WITH p.email AS email, COLLECT(p) AS nodes
WHERE SIZE(nodes) > 1
RETURN email, nodes;
Explanation of the Query
MATCH (p:Person)
: This clause searches for all nodes labeledPerson
.WITH p.email AS email, COLLECT(p) AS nodes
: Collects all nodes with the same email into a list.WHERE SIZE(nodes) > 1
: Filters only those email groups that have more than one node (duplicates).RETURN email, nodes
: Outputs the duplicate email and their corresponding nodes.
Running this query will give you a list of duplicate email addresses and the nodes associated with them.
Step 2: Remove Duplicate Nodes
Once you’ve identified duplicate nodes, you can proceed to eliminate them. Here’s how:
Strategy: Keep One and Delete the Others
Generally, the best approach is to keep one of the duplicate nodes and delete the rest. You might want to keep the one with the most relationships or the one that was created first. Below is an example where we choose to keep the node with the lowest internal ID:
MATCH (p:Person)
WITH p.email AS email, COLLECT(p) AS nodes
WHERE SIZE(nodes) > 1
FOREACH (x IN TAIL(nodes) |
DETACH DELETE x
);
Explanation of the Delete Query
FOREACH (x IN TAIL(nodes) | DETACH DELETE x)
: This clause iterates over all nodes except the first (which we assume to keep) and deletes each of the duplicate nodes irreversibly.
Important Consideration
After deleting duplicates, you should verify whether any relationships are affected. The DETACH DELETE
command will remove both the node and its relationships, which is why it’s recommended to ensure that the remaining node has all the necessary relationships from the deleted nodes.
Step 3: Re-Create Relationships if Necessary
In some cases, if you want to retain relationships from all duplicates, you might consider transferring relationships before deleting the duplicates. This can be done by first collecting the relationships and then re-attaching them to the node you want to keep.
Here’s a rough example:
MATCH (p:Person)
WITH p.email AS email, COLLECT(p) AS nodes
WHERE SIZE(nodes) > 1
UNWIND nodes AS n
WITH n, HEAD(nodes) AS keep // Keep the first found
MATCH (n)<-[r]->()
CREATE (keep)<-[r]-(n) // Recreate the relationships
DETACH DELETE n; // Now delete the duplicate
Explanation
UNWIND nodes AS n
: This expands the collection of nodes into individual nodes.WITH n, HEAD(nodes) AS keep
: Here, we specify that the first node is our keeper.MATCH (n)<-[r]->()
: This matches all incoming relationships to the node.CREATE (keep)<-[r]-(n)
: This creates the relationships from the duplicates to the keeper.DETACH DELETE n;
: Finally, this deletes the duplicate node.
To Wrap Things Up
Eliminating duplicate nodes in Neo4j is an essential maintenance task that ensures data integrity and efficient querying. With the techniques outlined above, you should be able to proficiently identify and remove duplicates from your database.
Additional Resources
- Neo4j Documentation: Constraints and Indexes
- Understanding Cypher queries
- Neo4j Graph Data Science
By leveraging Neo4j’s unique constraints and employing efficient query techniques, your graph database can maintain high performance and reliability as it scales. Happy querying!
Checkout our other articles