Struggling with Multiple Router Functions in Spring WebFlux?
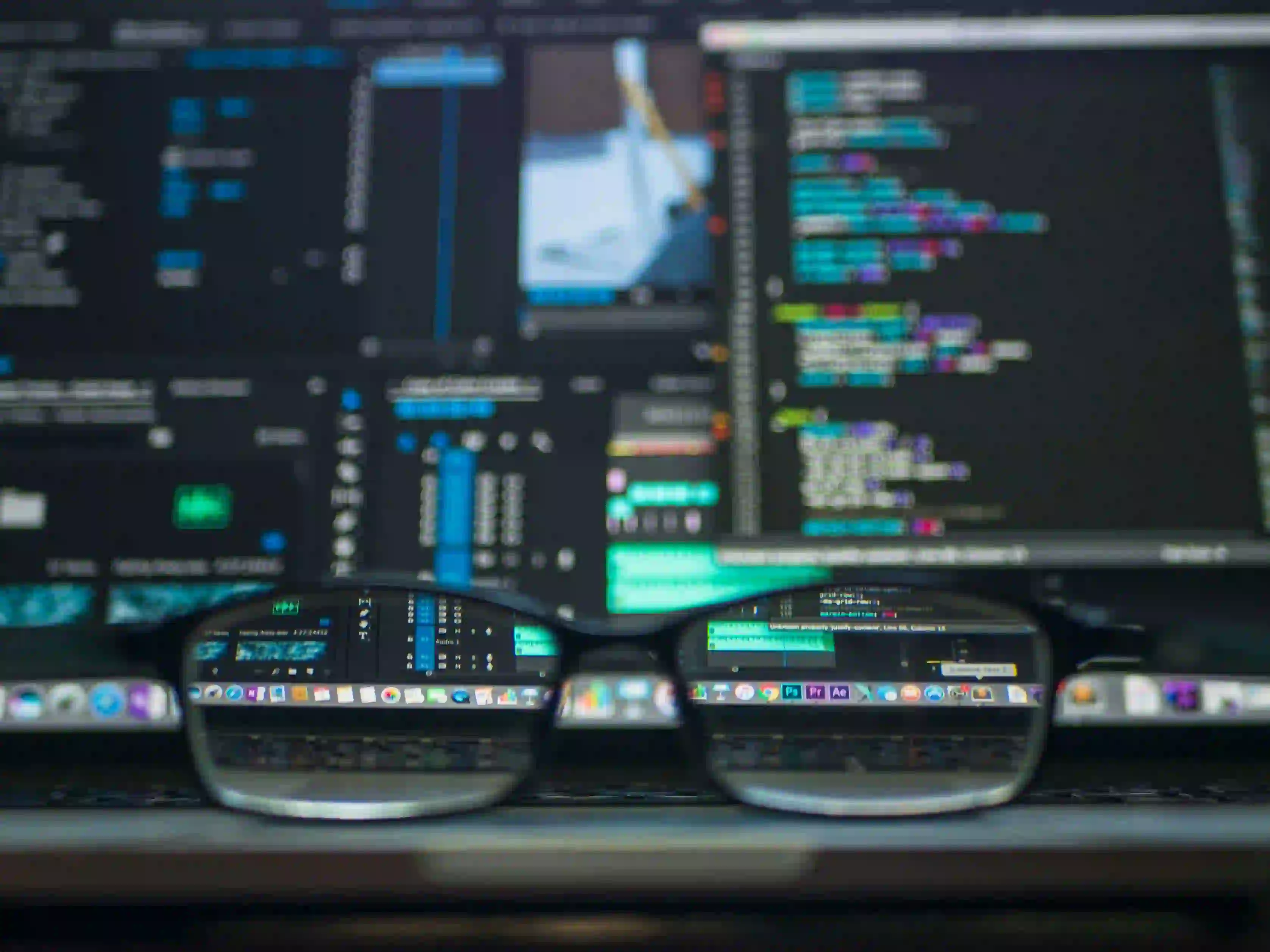
Struggling with Multiple Router Functions in Spring WebFlux?
When developing reactive applications with Spring WebFlux, routing can sometimes become a complicated task. Whether you are a seasoned developer or just stepping into the realm of reactive programming, understanding how to manage multiple router functions effectively can significantly improve your code quality and application performance.
In this blog post, we will cover everything you need to know about creating and managing multiple router functions in Spring WebFlux. We will discuss best practices, provide sample code snippets, and explore some advanced scenarios to help you become proficient in using router functions.
What is Spring WebFlux?
Spring WebFlux is part of the larger Spring 5 framework and offers a reactive programming model for building non-blocking, event-driven applications. Unlike Spring MVC, which is blocking and thread-per-request, WebFlux uses a reactive stack, enabling efficient resource management and higher scalability.
Key Features of Spring WebFlux:
- Non-blocking: It allows for handling many concurrent connections with fewer resources.
- Reactive: Supports reactive programming paradigms using Project Reactor, which promotes asynchronous data processing.
- Flexible: You can use it with different runtimes including Netty, Undertow, and Servlet containers.
For detailed information about setting up a Spring WebFlux project, refer to the official Spring WebFlux Documentation.
Understanding Router Functions
Router functions in Spring WebFlux provide a programmatic way of defining routes for handling HTTP requests. Rather than using controllers, you can create a configuration of routes using functional programming.
Why Use Router Functions?
- Separation of Concerns: Keeping routing logic separate from business logic.
- Composability: Easily compose routes through higher-order functions.
- Declarative Routing: Allows for a cleaner, more simplified way to define routes without the overhead of annotations.
Basic Example
Let's start with a simple example of routing using router functions:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.reactive.function.server.RouterFunction;
import org.springframework.web.reactive.function.server.ServerRequest;
import org.springframework.web.reactive.function.server.ServerResponse;
import static org.springframework.web.reactive.function.server.RouterFunctions.route;
import static org.springframework.web.reactive.function.server.ServerResponse.ok;
@Configuration
public class RouterConfig {
@Bean
public RouterFunction<ServerResponse> routes() {
return route()
.GET("/hello", this::helloHandler)
.build();
}
private Mono<ServerResponse> helloHandler(ServerRequest request) {
return ok().bodyValue("Hello, World!");
}
}
Explanation of Code Snippet:
- Configuration Class: The
RouterConfig
class is annotated with@Configuration
. This tells Spring that it contains beans to be processed. - Routes Method: The
routes
method returns aRouterFunction<ServerResponse>
. It defines how to map HTTP requests to handler functions. - Handler Method: The
helloHandler
returns a greeting message when the/hello
endpoint is accessed.
Managing Multiple Router Functions
In a real-world application, you'll likely have multiple routes resembling different functionalities. To keep the code organized, it can be useful to group routes logically.
Grouping Routes
Here's how to handle multiple routes grouped by functionality:
@Configuration
public class UserRouter {
@Bean
public RouterFunction<ServerResponse> userRoutes(UserHandler userHandler) {
return route()
.GET("/users", userHandler::listUsers)
.GET("/users/{id}", userHandler::getUser)
.POST("/users", userHandler::createUser)
.build();
}
}
@Configuration
public class OrderRouter {
@Bean
public RouterFunction<ServerResponse> orderRoutes(OrderHandler orderHandler) {
return route()
.GET("/orders", orderHandler::listOrders)
.GET("/orders/{id}", orderHandler::getOrder)
.POST("/orders", orderHandler::createOrder)
.build();
}
}
Explanation of Code Snippet:
- Separation of Concerns: User and Order routes are defined in separate configuration classes (
UserRouter
andOrderRouter
). This makes the code more maintainable. - Parameterized Routes: The
/users/{id}
and/orders/{id}
routes demonstrate parameterized paths, allowing you to retrieve specific resources based on their IDs.
Error Handling in Router Functions
Error handling is crucial in any application. With WebFlux, you can handle errors gracefully using custom handlers.
Adding an Error Handler
Here's how to set up a global error handler:
import org.springframework.web.reactive.function.server.ServerResponse;
public class CustomErrorHandler {
public Mono<ServerResponse> handleException(ServerRequest request, Throwable throwable) {
return ServerResponse.status(HttpStatus.INTERNAL_SERVER_ERROR)
.bodyValue("An error occurred: " + throwable.getMessage());
}
}
Integrating Error Handling with Router Functions
You can integrate the custom error handler within your routes:
@Bean
public RouterFunction<ServerResponse> userRoutes(UserHandler userHandler, CustomErrorHandler errorHandler) {
return route()
.GET("/users", userHandler::listUsers)
.onError(Throwable.class, errorHandler::handleException)
.build();
}
Explanation of Code Snippet:
- Custom Error Handler: The
CustomErrorHandler
class captures exceptions and provides a meaningful response. - Global Error Handling: The
.onError
method ensures that any errors occurring within the route will be handled by thehandleException
method.
The Last Word
Managing multiple router functions in Spring WebFlux may seem daunting initially, but with an organized approach and understanding of the concepts, it can become second nature. By separating concerns and leveraging functional routing, you can create cleaner, more maintainable applications.
As you dive deeper into Spring WebFlux, remember to explore the official Spring documentation and Project Reactor for advanced topics like reactive streams and schedulers.
Further Reading
- Spring WebFlux Getting Started
- Functional Endpoints with Spring WebFlux
With these resources and code examples, you are well on your way to mastering Spring WebFlux routing in your applications. Happy coding!