Java Concurrency: Handling Callable vs. Runnable Confusion
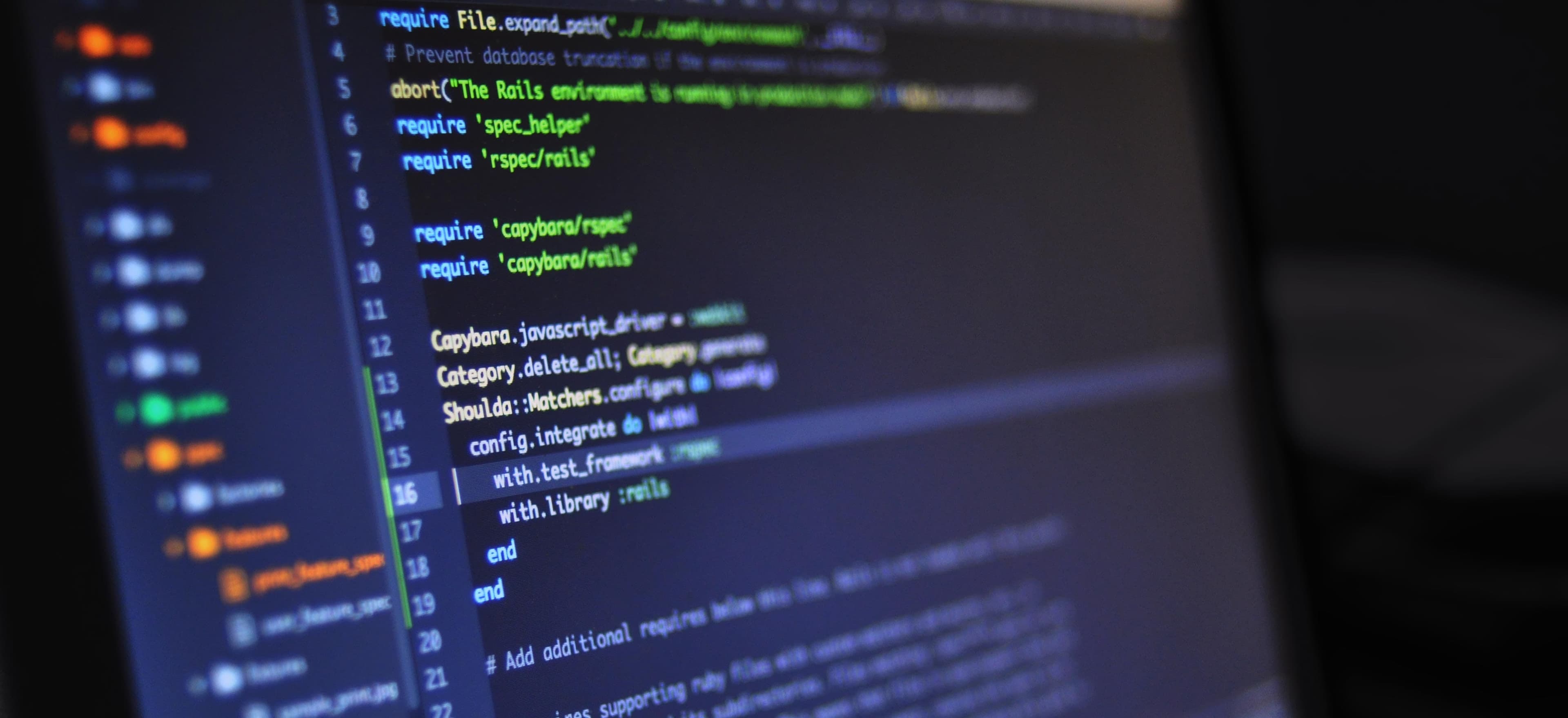
- Published on
Java Concurrency: Handling Callable vs. Runnable Confusion
Java concurrency can be a complex area for many developers, especially when it comes to understanding the differences between Runnable
and Callable
. In this blog post, we will clarify these concepts, delve into their use cases, and provide relevant code snippets to help you gain a better grasp of how they operate in a concurrent context.
Understanding Runnable
Firstly, let's take a look at the Runnable
interface. It represents a task that can be executed by a thread. One of the key features of Runnable
is that it does not return a result or throw a checked exception. This means its use cases are typically limited to tasks that do not require any output or error handling in a granular way.
Basic Example of Runnable
Here is a simple example of a Runnable
implementation:
public class MyRunnable implements Runnable {
@Override
public void run() {
System.out.println("Executing Runnable Task");
}
}
// Usage
Thread thread = new Thread(new MyRunnable());
thread.start();
In this example:
MyRunnable
implements theRunnable
interface and overrides therun
method.- The
run
method contains the logic to be executed. - A new
Thread
object is created, passing in theMyRunnable
instance, and the thread is started using thestart()
method.
Why Choose Runnable?
- Simplicity: For straightforward tasks where you don’t require a return value.
- Lightweight:
Runnable
is less resource-intensive since it does not require capturing a return value.
Understanding Callable
On the other hand, Callable
is a more flexible and powerful alternative to Runnable
. It is also a functional interface that can be used to define tasks meant to be executed by threads. The crucial distinction to note is that Callable
can return a result and can throw checked exceptions, making it ideal for tasks where error handling and results matter.
Basic Example of Callable
Here is an example of a Callable
implementation:
import java.util.concurrent.Callable;
public class MyCallable implements Callable<String> {
@Override
public String call() throws Exception {
return "Result from Callable Task";
}
}
// Usage
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
ExecutorService executorService = Executors.newSingleThreadExecutor();
Future<String> futureResult = executorService.submit(new MyCallable());
try {
String result = futureResult.get();
System.out.println(result);
} catch (Exception e) {
e.printStackTrace();
} finally {
executorService.shutdown();
}
In this example:
MyCallable
implementsCallable<String>
and overrides thecall
method.- The
call
method returns aString
, which is the result of the computation. - An
ExecutorService
is created to manage the thread pool, and the callable is submitted. - The result is obtained through the
Future
interface, allowing for synchronous retrieval of the result.
Why Choose Callable?
- Return Value: Perfect for tasks that need to produce results.
- Exception Handling: Can throw checked exceptions, offering better error managing solutions.
Key Differences between Runnable and Callable
To further clarify the confusion that sometimes arises with these two interfaces, consider the following key differences:
| Feature | Runnable | Callable | |---------|----------|----------| | Return Type | void | Any type (e.g., String, Integer) | | Exception Handling | Cannot throw checked exceptions | Can throw checked exceptions | | Usage in ExecutorService | Can be submitted but return nothing | Can be submitted and returns a Future object |
Performance Considerations
Both Runnable
and Callable
are critical in the construction of concurrent applications. However, their performance implications can vary based on usage context. For instance, if a task is purely computational and does not yield a useful result, Runnable
can be considered more lightweight. In contrast, if your application requires results from concurrent tasks, Callable
can facilitate that more effectively.
Practical Use Cases
When to Use Runnable
- Background tasks: For tasks where the result is not essential, such as logging events or performing cleanup operations.
- Fire-and-forget scenarios: Operations where you initiate a task but don't need to know the outcome.
When to Use Callable
- Concurrency with results: When the execution of tasks requires a return value that might be further processed.
- Complex error handling: Through the ability to throw checked exceptions, Callable is fitting for tasks requiring in-depth error management.
In Conclusion, Here is What Matters
In summary, understanding the distinction between Runnable
and Callable
is essential for effectively utilizing Java's concurrency framework. Each interface has its particular strengths and ideal use cases.
- Use Runnable for tasks with no result or exceptions.
- Use Callable for tasks that need to return a result and can throw exceptions.
By keeping these guidelines in mind, you can make informed decisions about which interface to implement in your Java applications effectively.
For further learning, you might find these resources helpful:
With that said, it's time to dive into concurrency and optimize your Java applications! Happy coding!
Checkout our other articles