Streamline JNDI Resource Testing in Spring with JUnit
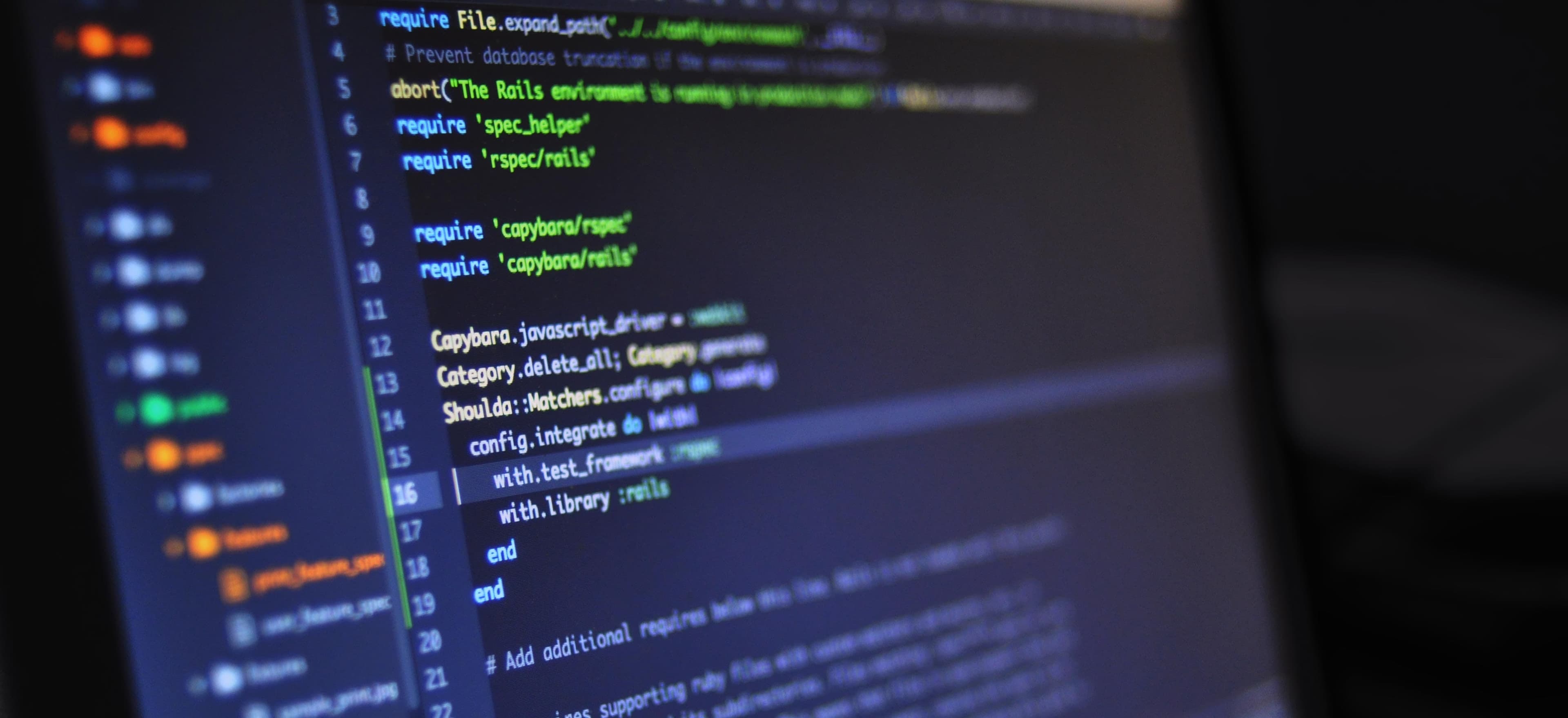
- Published on
Streamline JNDI Resource Testing in Spring with JUnit
When developing Java applications, especially in enterprise environments, managing JNDI (Java Naming and Directory Interface) resources can turn into a complex task. The Spring Framework has made integrating JNDI resources simpler, yet testing those integrations can still present challenges. This is where JUnit comes in. In this blog post, we will explore how you can streamline JNDI resource testing in Spring using JUnit while ensuring maintainability and efficiency in your tests.
Understanding JNDI
JNDI is an API that provides naming and directory functionality for Java applications. It allows clients to look up resources such as database connections and other Java objects. In typical applications, JNDI resources are configured in the application server's context. However, when it comes to unit testing, you often want to mock these resources instead of depending on a live server environment.
Integrating JNDI in Spring
Spring simplifies the integration with JNDI resources by allowing you to define them in a Spring application context. Here's a simple example of how you might declare a JNDI DataSource in your applicationContext.xml
:
<bean id="dataSource" class="org.springframework.jdbc.datasource.lookup.JndiDataSourceLookup">
<property name="jndiName" value="java:comp/env/jdbc/MyDataSource" />
</bean>
In the example above, we define a dataSource
bean that Spring will use to look up the JNDI resource called jdbc/MyDataSource
.
Setting Up Your Test
To unit test components that rely on JNDI resources, we can use Spring's testing framework along with JUnit. By utilizing a test context configuration, we can configure mock or in-memory resources. Here is how you can set it up:
Step 1: Dependencies
Make sure you have the following dependencies in your pom.xml
if you are using Maven:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.3.13</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-test</artifactId>
<version>5.3.13</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13.2</version>
<scope>test</scope>
</dependency>
Step 2: Create a Configuration for Tests
We will create a test configuration that sets up a mock JNDI resource. Here's an illustrative code snippet:
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Import;
import org.springframework.test.context.junit.jupiter.SpringJUnitConfig;
import javax.naming.Context;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.sql.DataSource;
import static org.junit.jupiter.api.Assertions.assertNotNull;
import static org.mockito.Mockito.mock;
@SpringJUnitConfig
public class DataSourceTest {
@Autowired
private DataSource dataSource;
@BeforeEach
void setUp() throws NamingException {
mockJndi();
}
private void mockJndi() throws NamingException {
Context context = new InitialContext();
context.bind("java:comp/env/jdbc/MyDataSource", mock(DataSource.class));
}
@Test
void testDataSourceNotNull() {
assertNotNull(dataSource);
}
@Configuration
static class TestConfig {
@Bean
public DataSource dataSource() {
return (DataSource) new InitialContext().lookup("java:comp/env/jdbc/MyDataSource");
}
}
}
Commentary on Code
-
mockJndi(): We simulate JNDI lookup using the
InitialContext
. This method binds a mockDataSource
to the JNDI context, ensuring that your tests won't fail due to a lack of actual resources. -
@SpringJUnitConfig: This annotation configures the application context for the test, enabling the user to inject beans directly.
-
Test Configuration: The
TestConfig
class defines adataSource
bean, corresponding to what you would expect in your main application configuration.
Running the Tests
Now that we’ve set up our test configuration and JNDI mocking, you can run your tests. Once executed, the test for dataSource
ensures that it properly retrieves the mocked DataSource
.
Here are the commands to run your tests on Maven:
mvn test
It will execute any test classes in your project, including those we set up earlier.
Handling Different Environments
In some cases, you may want to customize the behavior of your beans based on the environment, whether that’s production or testing. Spring profiles can be extremely useful in these scenarios. Here's how you might implement this:
Using Profiles
You can define separate bean configurations for various profiles. Below is a modified version of the prior DataSourceTest
with JNDI conditions based on profiles:
@Configuration
@Profile("test")
static class TestConfig {
@Bean
public DataSource dataSource() {
return (DataSource) new InitialContext().lookup("java:comp/env/jdbc/MyDataSource");
}
}
@Configuration
@Profile("prod")
static class ProdConfig {
@Bean
public DataSource dataSource() {
// Normally, you'd return the actual DataSource here
return ...; // your actual DataSource configuration
}
}
When you run tests, activate the "test" profile to run the mocked version by using the command:
mvn test -Dspring.profiles.active=test
Final Thoughts
Testing JNDI resources in Spring with JUnit can be a straightforward process if you take advantage of Spring's features, including context configuration, profiles, and mocking. By mocking JNDI resources, you ensure that your unit tests are fast, reliable, and independent of the actual server environment.
For further insights into testing with Spring, visit the official Spring Testing Documentation. You can also refer to this comprehensive guide on JNDI that elaborates on its functionalities.
By adopting these practices in your development workflow, you can drastically enhance your efficiency while maintaining test integrity and application quality. Happy coding!
Checkout our other articles