Challenges of Spring Prototype Beans with Dynamic Parameters
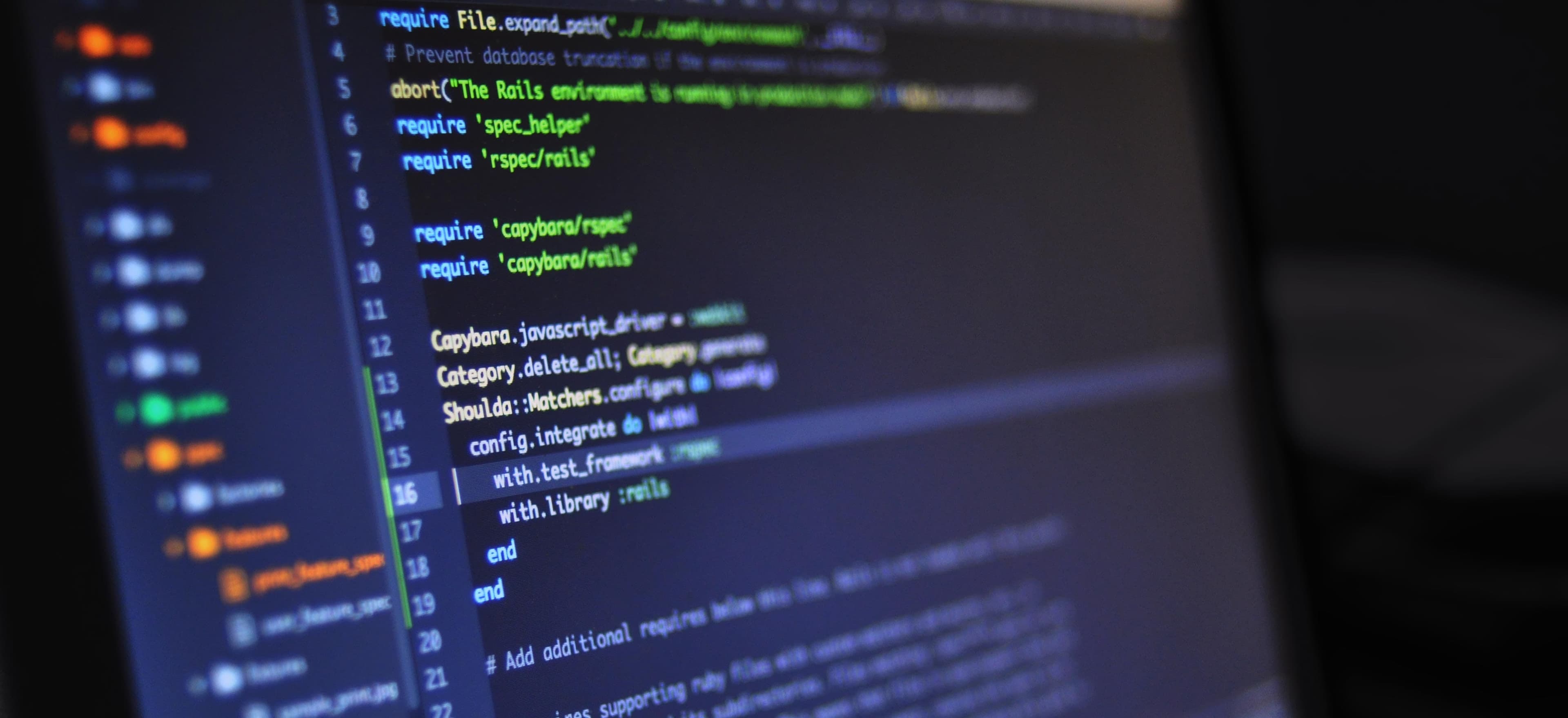
- Published on
Challenges of Spring Prototype Beans with Dynamic Parameters
Spring Framework is a widely-used tool for building Java applications due to its vast ecosystem and robust features. One notable aspect is its ability to manage beans, especially the various scopes that can be applied to them. Today, we will explore the challenges associated with Spring Prototype Beans when handling dynamic parameters.
Understanding Prototype Beans
Before we delve into the challenges, let’s clarify what Prototype Beans are. In Spring, when a bean is provided with a prototype scope, a new instance of that bean is created each time it is requested. This is fundamentally different from the singleton scope, where only one instance is created and shared.
When to Use Prototype Beans
Using Prototype Beans can be beneficial in scenarios where:
- Each operation requires a new instance: For example, in a multi-threaded application where multiple threads may alter the state of the same instance.
- Specific configurations per instance: If different clients need beans configured differently, prototype beans will meet this requirement.
Challenges with Prototype Beans and Dynamic Parameters
While Prototype Beans offer flexibility, they come with their own set of challenges, especially when dynamic parameters are involved. Here are the primary issues one might encounter:
1. Dependency Management
Issue: Each prototype bean needs its dependencies defined. When these dependencies are dynamic, maintaining the state for each instance can become cumbersome.
Solution: Use contextual information to dynamically inject dependencies based on the parameters each instance requires.
Example Code
@Component
public class DynamicService {
public String process(String parameter) {
// Placeholder for processing logic
return "Processed: " + parameter;
}
}
@Component
@Scope("prototype")
public class PrototypeBean {
private final DynamicService dynamicService;
@Autowired
public PrototypeBean(DynamicService dynamicService) {
this.dynamicService = dynamicService;
}
public String execute(String input) {
return dynamicService.process(input);
}
}
2. Lack of Singleton Benefits
Issue: Prototype Beans do not support singleton benefits, including caching of expensive resources. If you repeatedly create the same bean, you may encounter performance problems.
Solution: Use a combination of factory patterns to manage object creation efficiently.
Example Code for Factory Pattern
@Component
public class PrototypeBeanFactory {
@Autowired
private ApplicationContext context;
public PrototypeBean createPrototype(String parameter) {
PrototypeBean bean = context.getBean(PrototypeBean.class);
// You may inject parameters if needed through setter or direct methods
return bean;
}
}
3. Aware of Thread-Safety Issues
Issue: If multiple threads request prototype beans with the same parameters, it can lead to unintended side-effects if shared mutable state is used.
Solution: Ensure that each prototype bean is fully encapsulated and does not maintain shared mutable state.
4. Managing Lifecycle Events
Issue: Unlike singleton beans, lifecycle events such as initialization and destruction (postConstruct, preDestroy) need to be handled for each instance separately.
Solution: Implement the DisposableBean
and InitializingBean
interfaces or use annotations like @PostConstruct
and @PreDestroy
.
Example Lifecycle Management Code
@Component
@Scope("prototype")
public class LifecycleManagedBean implements InitializingBean, DisposableBean {
@Override
public void afterPropertiesSet() {
// Initialization logic
}
@Override
public void destroy() {
// Cleanup logic
}
}
5. Resource Management
Issue: Creating many instances can lead to resource leakage if not managed properly.
Solution: Use a pool of reusable objects or properly manage your resources when instances are no longer needed.
Practical Implementation
Let's put together a more complete example that illustrates all these concepts.
Full Example
@Configuration
public class AppConfig {
@Bean
@Scope("prototype")
public PrototypeBean prototypeBean() {
return new PrototypeBean();
}
@Bean
public DynamicService dynamicService() {
return new DynamicService();
}
@Bean
public PrototypeBeanFactory prototypeBeanFactory() {
return new PrototypeBeanFactory();
}
}
public class Client {
private final PrototypeBeanFactory beanFactory;
@Autowired
public Client(PrototypeBeanFactory beanFactory) {
this.beanFactory = beanFactory;
}
public void execute() {
PrototypeBean bean1 = beanFactory.createPrototype("First Call");
System.out.println(bean1.execute("Hello World"));
PrototypeBean bean2 = beanFactory.createPrototype("Second Call");
System.out.println(bean2.execute("Java Spring"));
}
}
Explanation of Full Example
PrototypeBeanFactory
: Manages the creation of prototype beans without directly exposing the prototype scope outside of its context.DynamicService
: Represents a service that performs custom behavior based on the input provided by other classes.Client
: Demonstrates how to create and use prototype beans with dynamic parameters effectively.
Closing the Chapter
In conclusion, while Spring's Prototype Beans are powerful tools that allow for the creation of unique instances suited to varied situations, they come with several challenges when handling dynamic parameters. By understanding these challenges and applying best practices such as dependency management, lifecycle event handling, and careful management of resources, developers can harness the full potential of Prototype Beans.
For further reading on Spring Framework capabilities, check out the Spring Documentation and explore additional topics such as Bean Scopes.
By being aware of the intricacies of Spring's bean management, you can build more resilient and scalable applications that cater efficiently to varied user needs. Happy coding!