Overcoming Common Docker Testing Pitfalls
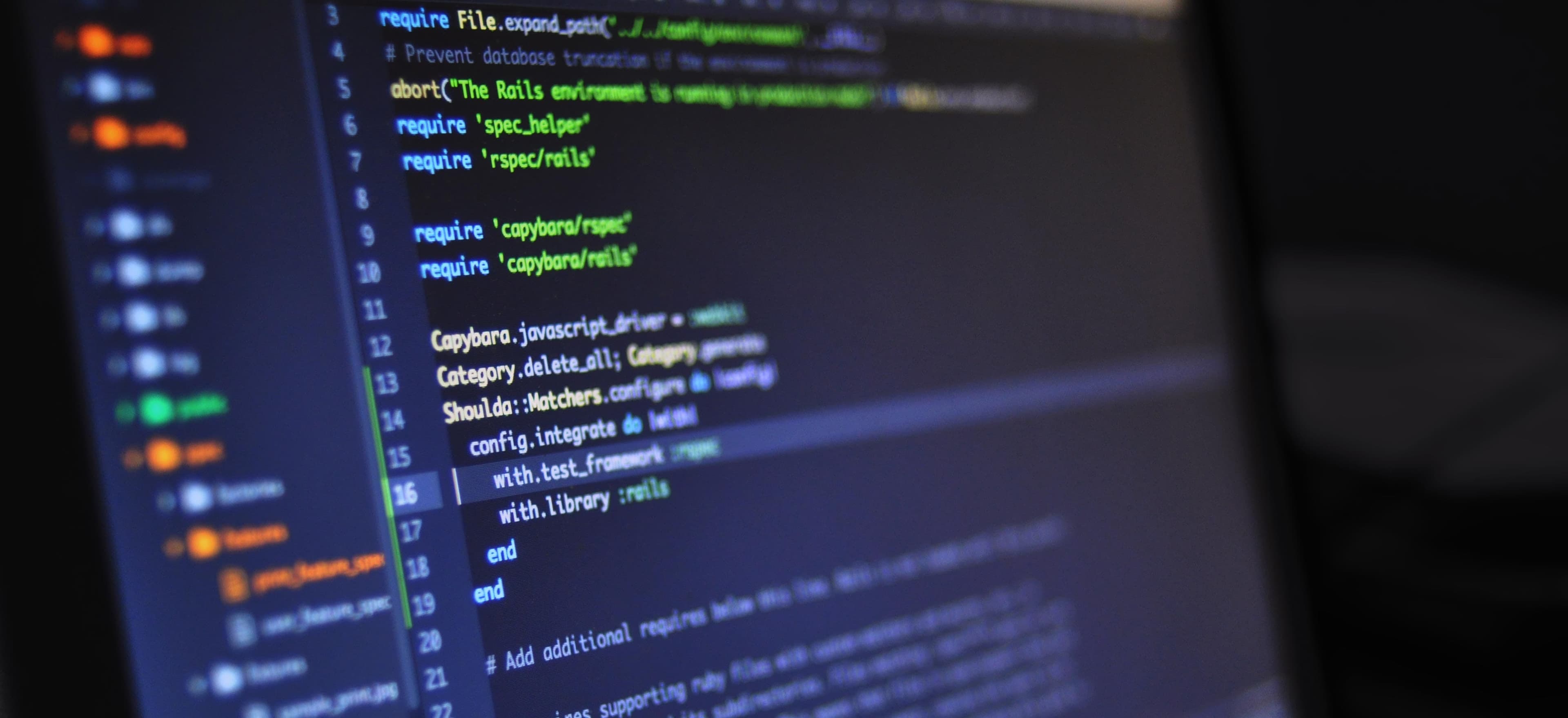
- Published on
Overcoming Common Docker Testing Pitfalls
Docker has revolutionized the way developers deploy and test applications. Its containerization technology allows for consistent environments, reducing the "it works on my machine" syndrome. However, despite its many advantages, testing with Docker can come with its own set of challenges. This blog post will outline common pitfalls developers encounter while testing with Docker and provide strategies to overcome them.
1. Understanding Docker's Abstraction Layers
One of the initial pitfalls many developers face is misunderstanding how Docker abstracts the application environment.
- Why It Matters: Docker encapsulates applications in containers, which can lead to discrepancies in how applications behave in different environments. For instance, a service that works seamlessly on your local machine might run into compatibility issues on a staging or production server.
Strategy to Overcome
- Use a
.dockerignore
File: Exclude unnecessary files and directories from the Docker build context. This decreases image size and speeds up the build process.
# Sample .dockerignore file
node_modules
npm-debug.log
*.md
- Emulate the Production Environment: Use the same base image and service configurations in both your testing and production environments. An example of a Dockerfile for a Node.js application may look like this:
FROM node:14
# Set the working directory
WORKDIR /app
# Copy your package.json and install dependencies
COPY package*.json ./
RUN npm install
# Copy the rest of your application files
COPY . .
# Start the application
CMD ["node", "app.js"]
2. Networking Issues in Docker
Another common challenge arises from Docker's networking model. Testers often overlook how data passes between containers.
- Why It Matters: By default, containers run in an isolated network, which can cause issues when they need to communicate with one another or with external services.
Strategy to Overcome
- Utilize Docker Compose: For multi-container applications, Docker Compose simplifies running and managing connected services. Below is a sample
docker-compose.yml
file that demonstrates how to set up a web server and a database:
version: '3'
services:
web:
build: .
ports:
- "80:8080"
depends_on:
- db
db:
image: postgres:latest
environment:
POSTGRES_USER: user
POSTGRES_PASSWORD: password
- Network Configuration: Ensure to define networks in your
docker-compose.yml
. This allows containers to discover and communicate with each other easily.
3. Using Containers for Testing with Persistent Data
Testing often requires persistent data, and utilizing Docker's ephemeral nature can be misleading.
- Why It Matters: If you do not configure data persistence correctly, tests may fail due to missing states or earlier configurations, making the debugging process more complicated.
Strategy to Overcome
- Volumes and Bind Mounts: Use Docker volumes to retain data despite container restarts. For example:
version: '3'
services:
app:
image: my-app
volumes:
- app_data:/data
volumes:
app_data:
This configuration ensures that the data in /data
persists beyond the container’s lifecycle.
4. Testing with a Lack of Isolation
Many developers run tests against the same containers that serve their application. This can cause unforeseen side effects.
- Why It Matters: Sharing containers for testing and production can lead to performance issues and make it harder to diagnose problems.
Strategy to Overcome
- Dedicated Test Containers: Always run your tests in isolated containers. This means setting up your test environment in a separate Docker Compose file or creating a dedicated testing image.
docker build -t my-app:test .
- Container Cleanup: After testing, ensure containers and their resources are cleaned up, to prevent leftover data from interfering with future tests:
docker-compose down -v
5. Debugging Containerized Applications
Debugging applications in containers can be like finding a needle in a haystack.
- Why It Matters: The abstraction that Docker provides can make it hard to understand what's happening inside a running container.
Strategy to Overcome
- Use Docker Exec for Debugging: You can use the
docker exec
command to open a terminal in a running container. This is useful for inspecting a running application.
docker exec -it my_container /bin/bash
- Logging: Ensure that your application logs to the console, as Docker captures these logs by default. You can view them with:
docker logs my_container
6. Failing to Optimize the Dockerfile
Neglecting Dockerfile optimization can lead to unnecessary large image sizes and slow build times.
- Why It Matters: Every layer in a Dockerfile increases the image size. This can result in slower deployments and inefficient resource usage.
Strategy to Overcome
- Minimize Number of Layers: Combine commands that can be run in the same layer. For example, instead of:
RUN apt-get update
RUN apt-get install -y curl
Combine them into one command:
RUN apt-get update && apt-get install -y curl
- Use Multi-Stage Builds: This technique allows you to create smaller production images by only copying the necessary artifacts from a larger build image.
# First stage
FROM node:14 AS builder
WORKDIR /app
COPY . .
RUN npm install && npm run build
# Second stage
FROM nginx:alpine
COPY --from=builder /app/build /usr/share/nginx/html
Bringing It All Together
Docker is a powerful tool for application deployment and testing, but it comes with its challenges. By understanding these common pitfalls and implementing the strategies outlined in this post, developers can greatly enhance their Docker testing process. Testing is critical to the software development lifecycle, and as Docker continues to evolve, mastering its intricacies will allow teams to deliver more reliable applications.
For more detailed insights into Docker and containerization, consider checking Docker's Official Documentation or Kubernetes Guides. These resources provide comprehensive coverage of container orchestration and management, enhancing your knowledge and capabilities in the container world.
Now get out there, refine your Docker practices, and test your applications with confidence!