Preventing Inadvertent Recursion in Java with ThreadLocals
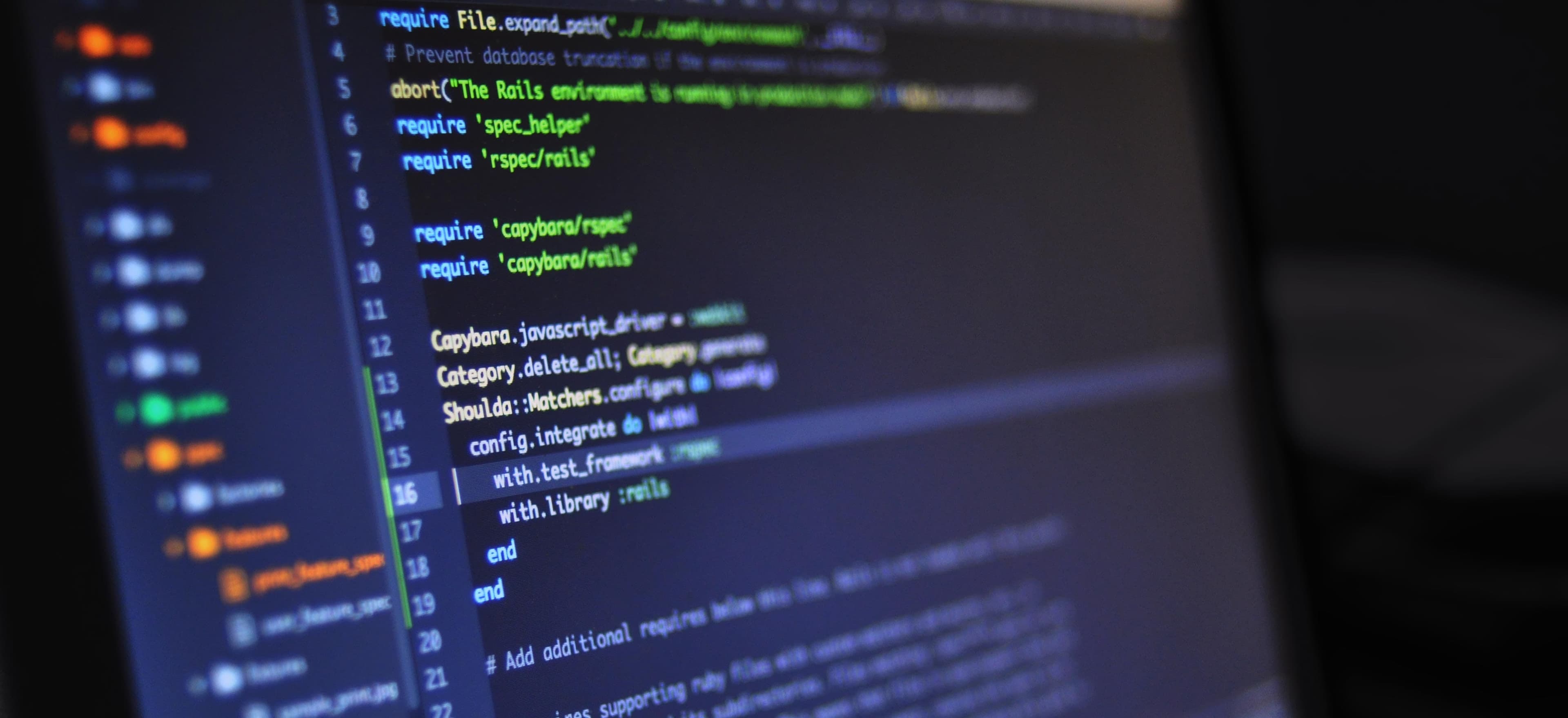
- Published on
Preventing Inadvertent Recursion in Java with ThreadLocals
In the world of programming, recursion is often a key technique used to simplify complex problems. However, it can lead to inadvertent issues, especially when there's a lack of control over the recursive calls. This is particularly true in multithreaded environments, where threads may unintentionally invoke the same method recursively, resulting in stack overflow errors or performance degradation. In this blog post, we will explore how to prevent inadvertent recursion in Java by utilizing the ThreadLocal
feature.
Understanding Recursion and Its Pitfalls
Recursion happens when a method calls itself in order to solve a smaller instance of the same problem. While recursion can lead to elegant solutions, it can also introduce complications.
Why Use Recursion?
- Simplicity: Recursive solutions often lead to cleaner, more concise code.
- Natural Fit for Certain Problems: Problems such as tree traversals, factorial calculations, and the Fibonacci sequence are naturally expressed in recursive form.
However, recursive methods can quickly spiral out of control. This can happen often in multi-threaded applications where methods are invoked on different threads without appropriate checks.
The Risks of Inadvertent Recursion
When more than one thread accesses the same method that calls itself, you risk:
- Stack Overflow: Each recursive call consumes stack space. If too many calls stack up, your application will crash.
- Performance Issues: Excess recursion can degrade performance, consuming CPU cycles and memory.
Understanding these potential issues is the first step toward building safer applications.
Introducing ThreadLocal
Java provides a class called ThreadLocal
that allows you to create variables that are local to a thread. Each thread accessing a variable holds its own, independently initialized copy of the variable.
Why Use ThreadLocal?
- Isolation: Each thread can have a unique value for a variable.
- Thread-Safety: Since each thread has its copy, you can avoid race conditions.
How to Use ThreadLocal to Prevent Inadvertent Recursion
To prevent inadvertent recursion, you can employ a ThreadLocal
variable to track whether a method is currently being executed by the thread. Let's look at a basic implementation.
Basic Usage Example
public class RecursiveMethodHandler {
private static final ThreadLocal<Boolean> isRecursionActive = ThreadLocal.withInitial(() -> false);
public void safeRecursiveMethod(int n) {
// Check if recursion is already active for this thread
if (isRecursionActive.get()) {
throw new IllegalStateException("Recursive call detected!");
}
isRecursionActive.set(true); // Set the flag to indicate entry into the method
try {
if (n <= 0) {
return;
}
System.out.println("Processing: " + n);
safeRecursiveMethod(n - 1); // Recursive call
} finally {
isRecursionActive.remove(); // Always clear the ThreadLocal variable
}
}
}
Commentary on the Code
- ThreadLocal Initialization: The
ThreadLocal
is initialized tofalse
, indicating no active recursion initially. - Recursion Check: Before entering the recursive method, check if recursion is already active by examining the
ThreadLocal
variable. - Setting the Flag: If not active, set the flag to
true
and proceed with the recursion. - Try-Finally Block: It's crucial to ensure that the
ThreadLocal
variable is cleared after the method execution to avoid memory leaks and incorrect checks on subsequent method calls.
This setup ensures that if the method is invoked recursively from the same thread, an exception will be thrown, thereby preventing further recursive calls.
Additional Best Practices
1. Limit Maximum Depth
While ThreadLocal
helps avoid inadvertent recursion, consider implementing a maximum recursion depth to enhance safety.
private static int maxDepth = 10;
private static ThreadLocal<Integer> depthCounter = ThreadLocal.withInitial(() -> 0);
public void depthLimitedMethod(int n) {
if (depthCounter.get() >= maxDepth) {
throw new StackOverflowError("Maximum recursion depth reached!");
}
depthCounter.set(depthCounter.get() + 1);
try {
// Your logic here...
depthLimitedMethod(n - 1);
} finally {
depthCounter.set(depthCounter.get() - 1); // Decrease counter
}
}
2. Use of Logs
When debugging recursive methods, proper logging can provide insights into the flow of execution. Use a logger to track method entries and exits.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
private static final Logger logger = LoggerFactory.getLogger(RecursiveMethodHandler.class);
public void enhancedLoggingMethod(int n) {
logger.info("Entering method with value: {}", n);
// Method logic...
logger.info("Exiting method with value: {}", n);
}
Closing Remarks
Inadvertent recursion can be a programming pitfall that leads to significant errors within an application. By leveraging ThreadLocal
, Java developers can effectively manage recursion, ensuring that methods do not call themselves unexpectedly in a multithreaded environment.
This technique not only allows for safer code but also ensures that developers can utilize recursion elegantly without compromising application performance.
For further reading on Java's concurrency utilities, check out the official Java documentation here: Java Concurrency API.
By maintaining clear code and adopting best practices, you can navigate the complexities of recursion while enhancing your application's stability and performance. Happy coding!
Checkout our other articles