Resolving JSON Serialization Issues in WildFly 8.0 JAX-RS
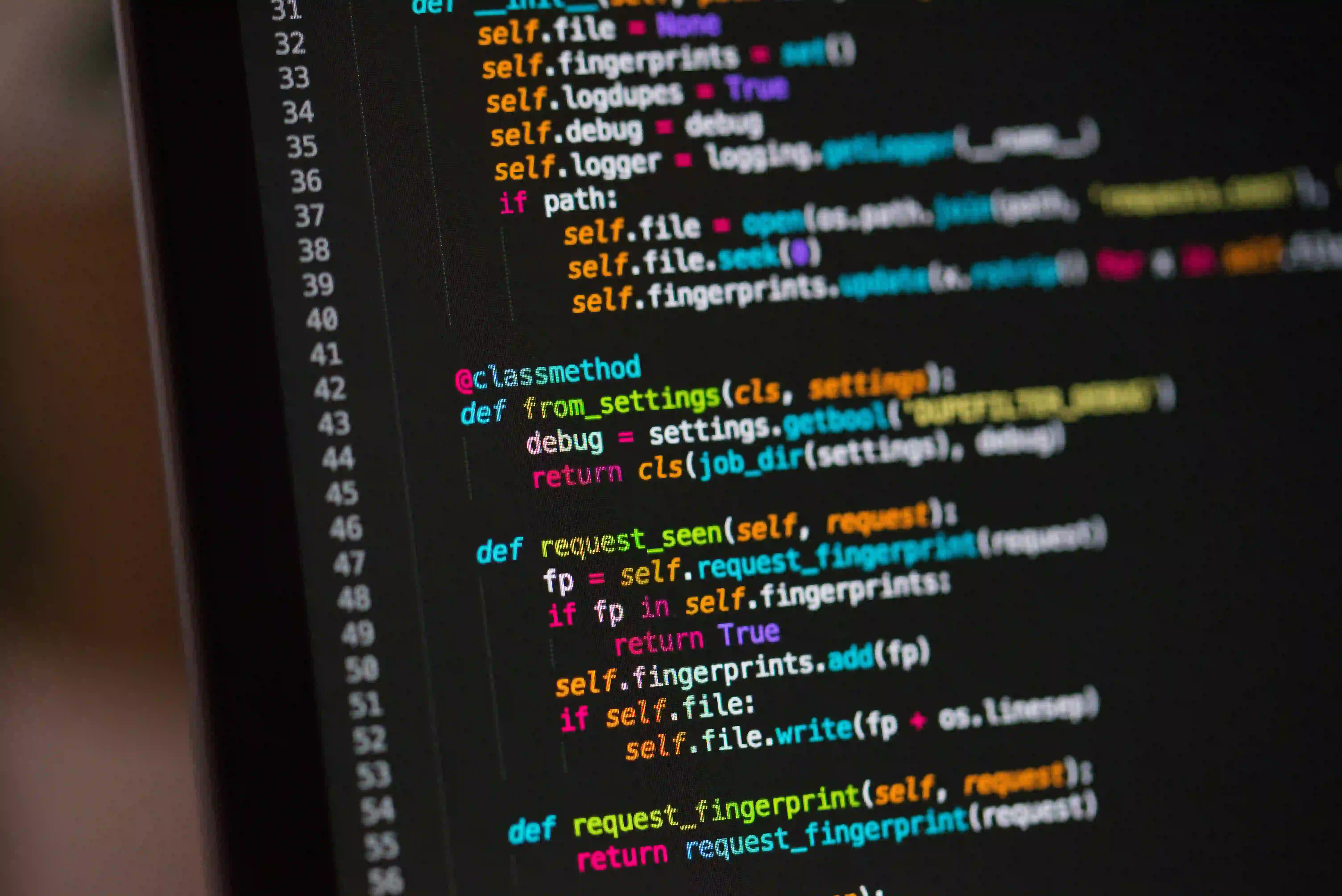
Resolving JSON Serialization Issues in WildFly 8.0 JAX-RS
In the world of Java web applications, JSON serialization is a critical aspect for effective client-server communication. When developing services using the JAX-RS framework on WildFly 8.0, one might encounter various JSON serialization issues. This post addresses common problems and provides solutions to enhance your JSON handling in WildFly.
Understanding JSON Serialization
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. In a Java context, serialization is the process of converting an object into a JSON format, enabling data exchange across different technology stacks.
Importance of Correct JSON Serialization
Improper serialization can lead to several issues:
- Data Loss: Incorrect serialization may omit crucial data.
- Performance Degradation: Inefficient serialization can slow down your application.
- Incompatibility: Different versions of entities can lead to issues when they are serialized.
With that said, let’s explore how to tackle some common JSON serialization issues in WildFly 8.0.
Common JSON Serialization Issues
Here are some frequent challenges developers face:
- Incorrect Content-Type Headers
- Circular References
- Date Formatting Issues
- Field Exclusion Problems
Issue #1: Incorrect Content-Type Headers
When returning JSON responses, the specified content type is vital. If not set correctly, clients may not interpret the response as JSON.
Solution
You can specify the content type in your JAX-RS resource method as shown below:
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
@Path("/example")
public class ExampleResource {
@GET
@Produces(MediaType.APPLICATION_JSON)
public MyData getExampleData() {
return new MyData("Sample", 123);
}
}
Why this works: The @Produces(MediaType.APPLICATION_JSON)
annotation explicitly states that the output should be in JSON format, ensuring the client receives and interprets the data correctly.
Issue #2: Circular References
Circular references can easily lead to StackOverflowError
during serialization, particularly when entities reference each other.
Solution
To combat this, you can use @JsonIgnore
from the Jackson library to ignore certain fields:
import com.fasterxml.jackson.annotation.JsonIgnore;
public class User {
private String username;
@JsonIgnore
private Profile profile; // Avoid circular reference
// Getters and Setters
}
Why this works: By ignoring the profile
field, the serializer prevents recursion during the serialization process, thus avoiding errors.
Issue #3: Date Formatting
JSON serialization often struggles with date fields, leading to undesirable date formats that are not user-friendly.
Solution
To specify a format for date serialization, you can use the @JsonFormat
annotation:
import com.fasterxml.jackson.annotation.JsonFormat;
import java.util.Date;
public class Event {
private String name;
@JsonFormat(shape = JsonFormat.Shape.STRING, pattern = "yyyy-MM-dd HH:mm:ss")
private Date eventDate;
// Getters and Setters
}
Why this works: This annotation controls how the date is represented in JSON, preventing ambiguity and ensuring proper formatting for client-side handling.
Issue #4: Field Exclusion Problems
Sometimes, you may want to exclude specific fields from being serialized based on certain conditions.
Solution
This can be achieved through the @JsonInclude
annotation:
import com.fasterxml.jackson.annotation.JsonInclude;
public class UserProfile {
private String username;
@JsonInclude(JsonInclude.Include.NON_NULL)
private String bio; // Will be excluded if null
// Getters and Setters
}
Why this works: By using NON_NULL
, the serializer will omit any field with a null
value, thus generating cleaner JSON.
Integrating with WildFly 8.0
To ensure everything works smoothly with WildFly 8.0, thorough integration checks are essential. The server comes bundled with JAX-RS capabilities, but you may want to verify that your application is configured properly with the right libraries.
Make sure to include the Jackson library in your pom.xml
if you are using Maven for dependency management:
<dependency>
<groupId>com.fasterxml.jackson.jaxrs</groupId>
<artifactId>jackson-jaxrs-json-provider</artifactId>
<version>2.7.9</version>
</dependency>
Why this matters: Jackson is the default JSON provider for JAX-RS. Including it ensures that your application can serialize Java objects into JSON format effortlessly.
Best Practices for JSON Serialization in WildFly 8.0
-
Consistency in Annotations: Always ensure that you are using a consistent set of annotations based on the libraries in play (for example, Jackson).
-
Consistent API Responses: Strive to maintain a consistent response structure across your API endpoints. This makes them predictable and easier to handle on the client side.
-
Exception Handling: Implement robust error handling to provide clear messages when serialization fails. Use the
ExceptionMapper
interface for catching serialization exceptions gracefully. -
Regular Testing: Unit tests are vital for validating JSON serialization logic. Use frameworks such as JUnit combined with mock libraries like Mockito to simulate various scenarios.
Key Takeaways
Understanding and resolving JSON serialization issues in WildFly 8.0 using JAX-RS can dramatically improve the performance and reliability of your applications. By adhering to best practices and utilizing annotations effectively, you can achieve a smooth and error-free communication channel between your server and clients.
For further reading, consider exploring JAX-RS specification, and the Jackson documentation, which provides in-depth insights into effective JSON handling.
By employing these strategies, developers can streamline their backend processes, ensuring a better experience for end-users and maintaining the integrity and efficiency of their applications.