Navigating JEP 181: Challenges with Nesting Classes
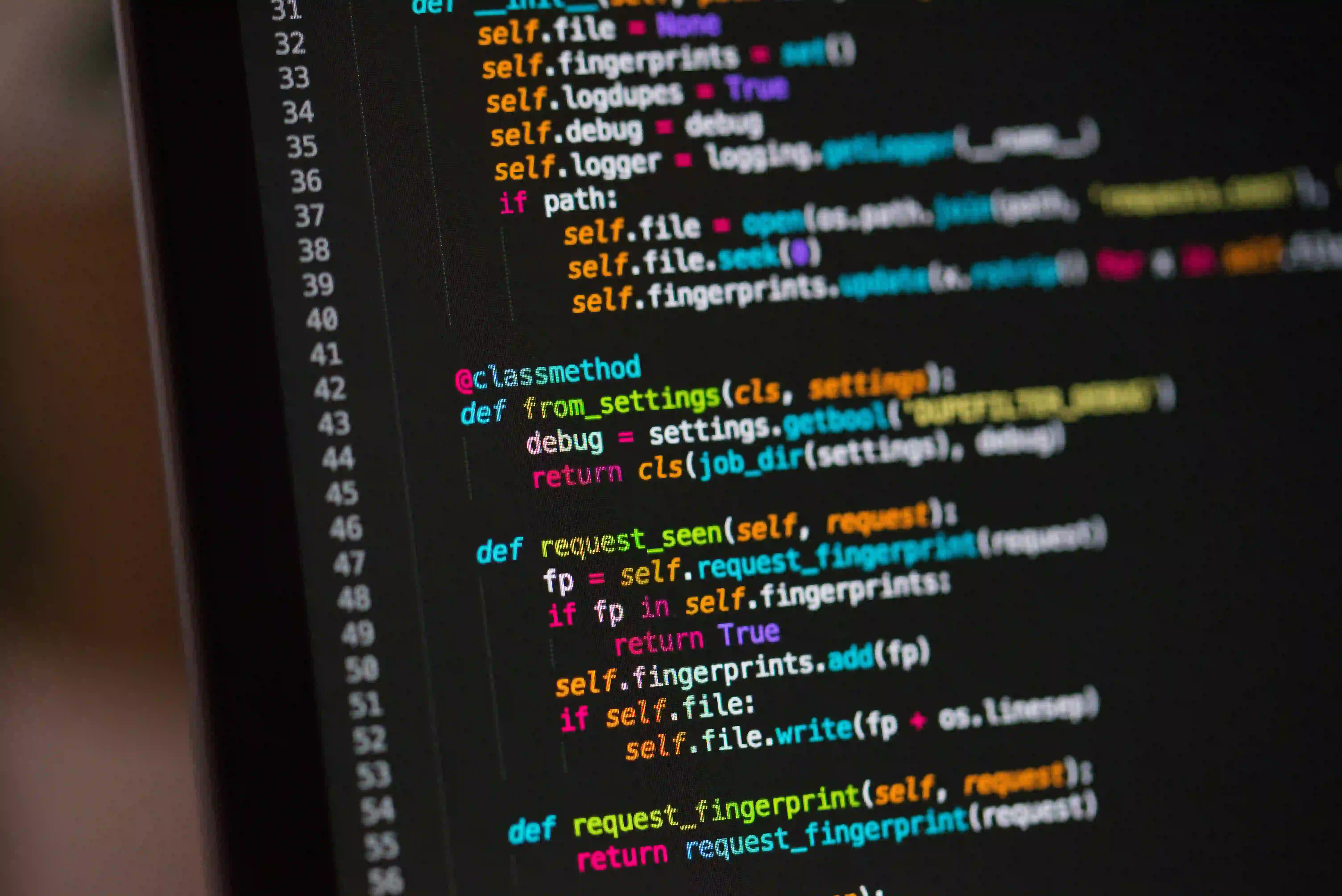
Navigating JEP 181: Challenges with Nesting Classes
Java, a language widely revered for its robustness and versatility, continues to evolve, adjusting to the needs of modern programming paradigms. One recent advancement that has stirred considerable discussion is JEP 181: Nest-Based Access Control. In this blog post, we will delve into the implications of this enhancement, discuss the challenges associated with nesting classes, and explore how JEP 181 addresses them.
Understanding Nest-Based Access Control
Way back in Java 8, the advent of lambdas created a new realm of flexibility in coding. However, this also led to structural complications, primarily regarding access control. Prior to JEP 181, the Java language primarily supported 'static' inner classes. With evolving needs, developers sought a solution that would make handling nested classes more robust and intuitively aligned with object-oriented principles.
JEP 181 introduces an elegant solution: nest-based access control. This means that nested classes, including non-static inner classes and enums, can access each other's private members even if they aren't in the same class file. This dramatically improves flexibility, leads to cleaner code, and reduces boilerplate procedures.
The Challenge of Nesting Classes
While nesting classes provides utility, it introduces complexity. Here are some challenges that developers often encounter:
1. Increased Complexity
Nesting classes can lead to confusion. When a class is defined within another, it is sometimes unclear which classes have access to which members. This can lead to inadvertent accessibility issues: classes that shouldn't access certain members may inadvertently gain access, leading to potential security risks.
2. Readability Issues
Code readability is crucial in software development. When nesting becomes rampant, it can make understanding the structure of a program difficult. Developers finding themselves deep within nested classes may struggle to track relationships and access points.
3. Maintainability Concerns
In a large codebase, maintaining stable access and understanding dependencies becomes cumbersome. Nested classes can lead to tightly coupled designs, making it tough to modify or extend code without impacting other components of the application.
Code Snippet: The Traditional Approach
Before JEP 181, handling access to private members among nested classes required careful structuring. Here’s a basic example of what traditional nested classes looked like:
public class Outer {
private String outerField = "I am outer!";
class Inner {
void display() {
System.out.println(outerField); // Accessing outer class member
}
}
class AnotherInner {
void show() {
System.out.println(outerField); // Still can access
}
}
public static void main(String[] args) {
Outer outer = new Outer();
Inner inner = outer.new Inner();
Inner2 anotherInner = outer.new AnotherInner();
inner.display();
anotherInner.show();
}
}
Commentary
In this example, Inner
and AnotherInner
can access outerField
. This works well, but as the application scales, the dependency on the Outer
class may lead to tight coupling. If we need to refactor Outer
, we may inadvertently break other parts of the code.
The Benefits of JEP 181
With the introduction of JEP 181, the rules around access control among nested classes become more audacious, ensuring better clarity as well as ease of maintenance. The access modifier for nested classes is redesigned to offer new insights on access privileges.
Code Snippet: Utilizing Nest-Based Access Control
Here's a modern approach using nest-based access control defined in JEP 181:
public class Outer {
private String outerField = "I am outer!";
class Inner {
void display() {
System.out.println(outerField);
}
}
class AnotherInner {
void show(Inner inner) {
// Direct access to inner's method
inner.display();
}
}
public static void main(String[] args) {
Outer outer = new Outer();
Inner inner = outer.new Inner();
AnotherInner anotherInner = outer.new AnotherInner();
anotherInner.show(inner); // Passing inner instance
}
}
What Changed?
In the code above, we defined a method in AnotherInner
that takes an Inner
instance, thereby allowing indirect access to outerField
. The beauty lies in new possible instructions that JEP 181 enables; we can establish tighter configurations for nested classes without losing encapsulation.
Navigating the Transition
Transitioning to the benefits of JEP 181 might feel daunting. Here are some strategies and best practices for developers:
Embrace Nesting Judiciously
While nesting can be powerful, don’t use it indiscriminately. Carefully consider whether a nested class is truly needed. Favor keeping classes independent when possible.
Maintain Content Clarity
Name your nested classes clearly. Ensure that their purpose is evident to anyone reading the code, aiding in understanding and maintainability.
Document Relationships
In case nesting is utilized, ensure to document why a class is nested within another. Clear documentation can ease troubleshooting later down the line.
Leverage Access Modifiers Wisely
Utilize appropriate access modifiers for methods and fields. This ensures restrictiveness in case the structure must be changed or if unintended accessibility creeps in.
Closing Remarks
JEP 181 symbolizes a significant stride toward enhancing Java’s handling of nested classes. By addressing the challenges associated with nesting while embracing more intuitive access control mechanisms, it streamlines core principles of Java, making the language more efficient and powerful.
As you embrace these advancements in your Java projects, remember the balance of flexibility with clarity. Aim for a codebase that reflects both the power of nesting classes and the fragility of poorly managed access control.
For further details on JEP 181, check out the official documentation.
Happy coding!