Mastering Java Developer Tools: Avoiding Common Pitfalls
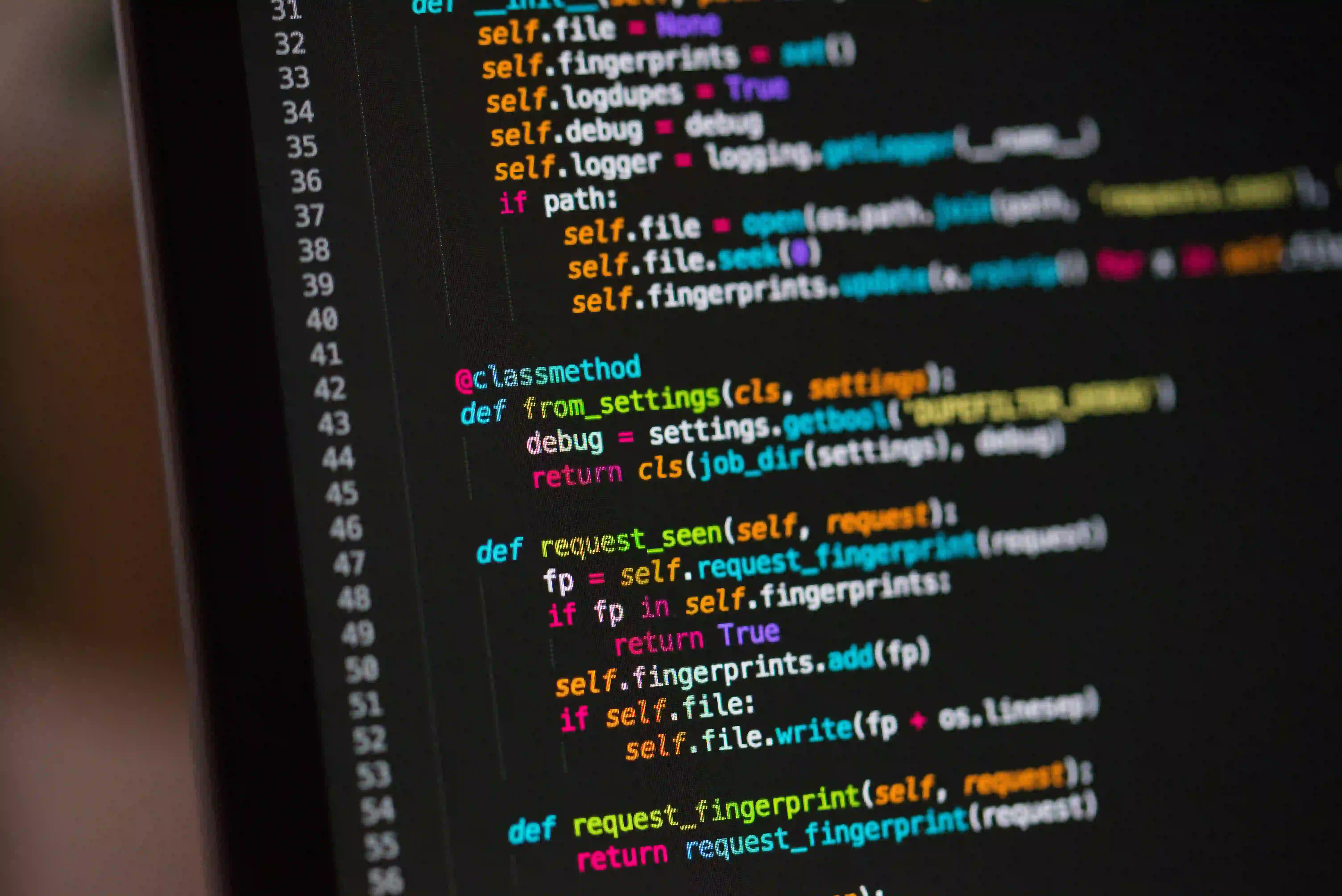
Mastering Java Developer Tools: Avoiding Common Pitfalls
Java, as one of the most versatile and widely-used programming languages, continues to be a top choice for developers around the globe. Part of this popularity stems from the robust ecosystem of tools available to streamline the development process. However, while these tools can enhance productivity and facilitate best practices, they can also lead to common pitfalls if misused.
In this blog post, we will explore some essential Java developer tools, highlight common mistakes developers make, and offer actionable insights to avoid these pitfalls. Whether you are a seasoned developer or just starting, enhancing your understanding of Java developer tools is crucial to your growth.
Understanding Java Developer Tools
Before we dive into specific pitfalls, it's essential to understand what we mean by "Java developer tools." These tools encompass a wide range of software designed to aid developers in writing, testing, debugging, and maintaining Java applications. Key categories include:
-
Integrated Development Environments (IDEs): Tools such as IntelliJ IDEA, Eclipse, and NetBeans provide a user-friendly interface for coding, debugging, and managing Java projects.
-
Build Tools: Maven and Gradle help manage project dependencies, compile source code, and create deployment packages.
-
Version Control Systems: Git, coupled with platforms like GitHub and Bitbucket, allows developers to track changes in the codebase collaboratively.
-
Testing Frameworks: JUnit and TestNG are essential for writing unit tests, ensuring code reliability and quality.
Common Pitfalls in Java Development Tools
1. Over-Reliance on IDE Features
The Pitfall: Many developers depend heavily on the features provided by their IDEs for code completion, refactoring, and error detection.
Why It's a Problem: While IDEs provide tremendous help, over-reliance can lead to poor code quality. Developers might skip understanding the underlying mechanics of the code and rely solely on automated suggestions.
Solution: Embrace the IDE features while retaining your understanding of Java fundamentals. For example, when using IntelliJ IDEA for code suggestions, take the time to learn why a certain refactoring is recommended.
// Example of a simple refactoring in IntelliJ IDEA
public class User {
private String username;
public User(String username) {
this.username = username;
}
// This method returns the username.
public String getUsername() {
return username;
}
}
In this case, if your IDE suggests renaming getUsername
to fetchUsername
, consider the implications of this naming change on your code's readability and consistency.
2. Ignoring Build Tool Configuration
The Pitfall: Many beginners treat build tools like Maven and Gradle as mere facilitators for compiling code. This often results in ignoring necessary configurations.
Why It's a Problem: Misconfigurations can lead to dependency clashes, resulting in runtime errors and additional debugging time. For instance, not properly specifying the Java version in the pom.xml
can lead to compatibility issues.
Solution: Always ensure your build configuration is verbose and well-documented. Here’s a sample configuration for Maven:
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>myapp</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>17</maven.compiler.source>
<maven.compiler.target>17</maven.compiler.target>
</properties>
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter</artifactId>
<version>5.7.0</version>
<scope>test</scope>
</dependency>
</dependencies>
</project>
In this example, we specify the Java version, ensuring our application remains compatible with the Java ecosystem.
3. Neglecting Version Control Best Practices
The Pitfall: New developers often underestimate the importance of version control hygiene, such as writing meaningful commit messages or managing branches.
Why It's a Problem: Poor version control practices can lead to lost progress, tangled code changes, and difficult collaboration. Developers may find themselves in a complicated merge conflict that could have been avoided.
Solution: Develop a consistent version control strategy. Always write detailed commit messages:
Fix: Corrected login validation logic to prevent invalid access
Additionally, maintain a clean branching strategy, such as using feature branches for new developments.
Learn more about Git best practices on Atlassian.
4. Failing to Write Tests
The Pitfall: Many developers put off writing tests due to time constraints, believing they can “test” the code manually.
Why It's a Problem: Without tests, it’s challenging to pinpoint bugs, and if bugs arise, fixing them could disrupt other features. Moreover, this practice hampers the project's longevity.
Solution: Adopt Test-Driven Development (TDD). Writing tests before your code can help ensure that the functionality you build meets the specified requirements upfront.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class UserTest {
@Test
public void testGetUsername() {
User user = new User("john123");
assertEquals("john123", user.getUsername(), "Username should match.");
}
}
In this example, the unit test verifies the getUsername
method works as expected, enhancing code reliability.
5. Not Keeping Up With Tool Updates
The Pitfall: Technology evolves rapidly, and neglecting updates to Java tools can lead to performance issues and security vulnerabilities.
Why It's a Problem: Using outdated tools can result in missing new features, performance improvements, and critical security patches. This can seriously affect your project's stability.
Solution: Regularly check for updates from your development environment, build tools, and frameworks. Follow release notes and explore new features:
- IntelliJ IDEA: Check out the latest features here.
- Maven: Stay updated with Maven releases.
Lessons Learned
Mastering Java development tools involves understanding their capabilities and avoiding common pitfalls. As we’ve discussed, from over-reliance on IDEs to neglecting testing, developers can face significant hurdles if they don’t approach tool usage with care.
By implementing the solutions provided, you can enhance your productivity, improve code quality, and ultimately develop more robust Java applications. Keep learning, stay updated on resources, and remember that mastery in software development is a continuous journey.
Happy coding!