Streamlining A/B Testing in Java with Feature Flags
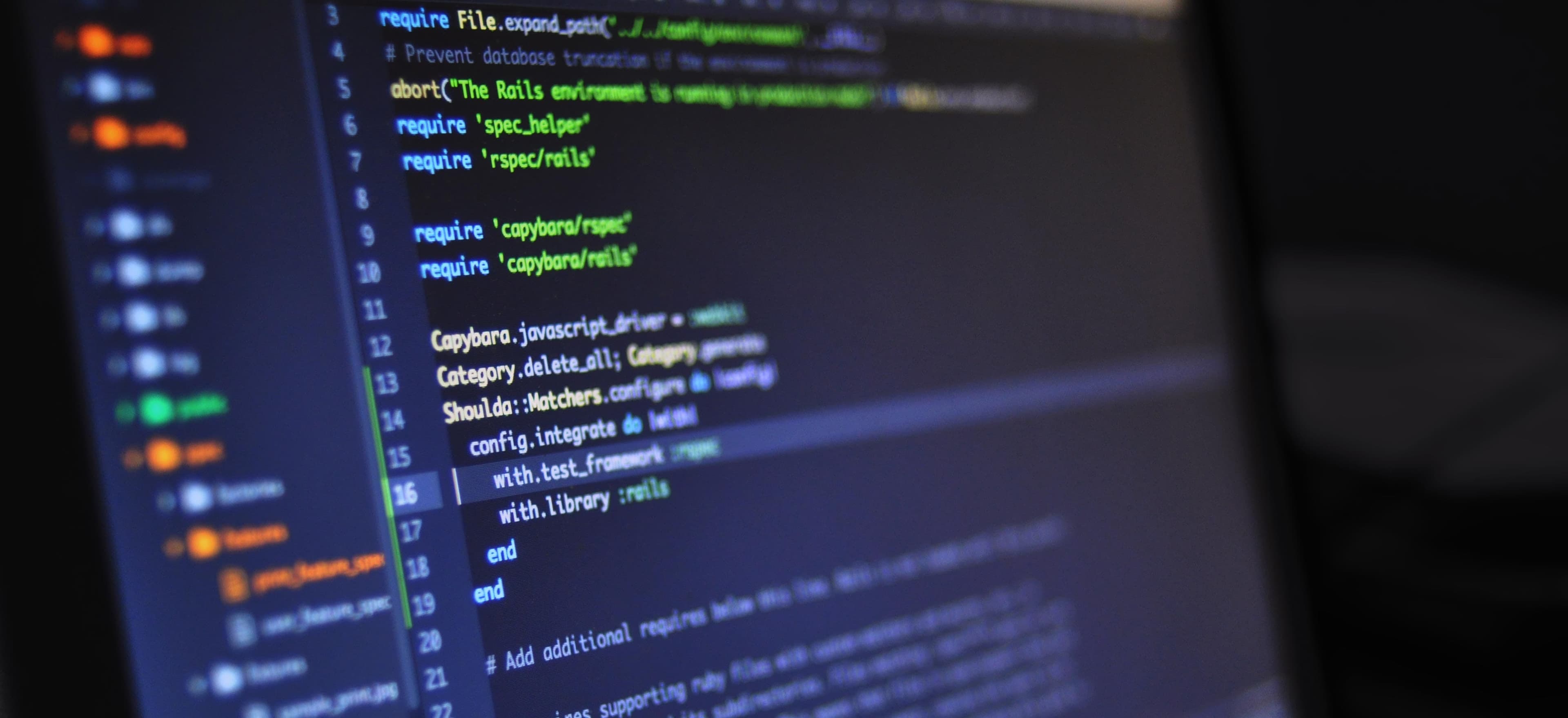
- Published on
Streamlining A/B Testing in Java with Feature Flags
A/B testing is a critical component of modern software development. It allows teams to test different variations of a feature and determine which one performs better based on user interactions. As we dive into this topic, we'll specifically look at how you can streamline A/B testing in Java applications using feature flags.
Feature flags allow developers to enable or disable specific features in their codebase without deploying new versions of the application. This flexibility is invaluable in A/B testing scenarios where you need to assess user behavior effectively. For a deeper understanding of A/B testing principles, consider checking out the article titled Optimizing A/B Testing with Feature Flag Configuration.
What are Feature Flags?
Feature flags, often called feature toggles, are a software development technique that allows you to turn features on or off dynamically. By wrapping features in condition checks, you gain better control over your application's behavior. They also facilitate smoother releases and can bridge the gap between development and deployment.
Why Use Feature Flags in A/B Testing?
- Flexibility: You can toggle features without needing a full deployment.
- Gradual Rollouts: Slowly expose new features to subsets of users to monitor performance.
- User Segmentation: Enable features for specific user groups based on criteria.
- Quick Experimentation: Rapidly test different variations of features with minimal risk.
Implementing Feature Flags in Java
Let's consider a simple Java application where we want to test two versions of a new welcome message. We will use a feature flag to control which message is displayed.
Step 1: Define Feature Flags
You can leverage a simple enum to define your feature flags. This can help maintain readability in your code.
public enum FeatureFlag {
NEW_WELCOME_MESSAGE,
OLD_WELCOME_MESSAGE
}
Step 2: Create a Feature Flag Manager
Next, implement a class responsible for managing the feature flags. You could use a simple HashMap for storing the current state of each flag.
import java.util.HashMap;
import java.util.Map;
public class FeatureFlagManager {
private Map<FeatureFlag, Boolean> featureFlags;
public FeatureFlagManager() {
featureFlags = new HashMap<>();
// Default all flags to false
for (FeatureFlag flag : FeatureFlag.values()) {
featureFlags.put(flag, false);
}
}
public void setFlag(FeatureFlag featureFlag, boolean status) {
featureFlags.put(featureFlag, status);
}
public boolean isFlagEnabled(FeatureFlag featureFlag) {
return featureFlags.getOrDefault(featureFlag, false);
}
}
Why This Approach?
By using a separate manager, you keep your feature flag logic abstracted from the main business logic. This makes it easier to manage, test, and extend.
Step 3: Utilizing Feature Flags in Code
We will use the FeatureFlagManager
in our application to decide which welcome message to display.
public class WelcomeService {
private FeatureFlagManager featureFlagManager;
public WelcomeService(FeatureFlagManager featureFlagManager) {
this.featureFlagManager = featureFlagManager;
}
public String getWelcomeMessage() {
if (featureFlagManager.isFlagEnabled(FeatureFlag.NEW_WELCOME_MESSAGE)) {
return "Welcome to our new exciting platform!";
}
return "Welcome to our platform!";
}
}
Explaining the Logic
In the getWelcomeMessage
method, we check whether the NEW_WELCOME_MESSAGE
flag is enabled. If it is, we return the new welcome message; otherwise, we fall back to the old message. This keeps our code clean and easy to read.
Step 4: Testing the Implementation
You can quickly test the implementation by simulating different flag states.
public class Main {
public static void main(String[] args) {
FeatureFlagManager flagManager = new FeatureFlagManager();
WelcomeService welcomeService = new WelcomeService(flagManager);
// Default message
System.out.println(welcomeService.getWelcomeMessage());
// Enable the new message
flagManager.setFlag(FeatureFlag.NEW_WELCOME_MESSAGE, true);
System.out.println(welcomeService.getWelcomeMessage());
}
}
Output Explanation
The expected output of the above code would be:
Welcome to our platform!
Welcome to our new exciting platform!
This shows how easily we can control the flow of information based on the feature flag's state.
Best Practices for Feature Flag Management
- Document Flags: Set clear documentation for each feature flag to minimize confusion among team members.
- Prioritize Cleanup: Periodically review and remove obsolete flags to keep your codebase tidy.
- Use a Configuration System: Consider integrating a dedicated configuration service to manage feature flags effectively, especially as the application scales.
- Monitor and Analyze: Implement monitoring to analyze the impact of toggling feature flags.
Advance: Feature Flags with User Segmentation
For more sophisticated A/B testing scenarios, you may experiment with user segmentations, enabling features based on user demographics, behavior, or any such measure.
Sample Segmentation Based on User ID
import java.util.UUID;
public class WelcomeService {
// ... Other methods remain unchanged
public String getWelcomeMessage(UUID userId) {
if (shouldShowNewMessage(userId)) {
return "Welcome to our new exciting platform!";
}
return "Welcome to our platform!";
}
private boolean shouldShowNewMessage(UUID userId) {
// Simple example: show the new message to even user ID only
return userId.hashCode() % 2 == 0 && featureFlagManager.isFlagEnabled(FeatureFlag.NEW_WELCOME_MESSAGE);
}
}
Where to Go from Here?
Using feature flags in A/B testing enhances your ability to innovate and experiment with minimal risk. You can adapt the outlined approach based on the complexity of your application or the specifics of your feature requirement. For more insights into optimizing this process, refer back to the aforementioned article Optimizing A/B Testing with Feature Flag Configuration.
The Bottom Line
Incorporating feature flags into your Java applications not only simplifies A/B testing but also enriches the overall development process. They empower teams to iterate faster, safer, and more effectively capture user insights. By maintaining clean, organized, and efficient feature flag logic, you can foster a culture of experimentation and continuous improvement in your development practices.
Start implementing feature flags in your system today and experience the difference in your A/B testing initiatives. With the right setup, you can continue to enhance your application's user experience and drive more informed decision-making.
Checkout our other articles