Simplifying Java Projects: Dependency Injection Essentials
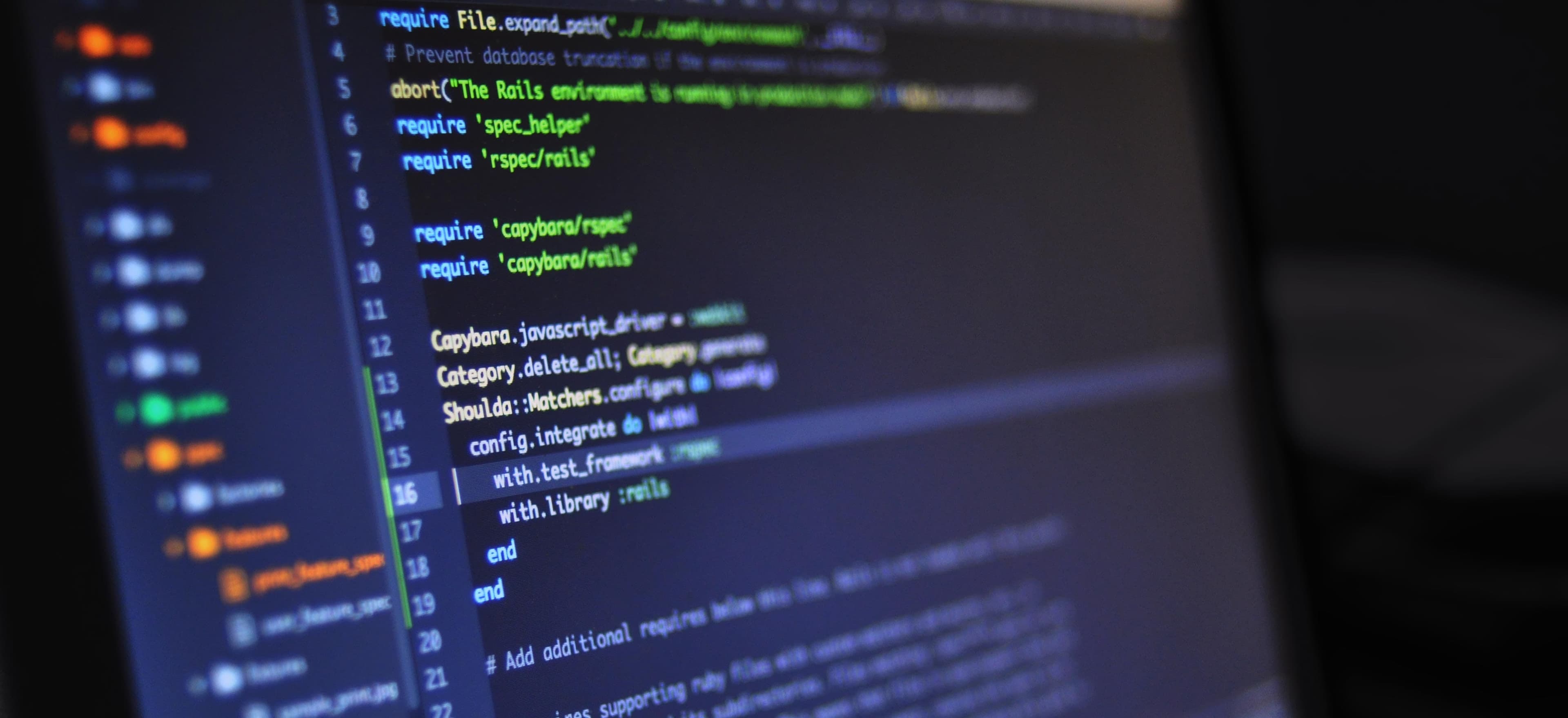
- Published on
Simplifying Java Projects: Dependency Injection Essentials
In the realm of software development, maintaining clean, modular code is paramount. With the rise of frameworks and libraries, one technique that has gained significant traction is Dependency Injection (DI). This blog post will delve into the essentials of Dependency Injection in Java, illustrating how it can simplify your projects. Notably, we will reference the useful insights offered in Streamline Your Code: Mastering Dependency Injection with StructureMap.
What is Dependency Injection?
Dependency Injection is a design pattern that promotes loose coupling and enhances code maintainability. Simply put, DI allows a class to receive its dependencies from an external source rather than creating them internally. This results in code that is easier to test, maintain, and extend.
Why Use Dependency Injection?
- Decoupling: Classes are not tightly bound to their dependencies, which allows for more flexible code design.
- Enhanced Testability: By using mock objects, you can test classes in isolation without needing their actual dependencies.
- Simplified Configuration: DI frameworks manage the creation and assembly of objects, streamlining application setup.
Key Concepts of Dependency Injection
Before we jump into code examples, let's clarify some core concepts of DI:
- Inversion of Control (IoC): A principle where the control of object creation is passed from the application code to a DI framework.
- Service: An object that performs a task or carries out business logic.
- Injector: The subsystem that constructs the services and injects them into dependent classes.
Types of Dependency Injection
There are several ways to implement DI in Java:
- Constructor Injection: Dependencies are provided through a class constructor.
- Setter Injection: Dependencies are set via setter methods.
- Interface Injection: The dependency provides an injector method that will inject the dependency into any client that passes the injector.
Now, let's explore these types with code examples.
Constructor Injection
Constructor injection is the most commonly used form of DI. By using constructor parameters, you clearly define what a class needs to function.
class MessageService {
public void sendMessage(String message) {
System.out.println("Sending message: " + message);
}
}
class UserNotification {
private final MessageService messageService;
// Constructor Injection
public UserNotification(MessageService messageService) {
this.messageService = messageService;
}
public void notifyUser(String message) {
messageService.sendMessage(message);
}
}
Why Constructor Injection?
Using constructor injection enforces compile-time checking of dependencies. This means that if any dependency is missing, the code won’t compile, leading to early error detection.
Setter Injection
Setter injection allows developers to change dependencies after the object has been constructed.
class MessageService {
public void sendMessage(String message) {
System.out.println("Sending message: " + message);
}
}
class UserNotification {
private MessageService messageService;
// Setter Injection
public void setMessageService(MessageService messageService) {
this.messageService = messageService;
}
public void notifyUser(String message) {
if (messageService == null) {
throw new IllegalStateException("MessageService not set");
}
messageService.sendMessage(message);
}
}
Why Setter Injection?
Setter injection is suitable when the dependency is optional or can be changed after object creation. However, it can lead to runtime errors if a method is called before setting the dependency.
Interface Injection
Interface injection is less common but offers a flexible approach by defining an injector in the dependency itself.
interface MessageServiceInjector {
void injectMessageService(UserNotification userNotification);
}
class MessageServiceInjectorImpl implements MessageServiceInjector {
@Override
public void injectMessageService(UserNotification userNotification) {
userNotification.setMessageService(new MessageService());
}
}
// Client code
public class Main {
public static void main(String[] args) {
UserNotification userNotification = new UserNotification();
MessageServiceInjector injector = new MessageServiceInjectorImpl();
injector.injectMessageService(userNotification);
// Now we can use UserNotification
userNotification.notifyUser("Hello User!");
}
}
Why Interface Injection?
While less common, interface injection can offer high flexibility, especially in complex systems with multiple dependencies. However, it may also lead to more boilerplate code.
Benefits of Using DI Frameworks
The above examples demonstrate manual dependency management. However, as project complexity grows, handling dependencies manually can become cumbersome. Here, DI frameworks like Spring, Guice, or Dagger shine.
Why Use a DI Framework?
- Automation: Frameworks automate the process of instantiating classes and resolving their dependencies.
- Configuration Management: They often provide XML or annotation-based configurations, allowing for dynamic substitution of classes.
- AOP Support: Most DI frameworks include support for Aspect-Oriented Programming (AOP), allowing separation of cross-cutting concerns.
Example with Spring Framework
Let’s see how Spring simplifies DI.
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
@Configuration
class AppConfig {
@Bean
public MessageService messageService() {
return new MessageService();
}
@Bean
public UserNotification userNotification() {
return new UserNotification(messageService());
}
}
public class Main {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
UserNotification userNotification = context.getBean(UserNotification.class);
userNotification.notifyUser("Hello from Spring!");
}
}
Why Use Spring?
Spring handles the complexity of DI by managing the lifecycle of objects and their dependencies. This allows developers to focus on business logic rather than object management.
Choosing the Right DI Strategy
Selecting the most suitable DI method depends on several factors:
- Complexity: For simple projects, constructor injection may suffice. For larger systems, a DI framework could be more advantageous.
- Flexibility: If you anticipate changes in your dependencies, setter or interface injection might be beneficial.
- Testing: If testability is a priority, prefer constructor injection to ensure dependencies are explicit and required.
Closing Remarks
Dependency Injection is a powerful pattern that can significantly simplify your Java projects. By reducing coupling and enhancing testability, DI lays the groundwork for a more maintainable and flexible codebase. If you're looking to dive deeper into DI and structure, make sure to explore the intricacies of frameworks like StructureMap as discussed in Streamline Your Code: Mastering Dependency Injection with StructureMap.
By implementing DI effectively, you can elevate your Java applications to new heights of clarity and efficiency. Happy coding!