Java Solutions for Efficiently Managing Large Datasets
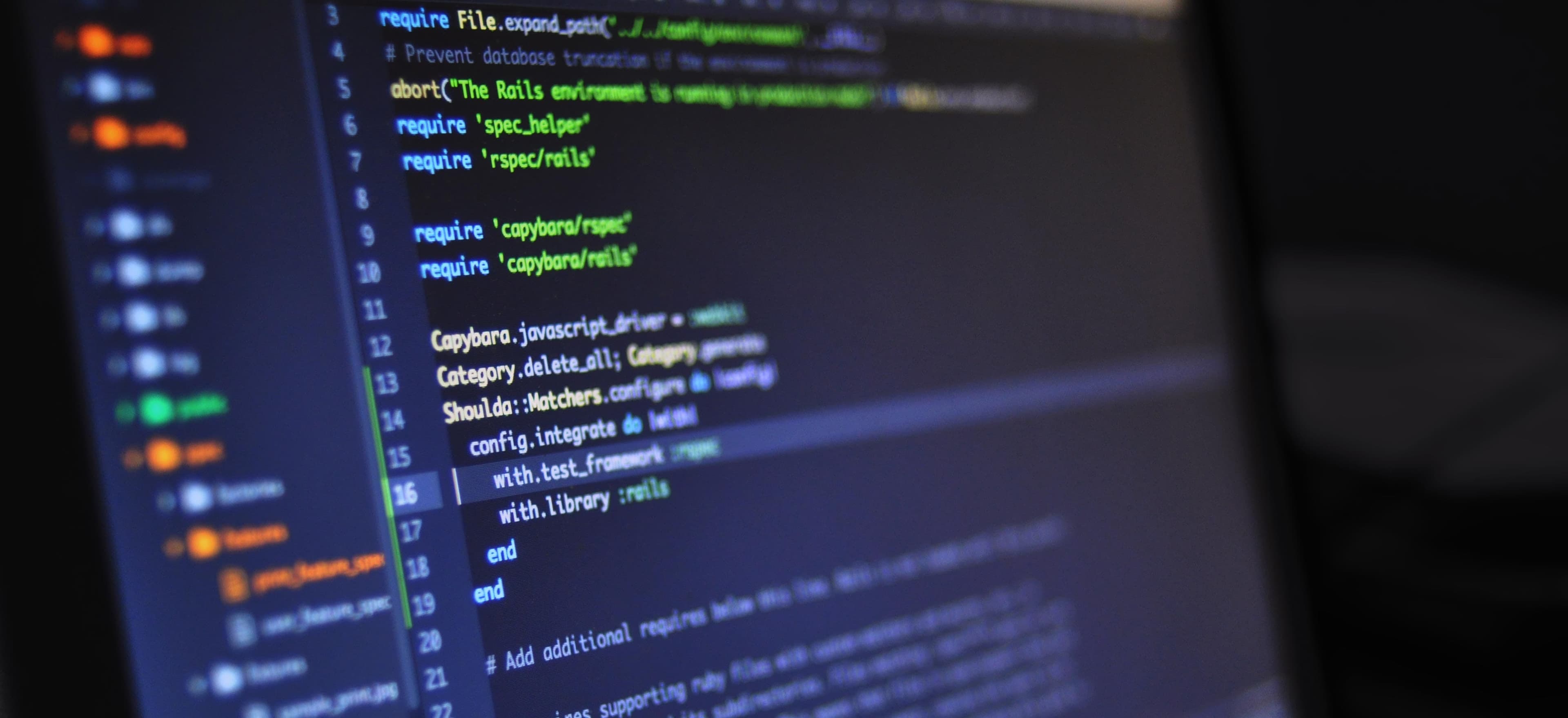
- Published on
Java Solutions for Efficiently Managing Large Datasets
In today's data-driven world, efficiently managing large datasets is paramount for developers, data scientists, and businesses alike. With the exponential growth of data, tools and frameworks to manage and manipulate these datasets become essential. In this blog post, we will explore how to use Java to efficiently manage large datasets, drawing from existing SQL techniques. For a deeper understanding of database optimization, check out the article titled Managing Large Datasets: Efficient SQL Techniques.
Why Java for Data Management?
Java is a powerful language recognized for its ability to handle complex data manipulation tasks. It boasts robust libraries, excellent concurrency control, and significant community support. These features make Java a go-to for large-scale data processing. Additionally, Java's platform independence ensures it can be run on various systems without compatibility concerns.
Key Java Concepts for Data Management
1. Collections Framework
Java's Collections Framework provides a set of classes and interfaces for storing and manipulating groups of data. When managing large datasets, the choice of collection type is crucial.
Code Snippet: Using HashMap for Fast Lookups
import java.util.HashMap;
public class DataManagement {
public static void main(String[] args) {
HashMap<Integer, String> dataMap = new HashMap<>();
// Adding data
dataMap.put(1, "Data 1");
dataMap.put(2, "Data 2");
// Fast retrieval
String retrievedData = dataMap.get(1);
System.out.println("Retrieved: " + retrievedData);
}
}
Why HashMap? HashMap provides constant-time complexity for get
and put
operations, making it efficient for fast lookups, which is critical when dealing with large datasets.
2. Stream API
Introduced in Java 8, the Stream API allows for functional-style operations on collections. It enables you to process data in a more declarative way, which can make the code cleaner and potentially more efficient.
Code Snippet: Filtering and Collecting Data with Streams
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<String> dataset = new ArrayList<>();
dataset.add("Apple");
dataset.add("Banana");
dataset.add("Cherry");
dataset.add("Date");
// Filter and collect
List<String> filteredList = dataset.stream()
.filter(s -> s.startsWith("B"))
.collect(Collectors.toList());
System.out.println("Filtered List: " + filteredList);
}
}
Why Use Streams? The Stream API allows for both readability and efficiency. It can also leverage multi-core architectures for performance gains, especially in data-intensive applications.
3. JDBC for Database Interaction
Java Database Connectivity (JDBC) is crucial for interacting with SQL databases. Efficiently managing large datasets often requires direct communication with a database to retrieve and manipulate data.
Code Snippet: Basic JDBC Query
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class JDBCExample {
public static void main(String[] args) {
String url = "jdbc:mysql://localhost:3306/mydatabase";
String user = "username";
String password = "password";
try (Connection conn = DriverManager.getConnection(url, user, password);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery("SELECT * FROM large_table")) {
while (rs.next()) {
System.out.println("Data: " + rs.getString("column_name"));
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Why JDBC? JDBC enables Java applications to connect directly to SQL databases, allowing developers to execute queries efficiently and handle large datasets. By utilizing prepared statements, you can also prevent SQL injection attacks.
Best Practices for Managing Large Datasets
A. Optimize Your Database
As per the previously mentioned article, optimizing your SQL queries can significantly reduce data retrieval time. Consider indexing frequently searched fields and employing techniques such as partitioning.
B. Use Buffered Streams
When dealing with file I/O operations involving large datasets, consider using Buffered Streams to enhance performance.
Code Snippet: BufferedReader for Efficient File Reading
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReadExample {
public static void main(String[] args) {
String filePath = "large_dataset.txt";
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why BufferedReader? BufferedReader reads text from a character-input stream, buffering characters to provide efficient reading. This is particularly necessary when handling large files to minimize the number of I/O operations.
C. Utilize Caching
Caching frequently accessed data can drastically reduce query times. Consider using a cache library such as EHCache or Caffeine.
Code Snippet: Simple Caching Mechanism
import java.util.HashMap;
public class SimpleCache {
private final HashMap<String, String> cache = new HashMap<>();
public String getData(String key) {
return cache.getOrDefault(key, null);
}
public void putData(String key, String value) {
cache.put(key, value);
}
}
Why Caching? Access times for in-memory data are significantly faster than hitting a database or I/O resource. Caching reduces load times and optimizes performance.
My Closing Thoughts on the Matter
Java provides a rich set of tools and techniques for managing large datasets efficiently. From using collections and streams to leveraging JDBC for database interactions, developers can create robust applications tailored for data processing. As we continue this journey into data management, remember to always refer back to the best practices and optimize your SQL as discussed in the article Managing Large Datasets: Efficient SQL Techniques.
By applying these methodologies and using Java's capabilities, you can transform your approach to data management, ensuring scalable and performant solutions for the ever-growing datasets of today and tomorrow.