Java Session Management: Tackling Inactive Session Issues
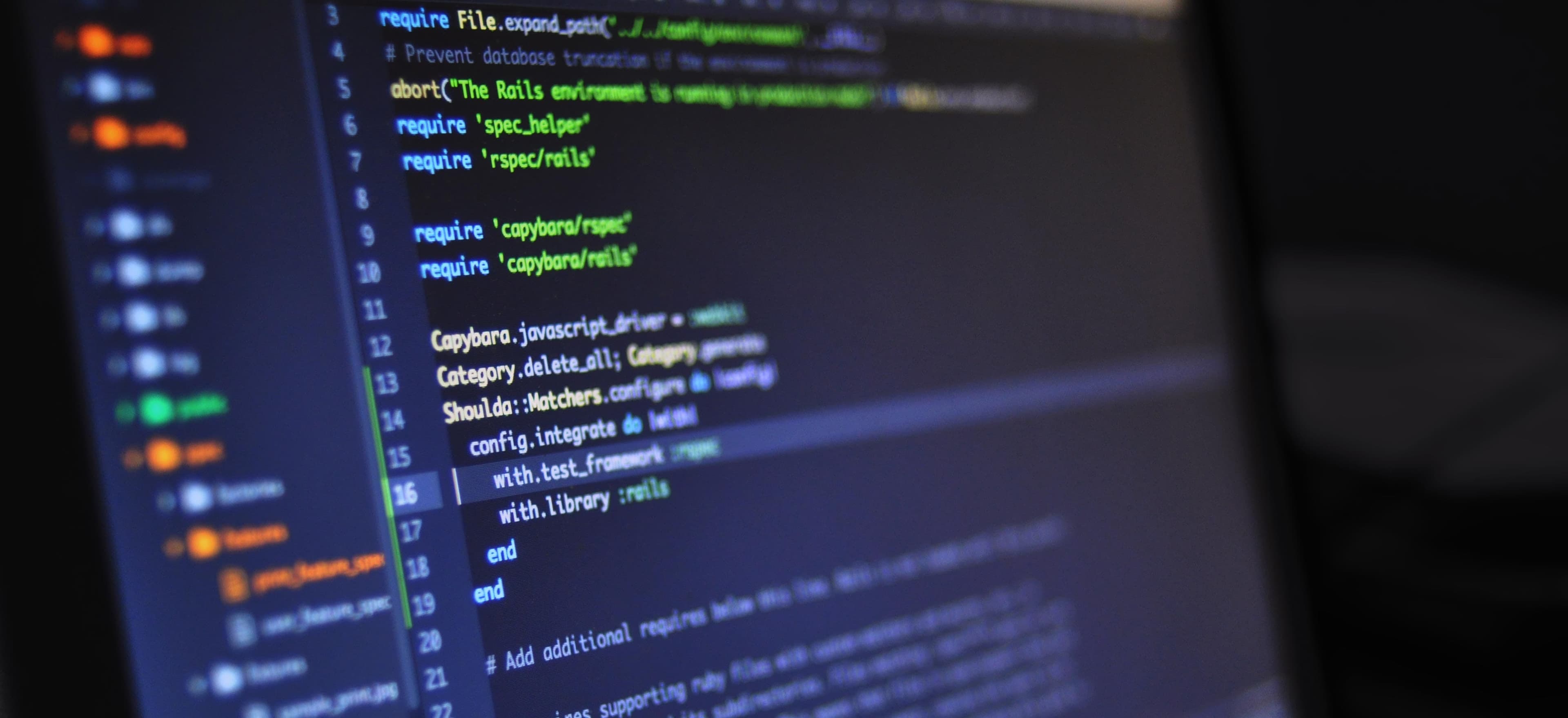
- Published on
Java Session Management: Tackling Inactive Session Issues
In modern web applications, effective session management is crucial for both security and user experience. Java, with its robust framework landscape, offers several techniques for managing sessions efficiently. This article delves into the important aspects of Java session management, common issues related to inactive sessions, and practical solutions, particularly in light of the article "Troubleshooting: Inactive Sessions Causing Disruptions" found at infinitejs.com/posts/troubleshooting-inactive-sessions-disruptions.
Understanding Sessions in Java Web Applications
A session refers to a client-server interaction over a period of time. When a user connects to a web application, a session is created to store user-specific data. This can include user preferences, shopping cart items, or authentication states.
In Java web applications, session management primarily relies on the servlet API. Each user session is assigned a unique ID, which is commonly stored in a cookie or as part of the URL.
Example: Creating a Session
The following code snippet demonstrates how to create an HTTP session in a servlet.
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import java.io.IOException;
public class SessionCreationServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
HttpSession session = request.getSession(); // Create a session if it doesn't exist
session.setAttribute("username", "JohnDoe"); // Store data in the session
response.getWriter().println("Session created for user: " + session.getAttribute("username"));
}
}
In the code above, we create a session by calling request.getSession()
. This method creates a new session if one doesn’t already exist. We store the username in the session to maintain state across multiple requests.
Common Issues with Inactive Sessions
Inactive sessions can lead to significant disruptions in user experience. Here are common pitfalls when dealing with these sessions:
-
Session Timeout: If a user's session is inactive for a prolonged period, it may expire, leading to lost data and frustration.
-
Inconsistent Session States: Multiple tabs or processes might cause changes in session states that lead to inconsistent data representation.
-
Security Risks: Inactive sessions could still be vulnerable if not properly managed, leading to unauthorized access if a user forgets to log out.
Managing Session Timeout
Java allows developers to set a session timeout value. The default timeout is typically 30 minutes, but it can be adjusted based on your application needs.
session.setMaxInactiveInterval(15 * 60); // Set timeout to 15 minutes
In this example, we specify a maximum inactive interval of 15 minutes for the session, ensuring that it will expire sooner, which can be crucial for applications demanding higher security.
To ensure that users are reminded before their sessions expire, you can implement a mechanism to warn users.
Example: Warning the User
Using JavaScript, we can alert the user before a session expires. Here’s an approach that leverages timers:
<script>
var warningTime = 12 * 60 * 1000; // 12 minutes in milliseconds
var expirationTime = 15 * 60 * 1000; // Same as session timeout
setTimeout(function() {
alert("Your session will expire in 3 minutes. Please save your work.");
}, warningTime);
setTimeout(function() {
// Redirect to logout or some information page
}, expirationTime);
</script>
The JavaScript code above sets two timers. The first timer notifies the user 12 minutes into their session, while the second one handles the session expiration behavior. This often enhances the overall user experience.
Session Fixation Attack Prevention
Another crucial aspect of session management is preventing session fixation attacks. These occur when an attacker tricks a user into using a session ID that they control. To mitigate this risk, you can regenerate session IDs upon successful authentication.
Example: Regenerating Session IDs
if (user.isAuthenticated()) {
HttpSession oldSession = request.getSession(false);
if (oldSession != null) {
oldSession.invalidate(); // Invalidate the old session
}
HttpSession newSession = request.getSession(true);
// Store user information in new session
}
In this code snippet, we first fetch the existing session. If a user is authenticated successfully, we invalidate the old session to generate a new session. This ensures that any potential malicious session IDs are not reused.
Common Strategies to Handle Inactive Sessions
-
Persistent Login: Allow users to choose to stay logged in, which can typically be achieved through the use of persistent cookies.
-
Session Heartbeat: For applications requiring prolonged user interaction (like chat applications), consider implementing session heartbeats. These are periodic AJAX calls to keep the session alive.
-
User Feedback: Clearly communicate the session timeout details to users via UI elements or notifications.
-
Server-Side Session Management: Use frameworks like Spring Session to manage sessions more effectively across distributed systems.
To understand how to troubleshoot issues arising from inactive sessions, check out the insightful article "Troubleshooting: Inactive Sessions Causing Disruptions" at infinitejs.com/posts/troubleshooting-inactive-sessions-disruptions.
Wrapping Up
Effective session management in Java applications is a proactive step toward enhancing user experience and securing application data. By understanding the intricacies of session timeout behavior and implementing strategies to handle inactive sessions, developers can create more resilient applications. Remember, it's always better to tackle session management issues before they disrupt user activities, turning potential challenges into seamless experiences.
With proper session management, you can ensure your web applications remain efficient and user-friendly—allowing both you and your users to focus on what truly matters.
By employing these strategies, you not only foster a better interaction design but also build trust among your user base. Effective Java session management could make all the difference—so start implementing these best practices today!