Resolving Inactive Java Sessions in Web Applications
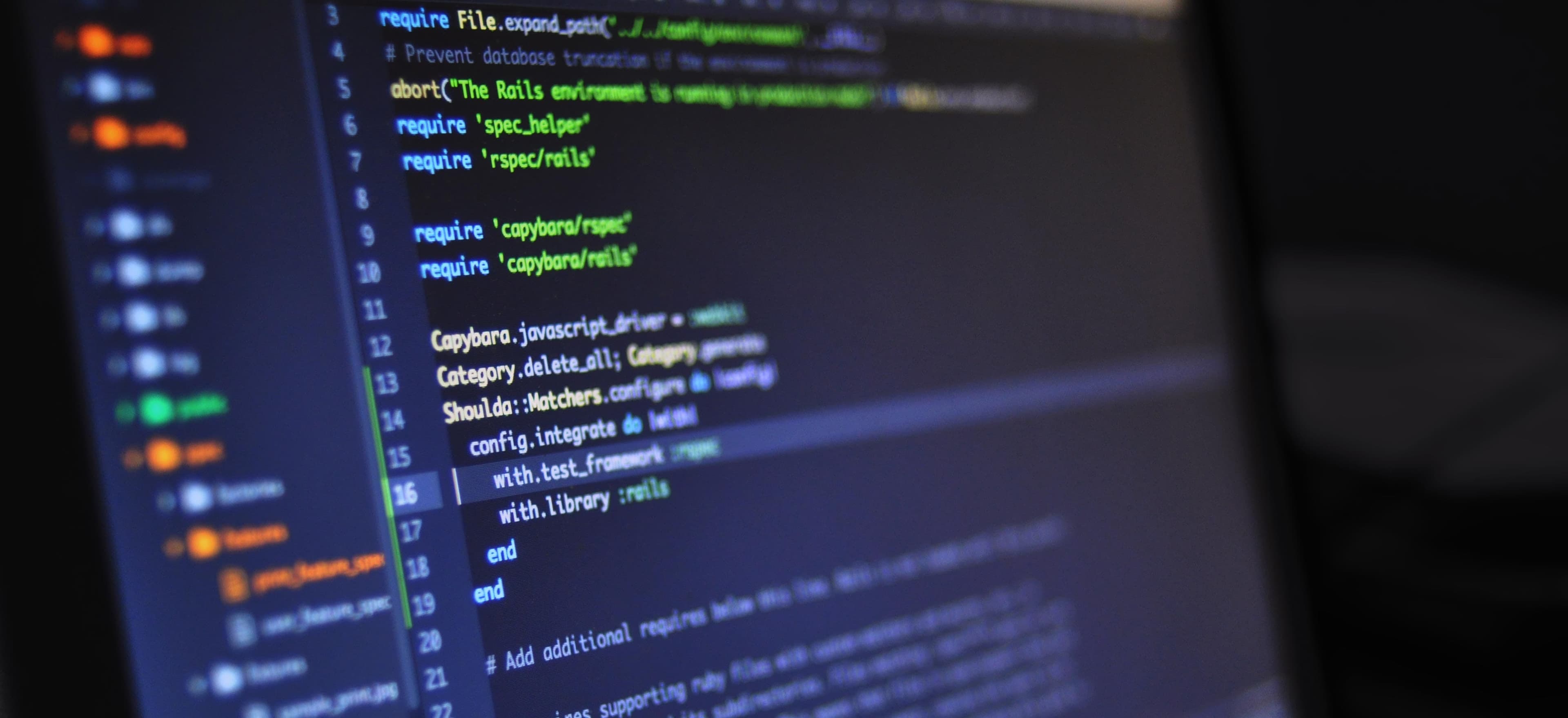
- Published on
Resolving Inactive Java Sessions in Web Applications
In the realm of web applications, session management plays a critical role in ensuring a seamless user experience. However, one common issue faced by developers and system administrators is the occurrence of inactive sessions. Not only do these sessions consume server resources, but they can also cause functionality disruptions and hinder overall productivity. In this blog post, we will delve into the causes of inactive sessions in Java web applications and explore effective strategies for resolving these problems, enhancing both performance and usability.
Understanding Java Sessions
Java web applications typically manage user interactions through sessions, which are stored on the server and tied to a unique session identifier. Each time a user interacts with the application, the server checks for this session to retrieve the required data, ensuring a personalized experience. However, when users become inactive for extended periods, these sessions can become stale and unresponsive, leading to potential server overloads or errors.
What Causes Inactive Sessions?
Inactive sessions can arise due to various reasons, including:
- User Inactivity: Users may leave a session open without any interaction for a prolonged time.
- Network Issues: Intermittent connectivity problems can lead to sessions being left open without user actions.
- Long Processing Times: If a web application takes too long to process a request, the user may navigate away, resulting in an inactive session.
- Browser Issues: Sometimes, browser caches or specific configurations can interfere with session updates.
Recognizing these challenges helps developers create systems that prevent session-related disruptions.
Best Practices for Managing Inactive Sessions
1. Implement Session Timeouts
One of the simplest and most effective ways to manage inactive sessions is by defining session timeouts. In Java web applications, you can set a specific duration for how long a session can remain idle before it is automatically invalidated.
Here's an example of how you can configure session timeouts in a Java servlet:
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpSession;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/MyServlet")
public class MyServlet extends HttpServlet {
@Override
public void init() throws ServletException {
super.init();
// Set a default session timeout of 30 minutes
getServletContext().getSession().setMaxInactiveInterval(30 * 60);
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) {
HttpSession session = request.getSession();
// Example of adding an attribute to the session
session.setAttribute("user", "JohnDoe");
// Additional logic for handling the request...
}
}
Why Timeout?: Implementing session timeouts is crucial to free up resources. They help ensure that inactive user sessions do not linger indefinitely, thereby conserving server memory. Furthermore, it enhances security by reducing potential vulnerabilities from stale sessions.
2. Utilize AJAX for Session Renewal
Another effective method is to use AJAX calls to refresh sessions dynamically. When a user is still active but their session is approaching the timeout limit, you can send a request to the server to renew the session. This prevents timeouts without requiring user interactions.
Here’s a simple example using JavaScript:
setInterval(function() {
fetch('/MyServlet/renewSession', { method: 'POST' })
.then(response => response.json())
.then(data => {
console.log('Session renewed:', data);
})
.catch(error => console.error('Error renewing session:', error));
}, 15 * 60 * 1000); // Renew session every 15 minutes
Why AJAX Renewal?: This approach not only keeps the session alive as long as the user is active, but also eliminates the frustration of session expiration during critical tasks. This enhances user experience and encourages engagement.
3. Handle Session Invalidations Gracefully
It is essential to provide informative feedback to users when their sessions expire. Instead of leaving them in the dark, implement a mechanism that informs them of the status of their session and provides options to log back in or restart their tasks.
Here's how you can handle invalid sessions gracefully in a servlet:
protected void doGet(HttpServletRequest request, HttpServletResponse response) {
HttpSession session = request.getSession(false); // Don't create a new session
if (session == null || session.getAttribute("user") == null) {
try {
response.sendRedirect("sessionExpired.jsp");
} catch (IOException e) {
e.printStackTrace();
}
} else {
// Proceed with business logic
}
}
Why Focus on User Experience?: By guiding users through session expirations, you reduce frustration and retain user satisfaction. A well-structured session management strategy enhances overall application usability.
4. Monitor and Analyze User Behavior
Understanding user behavior patterns can greatly assist in managing inactive sessions. By logging session events, developers can analyze data on user interactions and inactivity. This information can help refine session timeout settings and improve application performance.
For more information on dealing with inactive sessions, consider checking out the article "Troubleshooting: Inactive Sessions Causing Disruptions" here.
Why Monitor Behavior?: Continuous monitoring assists in identifying trends that can inform optimization strategies. It empowers developers to adjust session management policies based on real-world usage rather than static configurations.
5. Utilize a Distributed Session Management System
For larger applications, particularly those using microservices architectures, consider utilizing a distributed session management system. Tools like Redis or Memcached can effectively manage sessions across various servers, ensuring that inactive sessions are tracked and handled efficiently.
Implementing a Basic Redis Session Store
Here’s how you might configure a Redis session store in a Java application:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
@EnableRedisHttpSession
public class HttpSessionConfig {
@Bean
public RedisConnectionFactory connectionFactory() {
return new JedisConnectionFactory();
}
}
Why Distributed Management?: A distributed session management system scales better, especially when faced with high traffic. It decreases the load on individual servers and tends to provide better performance and availability.
Wrapping Up
Managing inactive sessions is vital for any web application's health and performance. With the practices outlined above, you will be better equipped to tackle inactive sessions effectively. From implementing timeouts and AJAX renewal techniques to utilizing monitoring and distributed management systems, each approach enhances both user experience and system efficiency.
By proactively addressing inactive sessions, you not only optimize server performance but also foster a more engaging environment for users. As technologies and practices continue to evolve, staying informed and adaptable will be instrumental in maintaining robust session management protocols.
Remember to regularly review your session management strategies and analyze user patterns for continuous improvement. For a deeper understanding of session-related issues, don’t hesitate to refer to the informative article "Troubleshooting: Inactive Sessions Causing Disruptions" here.
Happy coding!