Fixing Input Size Issues for Java Web Apps in Browsers
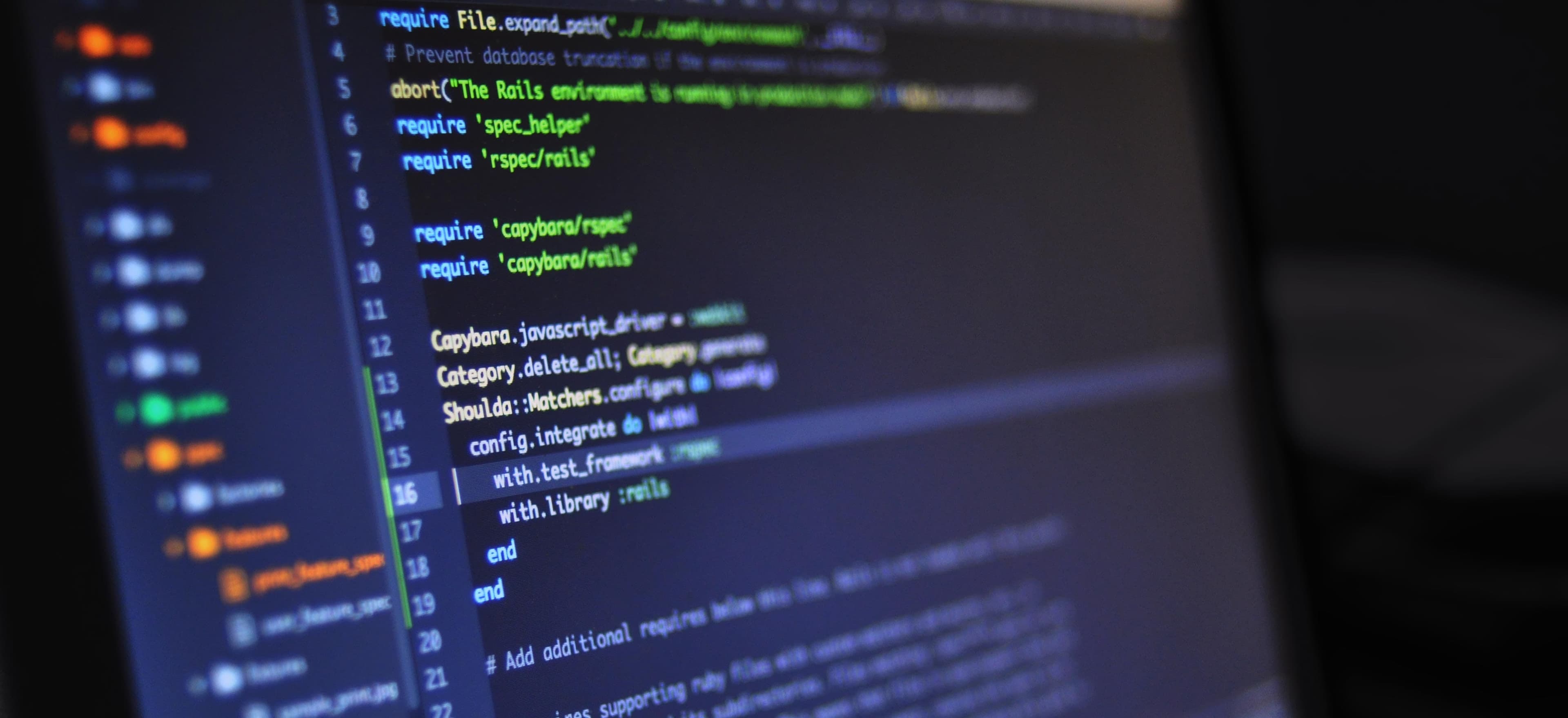
- Published on
Fixing Input Size Issues for Java Web Apps in Browsers
When developing Java web applications, ensuring that your forms and input elements display consistently across different browsers can be challenging. One common issue developers encounter is the inconsistency in input size, especially with text fields and buttons. In this post, we’ll discuss how to address these inconsistencies effectively and offer practical code snippets to enhance your web application’s user experience.
Understanding the Issue
Different browsers have varying default styles for HTML elements. This means an input field in Firefox can look entirely different in Internet Explorer or even Chrome. The article "Inconsistent Search Input and Button Sizes in IE7 and Firefox" from Tech Snags highlights these discrepancies and provides a context that is crucial for understanding why we need to address them. You can read the article here.
The challenge is compounded when you consider the multitude of devices and resolutions users might be using to access your web applications. This variability can lead to a poor user experience if elements appear out of alignment.
Why is Consistency Important?
- User Experience: A uniform look reinforces user trust and provides an intuitive interface.
- Branding: Consistent element sizes align with your brand’s aesthetic.
- Accessibility: Ensuring compatibility across browsers and devices makes your app accessible to a broader audience.
CSS Reset
The first step to achieving consistency is to use a CSS reset. A CSS reset levels the playing field by reducing the default styling differences among browsers. Here’s a simple CSS reset snippet you can use:
/* CSS Reset Example */
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
Why Use a CSS Reset?
- Margin and Padding: Different browsers have different default margins and paddings. Resetting these helps to start from a common point.
- Box Sizing: Setting
box-sizing: border-box;
allows you to include padding and border size in the element’s total width and height. This simplifies sizing inputs, as you’ll have better control.
Custom Styles for Input Fields
After you have your reset in place, you will want to apply consistent styles to your input fields. Here’s a sample CSS that styles the text input and button uniformly across different browsers:
/* Custom Input Styles */
input[type="text"], input[type="submit"] {
width: 100%; /* Makes the input take the full width of its container */
padding: 10px; /* Adds space inside the input */
border: 1px solid #ccc; /* Provides a solid border */
border-radius: 4px; /* Creates rounded edges */
font-size: 16px; /* Adjusts font size for readability */
}
/* Button Specific Styles */
input[type="submit"] {
background-color: #4CAF50; /* A pleasing green color for the button */
color: white; /* Text color for better contrast */
cursor: pointer; /* Changes cursor to pointer on hover */
}
input[type="submit"]:hover {
background-color: #45a049; /* Darker green on hover */
}
Commentary on Code
- Width: Using
width: 100%
ensures your inputs stretch to fill available space, which can greatly enhance layouts. - Padding: Adding padding improves user interaction by providing enough space for typing.
- Borders and Colors: A well-defined border contributes to the visual hierarchy, while color choices can affect user focus on critical actions such as submitting forms.
Responsive Design Considerations
Now, let’s discuss how to make your input fields responsive across different devices. Media queries provide a great way to adjust styles based on the device width. Here’s an example:
@media (max-width: 600px) {
input[type="text"], input[type="submit"] {
font-size: 14px; /* Smaller font size for narrow screens */
padding: 8px; /* Reducing padding */
}
}
Why Employ Media Queries?
Media queries allow you to tailor your styles based on the device used. This is especially important in web environments today, where user behavior significantly varies between desktop and mobile devices.
Java Integration with CSS
Integrating your CSS styles effectively with your Java web application is essential. Let's look at a Java servlet example that generates an HTML form with styled inputs:
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/form")
public class FormServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
response.getWriter().println("<html><head><title>Styled Form</title>");
response.getWriter().println("<link rel='stylesheet' type='text/css' href='styles.css'>");
response.getWriter().println("</head><body>");
response.getWriter().println("<form action='/submit' method='POST'>");
response.getWriter().println("<input type='text' name='search' placeholder='Search...' required>");
response.getWriter().println("<input type='submit' value='Search'>");
response.getWriter().println("</form></body></html>");
}
}
Why is This Important?
This Java servlet dynamically generates a form embedded with the CSS designed to ensure consistency in appearance. Using servlets to deliver HTML pages with integrated CSS ensures your inputs always have the required styling, irrespective of the browser.
Testing Across Browsers
Finally, it's essential to test your application across multiple browsers:
- BrowserStack or CrossBrowserTesting: These tools let you check how your app behaves across different platforms.
- Developer Tools: Use built-in developer tools (e.g., Chrome DevTools) to simulate different devices and screen sizes.
The Closing Argument
Ensuring consistent input sizes across web applications can create a streamlined and user-friendly experience. From employing a CSS reset to creating responsive designs with media queries, it is vital to consider every aspect of the input fields. By integrating the styles with Java applications, you ensure a professional appearance throughout your project.
Your users deserve the best; don't let inconsistent styles be a barrier to their experience. Always test across browsers to make sure you’ve met those standards.
Feel free to check out more information on related topics at Tech Snags and refer back to their article for a focused discussion on search input and button sizes if you face these issues frequently: Inconsistent Search Input and Button Sizes in IE7 and Firefox.
Happy coding!