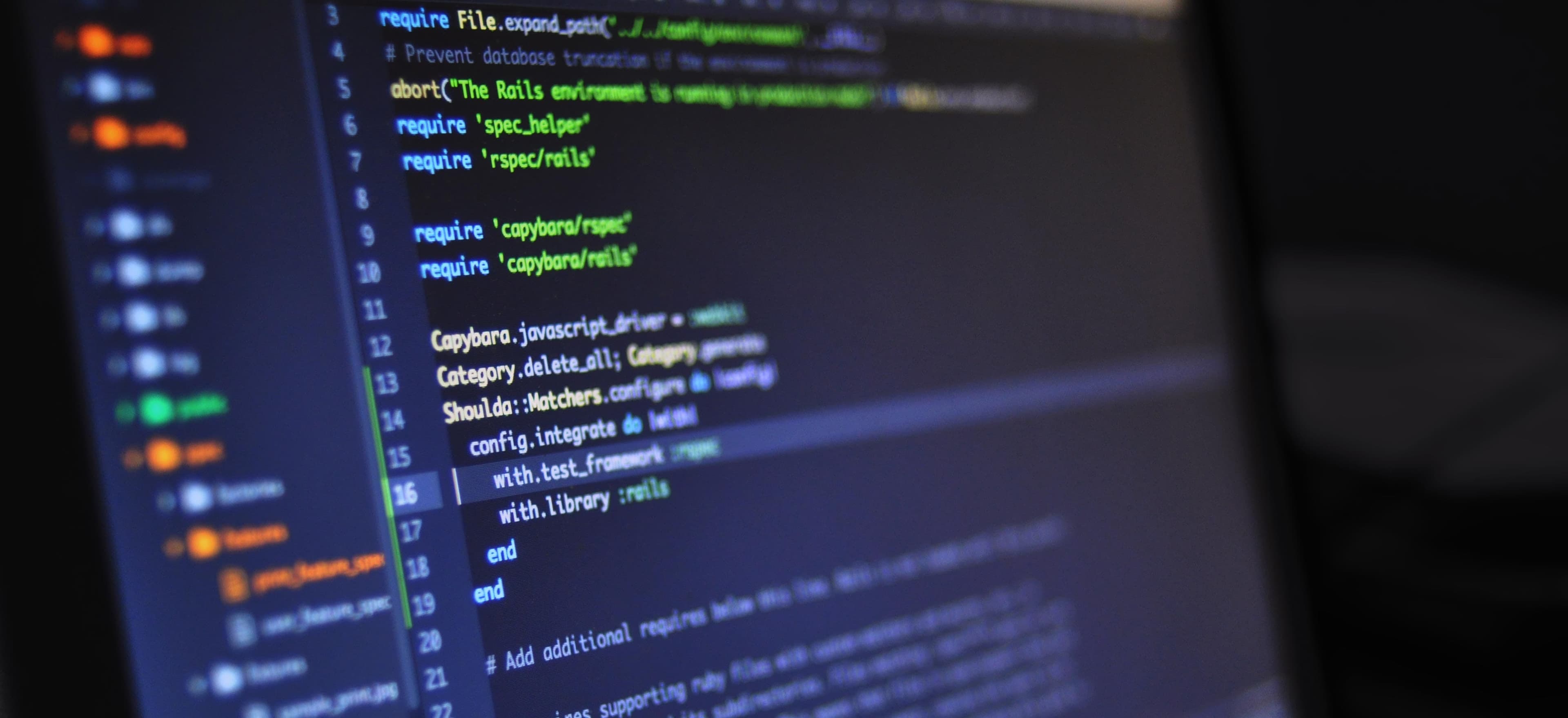
- Published on
Fixing Search Bar Consistency in Java Web Apps
The user experience (UX) is critical in web applications, especially when offering a seamless interaction for the end user. One common issue developers face is ensuring consistency in UI elements—specifically, the search bar. Browsers can behave differently regarding spacing, padding, and size, leading to an inconsistent look across platforms. This blog post will guide you through fixing search bar inconsistencies using Java Web applications.
Understanding the Problem
According to a helpful article titled Inconsistent Search Input and Button Sizes in IE7 and Firefox, discrepancies in how different browsers render UI elements can lead to poor UX. The article highlights significant design challenges due to these inconsistencies, which can affect user trust and satisfaction.
To resolve these issues, we will focus on HTML and CSS adjustments using Java technologies for web applications. Ensuring that your search bar has a consistent look across all browsers will run smoother before moving to more complex components.
Setting Up Your Java Web Application
Before we jump into the technical aspects, let's ensure your Java web application is ready for modifications. You should have the following:
- A Java web application framework (e.g., Spring, JSF)
- A basic understanding of HTML, CSS, and Java
- A project set up and running on a local or staging server
Sample Search Bar Setup
Here's a fundamental example of setting up a search bar HTML structure in your Java web application:
<form action="/search" method="get">
<input type="text" id="searchQuery" name="query" placeholder="Search..." />
<button type="submit">Search</button>
</form>
Why This Structure?
- Form Element: wrapping your inputs and buttons in a form allows you to handle submissions more intuitively.
- Input Field: uses a text input to collect search queries, providing a placeholder text for guidance.
- Button: an intuitive 'submit' button to trigger the search.
Styling Your Search Bar Consistently
To ensure consistency across browsers, it's essential to reset some default browser styles. Let's start with basic CSS styling of the search bar, focusing on padding, margin, border, and font-size:
form {
display: flex;
justify-content: center;
align-items: center;
margin: 20px;
}
input[type="text"] {
padding: 10px;
margin-right: 5px;
border: 1px solid #ccc;
border-radius: 4px;
font-size: 16px;
width: 250px; /* Fixed width for better consistency */
}
button {
padding: 10px 20px;
background-color: #007BFF;
color: white;
border: none;
border-radius: 4px;
font-size: 16px;
cursor: pointer;
}
button:hover {
background-color: #0056b3;
}
Why These Styles?
-
Flexbox: The
display: flex;
property aligns input and button neatly. -
Padding & Margin: Specific padding and margin values help prevent any inconsistencies due to default browser values (which vary).
-
Border Radius: A border-radius creates a modern, uniform look, which is particularly important for touch screens.
-
Fixed Width: Setting a fixed width for the input ensures that it will be visualized the same way across browsers.
Responsive Design Considerations
While maintaining consistency across different browsers, don’t forget about responsiveness. A consistent search bar on a mobile device looks quite different than on a desktop. Below is a media query to adjust the styles for smaller screens:
@media (max-width: 768px) {
input[type="text"] {
width: 100%; /* Make input full width on mobile */
margin-right: 0;
}
button {
width: 100%; /* Button can also span the full width */
}
}
Why Media Queries?
Responsive design is vital in creating a seamless user experience on various devices. These adjustments ensure that your search bar won't feel cramped on mobile devices, maintaining an inviting look for users.
Java Backend Handling
On the server-side, you'll handle search requests submitted via the search form. Using a Java framework like Spring, you can set up a simple controller to handle this:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
@Controller
public class SearchController {
@GetMapping("/search")
public String performSearch(@RequestParam("query") String query) {
// Add your search logic here to handle the database search or logic you desire
// e.g., List<SearchResult> results = searchService.performSearch(query);
return "resultsPage"; // Return view name
}
}
Explanation of the Code
- Controller Annotation: Marks this class as a Spring MVC controller.
- GetMapping Annotation: Maps HTTP GET requests for the "/search" endpoint.
- RequestParam Annotation: Fetches the user's search query seamlessly.
Testing Across Browsers
After implementing the above HTML, CSS, and Java code, test your application across multiple browsers. Tools like BrowserStack or physical devices can help you ensure consistency.
Browser Testing Tips
- Look for Layout Changes: Check how padding, margins, and button sizes look in each browser.
- Font Rendering: Compare font sizes and styles; adjust CSS as necessary.
- Functionality: Ensure that the submitted search queries are processed correctly on all browsers.
My Closing Thoughts on the Matter
Ensuring a consistent search bar experience in your Java web application can greatly impact user satisfaction. By utilizing structured HTML, proper CSS styling, responsive design techniques, and a functioning Java backend, you can help create a more uniform search experience that reflects professionalism.
For further reading on design inconsistencies in browser rendering, revisiting this article will provide you with more insights.
By addressing these UI concerns, you're well on your way to creating a more cohesive and enjoyable user experience throughout your application. Happy coding!
Checkout our other articles