Mastering Change Detection in Angular with Java Integration
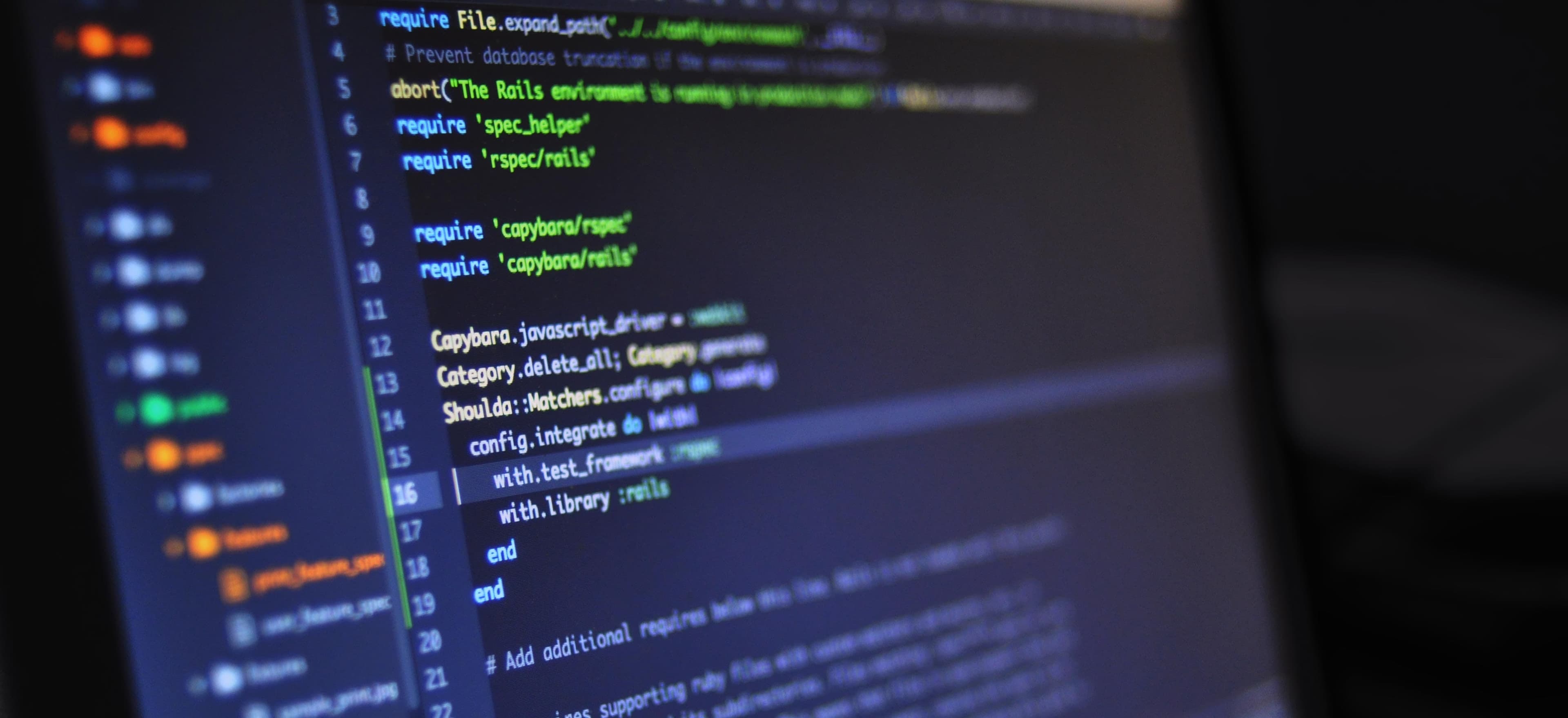
- Published on
Mastering Change Detection in Angular with Java Integration
In the modern web development landscape, mastering the integration and functionality of various technologies can significantly enhance your applications. One of the intriguing aspects of Angular, a popular front-end framework, is its change detection mechanism. This article will dive deep into understanding Angular's change detection and how you can seamlessly integrate it with Java back-end services.
Before we delve into the intricacies, it might be beneficial to reference an insightful article titled Demystifying Angular: Solving Change Detection Mysteries, which provides foundational knowledge that complements our exploration here.
What is Change Detection?
Change detection in Angular is a mechanism that ensures the UI stays in sync with the application data. Whenever there are changes in the state of data, Angular checks and updates the view accordingly. This process is crucial for creating responsive and interactive applications.
Angular employs two primary change detection strategies:
- Default Change Detection: Angular checks all components every time an event occurs.
- OnPush Change Detection: Angular only checks the component when its input properties change or an event is triggered from within the component.
Understanding when to use each method can lead to optimized performance and a snappier user experience.
Change Detection Strategies
Default Change Detection
This is the standard mode of change detection in Angular. It checks all components in the component tree to ensure that they reflect the latest data. However, while Default Change Detection is straightforward and works seamlessly in many scenarios, it can become inefficient for complex applications.
Here’s a concise example:
import { Component } from '@angular/core';
@Component({
selector: 'app-default-change-detection',
template: `<h1>{{title}}</h1><button (click)="updateTitle()">Update Title</button>`
})
export class DefaultChangeDetectionComponent {
title = 'Hello Default Change Detection';
updateTitle() {
this.title = 'Title Updated!';
}
}
Why Use Default Change Detection?
- Best for simple and medium-sized applications where data changes are predictable.
- Easy to implement as it requires minimal configuration steps.
OnPush Change Detection
OnPush is a more advanced strategy designed to enhance performance. By marking a component with the OnPush strategy, Angular will only check that component's view if it receives new inputs or triggers an event.
Example of using OnPush strategy:
import { Component, ChangeDetectionStrategy } from '@angular/core';
@Component({
selector: 'app-onpush-change-detection',
template: `<h1>{{title}}</h1><button (click)="updateTitle()">Update Title</button>`,
changeDetection: ChangeDetectionStrategy.OnPush
})
export class OnPushChangeDetectionComponent {
title = 'Hello OnPush Change Detection';
updateTitle() {
this.title = 'Title Updated!'; // Will not trigger change detection by default
}
}
Why Utilize OnPush Change Detection?
- Reduces the number of checks Angular performs.
- Enhances the performance of larger applications.
- Ideal for scenarios where data is immutable.
Best Practices for Change Detection in Angular
-
Use OnPush Strategically: Not every component needs OnPush. Use it for components that rely heavily on inputs and are less likely to trigger changes internally.
-
Immutable Data Structures: Embrace immutable data practices. Angular's OnPush change detection works effectively when your application data remains immutable.
-
Avoid Memory Leaks: Ensure that subscriptions to observables are cleaned up when components are destroyed to prevent memory leaks, especially when using OnPush.
-
Utilize Observables: Using libraries like RxJS can help manage asynchronous data streams efficiently while keeping the code clean and maintainable.
-
Profile and Test: Performance monitoring tools can help you assess when and how change detection occurs. Utilize Angular’s built-in profiling tools to identify bottlenecks.
Integrating Angular with Java Back-End
Now that we've established a firm grasp on Angular's change detection, let's explore how to integrate this front-end framework with a Java back-end service.
Setting Up a Java REST API
Creating a RESTful service with Java is a common approach to allow your Angular application to consume data. Below is a simple example using Spring Boot.
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class GreetingController {
@GetMapping("/api/greetings")
public String getGreeting() {
return "Hello from Java Back-End!";
}
}
Angular Service to Consume Java REST API
Once the Java back-end is set up, you can create an Angular service to communicate with the Java REST API.
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root'
})
export class GreetingService {
private apiUrl = 'http://localhost:8080/api/greetings'; // Update with your API URL
constructor(private http: HttpClient) {}
getGreeting(): Observable<string> {
return this.http.get<string>(this.apiUrl);
}
}
Example Component Using the Service
Next, we will implement a component that leverages the service to display messages from the Java back-end.
import { Component, OnInit } from '@angular/core';
import { GreetingService } from './greeting.service';
@Component({
selector: 'app-greeting',
template: `<h1>{{ greeting }}</h1><button (click)="fetchGreeting()">Fetch Greeting</button>`
})
export class GreetingComponent implements OnInit {
greeting: string = '';
constructor(private greetingService: GreetingService) {}
ngOnInit() {
this.fetchGreeting();
}
fetchGreeting() {
this.greetingService.getGreeting().subscribe(
data => this.greeting = data,
error => console.error('Error fetching greeting', error)
);
}
}
My Closing Thoughts on the Matter
The interplay between Angular's change detection and Java back-end services is a powerful combination that can be harnessed for developing dynamic web applications. By mastering change detection strategies—whether Default or OnPush—you can optimize your application for performance and responsiveness.
Understanding and implementing best practices ensures your application remains scalable and easy to maintain. Besides, by leveraging the integration capabilities of Angular and Java, you can create responsive user experiences that reflect real-time data changes.
For more insights into Angular’s intricacies, don’t forget to check out Demystifying Angular: Solving Change Detection Mysteries.
By taking the time to comprehend and implement these strategies, you'll be well on your way to mastering Angular and Java integration, elevating both your development skills and the user experience of your applications.
Checkout our other articles