Dynamic Content in Java: Tackling Variable GridView Complexity
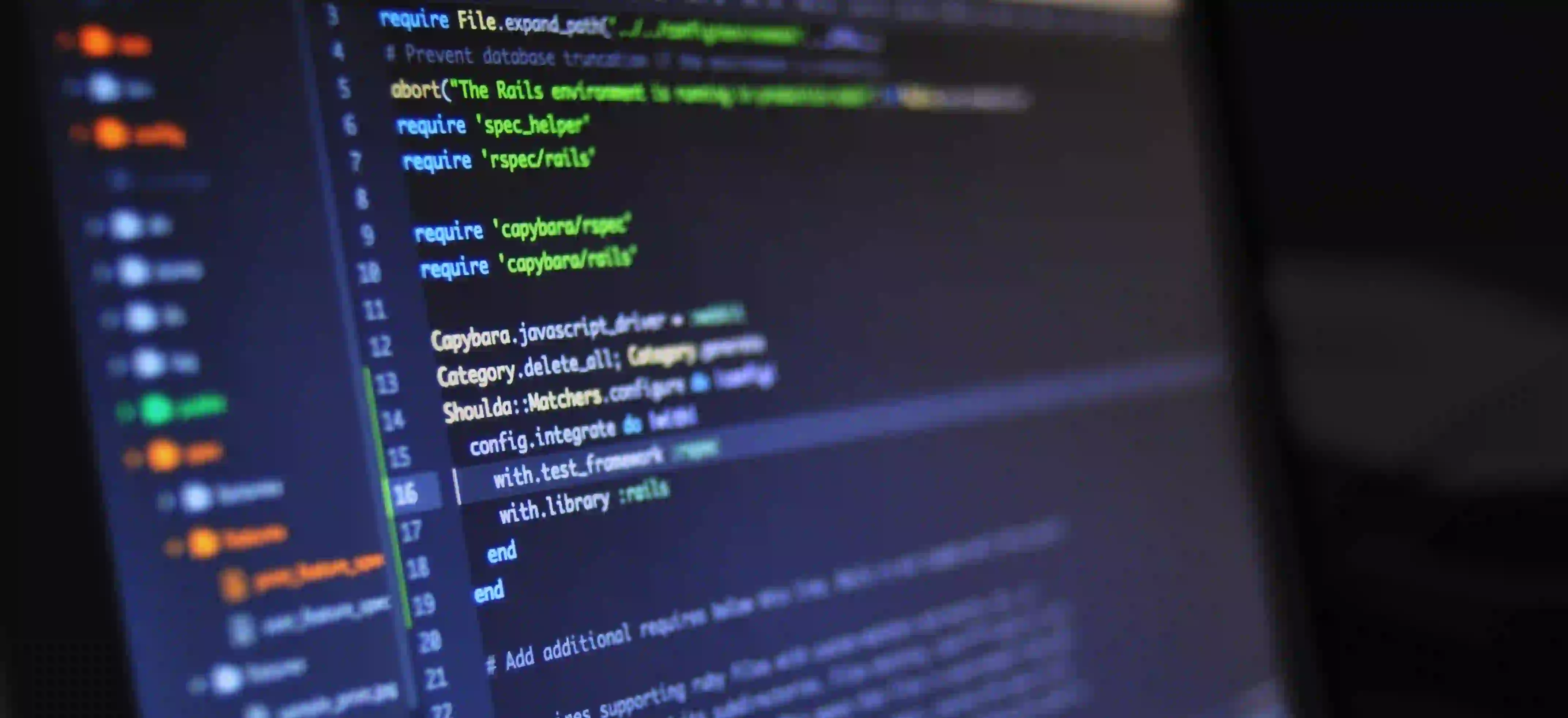
Dynamic Content in Java: Tackling Variable GridView Complexity
In modern software development, the need for dynamic content rendering is paramount. This is particularly true within Java applications where user interfaces must respond fluidly to data changes. One common challenge developers face is managing a GridView that adapts based on variable content. In this post, we will explore strategies to overcome this complexity while keeping our code clean and maintainable.
If you wish to deepen your understanding of dynamic content rendering, you might find our reference to the existing article, "Mastering Dynamic Content: Variable GridView Challenges", particularly beneficial.
Understanding GridView
A GridView is a control that helps display data in a grid layout. It organizes content in rows and columns, allowing for easy viewing and manipulation. The real power of GridView comes into play when it is paired with dynamic data sources. However, properly managing this dynamic behavior presents many challenges, such as performance issues, state management, and UI responsiveness.
Key Challenges
- Dynamic Data Updates: How do we ensure that the GridView reflects changes in the underlying dataset without reloading the entire interface?
- Variable Content Sizes: How do we manage different data types and sizes, while maintaining a consistent user interface?
- Performance Optimization: How can we prevent lagging and ensure a responsive user experience?
Setting Up a Dynamic GridView
To exemplify how to tackle these challenges, let's create a simple dynamic GridView in a Java application. We will utilize Java Swing, a popular choice for creating GUIs.
Step 1: Basic Structure
Here's the initial setup to create a basic GridView structure using JTable.
import javax.swing.*;
import javax.swing.table.DefaultTableModel;
public class DynamicGridView {
private JFrame frame;
private JTable table;
private DefaultTableModel tableModel;
public DynamicGridView() {
this.frame = new JFrame("Dynamic GridView Example");
this.tableModel = new DefaultTableModel(new Object[]{"ID", "Name", "Age"}, 0);
this.table = new JTable(tableModel);
setupUI();
}
private void setupUI() {
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(600, 400);
frame.add(new JScrollPane(table));
frame.setVisible(true);
}
public static void main(String[] args) {
SwingUtilities.invokeLater(DynamicGridView::new);
}
}
Code Explanation
- JTable: We are using JTable to represent the GridView. This component is flexible and allows for row and column management.
- DefaultTableModel: This model manages the data we want to display, initialized with column headers "ID", "Name", and "Age".
- Scroll Pane: Wrapping the JTable in a JScrollPane allows for scrolling capabilities when more data exceeds the viewable area.
Step 2: Adding Dynamic Content
Now let’s add the ability to dynamically update the GridView with new records.
public void addRecord(int id, String name, int age) {
tableModel.addRow(new Object[]{id, name, age});
}
Why AddRecord?
addRecord
: This method facilitates the addition of new entries directly to the GridView.- This keeps the user experience seamless; users can see updates without needing to refresh the entire view.
Step 3: Handling Variable Content
Imagine we want to accommodate variable content sizes, meaning that some entries might have additional columns or different data types. Here is how we can approach this complexity:
public void updateGridView(int id, String name, Object... otherData) {
// Example logic to update or set dynamic columns based on some conditions
for(int i = 0; i < otherData.length; i++) {
tableModel.setValueAt(otherData[i], id, i + 3); // Assuming columns beyond 3 are variable
}
}
Handling Variable Data with UpdateGridView
- The
updateGridView
method ensures that we can handle various content types and sizes dynamically. - By accepting an array of additional data, we can adapt our GridView according to the specific requirements of our dataset.
Step 4: Performance Optimization
When dealing with a dynamic GridView, performance occurs at the forefront of our design considerations. If the data grows too large, our application might lag.
Here are some strategies that can be employed:
- Pagination: Instead of loading all data simultaneously, load it in "pages".
- Asynchronous Data Loading: Implement background tasks for data updates.
- Column Visibility Management: Only show relevant columns as needed.
Implementing Pagination
To illustrate pagination briefly, here’s a sketch of how we could think about loading data in chunks:
public void loadPage(int pageNumber, int pageSize) {
tableModel.setRowCount(0); // Clear existing data
List<Data> pageData = loadDataFromSource(pageNumber, pageSize);
for (Data data : pageData) {
addRecord(data.getId(), data.getName(), data.getAge());
}
}
Summary
Creating a dynamic GridView in Java presents many challenges, especially when managing variable content. By understanding the fundamental components such as JTable, DefaultTableModel, and proper data manipulation methods, we can create an intuitive application that efficiently responds to user interactions.
For further reading and deeper insights on grid handling, check out the article "Mastering Dynamic Content: Variable GridView Challenges".
Closing the Chapter
With dynamic GridView capabilities at your fingertips, you can enhance your Java applications significantly. This guide outlined essential strategies for managing variable content while ensuring a responsive user experience. Always remember the balance between functionality and usability for successful software development.
By implementing these strategies and continually learning, you can position yourself for success in managing sophisticated Java user interfaces. Happy coding!