Deciphering Java's Operator Precedence: A Common Pitfall
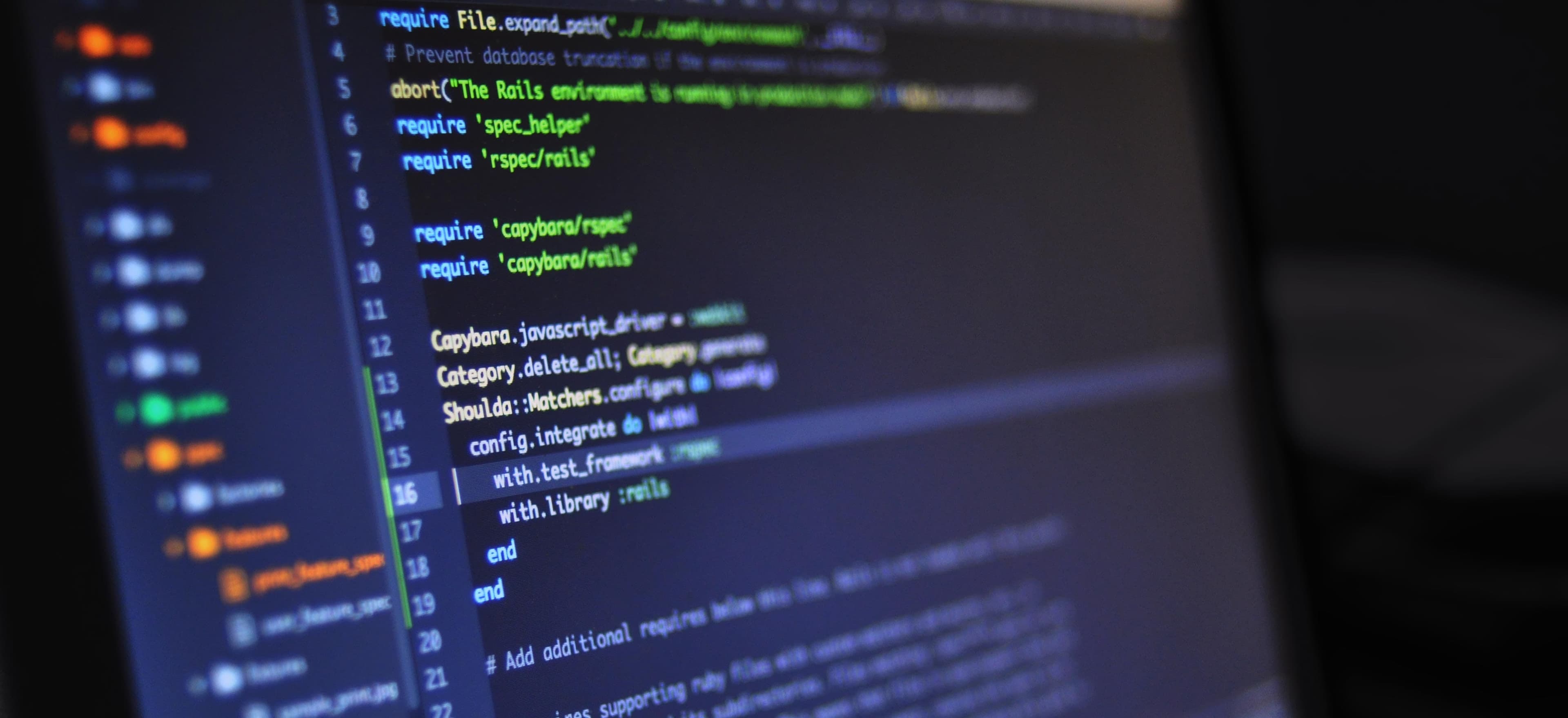
- Published on
Deciphering Java's Operator Precedence: A Common Pitfall
Java is a powerful programming language favored for its ease of use, readability, and strong performance. However, one common pitfall that many developers encounter is misunderstanding operator precedence. In this blog post, we’ll delve into the nuances of operator precedence in Java, clarify how it works, and highlight common mistakes to avoid, ensuring you wield the power of Java more effectively.
What is Operator Precedence?
Operator precedence determines the order in which different operations are evaluated in an expression. For instance, it dictates whether addition or multiplication is performed first. Understanding this is crucial to writing correct and efficient Java code.
Basic Order of Operations in Java
Here is a simplified list of operator precedence in Java, from highest to lowest:
- Postfix Operators:
expr++
,expr--
- Unary Operators:
++expr
,--expr
,+expr
,-expr
,!
- Multiplicative Operators:
*
,/
,%
- Additive Operators:
+
,-
- Shift Operators:
<<
,>>
,>>>
- Relational Operators:
<
,>
,<=
,>=
,instanceof
- Equality Operators:
==
,!=
- Bitwise AND:
&
- Bitwise XOR:
^
- Bitwise OR:
|
- Logical AND:
&&
- Logical OR:
||
- Ternary Operator:
? :
- Assignment Operators:
=
,+=
,-=
, etc.
Example: Basic Evaluation of Precedence
Consider the following Java expression:
int result = 10 + 20 * 30 / 15 - 5;
Breakdown of Expression
- Following the precedence rules, multiplication and division will be evaluated before addition and subtraction.
- First,
20 * 30
evaluates to600
. - Then,
600 / 15
evaluates to40
. - Finally,
10 + 40 - 5
results in45
.
So, the value of result
will be 45
.
Why Operator Precedence Matters
Incorrect assumptions about operator precedence can lead to bugs that are hard to trace. It is essential to be mindful of how operations are ordered.
Take, for example, a common mistake made with the unary and binary operators:
int a = 5;
int b = 10;
int result = a + b * -2; // What is the result?
Evaluating the Example
In this case:
- The multiplication occurs first due to higher precedence.
- Thus, the expression is evaluated as
a + (b * -2)
, resulting in5 + (-20)
, which gives-15
.
If one were to write int result = (a + b) * -2;
, then the outcome would be different (which is -30
). This demonstrates the significance of understanding operator precedence.
Common Mistakes to Avoid
1. Misunderstanding Grouping
When operators of the same precedence level are used, it's evaluated from left to right. For instance:
int value = 4 - 2 + 1; // Left to right: (4 - 2) + 1 => 3
It's crucial to remember that simplistically reading from left to right isn’t always true when mixing categories of operators.
2. Overusing Parentheses
While parentheses can clarify expressions, excessive use can clutter the code. Strive for balance. Use them where necessary, not as a go-to solution.
int result = (a + b) * (c - d); // Clear and concise
However, unnecessary parentheses can create confusion.
3. Neglecting Operator Shortcuts
Developers sometimes overlook built-in shortcuts or operator overrides:
int a = 5;
int b = a++; // Increments a after assigning its current value to b.
Here, b
becomes 5
, but a
changes to 6
.
Clarifying with an Example
Let’s take a method that checks if a number is even or odd using modulo:
public String checkEvenOdd(int number) {
return (number % 2 == 0) ? "Even" : "Odd";
}
In this piece of code:
- The modulus operator is applied first, determining whether the result is zero before making a decision.
This expression emphasizes the clearer flow when leveraging operator precedence.
Advanced Tips
1. Readability First
Always prioritize writing readable code. While expecting someone to remember the precedence rules can be unrealistic, surrounding your expressions with parentheses boosts understanding.
2. Refactoring Code
If you find complex statements, consider breaking them down:
int sum = a + b;
int product = sum * c;
Instead of:
int finalResult = a + b * c;
Such refactoring helps ensure clarity and minimizes errors.
3. Consulting Documentation
Always refer to the Java Documentation for quick clarifications concerning operator precedence.
Concluding Thought
Understanding operator precedence can prevent a myriad of common programming pitfalls. In maintaining careful attention to how expressions are evaluated, you build cleaner, more reliable Java applications.
For deeper insights into related terminology and the potential for confusion in operand evaluation, check out the article "Understanding the Confusion: Why 'a-b' Isn't 'a-a-b'" at infinitejs.com/posts/understanding-a-b-not-aab for further reading.
Incorporating these rules and guidelines will not only help avoid mistakes but also foster better coding practices moving forward. Happy coding!
Checkout our other articles