Simplifying Java App Deployment on Kubernetes: Key Tips
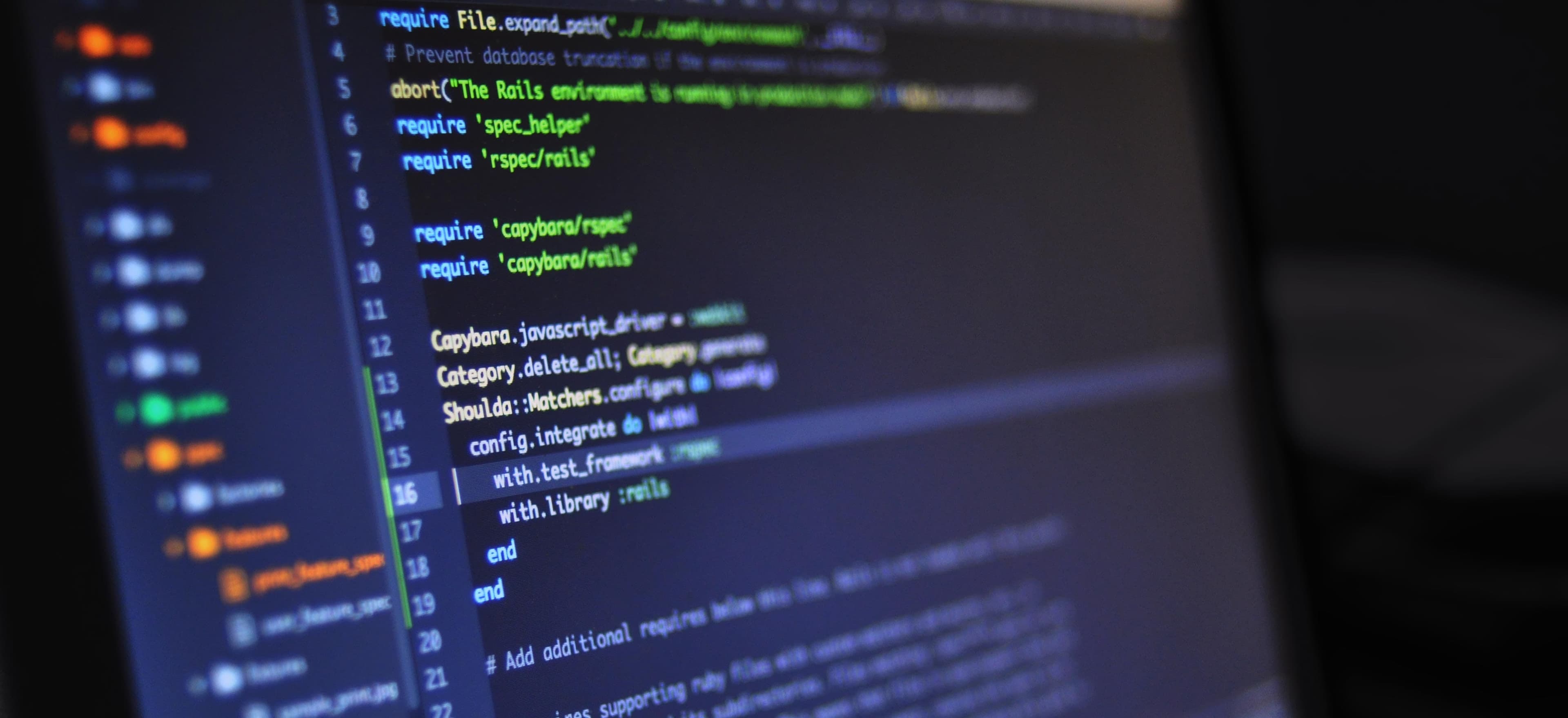
- Published on
Simplifying Java App Deployment on Kubernetes: Key Tips
The world of application development has rapidly evolved, and with it, so has the way we deploy applications. Kubernetes has become the go-to platform for managing containerized applications, and for good reason. In this article, we will explore how to simplify your Java app deployment on Kubernetes by incorporating best practices and understanding essential concepts.
Understanding the Basics of Kubernetes
Before diving into deployment strategies, it is crucial to understand what Kubernetes is and how it operates. Kubernetes is an open-source container orchestration platform designed to automate the deployment, scaling, and management of applications.
In a Kubernetes environment, your applications run within containers. For Java applications, this typically means packaging your code in Docker containers.
To manage the lifecycle of your applications, Kubernetes has several key components, including:
- Pods: The smallest deployable units in Kubernetes. A pod can host one or more containers.
- Deployments: Specify the desired state for your pods and manage their updates.
- Services: Define a logical set of pods and a policy to access them.
Understanding these basics is essential for efficiently deploying Java applications.
Getting Started with Java on Kubernetes
Step 1: Containerize Your Java Application
Before deploying a Java application, you need to package it into a Docker container. Below is a simple example of a Dockerfile for a Java application.
# Use a base image with Java pre-installed
FROM openjdk:11-jre-slim
# Set the working directory
WORKDIR /app
# Copy the built JAR file into the container
COPY target/my-java-app.jar my-java-app.jar
# Expose the port your application runs on
EXPOSE 8080
# Command to run the application
CMD ["java", "-jar", "my-java-app.jar"]
Commentary: Why this Dockerfile?
- Base Image: We choose a lightweight base image like
openjdk:11-jre-slim
to reduce the size of our container without sacrificing performance. - Work Directory: Setting a working directory (
/app
) helps keep our application organized. - Copy Command: The
COPY
command brings your JAR file into the image, and usingtarget/my-java-app.jar
points to the output of your Maven build. - Expose Command: This is crucial for routing traffic to your application.
- Run Command: The
CMD
directive ensures that our application starts when the container is run.
Step 2: Creating Kubernetes Deployment and Service
With your application containerized, the next step is to create a deployment and service for your Java app in Kubernetes.
Here is an example of a Kubernetes deployment file (deployment.yaml
):
apiVersion: apps/v1
kind: Deployment
metadata:
name: java-app
spec:
replicas: 3
selector:
matchLabels:
app: java-app
template:
metadata:
labels:
app: java-app
spec:
containers:
- name: java-app
image: your-docker-repo/java-app:latest
ports:
- containerPort: 8080
And a corresponding service configuration (service.yaml
):
apiVersion: v1
kind: Service
metadata:
name: java-app-service
spec:
type: LoadBalancer
selector:
app: java-app
ports:
- protocol: TCP
port: 80
targetPort: 8080
Commentary: Why This Configuration?
- Deployment:
- The
replicas
field ensures the app is highly available, with three instances running. - The
selector
field defines which pods belong to the deployment.
- The
- Service:
- Using
LoadBalancer
type makes the application accessible externally. - Connecting service ports to container ports enables smooth traffic routing.
- Using
Step 3: Deploying Your Java Application
Once your deployment and service files are ready, use kubectl
commands to deploy them:
kubectl apply -f deployment.yaml
kubectl apply -f service.yaml
Step 4: Verify Deployment
After deploying, verify the status of your application with:
kubectl get pods
kubectl get services
These commands provide crucial information about your pods and services, ensuring the deployment is running smoothly.
Troubleshooting Common Issues
Even with the best practices, you may encounter issues during deployment. For addressing common Kubernetes issues, refer to the article titled Overcoming Common Kubernetes Devspace Configuration Issues. This resource includes insightful techniques and troubleshooting tips for a seamless deployment experience.
Enhancing Your Deployment Strategy
Use ConfigMaps and Secrets
Managing configuration and sensitive data is necessary for any deployment. Kubernetes provides ConfigMaps
and Secrets
for managing configurations separately from your application code.
Here’s a brief example of a ConfigMap:
apiVersion: v1
kind: ConfigMap
metadata:
name: java-app-config
data:
SPRING_PROFILES_ACTIVE: "production"
This snippet illustrates how to manage environment variables outside your Docker image. You've created a cleaner way to manage configuration changes without a complete redeployment.
Monitoring and Logging
To maintain the health of your deployed applications, implement monitoring and logging practices. Tools like Prometheus for monitoring and ELK Stack for logging can vastly improve how you manage your applications.
Lessons Learned
Deploying Java applications on Kubernetes does not have to be a convoluted process. By following the outlined steps, employing best practices for configuration management, and utilizing monitoring tools, you can streamline your deployment process significantly.
Kubernetes opens up a significant realm of possibilities for Java developers. The complexity of modern infrastructure can be daunting, but with the right resources and practices, such as those mentioned above and the guidance provided in Overcoming Common Kubernetes Devspace Configuration Issues, you can successfully manage deployments with ease.
The demand for efficient and scalable Java application deployment will only increase, and embracing Kubernetes now will prepare you for future challenges in software development. Happy deploying!
By addressing both the technical and conceptual aspects, this blog post aims to educate and engage readers about deploying Java applications on Kubernetes effectively while guiding them toward additional resources for deeper exploration.
Checkout our other articles