Mastering Java: Avoiding Version Conflicts in Spring Boot
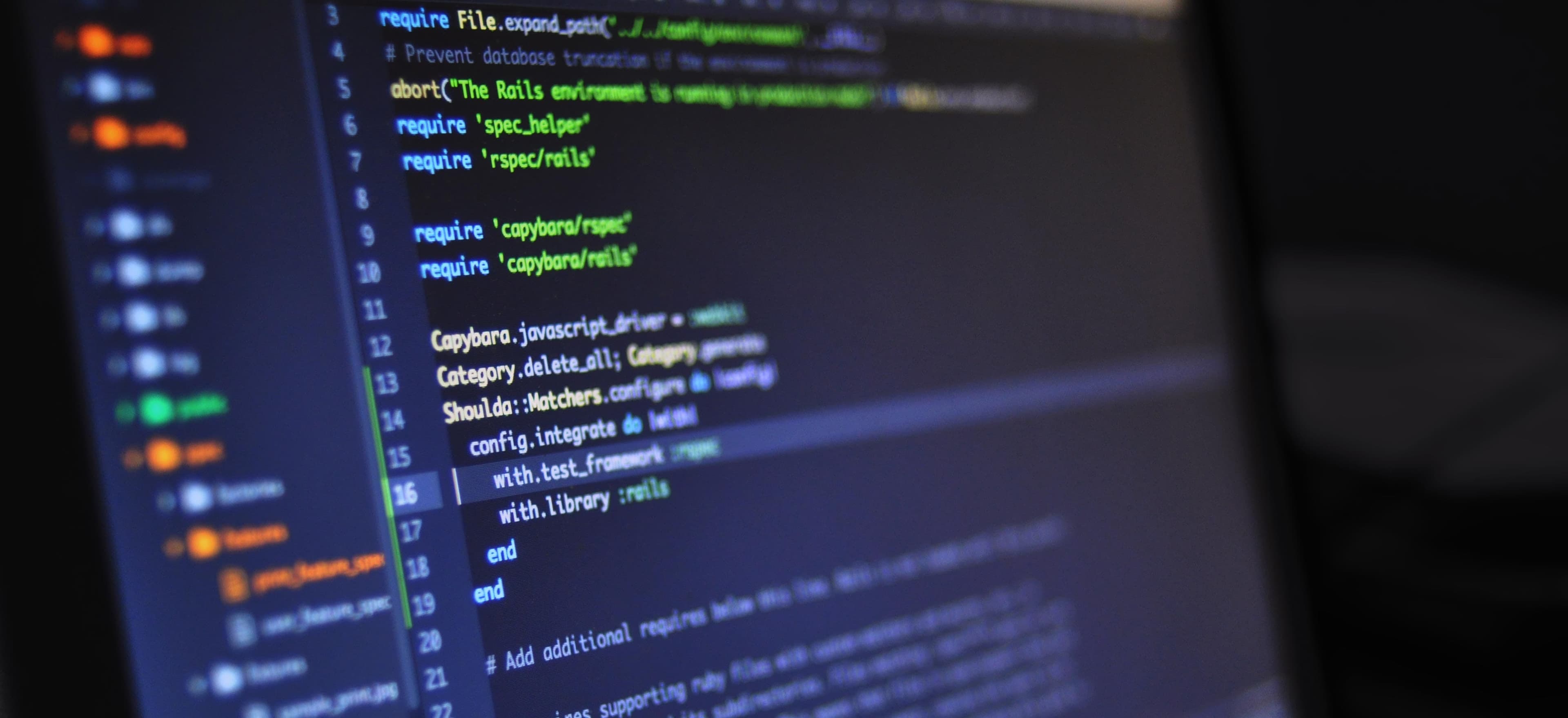
- Published on
Mastering Java: Avoiding Version Conflicts in Spring Boot
Java developers are no strangers to the importance of managing dependencies and their versions effectively. In large projects, especially those built on frameworks like Spring Boot, version conflicts can quickly turn into significant headaches. This blog post aims to provide you with the knowledge and tools necessary to avoid these pitfalls, enhancing your development experience and ensuring that your applications run smoothly.
Understanding Dependency Management in Spring Boot
Spring Boot simplifies the process of managing project dependencies through its starter dependencies. These are carefully curated dependencies that bring together various libraries that are compatible with each other and the Spring Framework. However, when you start adding new dependencies or upgrading existing ones, you may inadvertently introduce version conflicts.
What is a Version Conflict?
A version conflict occurs when two or more dependencies require different versions of the same library. This can lead to class loading issues, unexpected behaviors, or even application crashes. One common scenario in Spring Boot is when a new version of a library you depend on is not compatible with another one already included via a starter dependency.
Using the Spring Dependency Management Plugin
Spring provides a powerful dependency management capability through the Spring Dependency Management Plugin. This allows you to manage dependency versions in a centralized manner. Here's how to set it up in your build.gradle
file:
plugins {
id 'org.springframework.boot' version '2.5.4'
id 'io.spring.dependency-management' version '1.0.11.RELEASE'
id 'java'
}
group = 'com.example'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '11'
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter'
implementation 'org.springframework.boot:spring-boot-starter-web'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
Why Centralized Dependency Management?
By using the Spring Dependency Management Plugin, you can specify versions for certain libraries and ensure that all sub-dependencies respect these versions. This reduces the risk of conflicts and simplifies the process of updating libraries when needed. Centralized management also makes it easier to track the versions being used in your application, contributing to better maintainability.
Checking for Dependency Conflicts
One of the key strategies to avoid version conflicts is to regularly check for them. The Gradle Command Line Interface (CLI) offers a handy command to help you identify dependency problems:
./gradlew dependencies
Sample Output and Interpretation
The command above will produce an extensive tree of your dependencies. Pay close attention to any warning messages related to dependency resolution or potential conflicts. They typically appear as:
Conflicting dependency versions found for:
org.slf4j:slf4j-api:1.7.30
- used by com.example:your-app:0.0.1-SNAPSHOT
- used by org.springframework.boot:spring-boot-starter-logging:2.5.4
The Importance of Regular Checks
By running this command continuously, especially after updates to your dependencies, you can catch conflicts early before they affect your application. Not all conflicts produce build failures; some may result in hidden runtime errors that could be hard to debug.
Leveraging the Spring Boot Starter Ecosystem
Spring Boot's starters are designed to bring in all the necessary libraries and their compatible versions. Instead of manually specifying each dependency, leverage starters when possible. For example, if you're working with a web application, consider:
implementation 'org.springframework.boot:spring-boot-starter-web'
Why Use Starters?
Using a Spring Boot starter reduces the cognitive load of managing individual dependencies. You gain an entire suite of libraries, all compatible and tailored to work seamlessly together. This approach often leads to a significant reduction in versioning headaches, as starters are designed with dependency compatibility in mind.
Handling Transitive Dependencies
Sometimes, you might not even be directly dependent on a conflicting library. In these cases, the conflict arises from transitive dependencies introduced by libraries you include in your project.
To understand and manage transitive dependencies, you can:
- Use the
./gradlew dependencies
command to see how one library pulls in another. - Exclude a transitive dependency that might cause issues.
Example: Excluding a Transitive Dependency
dependencies {
implementation ('org.example:some-library') {
exclude group: 'org.slf4j', module: 'slf4j-api'
}
}
Why Exclude Transitive Dependencies?
Excluding unnecessary libraries can prevent conflicts and reduce your application's footprint. This strategy also allows you to define exactly which version of a library you want to include, thus taking full control of your dependency graph.
Keeping Dependencies Up to Date
An outdated library can often lead to version conflicts, particularly with more frequently updated libraries. It is advisable to periodically look at newer versions of your dependencies. Several tools can help automate this process, including:
- Versions plugin
- Spring Initializr for generating updated project structures.
Updating Dependencies Example
An example of updating a dependency in your build.gradle
would look like this:
implementation 'org.springframework.boot:spring-boot-starter-data-jpa:2.5.4' // New version
Why Stay Updated?
Keeping your libraries updated reduces security vulnerabilities and can introduce enhancements and bug fixes that improve your application's performance and security posture.
Resources for Further Reading
While this article provides insights on avoiding version conflicts in Spring Boot, the best practices around Node.js also offer valuable lessons. For an interesting read on managing versions in Node.js, check out the article Taming Node.js Versions: Fish Shell's Common Pitfalls. Although the context differs, many principles of dependency management and version control apply universally across programming environments.
In Conclusion, Here is What Matters
Navigating the complex web of dependencies in a Spring Boot application does not have to be a daunting task. By utilizing the best practices outlined in this article, such as employing centralized dependency management, regularly checking for conflicts, leveraging starters, managing transitive dependencies, and keeping everything updated, you'll mitigate the risks of version conflicts.
In the end, the goal is to make your development experience smoother and your applications more robust. Embrace these strategies, and you'll be well on your way to mastering Java and Spring Boot!
Feel free to share your experiences with dependency management in the comments below! What challenges have you faced, and how have you overcome them? Let's learn together!
Checkout our other articles