Efficient Java Pagination: Tips for Smooth Data Navigation
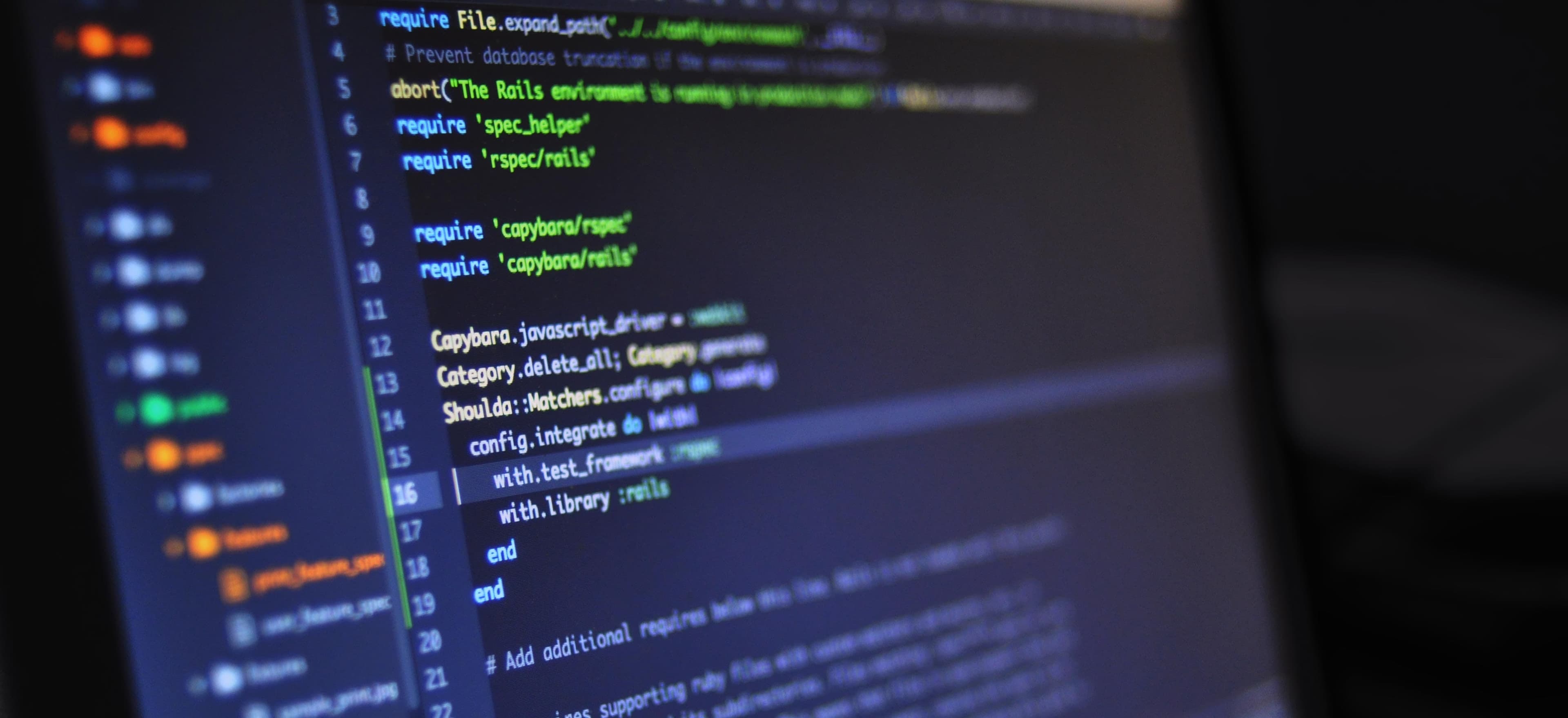
- Published on
Efficient Java Pagination: Tips for Smooth Data Navigation
In today's data-driven world, efficient pagination is essential for applications dealing with large datasets. Pagination provides a way to divide data into manageable chunks, which enhances performance and user experience. In this blog post, we will explore best practices for implementing pagination in Java, complete with code examples, detailed explanations, and key insights to ensure smooth data navigation.
Why Use Pagination?
Pagination is crucial for various reasons:
- Improved Performance: Loading a small subset of data reduces processing time and memory usage.
- Enhanced User Experience: Users can navigate through data more comfortably and find the information they need quickly.
- Reduced Server Load: By only retrieving a small set of records, server resources are used more efficiently.
Basic Concepts of Pagination
Before diving into code, let’s clarify some fundamental concepts related to pagination:
- Page Number: Indicates the current page of data being displayed.
- Page Size: Specifies how many records should be displayed per page.
- Offset: The number of records to skip when retrieving data for a specific page.
Simple Pagination Logic
Let's illustrate these concepts with a simple example:
- Suppose you have a total of 100 records.
- If you want to display 10 records per page, the first page will show records 1 to 10, the second page will show records 11 to 20, and so on.
Pagination Formulae
To calculate which records to retrieve based on the current page and page size, you can use the following formula:
offset = (currentPage - 1) * pageSize
Where:
currentPage
is the number of the page the user wants to view.pageSize
is how many records are displayed on each page.
Implementing Pagination in Java
We'll create a simple example of pagination in a Java application. For this example, we'll assume we have a list of some data objects.
Sample Data
First, let’s create a sample data class called Item
:
public class Item {
private int id;
private String name;
public Item(int id, String name) {
this.id = id;
this.name = name;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
}
Generating Sample Data
Next, let’s create a method to generate a list of items:
import java.util.ArrayList;
import java.util.List;
public class DataGenerator {
public static List<Item> generateItems(int totalItems) {
List<Item> items = new ArrayList<>();
for (int i = 1; i <= totalItems; i++) {
items.add(new Item(i, "Item " + i));
}
return items;
}
}
This code creates a list of Item
objects, named according to their ID. Generating sample data helps you test pagination logic without needing a database connection at this stage.
Implementing Pagination Logic
Now, let’s implement the pagination logic. The following method retrieves a sublist of items based on the current page and page size:
import java.util.List;
public class PaginationUtil {
public static List<Item> paginate(List<Item> items, int currentPage, int pageSize) {
int fromIndex = (currentPage - 1) * pageSize;
if (fromIndex >= items.size()) {
return List.of(); // Return empty list if the page index exceeds the list size
}
int toIndex = Math.min(fromIndex + pageSize, items.size());
return items.subList(fromIndex, toIndex);
}
}
Code Explanation
-
Calculating
fromIndex
: It determines where to start retrieving records. If the user requests the third page with a page size of ten,fromIndex
would be 20 ((3-1)*10
). -
Handling Out-of-Bounds: If the
fromIndex
exceeds the size of the list, we return an empty list. -
Calculating
toIndex
: Ensures we do not exceed the total number of items. It usesMath.min
to prevent index overflow.
Example Usage
Now let's see how to use these classes together:
public class PaginationDemo {
public static void main(String[] args) {
List<Item> items = DataGenerator.generateItems(100); // generate 100 items
int pageSize = 10;
int currentPage = 3; // fetching the third page
List<Item> paginatedItems = PaginationUtil.paginate(items, currentPage, pageSize);
// Display paginated items
for (Item item : paginatedItems) {
System.out.println(item.getId() + ": " + item.getName());
}
}
}
Output
Running this code will output items 21 to 30. The pagination logic works perfectly by extracting the correct subset of data based on the requested page and the specified page size.
Advanced Pagination Techniques
While the basic pagination strategy is straightforward and effective for many scenarios, sometimes more advanced techniques may be required.
1. Cursor-Based Pagination
This technique uses a unique identifier in place of a page number. Instead of loading data based on page numbers, the application retrieves records starting from a specific identifier. This method is particularly useful for ensuring data integrity, especially in real-time applications where records are frequently updated.
2. Keyset Pagination
Keyset pagination is similar to cursor-based pagination. It uses the last record fetched as a reference point for the next batch of records. This approach leads to better performance, especially with large datasets, but requires that the dataset be ordered by a unique column.
When to Use Each Technique
The choice of pagination strategy depends on application requirements and the nature of the dataset. For a more elaborate discussion on pagination techniques in PHP, check out the article titled Mastering Pagination in PHP: Slider Implementation Tips.
A Final Look
Effective pagination is essential for building scalable and user-friendly applications. By implementing smart pagination strategies, Java developers can significantly improve data navigation experiences. Whether employing simple or advanced techniques, the principles of pagination remain similar. Always ensure that your pagination logic is efficient, user-oriented, and adaptable to varying data conditions.
With the understanding of both the simple and advanced techniques discussed, you should be well-equipped to implement pagination in your Java applications. Happy coding!
Checkout our other articles