Enhance Your Java Apps: Customizing HTML Form Colors
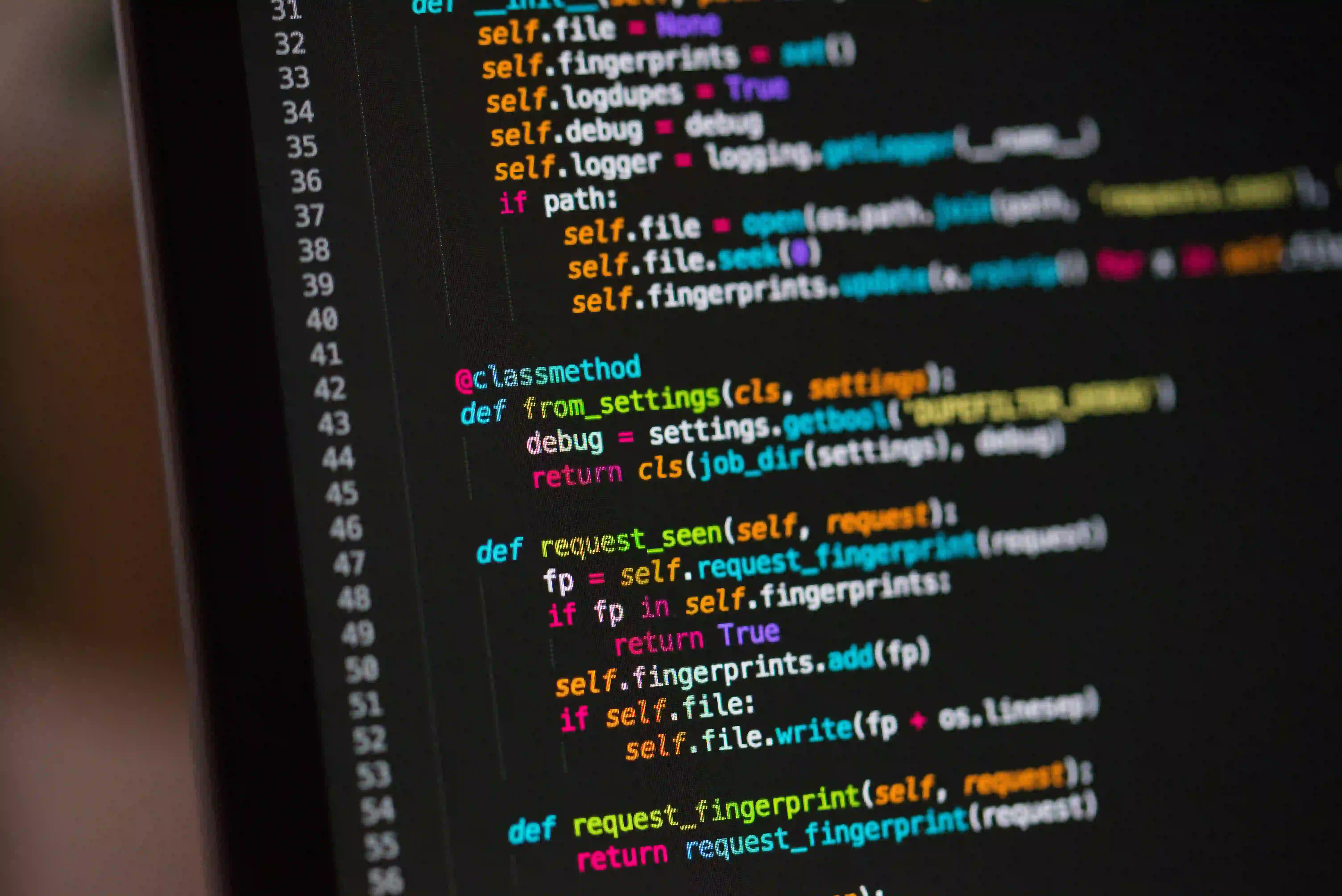
Enhance Your Java Apps: Customizing HTML Form Colors
When developing web applications using Java, it's essential to ensure that your user interfaces are not only functional but also visually appealing. One effective way to enhance the user experience is by customizing the colors of HTML form elements. This guide will delve into the significance of color customization, the technical aspects required for implementation, and will provide a practical example to get you started.
Understanding the Importance of Colors in UI
Colors play a pivotal role in user interface design. They can convey emotions, signal actions, and guide users through forms. A well-chosen color palette not only enhances aesthetic appeal but aids in usability and accessibility.
-
Emphasizing Action: Use brighter colors for call-to-action buttons to draw user attention.
-
Brand Identity: Align colors with your company's branding to create a cohesive experience.
-
User Comfort: Use contrasting colors for text and background to improve readability.
For a deeper dive into how color impacts HTML forms, consider reading the article How to Change Text Color in HTML Form Elements.
How to Change Text Colors in HTML Form Elements through Java Servlets
In Java applications that serve HTML content, you can manipulate form colors dynamically with a blend of Java and CSS. Below, we outline a simple example illustrating how to apply custom colors to form elements using Java Servlets.
Setting Up Your Java Servlet
First, ensure you have a Java Servlet environment set up. We will use Apache Tomcat for this purpose.
-
Create a New Servlet: Start by creating a servlet that generates HTML content.
☕snippet.javaimport java.io.IOException; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; @WebServlet("/colorForm") public class ColorFormServlet extends HttpServlet { @Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html"); // Generating HTML content with inline CSS for color customization String htmlResponse = "<html>" + "<head><title>Custom Color Form</title></head>" + "<body>" + "<h2>Custom Form Colors in Java</h2>" + "<form action='#'>" + "<label for='name'>Name:</label>" + "<input type='text' id='name' name='name' style='color:blue;'><br><br>" + "<label for='email'>Email:</label>" + "<input type='email' id='email' name='email' style='color:red;'><br><br>" + "<input type='submit' value='Submit' style='background-color: green; color: white;'>" + "</form>" + "</body>" + "</html>"; response.getWriter().write(htmlResponse); } }
Explanation of the Code
- HTTPServlet: We extend
HttpServlet
to create a servlet that handles HTTP requests. - doGet Method: This method processes HTTP GET requests. In it, we build our HTML response.
- Content Type: We set the content type to
text/html
to ensure that the browser interprets the response correctly. - Inline CSS Styles: We customize the colors of the input fields directly within the
style
attribute.
This approach allows you to define specific colors for HTML form elements. You can replace the colors with any CSS colors of your choice - hexadecimal, RGB values, or CSS named colors.
Testing the Servlet
To see your form in action:
- Run your servlet on Apache Tomcat.
- Navigate to
http://localhost:8080/yourapp/colorForm
to see your colorful form.
Applying External CSS
For a more organized approach, consider using external CSS rather than inline styles. Here's how to accomplish that:
-
Create a Separate CSS File:
styles.css
📄snippet.txt/* styles.css */ body { font-family: Arial, sans-serif; } input[type="text"] { color: blue; } input[type="email"] { color: red; } input[type="submit"] { background-color: green; color: white; }
-
Modify Your Servlet to Link the CSS:
☕snippet.java@Override protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { response.setContentType("text/html"); // Generating HTML content with external CSS for color customization String htmlResponse = "<html>" + "<head><link rel='stylesheet' type='text/css' href='styles.css'><title>Custom Color Form</title></head>" + "<body>" + "<h2>Custom Form Colors in Java</h2>" + "<form action='#'>" + "<label for='name'>Name:</label>" + "<input type='text' id='name' name='name'><br><br>" + "<label for='email'>Email:</label>" + "<input type='email' id='email' name='email'><br><br>" + "<input type='submit' value='Submit'>" + "</form>" + "</body>" + "</html>"; response.getWriter().write(htmlResponse); }
Benefits of Using External CSS
- Separation of Concerns: It separates presentation logic from business logic.
- Maintainability: You can manage styles from one location instead of littering your HTML with inline styles.
- Reusability: The CSS file can be reused across multiple pages, creating a consistent look and feel.
Summary
Color customization is a small yet impactful aspect of creating engaging Java applications. By dynamically modifying HTML form element colors, you not only enhance the user interface but also improve user experience.
Remember to consistently test your applications on different devices and browsers to ensure the fidelity of your color schemes, as these may render differently depending on various factors.
For more details on customizing HTML and CSS, refer to the article How to Change Text Color in HTML Form Elements.
This guide should help you get started with customizing your input fields, encouraging you to experiment with different styles, colors, and layouts. Happy coding!