Java File Upload: Solving Common Display Issues
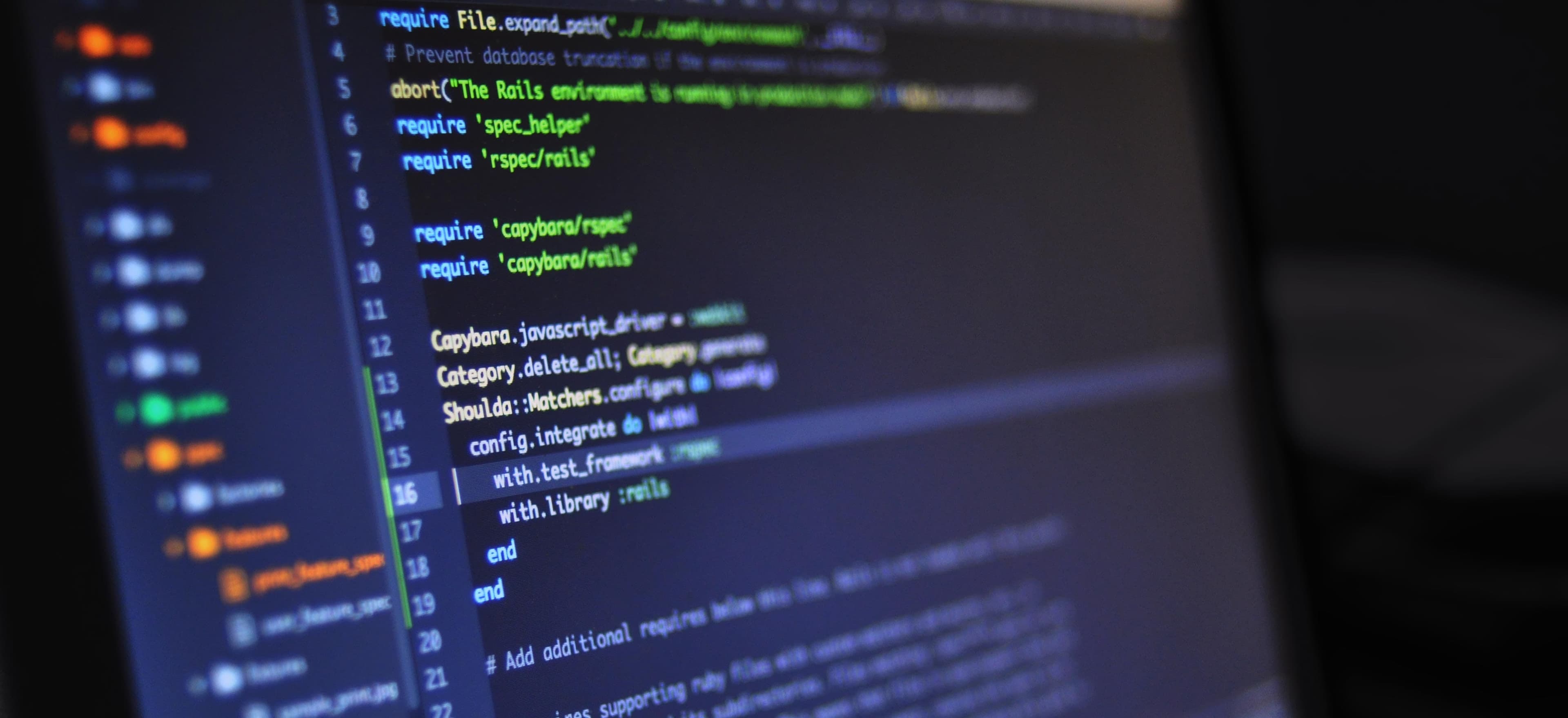
- Published on
Java File Upload: Solving Common Display Issues
File uploads are an essential feature in web applications, allowing users to share documents, images, and other files. However, just like with any feature, challenges can arise when implementing file upload functionality. If you're transitioning from another programming language like PHP, you might run into issues similar to those described in Troubleshooting PHP File Display Issues. This article aims to highlight common display issues faced during Java file uploads and how to solve them.
Understanding the Basics of File Upload in Java
Before we dive into common problems, let’s briefly outline how file uploads typically work in Java.
How File Uploads Work
Java file uploads commonly rely on a combination of:
- Servlets: For handling request and response objects.
- HTML Forms: For enabling user interactions.
- Multipart Request Libraries: Like Apache Commons FileUpload, enabling easy handling of file uploads.
Here’s a simple example of an HTML form for uploading a file:
<form action="upload" method="post" enctype="multipart/form-data">
<input type="file" name="file" />
<button type="submit">Upload</button>
</form>
In this example, we specify enctype="multipart/form-data"
to handle files properly. Now, let's create the server-side component responsible for processing this request.
Basic Servlet for File Upload
import java.io.File;
import java.io.FileOutputStream;
import java.io.InputStream;
import javax.servlet.ServletException;
import javax.servlet.annotation.MultipartConfig;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.Part;
@WebServlet("/upload")
@MultipartConfig
public class FileUploadServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, java.io.IOException {
// Retrieve the file part from the request
Part filePart = request.getPart("file");
// Get file name
String fileName = getFileName(filePart);
// Define a path to save the file (ensure it's valid)
File file = new File("C:/uploads/" + fileName);
try (InputStream fileContent = filePart.getInputStream();
FileOutputStream fos = new FileOutputStream(file)) {
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = fileContent.read(buffer)) != -1) {
fos.write(buffer, 0, bytesRead);
}
}
response.getWriter().println("File uploaded successfully!");
}
private String getFileName(Part part) {
String contentDisp = part.getHeader("content-disposition");
String[] items = contentDisp.split(";");
for (String s : items) {
if (s.trim().startsWith("filename")) {
return s.substring(s.indexOf("=") + 2, s.length() - 1);
}
}
return "";
}
}
Explanation of the Code
- Servlet Annotation: The
@WebServlet
annotation maps the servlet to a specific URL. - @MultipartConfig: Enables the servlet to handle file uploads.
- doPost Method: This method processes the incoming POST request containing the file.
- File Handling: We retrieve the
Part
of the uploaded file, extract its name, and then write it to the designated storage location.
Now that we have a basic upload functionality in place, let's explore some common display issues developers face.
Common Display Issues and Solutions
1. Missing File in Directory
Issue: Often, files appear to upload successfully, but they are not found in the designated directory.
Solution: Ensure that the application has the proper permissions to write to the directory. In addition, verify that the directory path exists.
Check your setup like this:
File uploadDir = new File("C:/uploads");
if (!uploadDir.exists()) {
uploadDir.mkdirs(); // Create the directory if it doesn't exist
}
2. File Size Limitations
Issue: By default, many web servers and servlet containers impose restrictions on the maximum file size.
Solution: Adjust the @MultipartConfig
annotation to include maxFileSize
and maxRequestSize
.
@MultipartConfig(maxFileSize = 1024 * 1024 * 5, // 5 MB
maxRequestSize = 1024 * 1024 * 10) // 10 MB
public class FileUploadServlet extends HttpServlet {
// ...
}
3. Incorrect Content Types
Issue: Sometimes files do not display correctly due to incorrect MIME types being set.
Solution: Always validate the file’s content type before processing it. Create a helper method to check acceptable file types.
private boolean isValidFileType(Part filePart) {
String contentType = filePart.getContentType();
return contentType.equals("image/jpeg") || contentType.equals("image/png");
}
4. Security Restrictions
Issue: Users might experience issues if they attempt to upload files that violate security restrictions.
Solution: Implement checks to disallow certain types of files from being uploaded, such as executables.
if (!isValidFileType(filePart)) {
response.getWriter().println("Invalid file type.");
return;
}
5. Interfacing with Other Technologies
Issue: Your Java application might not play well with other technologies, which can lead to display issues.
Solution: Ensure that your application can properly serve files, and maybe look at using libraries such as Spring MVC, which can make file handling easier.
@Controller
public class FileDownloadController {
@RequestMapping(value = "/files/{fileName:.+}", method = RequestMethod.GET)
@ResponseBody
public ResponseEntity<Resource> downloadFile(@PathVariable String fileName) {
Resource resource = // Load your file resource here
return ResponseEntity.ok()
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + resource.getFilename() + "\"")
.body(resource);
}
}
Closing Remarks
File uploading in Java can be straightforward, but various challenges arise during implementation. By understanding the common issues highlighted in this article, you can ensure a smoother experience when handling file uploads in your Java applications.
For further knowledge on troubleshooting file issues across different languages, consider reading the article Troubleshooting PHP File Display Issues. This comparative perspective might deepen your understanding of file handling nuances across programming environments.
With the right approach and careful coding practices, you can effectively manage file uploads in Java and mitigate any challenges that may arise. Happy coding!
Checkout our other articles