Resolving Java File Handling Issues in Web Applications
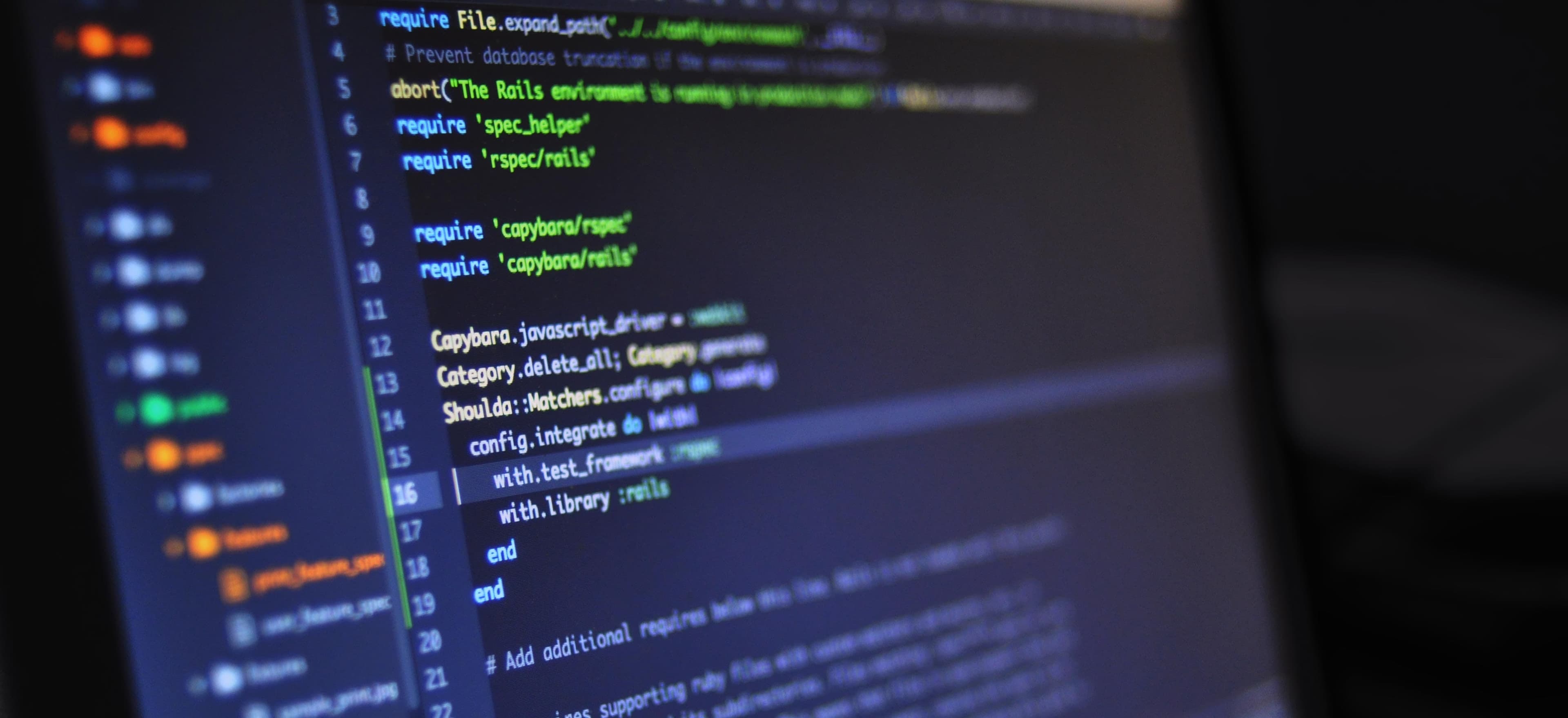
- Published on
Resolving Java File Handling Issues in Web Applications
In the world of web development, file handling—specifically dealing with the uploading, downloading, and management of files—is a critical component. Java, with its robust file handling libraries and frameworks, provides an extensive toolkit for developers to manage files effectively. However, just like any technology, developers can encounter issues while implementing file handling in Java-based web applications.
This comprehensive guide aims to provide insights into common file handling issues in Java web applications and offers proactive strategies to tackle these challenges. Additionally, we'll sprinkle in relevant links for further reading, including an article on PHP file display issues that highlights concepts that resonate across languages, such as Java.
Understanding File Handling in Java
Before diving into the common problems and solutions, let’s briefly explore how file handling is managed in Java.
Java offers built-in classes in the java.nio.file
package and java.io
package that allow developers to work with files easily. The most commonly used classes are:
- File: Represents a file or directory path in the file system.
- FileInputStream & FileOutputStream: Used for reading and writing byte data.
- BufferedReader & BufferedWriter: For efficient reading and writing of character data.
- FileReader & FileWriter: Specifically for handling character streams.
Example: Reading a File
Here’s a simple example of reading a text file using BufferedReader
:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReadExample {
public static void main(String[] args) {
String filePath = "example.txt"; // Path to the file
// Using try-with-resources to ensure resources are closed
try (BufferedReader br = new BufferedReader(new FileReader(filePath))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
System.err.println("Error reading the file: " + e.getMessage());
}
}
}
Why Use BufferedReader?
Using BufferedReader
enhances performance because it reads data in chunks rather than single bytes. This approach minimizes the number of I/O operations, which can significantly speed up file handling tasks especially for larger files.
Common File Handling Issues in Java Web Applications
While Java provides robust utilities for file handling, several common issues may arise:
- File Not Found Exception
- Access Denied Issues
- File Format and Encoding Problems
- Memory Leaks
- Inconsistent File Paths
- Concurrency Problems in Multi-threaded Applications
Let’s delve into these issues and outline practical resolutions.
1. File Not Found Exception
The most frequent issue encountered by developers is the FileNotFoundException
. This typically occurs due to incorrect file paths.
Resolution:
-
Absolute vs. Relative Paths: Ensure that you are using the correct path. Relative paths are relative to the execution directory of the application. Using absolute paths can eliminate ambiguities.
-
Check Resource Location: When dealing with web applications, ensure that resources are located in the appropriate directories, typically within the
/src/main/resources
folder for Maven-based projects.
2. Access Denied Issues
Security is paramount, and users may encounter permission issues when attempting to read or write files.
Resolution:
-
File Permissions: When deploying applications, check the file permissions on your server or environment. Use commands like
chmod
in Unix/Linux to modify permissions as needed. -
Use Java Security Manager: Implement a security manager to enforce access control at the application level.
3. File Format and Encoding Problems
Working with various file formats (like CSV, XML, JSON) often exposes encoding issues.
Resolution:
- Specify Encoding: When reading or writing files, always specify the file encoding:
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.InputStreamReader;
public class FileWithEncoding {
public static void main(String[] args) {
String filePath = "example.csv";
try (BufferedReader reader = new BufferedReader(
new InputStreamReader(new FileInputStream(filePath), "UTF-8"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this code, we ensure the correct encoding (UTF-8) is used while reading the file, which helps avoid common issues with special characters.
4. Memory Leaks
Failure to close file streams can lead to memory leaks, causing the application to slow down or crash.
Resolution:
- Try-With-Resources: Always use the try-with-resources statement to manage file streams. This ensures that streams are closed automatically.
5. Inconsistent File Paths
Different environments (development, staging, production) can have different file paths leading to deployment errors.
Resolution:
- Configuration Files: Store file paths in configuration files. Your application can then read these configurations at runtime, enabling it to adapt to different environments without code changes.
6. Concurrency Problems in Multi-threaded Applications
When multiple threads access or modify a file simultaneously, it can result in data corruption.
Resolution:
- Synchronized Blocks: Use synchronized blocks or the
ReentrantLock
class for controlling access to files, ensuring that only one thread can access a file at a time.
Example: Synchronized File Writing
import java.io.FileWriter;
import java.io.IOException;
public class SyncFileWrite {
private static final Object lock = new Object();
public static void writeToFile(String content) {
synchronized (lock) {
try (FileWriter writer = new FileWriter("output.txt", true)) {
writer.write(content + "\n");
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
This example ensures that the writeToFile
method can safely be called by multiple threads without corrupting the file.
The Last Word
Java offers robust solutions for file handling in web applications, but developers must remain vigilant against common issues. By following best practices, such as utilizing the try-with-resources pattern, managing file paths through configurations, and ensuring proper access control, these issues can be mitigated effectively.
For those interested in deeper dives into troubleshooting file display issues, consider checking out Troubleshooting PHP File Display Issues, which outlines similar challenges in the PHP ecosystem and can provide insights applicable to Java as well.
In the world of web applications, ensuring seamless file handling is indispensable for robust performance and user satisfaction. Making proactive adjustments based on the issues discussed in this article will significantly enhance the reliability of your Java web applications.
Checkout our other articles