Fixing Java File Upload Problems: Common Solutions
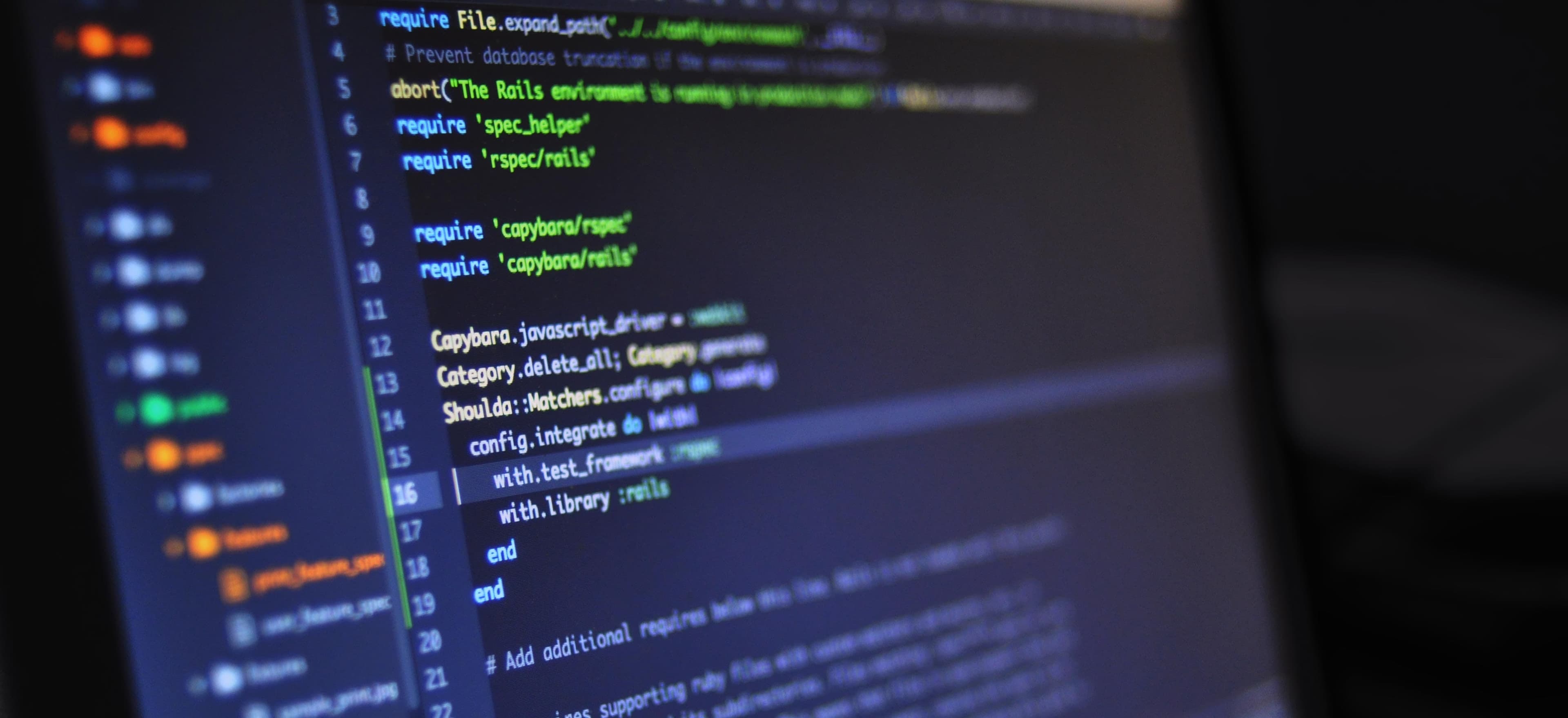
- Published on
Fixing Java File Upload Problems: Common Solutions
File uploading is a crucial feature in web applications, enabling users to exchange documents, images, and other file types effortlessly. Yet, despite its significance, many developers face issues when implementing file uploads in Java applications, leading to frustrating user experiences. In this post, we will explore common file upload problems in Java, discuss their causes, and provide practical solutions.
Understanding the File Upload Process in Java
Before diving into problem-solving, it's pertinent to understand how file uploads work in Java. When a user uploads a file, the file is typically sent as part of an HTTP request. The server receives this request and processes the incoming data to store the file on the server.
Java Servlets commonly handle this process. They can read the multipart request—different parts of which can include file data, text inputs, etc.—facilitating easy file handling.
Example of a Basic File Upload Servlet
Here's a simple example of a Java Servlet that handles file uploads:
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.InputStream;
import javax.servlet.ServletException;
import javax.servlet.annotation.MultipartConfig;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.Part;
@WebServlet("/upload")
@MultipartConfig
public class FileUploadServlet extends HttpServlet {
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
Part filePart = request.getPart("file"); // Retrieves <input type="file" name="file">
String fileName = getFileName(filePart);
File file = new File("/uploads/" + fileName);
try (InputStream fileContent = filePart.getInputStream();
FileOutputStream fos = new FileOutputStream(file)) {
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = fileContent.read(buffer)) != -1) {
fos.write(buffer, 0, bytesRead);
}
}
response.getWriter().print("File uploaded successfully: " + fileName);
}
private String getFileName(Part part) {
for (String content : part.getHeader("content-disposition").split(";")) {
if (content.trim().startsWith("filename")) {
return content.substring(content.indexOf('=') + 2, content.length() - 1);
}
}
return null;
}
}
Why This Code Matters
@MultipartConfig
: This annotation tells the servlet to handle multipart requests. It's essential for handling file uploads.Part
: It represents a file part of the request, making it easier to manage file data.- InputStream and FileOutputStream: These classes are vital for reading incoming file data and writing it to the server, ensuring that the file is transferred correctly.
Common Problems and Solutions
Now that we've covered the basics, let's investigate some frequent file upload problems developers encounter, along with their solutions.
1. File Size Limit Exceeded
Problem: Java applications may refuse to upload files that exceed the defined size limits, commonly resulting in an IOException
.
Solution: Adjust the limits set in your servlet configuration:
@MultipartConfig(maxFileSize = 10485760) // 10 MB
This adjustment allows files to upload up to 10 MB in size. Always ensure you set this limit according to the needs of your application and server capabilities.
2. Unsupported File Type
Problem: Users frequently attempt to upload unsupported file types, leading to confusion and failed uploads.
Solution: Implement validation logic to check the file type:
String contentType = filePart.getContentType();
if (!contentType.equals("image/png") && !contentType.equals("image/jpeg")) {
throw new ServletException("Unsupported file type: " + contentType);
}
By providing meaningful error messages to users, you can improve their experience while preventing unnecessary uploads.
3. Incorrect File Path
Problem: Determining where to save uploaded files can be troublesome, especially when absolute paths are relied on without consideration for different environments (like local vs. production).
Solution: Use relative paths or configuration settings allowing flexibility. For example:
String uploadPath = getServletContext().getRealPath("") + File.separator + "uploads";
File uploadDir = new File(uploadPath);
if (!uploadDir.exists()) uploadDir.mkdir();
By utilizing getServletContext().getRealPath("")
, we ensure the application creates the uploads directory relative to the context.
4. Permissions Issues
Problem: Sometimes, uploaded files cannot be saved due to insufficient permissions on the directory or drive.
Solution: Check and adjust file permissions on the server. Ensure that the directory where files are being saved has write permissions set for the user running the Java application.
5. Timeout Errors
Problem: Uploading large files may lead to timeout errors due to server limits.
Solution: Adjust server settings to allow for longer processing times. For example, in Tomcat, update settings in server.xml
:
<Connector port="8080" protocol="HTTP/1.1"
connectionTimeout="20000"
redirectPort="8443" />
By increasing the connectionTimeout
, you allow the server more time to process uploads before timing out.
6. Client-side Validation Fails
Problem: Users may upload files without any error-checking unless you implement client-side validation.
Solution: Use JavaScript to enforce validation before files are uploaded. In the following example, we prevent users from uploading files larger than a certain limit:
<input type="file" id="fileInput" onchange="validateSize()"/>
<script>
function validateSize() {
const fileInput = document.getElementById('fileInput');
const file = fileInput.files[0];
if (file.size > 10485760) { // 10 MB
alert("File size exceeds 10 MB");
fileInput.value = ""; // Reset the file input
}
}
</script>
This validation adds another layer of security and usability, preventing users from attempting to upload files that will be rejected server-side.
Closing Remarks
File uploading in Java can be straightforward but is often complicated by a variety of factors. Whether it’s size limits, unsupported file types, or server configuration, understanding these common file upload problems can greatly enhance your ability to troubleshoot effectively.
By applying the solutions outlined in this guide, you can create a robust file upload feature in your Java applications, which provides a seamless experience for users.
For additional information on handling file issues with PHP, you may find the article titled Troubleshooting PHP File Display Issues helpful.
By being proactive and implementing good practices, you'll not only smooth the user experience but also fortify your application against potential uploading pitfalls. Happy coding!
Checkout our other articles