Maximizing Java Efficiency: The Role of Data Analysis
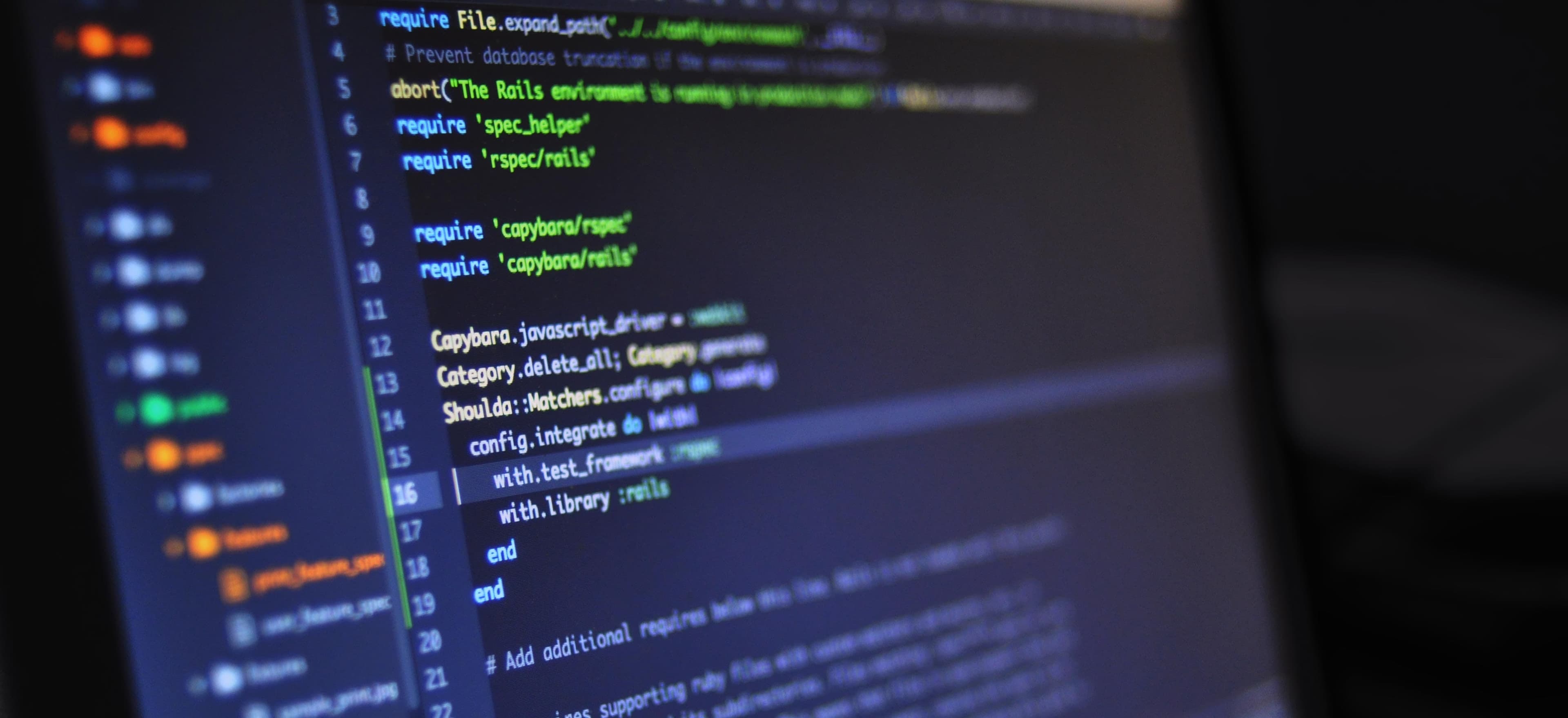
- Published on
Maximizing Java Efficiency: The Role of Data Analysis
In the ever-evolving landscape of software development, Java remains a steadfast programming language, powering everything from enterprise applications to mobile applications. However, as the complexity of applications increases, so does the need for efficiency and optimization in Java programs. One pivotal aspect of achieving such efficiency is through data analysis. In this blog post, we will explore how data analysis techniques can optimize Java applications and enhance performance, while also providing practical code snippets to demonstrate these concepts in action.
The Importance of Data Analysis in Java
Data analysis involves collecting, processing, and analyzing data to derive meaningful insights, which can significantly influence the performance of Java applications. By implementing data-driven approaches, developers can identify performance bottlenecks, streamline processes, and make informed decisions about resource allocation.
Moreover, data analysis enables predictive analytics, allowing Java applications to anticipate user behavior and adapt accordingly. This not only improves user experience but also increases operational efficiency. To learn more about the importance of mathematics in driving such growth and success, refer to Mathematik in der Wirtschaft: Ein Schlüssel zum Erfolg.
Key Areas Where Data Analysis Enhances Java Efficiency
1. Performance Monitoring
Performance monitoring is essential for understanding how an application behaves under different conditions. By analyzing performance metrics—such as memory usage, CPU load, and response times—developers can pinpoint inefficiencies.
Example Code Snippet: Performance Monitoring with JMX
Java Management Extensions (JMX) can be employed for performance monitoring. Here’s how to implement a simple JMX monitor:
import javax.management.*;
import java.lang.management.ManagementFactory;
public class JMXMonitor {
private final MBeanServer mBeanServer;
private final ObjectName objectName;
public JMXMonitor() throws MalformedObjectNameException {
mBeanServer = ManagementFactory.getPlatformMBeanServer();
objectName = new ObjectName("com.example:type=Monitoring");
mBeanServer.registerMBean(this, objectName);
}
public void printMemoryUsage() {
MemoryMXBean memoryMXBean = ManagementFactory.getMemoryMXBean();
MemoryUsage heapMemoryUsage = memoryMXBean.getHeapMemoryUsage();
System.out.println("Heap Memory Used: " + heapMemoryUsage.getUsed() + " bytes");
}
public static void main(String[] args) {
try {
JMXMonitor monitor = new JMXMonitor();
monitor.printMemoryUsage();
} catch (Exception e) {
e.printStackTrace();
}
}
}
In this snippet, we define a JMX MBean that registers itself to monitor heap memory usage. Monitoring memory ensures we spot potential memory leaks early, which aids in improving overall application efficiency.
2. Profiling
Profiling involves measuring the size and frequency of various activity measures in your Java application, providing insights into where improvements can be made.
Example Code Snippet: Profiling with Java VisualVM
Although not a code snippet per se, profiling tools like Java VisualVM allow developers to assess application performance visually. To use Java VisualVM:
- Start your application with the following command:
java -javaagent:/path/to/visualvm.jar -jar yourapp.jar
- Open VisualVM, connect to your application, and monitor CPU and memory usage.
This visual approach to analyzing performance bottlenecks offers a powerful way to optimize your code based on real data.
3. Load Testing
Load testing emulates a significant number of users interacting with an application simultaneously. By analyzing the performance data generated during load tests, developers can identify scalability issues.
Example Code Snippet: Using Apache JMeter
Apache JMeter is a popular tool for load testing Java applications. Here’s a brief outline of a JMeter test configuration:
<TestPlan>
<ThreadGroup>
<ThreadGroupName>User Load Test</ThreadGroupName>
<NumThreads>100</NumThreads>
<RampTime>300</RampTime>
<Sampler>
<HTTPRequest>
<URL>http://yourapp.com/api/resource</URL>
</HTTPRequest>
</Sampler>
</ThreadGroup>
</TestPlan>
In this example, we create a test plan to simulate 100 users accessing an API endpoint. Analyzing the results from such tests helps you understand the maximum load your server can handle and where the application might suffer delays.
4. Utilizing Caching Strategies
Caching frequently accessed data improves application speed and reduces database load. Analyzing which data to cache can maximize efficiency.
Example Code Snippet: Simple Caching in Java
import java.util.HashMap;
public class SimpleCache {
private final HashMap<String, String> cache = new HashMap<>();
public String getFromCache(String key) {
return cache.get(key);
}
public void putInCache(String key, String value) {
cache.put(key, value);
}
public static void main(String[] args) {
SimpleCache cache = new SimpleCache();
cache.putInCache("user1", "John Doe");
// Retrieve from cache
String user = cache.getFromCache("user1");
System.out.println("Cached User: " + user);
}
}
In this code snippet, we implement a basic cache using a HashMap. Proper caching strategies minimize redundant database queries and serve user requests faster, thereby enhancing application efficiency.
5. Decision-Making and Predictive Analytics
Utilizing data analysis techniques for decision-making and predictive analytics can dramatically improve application responsiveness.
Example Code Snippet: Implementing a Simple Predictive Model
Incorporating machine learning techniques in your analysis can predict user behavior, like so:
import weka.classifiers.trees.J48;
import weka.core.Instances;
import weka.core.converters.ConverterUtils.DataSource;
public class PredictiveAnalytics {
public static void main(String[] args) throws Exception {
DataSource source = new DataSource("user_data.arff");
Instances data = source.getDataSet();
data.setClassIndex(data.numAttributes() - 1);
J48 model = new J48(); // Decision tree classifier
model.buildClassifier(data);
System.out.println("Classifier built successfully!");
}
}
In the snippet above, we utilize Weka, a powerful library for data mining tasks in Java. Building predictive models allows for proactive adjustments to application behavior, thus maximizing efficiency in user interactions.
A Final Look
Maximizing Java efficiency through data analysis is not merely an option, but a necessity in today’s competitive environment. By leveraging performance monitoring, profiling, load testing, caching strategies, and predictive analytics, developers can ensure their applications run optimally while delivering an unparalleled user experience.
Implementing these data analysis techniques in Java helps detect issues early, optimize resources, and ultimately leads to higher application performance. As data-driven decision-making becomes the norm, proficiency in data analysis will undoubtedly become a critical skill for Java developers.
For further insights into how mathematical principles are integrated into business success, check out Mathematik in der Wirtschaft: Ein Schlüssel zum Erfolg.
By embracing these practices, Java developers can position themselves at the forefront of innovation and efficiency. Start analyzing your data today and unlock the full potential of your Java applications!