Optimizing Java Algorithms for Economic Problem Solving
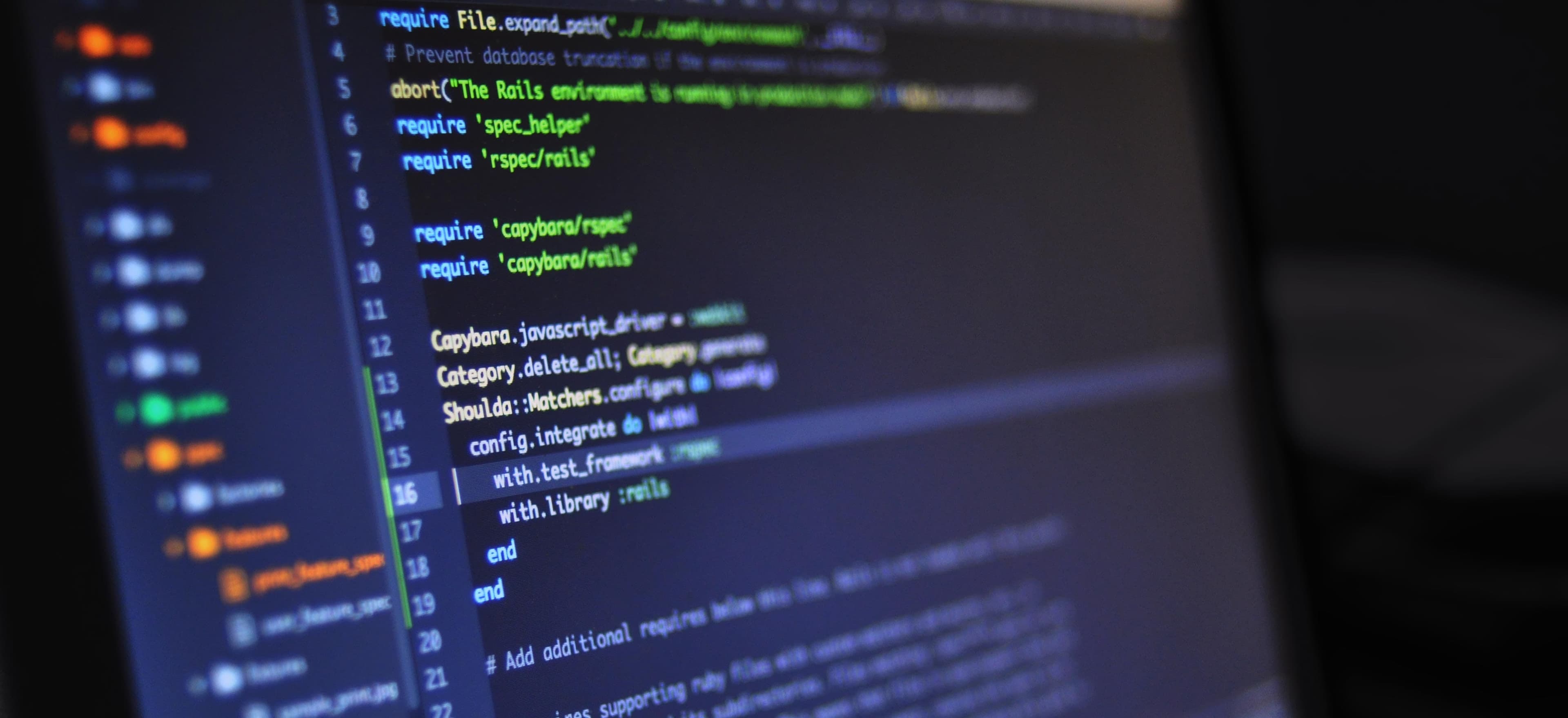
- Published on
Optimizing Java Algorithms for Economic Problem Solving
In today's data-driven world, understanding the synergy between mathematics and economics is crucial. Just like the article titled Mathematik in der Wirtschaft: Ein Schlüssel zum Erfolg emphasizes, mathematical principles play an essential role in economic problem solving. However, the efficiency of algorithms is equally important, especially when dealing with large datasets or complex calculations. In this blog post, we will explore how to optimize Java algorithms specifically for economic problems.
Why Optimize Algorithms?
Optimizing algorithms can drastically improve performance, which, in turn, leads to faster computations and better resource management. Here’s why it’s crucial:
- Efficiency: An optimized algorithm uses fewer computational resources.
- Scalability: As data grows, an optimized algorithm performs consistently without degradation in speed or efficiency.
- Cost-Effective: Reducing computation time can also lower operating costs, especially in enterprise applications.
Understanding Algorithm Complexity
Before diving into optimization techniques, it’s essential to grasp the concept of algorithm complexity. Generally, complexity is expressed in Big O notation, allowing developers to categorize algorithms based on performance.
- O(1): Constant time
- O(log n): Logarithmic time
- O(n): Linear time
- O(n log n): Linearithmic time
- O(n²): Quadratic time
Striving for lower complexity helps in creating responsive and efficient applications.
Case Study: Predicting Demand for Economic Goods
Consider a situation where we need to predict the demand for economic goods based on historical data. This model can be represented through regression analysis, which can be computationally heavy. Here, we will focus on the implementation aspect using Java.
Step 1: Use Efficient Data Structures
To handle large datasets, it's crucial to choose the right data structures. For instance, instead of using ArrayList
, consider using HashMap
or HashSet
for constant time complexity on lookups.
import java.util.HashMap;
import java.util.Map;
public class DemandPredictor {
private Map<String, Integer> historicalData = new HashMap<>();
public void addData(String product, int demand) {
historicalData.put(product, demand);
}
public Integer getDemand(String product) {
return historicalData.getOrDefault(product, 0);
}
}
Commentary
In the code snippet above, we opted for a HashMap
to ensure efficient data retrieval. This is essential in economic forecasting models where you may have thousands of products and their corresponding demands.
Step 2: Implement Caching
Caching can drastically reduce the amount of computation needed for repeated queries. By storing results from expensive function calls, you avoid redundant operations:
import java.util.HashMap;
public class DemandPredictor {
private HashMap<String, Integer> demandCache = new HashMap<>();
public int predictDemand(String product) {
if (demandCache.containsKey(product)) {
return demandCache.get(product);
}
// Simulating a complex computation
int demand = complexDemandCalculation(product);
demandCache.put(product, demand);
return demand;
}
private int complexDemandCalculation(String product) {
// Simulated complex logic (e.g., machine learning model)
return product.length() * 100; // Mock logic for demonstration
}
}
Commentary
Here, we use a caching mechanism that prevents recalculating demand for products previously analyzed. This optimization is particularly useful in cases where the same queries are made repeatedly, thus saving computation time.
Step 3: Parallel Processing
Java provides robust support for concurrent programming, enabling you to utilize multiple threads for intensive tasks such as running simulations or data analysis.
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ParallelDemandAnalysis {
private ExecutorService executor = Executors.newFixedThreadPool(4); // four parallel threads
public void analyzeDemand(String[] products) {
for (String product : products) {
executor.submit(() -> {
int demand = complexDemandCalculation(product);
System.out.println("Demand for " + product + ": " + demand);
});
}
executor.shutdown();
}
}
Commentary
In this snippet, we create an ExecutorService
for efficient handling of parallel tasks. By breaking down the calculations into smaller bits, we optimize the computing time, an approach often utilized in high-frequency trading systems where speed is critical.
Step 4: Use Libraries for Complex Algorithms
Sometimes, the best optimization isn’t in the code you write but in leveraging existing libraries. Tools like Apache Commons Math or JFreeChart can efficiently handle linear regression and statistical computations, which is often complex to implement.
import org.apache.commons.math3.stat.regression.SimpleRegression;
public class SimpleDemandModel {
public void runRegression(double[][] data) {
SimpleRegression regression = new SimpleRegression();
for (double[] datapoint : data) {
regression.addData(datapoint[0], datapoint[1]); // x is time, y is demand
}
double predictedDemand = regression.predict(5); // Predicting for x = 5
System.out.println("Predicted Demand: " + predictedDemand);
}
}
Commentary
Utilizing specialized libraries saves time on implementation and reduces the chance of bugs. Moreover, these libraries have already undergone optimization for performance, thus, offering better scalability.
In Conclusion, Here is What Matters
In this blog post, we explored various strategies for optimizing Java algorithms tailored for economic problem-solving. By selecting efficient data structures, employing caching, leveraging parallel processing, and utilizing existing libraries, developers can significantly improve their applications’ performance.
As highlighted in the article Mathematik in der Wirtschaft: Ein Schlüssel zum Erfolg, the marriage between mathematical concepts and algorithm optimization is fundamental. Embrace these techniques to excel in economic modeling and make informed decisions backed by data.
Successful economic problem solving is a blend of rigorous mathematical analysis and optimized computational techniques. Focusing on efficiency and scaling your algorithms will not merely solve problems but empower businesses to make strategic decisions faster and more effectively.