Java Integration: Simplifying CSS & JS Variable Management
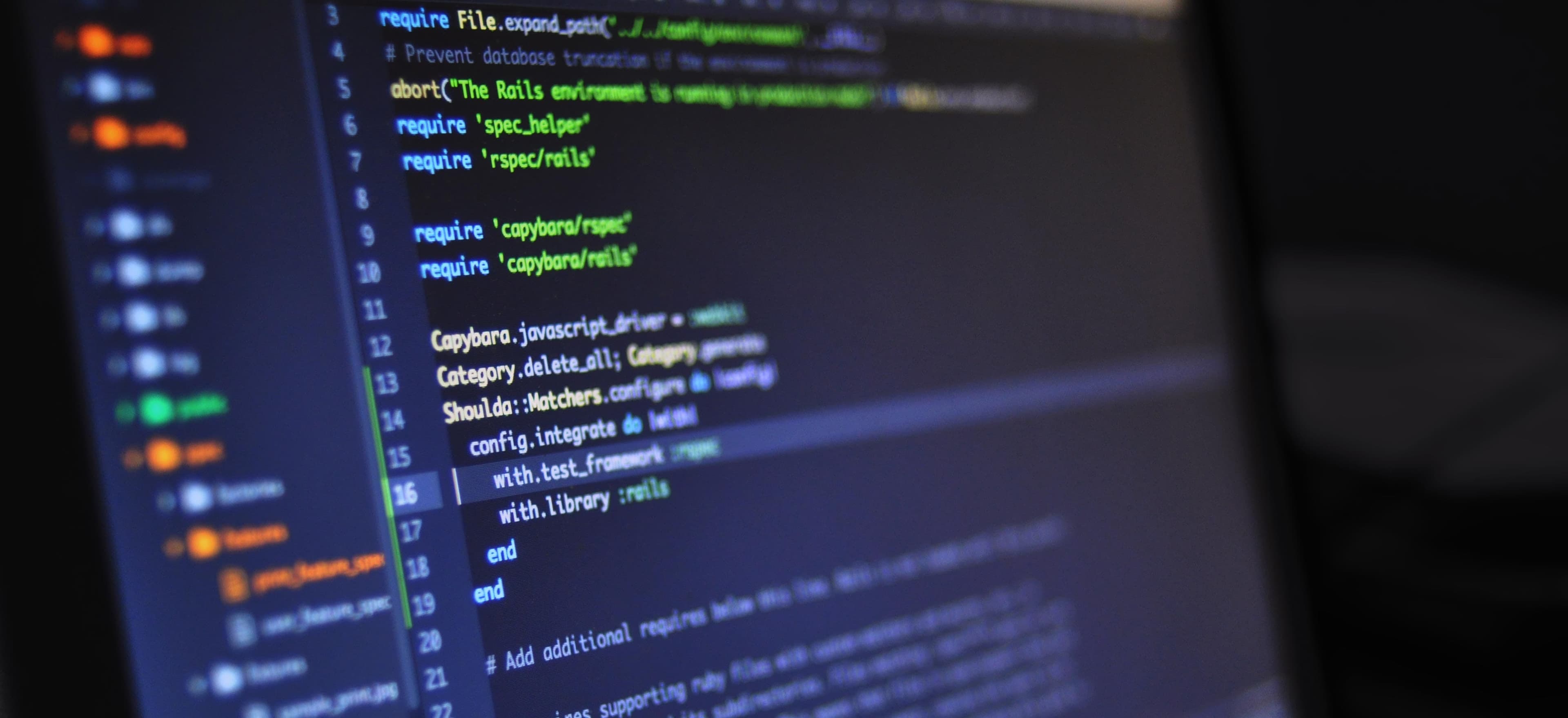
- Published on
Java Integration: Simplifying CSS & JS Variable Management
In modern web development, managing CSS and JavaScript variables can often become a daunting task. With a variety of frameworks and tools at our disposal, developers are constantly seeking ways to streamline their workflow. In this blog post, we’ll explore how Java can be effectively integrated into your development workflow, focusing specifically on CSS and JS variable management. This approach not only enhances productivity but also promotes maintainable and scalable code.
Understanding CSS and JS Variables
Before diving into Java integration, let’s briefly discuss what CSS and JavaScript variables are.
CSS Variables
CSS variables, also known as custom properties, allow you to store values that you can reuse throughout your stylesheet. They make it easier to maintain and manage your styles, as any changes to the variable will automatically reflect across all the stylesheets where they're used.
Example:
:root {
--main-color: #3498db;
}
.button {
background-color: var(--main-color);
}
JavaScript Variables
JavaScript variables hold data that can be manipulated programmatically. By using JavaScript to manage your CSS variables, you can create dynamic styles based on user interactions or specific conditions.
Example:
document.documentElement.style.setProperty('--main-color', '#e74c3c');
Now that we have a basic understanding of CSS and JS variables, let’s explore how Java can help manage these variables more efficiently.
The Role of Java in Web Development
Java has been a cornerstone of enterprise-level applications for decades. It is known for its robustness, scalability, and extensive libraries. While Java is not traditionally associated with frontend development, it can be used server-side to generate dynamic CSS and JS variables. This method can reduce the amount of repetitive code and make variable management simpler.
Why Use Java for Variable Management?
-
Maintainability: Centralizing your variable definitions in Java allows for better maintainability. It ensures that any changes to variables are consistently applied across your application.
-
Dynamic Content: Dynamically generating CSS and JS variables based on server-side logic can help create tailored experiences for users.
-
Integration with Frameworks: Java frameworks like Spring allow seamless integration with front-end technologies. This paves the way for a smooth workflow across various components of your application.
Implementing Java for CSS & JS Management
1. Setup Your Java Environment
To get started, ensure that you have the necessary environment set up for your Java backend. A simple Spring Boot application can serve as a robust foundation.
Project Structure
my-web-app/
├── src/
│ ├── main/
│ │ ├── java/
│ │ ├── resources/
│ │ └── templates/
│ └── test/
└── pom.xml
Gradle Dependencies
Ensure that you include the necessary dependencies in your pom.xml
if you're using Maven:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
</dependencies>
2. Creating Your CSS/JS Variable Management Logic
Here, we'll create a simple service that generates a JSON object containing CSS and JS variables.
import org.springframework.stereotype.Service;
import java.util.HashMap;
import java.util.Map;
@Service
public class VariableService {
public Map<String, String> getVariables() {
Map<String, String> variables = new HashMap<>();
variables.put("mainColor", "#3498db");
variables.put("secondaryColor", "#2ecc71");
return variables;
}
}
In this code, we declare a service class that holds our variables in a HashMap. The getVariables
method will be called whenever we need to access these variables on the frontend.
3. Exposing Variables Through a Controller
Next, create a controller to expose these variables via an API endpoint.
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.Map;
@RestController
public class VariableController {
@Autowired
private VariableService variableService;
@GetMapping("/api/variables")
public Map<String, String> getVariables() {
return variableService.getVariables();
}
}
This simple controller exposes an API that can be accessed from the frontend to retrieve our CSS and JS variable values.
4. Utilizing the Variables in the Frontend
To apply these variables in your JavaScript and CSS, you can use the fetch
API to retrieve the data and then dynamically update styles or use them directly in JavaScript.
fetch('/api/variables')
.then(response => response.json())
.then(variables => {
Object.keys(variables).forEach(key => {
document.documentElement.style.setProperty(`--${key}`, variables[key]);
});
});
This script will fetch the variables from our API endpoint and dynamically set them as CSS custom properties. As a result, any CSS that utilizes those variables will immediately reflect the changes.
5. Example: Using Variables in CSS
Now that we have the variables set up, we can style our elements dynamically based on the Java provided variables.
Example CSS:
.button {
background-color: var(--mainColor);
color: white;
}
This button will now adapt its background color based on the value we fetched from the Java backend.
The Bottom Line
Integrating Java for CSS and JS variable management not only simplifies your web development process but also enhances control over your styles and JavaScript behaviors. With a few lines of code, you can create a centralized and maintainable approach to manage your application's design aspects dynamically.
For further insight on streamlining web development practices, I recommend checking out the article titled "Streamlining Web Dev: How to Effortlessly Generate CSS & JS Variables" at infinitejs.com/posts/streamlining-web-dev-generate-css-js-variables. It elaborates on various methods to enhance development efficiency, providing a broader perspective to complement the Java integration technique discussed here.
Incorporating these strategies into your development stack can lead to a more cohesive and robust application, greatly benefiting both developers and end-users alike. Happy coding!
Checkout our other articles