Tackling Time Zone Challenges in Java's LocalDateTime
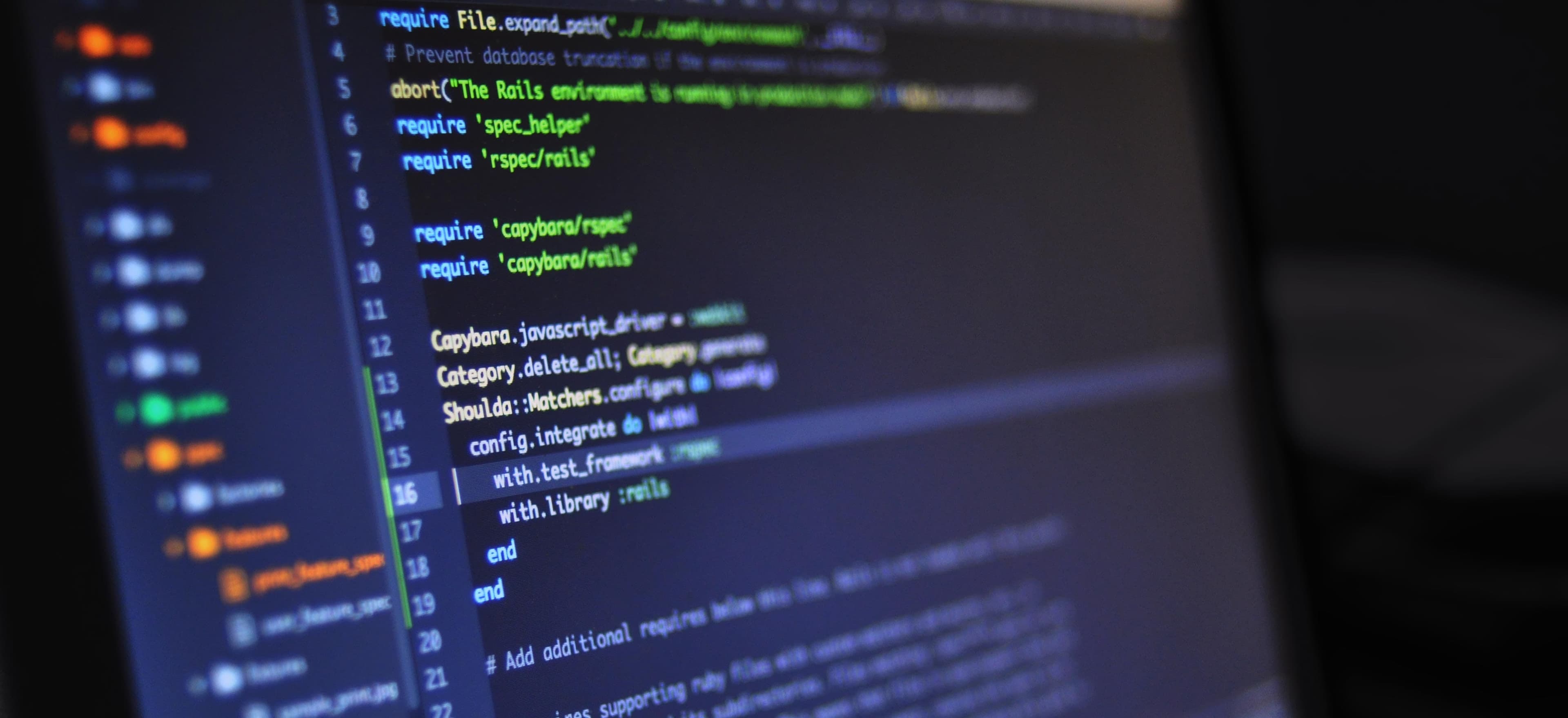
- Published on
Tackling Time Zone Challenges in Java's LocalDateTime
Handling dates and times can be a tricky business in any programming language. When time zones come into play, the complexity can increase exponentially. In Java, while the LocalDateTime
class provides a solid framework for managing dates and times, it does not account for time zones inherently. This can lead to potential pitfalls if you're not aware of how to navigate these challenges.
In this post, we will delve into the time zone challenges you might face when using LocalDateTime
in Java, the techniques to tackle these challenges, and finally, best practices for date-time handling. We will also reference the existing article "Mastering Time Zone Issues in JavaScript Date Handling", which offers valuable insights into how similar issues are addressed in JavaScript.
Understanding LocalDateTime
Before exploring how to manage time zones with LocalDateTime
, let’s clarify what it is. Introduced in Java 8 within the java.time
package, LocalDateTime
represents a date-time without a time zone in the ISO-8601 calendar system. This means that while it carries detailed information about the date and time, it ignores the time zone.
import java.time.LocalDateTime;
public class LocalDateTimeExample {
public static void main(String[] args) {
// Getting the current LocalDateTime
LocalDateTime dateTime = LocalDateTime.now();
System.out.println("Current DateTime: " + dateTime);
}
}
Why Use LocalDateTime?
- Clarity: By not involving a time zone,
LocalDateTime
is straightforward when only local date-time information is needed. - Precision: It offers nanosecond precision, which is useful in applications requiring fine-grained date-time accuracy.
The Time Zone Dilemma
The limitation of LocalDateTime
becomes evident when you're working across multiple time zones. For instance, storing a date-time value from New York and understanding how it relates to Tokyo can lead to misconceptions if time zones are not accounted for.
Converting LocalDateTime to ZonedDateTime
To effectively manage time zone issues, it's often beneficial to convert LocalDateTime
to ZonedDateTime
. This class includes all the components of a date-time with a specified time zone.
Here is a basic example of this conversion:
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.time.ZonedDateTime;
public class DateTimeConversion {
public static void main(String[] args) {
LocalDateTime localDateTime = LocalDateTime.now();
// Define a ZoneId for New York
ZoneId zoneIdNY = ZoneId.of("America/New_York");
// Convert LocalDateTime to ZonedDateTime
ZonedDateTime zonedDateTimeNY = localDateTime.atZone(zoneIdNY);
System.out.println("Zoned DateTime in New York: " + zonedDateTimeNY);
}
}
Commentary: Why Convert?
Without converting to ZonedDateTime
, all operations that depend on the correct interpretation of time in different regions would yield incorrect results. By attaching a time zone to the date-time value, you ensure accurate conversions and calculations suited for different locales.
Handling Time Zone Offsets
Another important consideration when managing time in Java involves time zone offsets. For example, during Daylight Saving Time (DST), the offset for certain regions might change, affecting how you interpret the stored LocalDateTime
.
Let’s see how we can factor this in:
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.time.ZonedDateTime;
public class TimeZoneOffsetExample {
public static void main(String[] args) {
LocalDateTime localDateTime = LocalDateTime.of(2023, 6, 15, 12, 0); // noon on June 15
ZoneId zoneIdNY = ZoneId.of("America/New_York");
ZonedDateTime zonedDateTimeNY = localDateTime.atZone(zoneIdNY);
// Display the offset
System.out.println("Zoned DateTime with Offset: " + zonedDateTimeNY + " UTC offset is: " + zonedDateTimeNY.getOffset());
}
}
Commentary: Why Important?
By checking the UTC offset, we can accurately determine how different regions might interpret the same LocalDateTime
. This insight is crucial, especially when you deal with global applications, scheduling systems, or log data.
Best Practices for Date-Time Handling
-
Always Specify Time Zones: If there’s a time zone involved, always work with
ZonedDateTime
rather thanLocalDateTime
. -
Use System Zone: When applicable, use the system default time zone with
ZoneId.systemDefault()
. -
Be Mindful of DST: Account for Daylight Saving Time changes when performing calculations across time zones.
-
Validation: Whenever inputting user dates, validate with respect to the intended time zone to avoid nondescript time anomalies.
Example to Illustrate Best Practices
The following code illustrates best practices for working with date-times across various zones:
import java.time.LocalDateTime;
import java.time.ZoneId;
import java.time.ZonedDateTime;
public class BestPracticesExample {
public static void main(String[] args) {
LocalDateTime localDateTime = LocalDateTime.now();
// Using system default zone
ZoneId systemZoneId = ZoneId.systemDefault();
ZonedDateTime zonedDateTime = localDateTime.atZone(systemZoneId);
// Displaying the results
System.out.println("Local DateTime: " + localDateTime);
System.out.println("Zoned DateTime: " + zonedDateTime);
// Demonstrating offset awareness
System.out.println("Current UTC offset: " + zonedDateTime.getOffset());
}
}
A Final Look
Dealing with time zones in Java can initially appear daunting, but with a solid understanding of LocalDateTime
and the associated classes like ZonedDateTime
, time zone challenges can be effectively managed. We explored converting between time types, acknowledged the significance of time zone offsets, and outlined best practices for effective handling.
As noted earlier, similar concepts are discussed concerning JavaScript in the article "Mastering Time Zone Issues in JavaScript Date Handling". It is beneficial to compare solutions across languages, as many underlying principles remain pertinent in various contexts.
By following these guidelines, you can sidestep many common pitfalls associated with date and time handling in Java, ensuring your applications are both accurate and reliable. Happy coding!
Checkout our other articles