Streamlining Java Code: Mastering File Change Validation
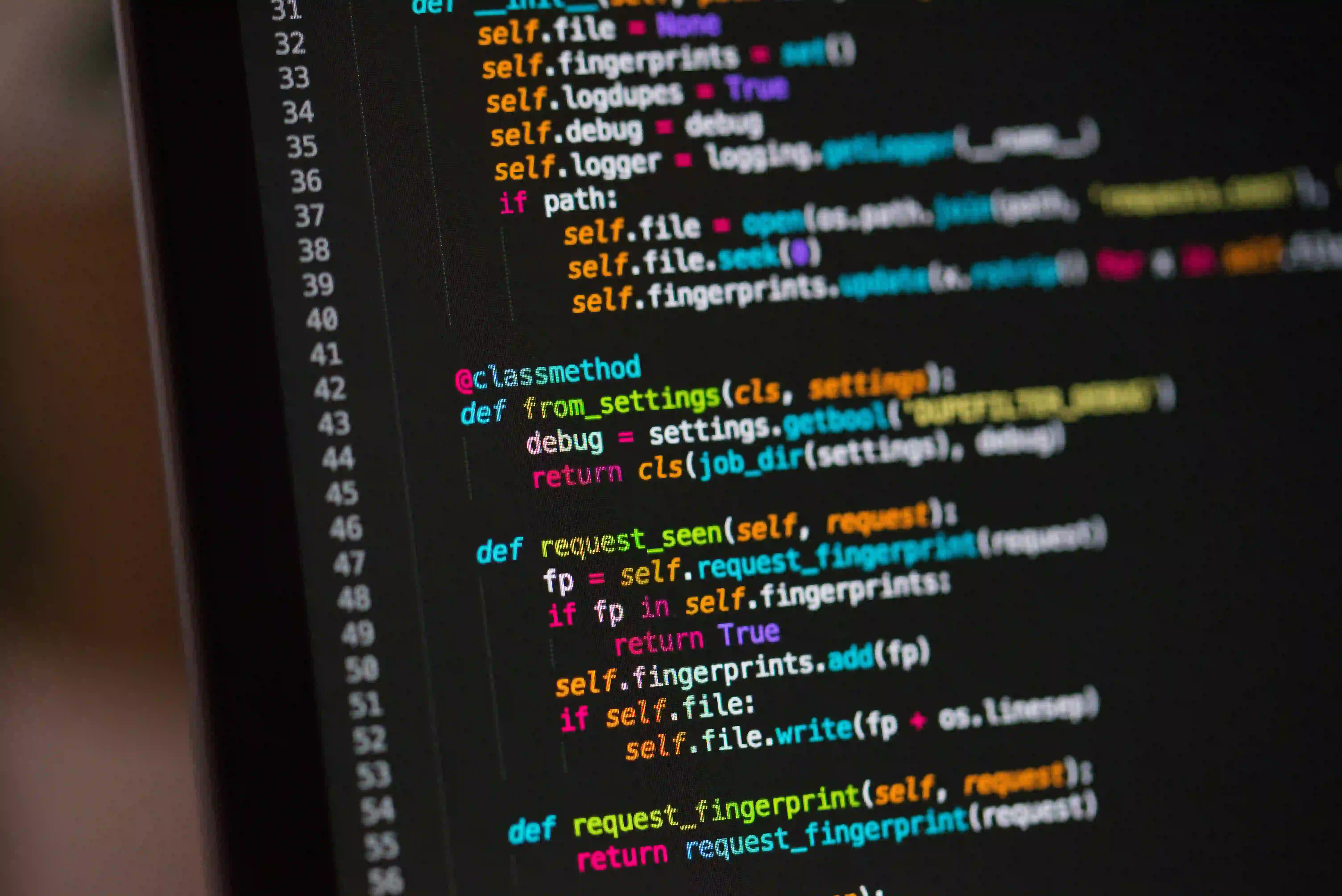
Streamlining Java Code: Mastering File Change Validation
When it comes to maintaining a robust codebase, validating changes in your files can be one of the most critical tasks in software development. This not only helps maintain code integrity but also minimizes bugs and redundancies. In this article, we will explore how to effectively validate changed files in your Java codebase, streamline your development process, and ensure that your code remains efficient and easy to manage.
Before diving into specifics, you may want to check out an insightful article titled How to Effectively Validate Changed Files in Your Codebase for a more foundational understanding of this topic. With that said, letβs get started!
What is File Change Validation?
File change validation refers to the mechanism through which developers ensure that any modification made to their codebase adheres to certain standards and criteria. This is critical to ensure that only valid and intended changes are integrated into the project. Validating changes helps prevent regressions, unforeseen bugs, and compatibility issues.
Why File Change Validation Matters
-
Maintains Code Quality: Regular validation keeps your code clean and adheres to best practices, which is vital for collaboration in team-based environments.
-
Reduces Bugs: By catching issues early, developers reduce the cost and complexity of fixes later in development.
-
Improves Team Collaboration: When validation is done consistently, team members can trust that the code they are working with is in a good state.
-
Enhanced Deployment Safety: Ensuring that only validated code gets deployed mitigates risk in production.
Setting Up File Change Validation in Java
Using Git Hooks for File Validation
One robust solution for file validation is utilizing Git hooks. Git hooks are scripts that Git executes before or after events such as commits or pushes. By setting up a pre-commit hook, you can automate validation.
-
Navigate to Your Git Hooks Directory:
In your project, navigate to the
.git/hooks
directory. Here, you can create scripts that Git will execute. -
Create a Pre-Commit Hook:
Create a file named
pre-commit
in the hooks directory. The script will run every time a commit is made.
#!/bin/sh
# This script checks for the presence of Java syntax errors before a commit.
# A simple way to validate Java files before committing
if ! mvn clean compile; then
echo "Error: Java validation failed!"
exit 1
fi
echo "Java files validated successfully."
The Importance of the Code Snippet Above
-
Why Use Maven?: The command
mvn clean compile
does a complete build of your project. If there are any syntax errors or issues, the commit will be prevented. This ensures that only code that can compile properly makes it into the repository. -
Exit Codes: The exit code (
exit 1
) ensures that if validation fails, the commit halts, preserving the integrity of your codebase.
Implementing Custom Validation Logic
Sometimes, you may want to incorporate more specific validation logic. Below is an example of validating file changes against a set of rules.
import java.io.File;
import java.io.IOException;
// Custom class to validate Java files
public class FileValidator {
// Method to check if a file is valid
public boolean isValid(File file) throws IOException {
// Define validations; for instance, checking file extension
if (!file.getName().endsWith(".java")) {
throw new IllegalArgumentException("File must be a Java source file.");
}
// Add additional checks (e.g., checking file contents)
// ...
return true;
}
// Main method to execute validation
public static void main(String[] args) {
try {
File file = new File("path/to/your/File.java");
FileValidator validator = new FileValidator();
if (validator.isValid(file)) {
System.out.println("File validation successful.");
}
} catch (IllegalArgumentException | IOException e) {
System.err.println("Validation failed: " + e.getMessage());
}
}
}
Explaining the Code
-
Custom Validations: The
isValid
method checks if the file ends with.java
. This is a basic but necessary check to ensure only Java files are processed. -
Handling Errors: Using exceptions to handle invalid cases helps in identifying issues early.
-
Ease of Use: By wrapping validation logic in a dedicated class, we follow the principles of Object-Oriented Design, making the code reusable and testable.
Advanced Validation with Libraries
Java boasts a rich ecosystem of libraries that can be utilized for more complex validations. One popular library to consider is Apache Commons Validator.
Example of Using Apache Commons Validator
To demonstrate, let's validate a property within a Java class using Commons Validator.
-
Set Up the Dependency:
If you are using Maven, add the following to your
pom.xml
.
<dependency>
<groupId>commons-validator</groupId>
<artifactId>commons-validator</artifactId>
<version>1.6</version>
</dependency>
- Implement Validation Logic:
import org.apache.commons.validator.routines.EmailValidator;
public class UserValidator {
// Method to validate email format
public boolean validateEmail(String email) {
EmailValidator validator = EmailValidator.getInstance();
return validator.isValid(email);
}
public static void main(String[] args) {
UserValidator userValidator = new UserValidator();
String emailToTest = "test@example.com";
if (userValidator.validateEmail(emailToTest)) {
System.out.println("Valid email address.");
} else {
System.out.println("Invalid email address.");
}
}
}
Why Use Third-Party Libraries?
-
Efficiency: Built-in validation methods save time and reduce bugs.
-
Community Support: Popular libraries come with community backing and documentation, ensuring reliability and ongoing updates.
The Last Word
Proper file change validation is an integral part of maintaining the health of a Java codebase. With tools like Git hooks, custom validation classes, and libraries like Apache Commons Validator, you can implement an effective strategy for this purpose. By automating and streamlining the validation process, you enable a focus on writing clean, efficient code, ultimately enhancing the quality of your software.
For those interested in further reading, don't forget to check out How to Effectively Validate Changed Files in Your Codebase. The journey towards maintaining a flawless codebase begins with understanding and effectively implementing changes. Happy coding!