Mastering High Cardinality in Java with Time Series Data
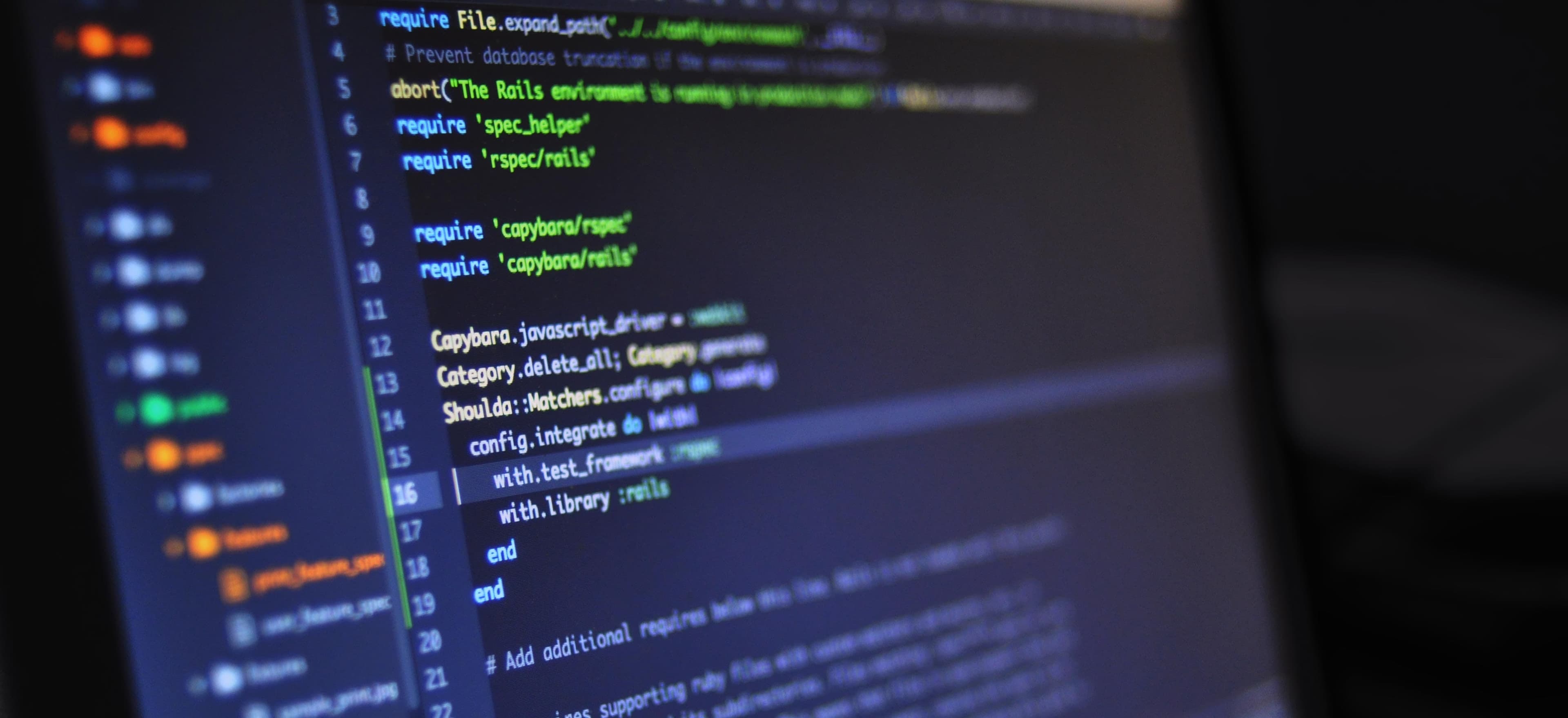
- Published on
Mastering High Cardinality in Java with Time Series Data
High cardinality data refers to datasets with many unique values across a relatively small number of records. This often appears in time-series data where timestamps and unique identifiers lead to complex data structures that need efficient handling. As Java developers, understanding how to manage high cardinality in time-series databases can significantly impact performance, scalability, and overall system response.
In this post, we will explore high cardinality data handling in Java, focusing on best practices and strategies. We will also reference the article titled "Comparison: Handling High Cardinality Data in Time Series Databases" (available at https://configzen.com/blog/handling-high-cardinality-data-time-series-databases) to give you further insights into this intricate topic.
Understanding High Cardinality Data
Before diving into practical implementations, it is crucial to grasp the concept of high cardinality.
What is Cardinality?
Cardinality refers to the uniqueness of data values contained within a particular column of a database table. High cardinality means that the column has a vast number of unique values, resulting in potentially heavy loads on data processing and storage.
Consider a dataset of user activities stored in a time series database:
- User IDs
- Timestamps
- Activities (e.g., login, action completion)
Each user has unique interactions, leading to high cardinality in user IDs when many users interact over time.
Why is High Cardinality Difficult to Manage?
High cardinality can lead to several challenges:
- Storage Overhead: Large numbers of unique values require extensive storage.
- Query Complexity: High cardinality can slow down queries as databases struggle to index unique values efficiently.
- Data Analysis: Analyzing and visualizing high cardinality data can become cumbersome.
To tackle these issues, we explore methodologies and techniques that can help manage high cardinality data more effectively in Java applications.
Best Practices for Handling High Cardinality Data
1. Data Aggregation
Aggregating data can significantly reduce cardinality. Instead of querying every unique data point, consider summarizing your data.
Example: Aggregating Input Data
Suppose you have a dataset of user logins over time. You can aggregate the user activity by hour or day.
import java.time.LocalDateTime;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ActivityAggregator {
public Map<LocalDateTime, Integer> aggregateByHour(List<LocalDateTime> timestamps) {
Map<LocalDateTime, Integer> hourlyActivityCount = new HashMap<>();
for (LocalDateTime timestamp : timestamps) {
LocalDateTime hour = timestamp.withMinute(0).withSecond(0).withNano(0);
hourlyActivityCount.put(hour, hourlyActivityCount.getOrDefault(hour, 0) + 1);
}
return hourlyActivityCount;
}
}
Why Aggregate Data?
Aggregating data reduces the number of unique entries, allowing for simpler and faster analysis. Instead of querying many unique timestamp values, this approach summarizes activity by hour, leading to less strain on your database.
2. Utilize Efficient Data Structures
Java collections can dramatically improve performance when dealing with high cardinality data.
Example: Using HashMaps for Quick Lookups
import java.util.HashMap;
import java.util.Map;
public class UniqueUserTracker {
private Map<String, Integer> userActivityMap = new HashMap<>();
public void recordActivity(String userId) {
userActivityMap.put(userId, userActivityMap.getOrDefault(userId, 0) + 1);
}
public int getActivityCount(String userId) {
return userActivityMap.getOrDefault(userId, 0);
}
}
Why Use HashMaps?
A HashMap offers average O(1) time complexity for insertions and lookups. This efficiency is vital when managing high cardinality data, allowing for quick retrieval and updates of user activity counts.
3. Leverage Time Series Database Features
Many modern databases offer features tailored for time series data that you should utilize.
- Ingestion Optimizations: Use bulk loading of data when possible to improve ingestion efficiency.
- Retention Policies: Implement policies to manage data lifespan, ensuring that only relevant data remains.
Refer to the article "Comparison: Handling High Cardinality Data in Time Series Databases" (https://configzen.com/blog/handling-high-cardinality-data-time-series-databases) for an in-depth comparison of time series databases and their features.
4. Implement Compression Techniques
Data compression can effectively minimize the amount of storage high cardinality data requires. Many time series databases come with built-in compression algorithms.
Example: Using Apache Parquet
Apache Parquet is a columnar storage file format that supports efficient compression.
import org.apache.parquet.example.data.GroupWriter;
import org.apache.parquet.hadoop.example.ExampleInputFormat;
// Use a parquet writer or input format to store data efficiently.
Why Compress Data?
Compression reduces the storage footprint and can speed up data reads due to decreased I/O operations.
5. Use Sampling Techniques
When dealing with massive datasets, it may not be necessary to analyze every unique data point. Sampling can provide insights without overloading your system.
Example: Random Sampling from a Data Stream
import java.util.Random;
public class DataSampler {
private Random random = new Random();
public <T> T sample(T[] data) {
return data[random.nextInt(data.length)];
}
}
Why Implement Sampling?
Sampling can help produce insights quickly while preventing degradation of system performance during data analysis.
In Conclusion, Here is What Matters
Mastering high cardinality data in Java requires understanding and leveraging various techniques and best practices. Data aggregation, effective data structures, utilizing time series database features, compression, and sampling are all critical strategies developers can implement.
Adopting these methods allows the developer to manage and analyze high cardinality data efficiently, paving the way for scalable and responsive applications.
For more in-depth discussions on handling high cardinality data in time-series databases, be sure to explore the article "Comparison: Handling High Cardinality Data in Time Series Databases" available at https://configzen.com/blog/handling-high-cardinality-data-time-series-databases.
By embracing these strategies, you can tackle high cardinality data head-on, transforming potential challenges into opportunities for optimization and efficiency. Happy coding!