Java Solutions for High Cardinality in Time Series Data
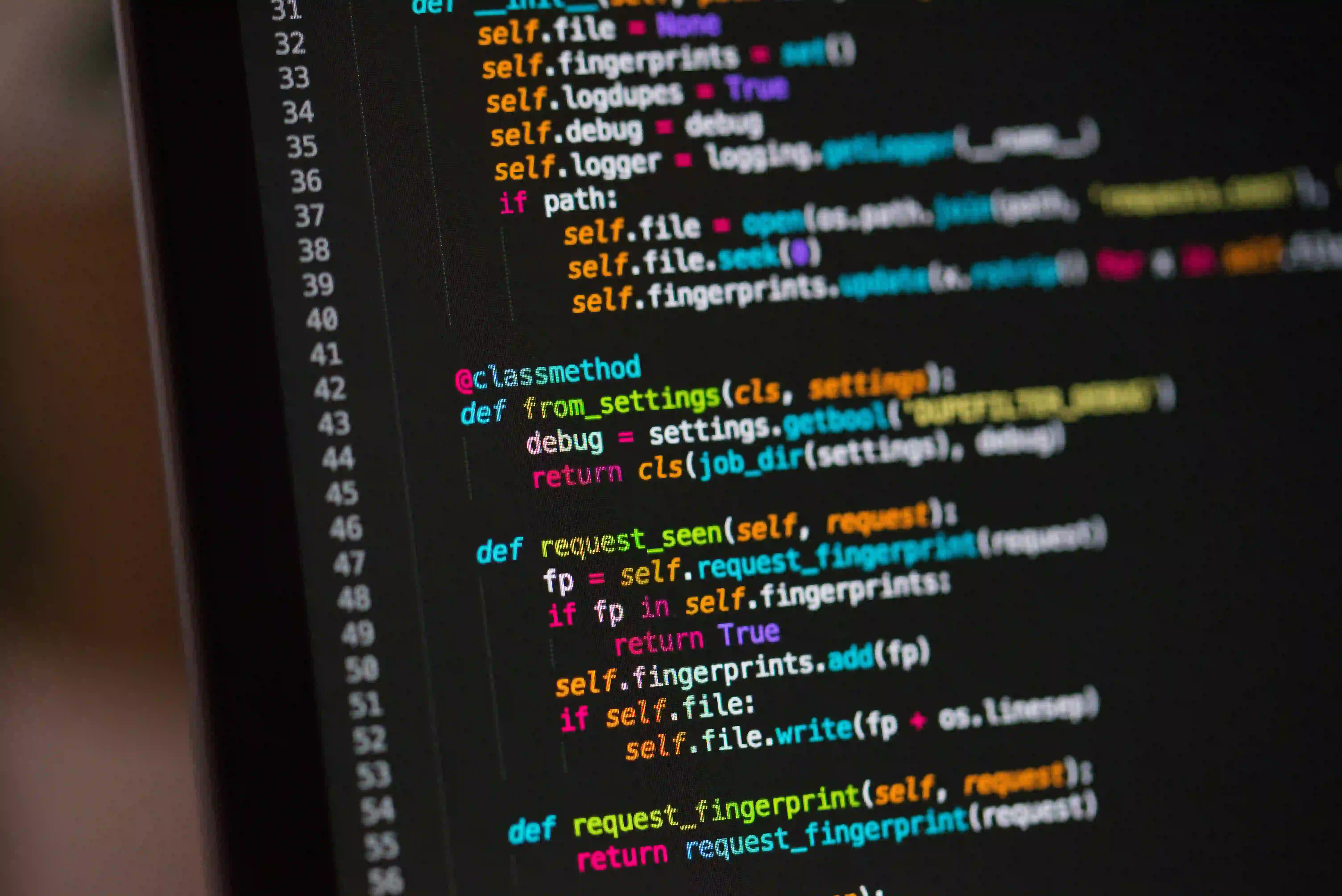
Java Solutions for High Cardinality in Time Series Data
In the realm of data analysis, especially within time series databases, managing high cardinality data can present significant challenges. Cardinality refers to the uniqueness of data values contained in a particular dataset. High cardinality means that the dataset includes a vast number of unique values, posing difficulties in storage, performance, and analysis. Understanding how Java can be utilized to tackle these issues effectively is essential for developers and data engineers alike.
This article will explore various Java solutions for dealing with high cardinality time series data, alongside best practices, exemplary code snippets, and reference material for deeper insights into the subject. If you're interested in detailed comparisons of methods for handling high cardinality data, I recommend checking out Comparison: Handling High Cardinality Data in Time Series Databases.
Understanding High Cardinality Data
Before diving into solutions, it's crucial to comprehend the implications of high cardinality in time series data. High cardinality can lead to:
- Storage Overhead: Storing unique time series metrics for numerous entities can require large amounts of disk space.
- Query Performance: Operations on high cardinality data can slow down your queries considerably, leading to potential performance bottlenecks.
- Increased Complexity: A larger number of unique values increases the complexity when aggregating and analyzing data.
Java and Time Series Databases
Java is a widely-used programming language favored for its robustness and scalability. With its support for concurrency, ease of handling data, and extensive libraries, Java can be an excellent choice for managing time series data, especially in high cardinality scenarios.
1. Choosing the Right Time Series Database
When dealing with high cardinality, the choice of time series database becomes critical. Options such as InfluxDB, TimescaleDB, and Apache Druid offer built-in features for better performance with high cardinality data. Java connectors for these databases simplify interaction.
Example: Connecting to InfluxDB
import org.influxdb.InfluxDB;
import org.influxdb.InfluxDBFactory;
import org.influxdb.dto.Point;
import java.util.concurrent.TimeUnit;
public class InfluxDBExample {
public static void main(String[] args) {
InfluxDB influxDB = InfluxDBFactory.connect("http://localhost:8086", "username", "password");
influxDB.setDatabase("your_database");
// Writing high cardinality data
Point point = Point.measurement("sensor_data")
.time(System.currentTimeMillis(), TimeUnit.MILLISECONDS)
.addField("temperature", 20.5)
.addField("humidity", 60)
.addField("location", "office")
.build();
influxDB.write(point);
influxDB.close();
}
}
In this example, we establish a connection to InfluxDB and write a data point that includes multiple fields. The location
field showcases how we can handle high cardinality by adding unique identifiers to each measurement.
2. Data Compression Techniques
When working with high cardinality data, using compression techniques can significantly alleviate storage problems. Apache Parquet is a columnar storage file format that helps handle high cardinality efficiently by using various compression methods. Java libraries such as Apache Arrow provide integration for working with Parquet files.
Example: Writing Parquet Files
import org.apache.parquet.example.data.GroupFactory;
import org.apache.parquet.hadoop.ParquetWriter;
import org.apache.parquet.hadoop.example.ExampleInputFormat;
import org.apache.parquet.schema.MessageType;
import java.io.IOException;
public class ParquetExample {
public static void main(String[] args) throws IOException {
ParquetWriter<Group> writer = ExampleInputFormat.getParquetWriter(new Path("data.parquet"));
// Write unique records as Group
Group group = new GroupFactory.buildWithBuilder();
group.append("user_id", 12345);
group.append("event_type", "click");
writer.write(group);
writer.close();
}
}
This code snippet demonstrates how to write high cardinality data to a Parquet file using Java. The Parquet format optimally compresses data, meaning that even with numerous unique values, storage remains efficient.
3. Aggregation and Downsampling
Another effective strategy for managing high cardinality data is to aggregate or downsample your data periodically. By creating summarizations, you reduce the number of unique entries you need to work with and improve the performance of your queries.
Example: Downsampling with Java
import java.util.HashMap;
import java.util.Map;
public class Downsampling {
public static void main(String[] args) {
Map<String, Double> timeSeriesData = new HashMap<>();
// Original time series data
timeSeriesData.put("1:00", 20.5);
timeSeriesData.put("1:01", 21.0);
timeSeriesData.put("1:02", 22.0);
// Downsample by taking the average of every two readings
Map<String, Double> downsampledData = new HashMap<>();
String newKey = "";
double avg = 0;
int count = 0;
for (Map.Entry<String, Double> entry : timeSeriesData.entrySet()) {
avg += entry.getValue();
count++;
// Every two entries downsample
if (count == 2) {
newKey = entry.getKey(); // You can modify to aggregate according to time intervals
downsampledData.put(newKey, avg / 2);
avg = 0;
count = 0;
}
}
System.out.println("Downsampled Data: " + downsampledData);
}
}
In this snippet, we simulate downsampling by averaging time series data points. This reduces the volume of unique data points you are working with, thus alleviating some issues of high cardinality.
4. Utilizing Caching Strategies
To further improve performance while dealing with high cardinality data, use caching strategies to store frequently accessed data. Java's caching libraries, like Caffeine or Ehcache, can significantly reduce the time taken to retrieve high cardinality datasets.
Example: Caching with Caffeine
import com.github.benmanes.caffeine.cache.Cache;
import com.github.benmanes.caffeine.cache.Caffeine;
import java.util.concurrent.TimeUnit;
public class CachingExample {
public static void main(String[] args) {
Cache<String, String> cache = Caffeine.newBuilder()
.expireAfterWrite(10, TimeUnit.MINUTES)
.maximumSize(1000)
.build();
// Storing high cardinality data
cache.put("user-1234", "data pertaining to user 1234");
// Retrieving the data
String data = cache.getIfPresent("user-1234");
System.out.println("Cached Data: " + data);
}
}
In this example, we create a cache where each unique data point can be stored and retrieved quickly, thereby boosting application performance even when dealing with a significant amount of cardinality.
Final Thoughts
Dealing with high cardinality data in time series can indeed be challenging, but leveraging Java's capabilities and adopting appropriate strategies can ease this burden. By using the right time series database, employing data compression techniques, downsampling, and implementing caching strategies, developers can efficiently manage high cardinality time series data.
As discussed, you can read more about various methods to handle high cardinality data in the article titled Comparison: Handling High Cardinality Data in Time Series Databases. With these insights and code examples, you are now better prepared to address the challenges posed by high cardinality in your Java applications.