How Java Applications Can Optimize Resource Management
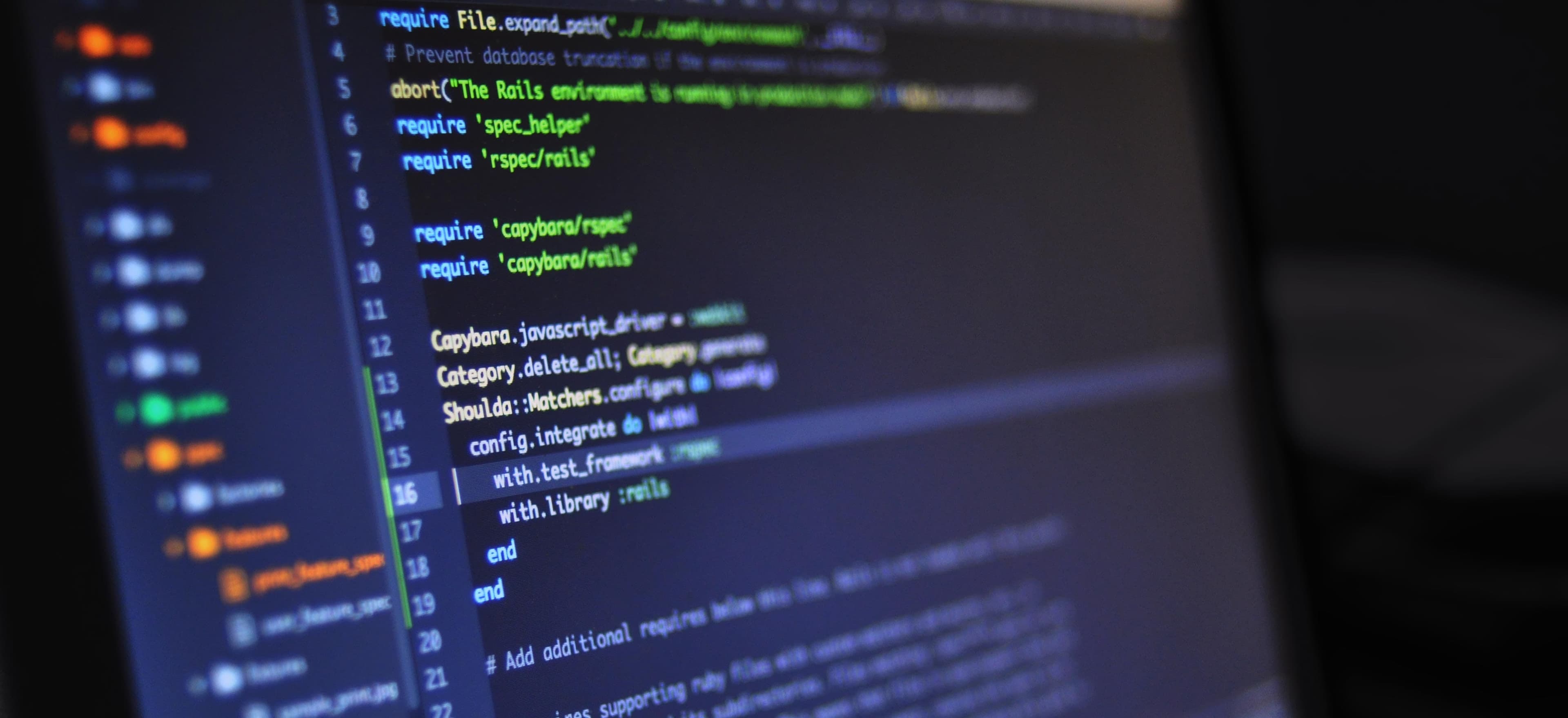
- Published on
How Java Applications Can Optimize Resource Management
In today's digital landscape, efficient resource management has become a crucial concern for both developers and organizations. By optimizing resource utilization, we can reduce costs, ensure sustainability, and improve the overall performance of applications. Among various programming languages that facilitate this, Java holds a prominent position due to its robust features, scalability, and extensive libraries. In this blog post, we will explore how Java applications can optimize resource management while keeping the discussion engaging and informative.
Understanding Resource Management in Java
Resource management refers to the methods and processes through which resources—such as memory, file handles, and database connections—are allocated, utilized, and released during the lifecycle of an application. Poor resource management can lead to memory leaks, inefficient CPU usage, and overall degraded performance. Hence, it is essential for developers to adopt practices that optimize resource utilization.
Importance of Resource Management
- Cost Efficiency: Optimized resource management can lead to reduced operational costs by minimizing resource wastage.
- Improved Performance: Efficiently managing resources can significantly enhance the performance of Java applications.
- Environmental Implications: Sustainable practices, such as effective resource utilization, contribute to environmental conservation. For example, Sustainable Firewood: Finding a Reliable Supply discusses how eco-friendly practices shape industries, and similar principles apply to resource management in tech.
Best Practices for Resource Management in Java
1. Automatic Resource Management with Try-With-Resources
Java provides a convenient feature called try-with-resources, which automatically closes resources when done, thus preventing memory leaks. It is particularly useful for managing I/O resources like file readers, database connections, and network sockets.
try (BufferedReader br = new BufferedReader(new FileReader("file.txt"))) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line); // Process the line
}
} catch (IOException e) {
e.printStackTrace();
}
Why Use Try-With-Resources?
Using this feature ensures that resources are closed promptly, without depending on explicit finally
blocks. This reduces boilerplate code and helps maintain cleaner, more readable code.
2. Connection Pooling
Establishing a database connection can be resource-intensive. Connection pooling is an efficient way to manage database connections by creating a pool of reusable connections. Java libraries such as HikariCP, Apache DBCP, and C3P0 provide robust connection pooling solutions.
Here's a basic example using HikariCP:
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydatabase");
config.setUsername("user");
config.setPassword("password");
config.addDataSourceProperty("cachePrepStmts", "true");
config.addDataSourceProperty("prepStmtCacheSize", "250");
config.addDataSourceProperty("prepStmtCacheSqlLimit", "2048");
HikariDataSource dataSource = new HikariDataSource(config);
// Usage
try (Connection connection = dataSource.getConnection()) {
// Perform database operations
} catch (SQLException e) {
e.printStackTrace();
}
Why Use Connection Pooling?
Connection pooling dramatically reduces the overhead of repeatedly establishing connections. It also ensures that connections are reused across multiple requests, thus optimizing resource utilization.
3. Efficient Data Structures
Choosing the right data structures can greatly impact memory consumption and performance. For instance, when implementing lists or maps, consider the expected size and usage patterns.
List<String> arrayList = new ArrayList<>(); // Requires more memory
List<String> linkedList = new LinkedList<>(); // Better for frequent insertions/deletions
Why Correct Data Structures Matter?
The choice of data structure can minimize memory overhead and improve execution time, leading to enhanced application performance.
4. Garbage Collection Tuning
Java's automatic garbage collection helps free memory occupied by objects that are no longer needed. However, you can further optimize garbage collection through tuning parameters in the Java Virtual Machine (JVM).
java -Xms512m -Xmx1024m -XX:+UseG1GC -jar yourapp.jar
Why Tune Garbage Collection?
Optimizing garbage collection can lead to lower latency and a smoother application experience by minimizing pauses during operation.
5. Caching Strategies
Implementing caching can significantly reduce resource usage. By storing results of expensive operations, you can save processing time and reduce database load.
Using the Guava library for an in-memory cache:
Cache<String, String> cache = CacheBuilder.newBuilder()
.maximumSize(100)
.expireAfterWrite(10, TimeUnit.MINUTES)
.build();
// Usage
cache.put("key", "value");
String value = cache.getIfPresent("key");
Why Use Caching?
Developing a caching strategy optimizes performance by reducing the load on underlying resources, such as databases or APIs.
6. Asynchronous Processing
Java provides several ways to handle asynchronous processing, such as using CompletableFuture, ExecutorService, or reactive programming frameworks (like RxJava). Asynchronous processing allows you to run tasks concurrently, freeing up resources for other operations.
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
// Perform a long-running task
return "Result";
});
// Non-blocking operation
future.thenAccept(result -> System.out.println("Result: " + result));
Why Use Asynchronous Processing?
Asynchronous programming can lead to higher throughput and less idle time, ensuring better resource allocation and faster application response times.
Closing Remarks
Java provides robust tools and frameworks to optimize resource management effectively. By applying best practices such as using try-with-resources, implementing connection pooling, selecting efficient data structures, tuning garbage collection, adopting caching strategies, and leveraging asynchronous processing, developers can build highly efficient and sustainable Java applications.
Remember that optimal resource management not only enhances application performance but also contributes to cost savings and environmental sustainability. For more insights on sustainable practices in other industries, refer to Sustainable Firewood: Finding a Reliable Supply.
By adopting these practices, you'll ensure that your Java applications are not just efficient but also environmentally responsible—just another step toward embracing sustainability in software development.