Avoiding Common Pitfalls When Integrating Java with HTML
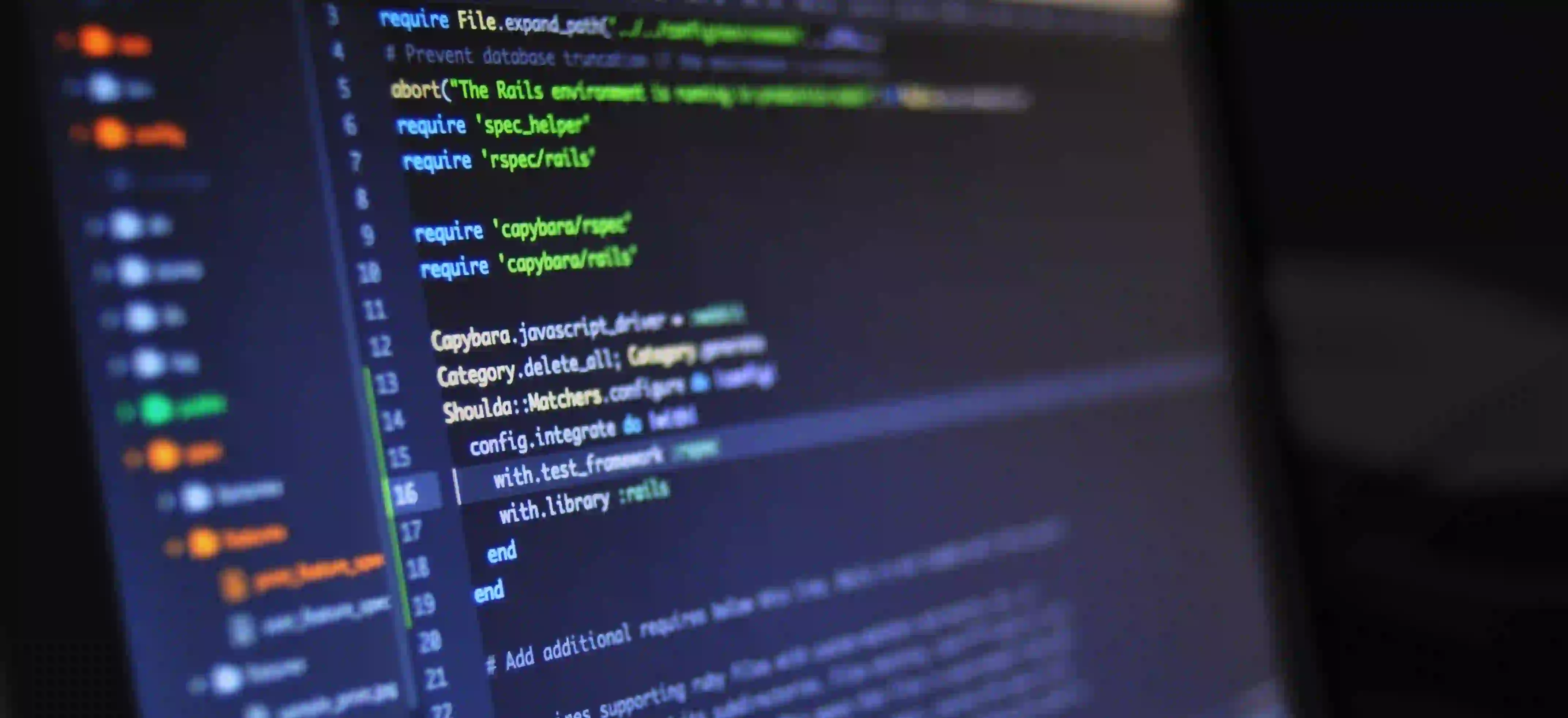
Avoiding Common Pitfalls When Integrating Java with HTML
Integrating Java with HTML can be quite challenging, especially for web developers who are used to working in a dynamic environment with multiple languages. Just as PHP and HTML can sometimes clash, as noted in the article "Blending PHP & HTML in Echo: A Common Pitfall" (https://tech-snags.com/articles/php-html-echo-pitfalls), Java also presents its own unique challenges when incorporating HTML. This blog post explores those pitfalls and how to avoid them to ensure a seamless integration that results in a smooth user experience.
Understanding the Java-HTML Relationship
Before diving into common pitfalls, it’s essential to understand how Java interacts with HTML in a web environment. Typically, Java is used on the server side with frameworks like Spring or JavaServer Pages (JSP) while HTML is served to the client in a web browser. This client-server paradigm can lead to common pitfalls, which we’ll discuss below.
1. Confusing Server-Side and Client-Side Logic
One of the most prevalent mistakes when integrating Java with HTML is the confusion between server-side logic (Java) and client-side logic (HTML/JavaScript). When writing code, it’s easy to think that Java can dynamically manipulate HTML as it would with data, but HTML is primarily a static representation that is rendered in the client’s browser.
Recommendation: Keep Logic Separate
Make sure to keep your UI (HTML/CSS) separate from your business logic (Java). For example:
// Java code to fetch user data
public String getUserData(int userId) {
// Simulating data fetching from a database
return "User Data for ID: " + userId;
}
In this code, we have logic to fetch user data. The resulting data needs to be passed to the HTML for rendering, such as:
<!-- HTML code to display user data -->
<div id="userData">
<h1>${userData}</h1>
</div>
This separation enables easier management of your code, making it more scalable and maintainable.
2. Not Escaping Special Characters
Another major pitfall involves not escaping special characters, particularly when rendering user-generated content in HTML. This can lead to Cross-Site Scripting (XSS) vulnerabilities—a serious security risk.
Example of a Pitfall:
// User-generated input
String userInput = "<script>alert('XSS');</script>";
String displayInput = "<div>" + userInput + "</div>";
In the snippet above, if displayInput
is rendered in the HTML without warning, it opens the application to XSS attacks.
Solution: Always Escape Output
You should always escape user-generated content before rendering it. This can be done using a library like Apache Commons Lang:
import org.apache.commons.text.StringEscapeUtils;
String safeInput = StringEscapeUtils.escapeHtml4(userInput);
String displayInput = "<div>" + safeInput + "</div>";
By escaping the input using StringEscapeUtils.escapeHtml4
, you ensure that the script tags are converted into harmless text.
3. Misusing JavaScript within HTML
Java developers may sometimes embed JavaScript directly into HTML improperly. It’s tempting to treat JavaScript as a backend language due to its power, but it serves functions on the client-side.
Common Mistake: Inline JavaScript
<script>
let value = "${javaValue}";
console.log(value);
</script>
When using inline JavaScript, be cautious with how you pass data. The above method can lead to issues if javaValue
contains special characters.
Best Practice: Use Data Attributes
A better approach would be to use data attributes in your HTML:
<div id="data" data-java-value="${javaValue}"></div>
<script>
let value = document.getElementById("data").getAttribute("data-java-value");
console.log(value);
</script>
This technique avoids direct insertion of Java values into JavaScript contexts, preventing potential syntax errors or runtime issues.
4. Not Leveraging Frameworks and Libraries
Java has a robust ecosystem with many libraries and frameworks that simplify the process of integrating with HTML. Failing to tap into these resources can lead developers to reinvent the wheel, often resulting in inefficient code.
Example Frameworks to Consider
- Spring MVC: It provides a powerful web framework that facilitates the easy integration of Java with various frontend technologies.
- Thymeleaf: A modern server-side Java template engine for web and standalone environments that allows HTML rendering in an intuitive way.
Leveraging these frameworks can help streamline development and enhance functionality significantly.
5. Underestimating AJAX and API Integration
Many Java developers focus exclusively on server-side rendering and ignore the potential of AJAX (Asynchronous JavaScript and XML) for creating dynamic pages. Avoiding AJAX can lead to slow, less interactive applications.
Utilizing AJAX with Java
Here’s an example of using AJAX to enhance your Java web application:
function fetchUserData(userId) {
fetch(`/user/${userId}`)
.then(response => response.json())
.then(data => {
document.getElementById("userData").innerText = data.userData;
});
}
@WebServlet("/user/*")
public class UserServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Assume logic to get user data
String jsonData = new Gson().toJson(userData);
response.setContentType("application/json");
response.getWriter().write(jsonData);
}
}
In this example, we use AJAX to asynchronously fetch user data from the server without reloading the entire page, improving user experience.
Lessons Learned
Integrating Java with HTML doesn't have to be fraught with pitfalls. By understanding the fundamentals and taking the necessary precautions—such as maintaining separate logic, escaping special characters, avoiding inline JavaScript, leveraging powerful frameworks, and utilizing AJAX—you can create robust applications that provide a compelling user experience.
To delve deeper into web integration challenges, consider reading about PHP and HTML interactions in the article "Blending PHP & HTML in Echo: A Common Pitfall" (https://tech-snags.com/articles/php-html-echo-pitfalls).
By applying these best practices, you can avoid common pitfalls and set yourself up for success in your Java web development journey. Happy coding!