Securing Java Apps on Azure: Terraform Group Essentials
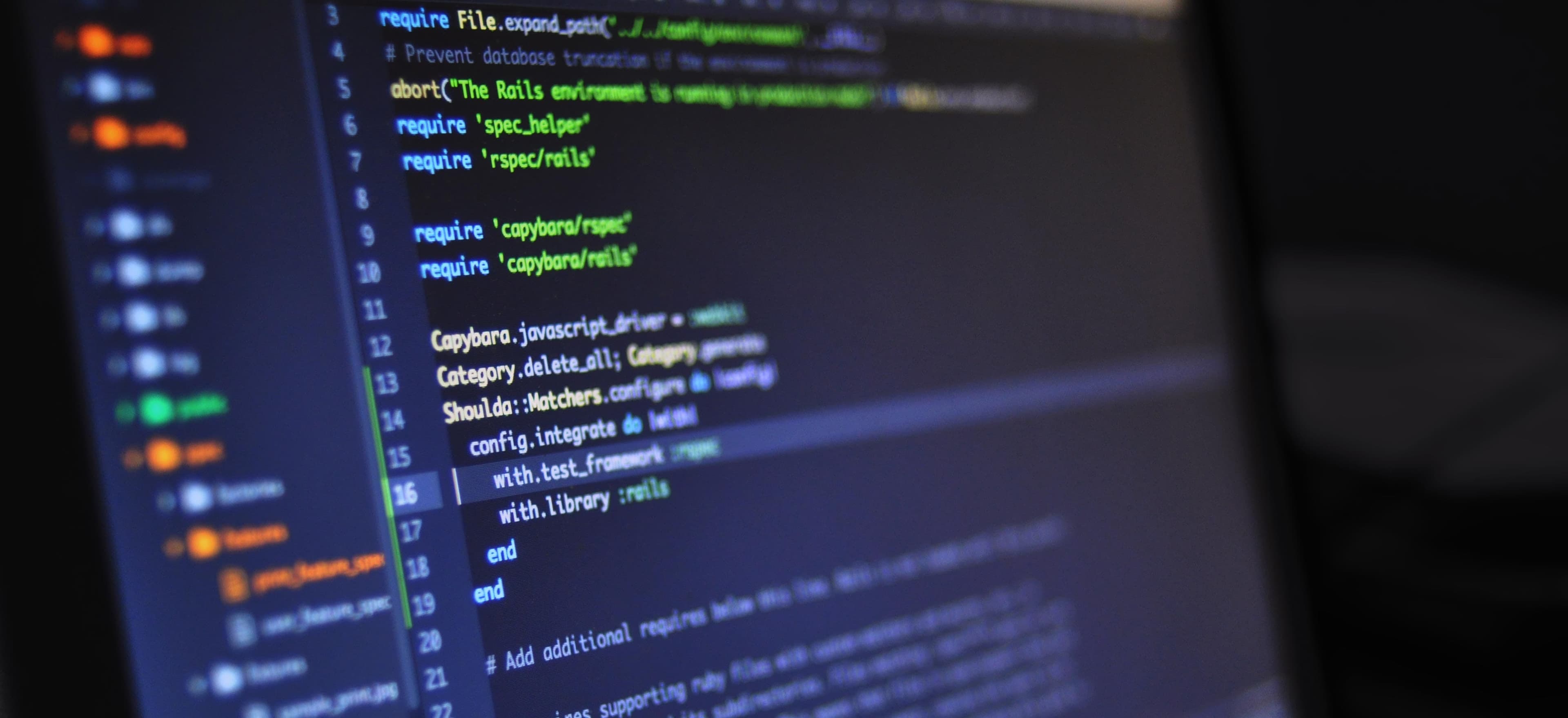
- Published on
Securing Java Apps on Azure: Terraform Group Essentials
As cloud computing becomes the norm, securing applications hosted on platforms like Microsoft Azure is crucial. When it comes to Java applications, using Infrastructure as Code (IaC) tools like Terraform can help streamline the management of security resources. This blog post will guide you through the process of securing your Java applications on Azure using Terraform to create security groups.
Why Use Security Groups?
Security groups are vital in controlling inbound and outbound traffic to your Azure resources. They serve as virtual firewalls, allowing you to manage network access. This is particularly important for Java applications where you might want to restrict access to specific ports or IP addresses.
Benefits of Security Groups
- Traffic Management: Define rules to control traffic flow.
- Segmentation: Different applications or services can have separate security rules.
- Scalability: Easily modify rules as your application grows or changes.
Before diving into how to create Azure Security Groups using Terraform, it's essential to understand how security works in Azure.
Understanding Azure Security
Azure uses a layered security approach, which includes the following components:
- Network Security Groups (NSGs): Control inbound and outbound traffic for Azure resources.
- Azure Firewall: A managed firewall service for advanced security.
- Application Security Groups: Group related VMs and apply security rules collectively.
For this article, we will focus on Network Security Groups since they provide the fundamental layer of security for your Azure resources.
Pre-requisites
Ensure you have the following set up before we proceed:
- An Azure account with necessary permissions.
- Terraform installed on your local machine.
- Basic knowledge of Java and cloud infrastructure.
Install Terraform
To get started with Terraform, you can download and install it here.
Setting Up Your Terraform Environment
Once you have Terraform installed, you need to configure it to interact with your Azure account.
Create a Terraform Configuration File
Start by creating a directory for your Terraform configuration files and create a file named main.tf
. This file will house all your Terraform code.
Sample Configuration
Here's a sample Terraform configuration to create an Azure Network Security Group:
provider "azurerm" {
features {}
}
resource "azurerm_resource_group" "example" {
name = "example-resources"
location = "East US"
}
resource "azurerm_network_security_group" "example" {
name = "example-nsg"
location = azurerm_resource_group.example.location
resource_group_name = azurerm_resource_group.example.name
security_rule {
name = "allow-http"
priority = 100
direction = "Inbound"
access = "Allow"
protocol = "Tcp"
source_port_range = "*"
destination_port_range = "80"
source_address_prefix = "*"
destination_address_prefix = "*"
}
security_rule {
name = "allow-https"
priority = 110
direction = "Inbound"
access = "Allow"
protocol = "Tcp"
source_port_range = "*"
destination_port_range = "443"
source_address_prefix = "*"
destination_address_prefix = "*"
}
security_rule {
name = "deny-all-inbound"
priority = 65000
direction = "Inbound"
access = "Deny"
protocol = "*"
source_port_range = "*"
destination_port_range = "*"
source_address_prefix = "*"
destination_address_prefix = "*"
}
}
Explanation of the Code
- Provider Block: Specifies that we'll be using the Azure Resource Manager (azurerm).
- Resource Group: Creates a resource group to house our NSG.
- Network Security Group: Defines the NSG itself.
- Security Rules: This section is crucial:
- The allow-http rule opens port 80 for HTTP traffic.
- The allow-https rule opens port 443 for secure HTTPS traffic.
- The deny-all-inbound rule ensures that all other inbound traffic is denied.
Why Security Rules Matter
In a Java application, typically running on a server, you may only want to expose HTTP and HTTPS traffic to the public. By allowing only specific ports and denying all others, you limit the attack surface considerably, enhancing security.
Initializing Terraform
Once your main.tf
file is prepared, navigate to the directory where it's saved and run the following command in your terminal:
terraform init
This command initializes Terraform, setting up the provider for Azure.
Applying Your Configuration
After initialization, you can apply your configuration, which will create the resources in Azure.
terraform apply
You will be prompted to confirm the creation of resources. Type yes and hit Enter.
Verifying the Creation
After the command is executed, you should verify if your Security Group is created:
- Log in to the Azure portal.
- Navigate to Resource Groups.
- Click on the resource group you created.
- You should see the Network Security Group listed under that resource group.
Additional Considerations
While setting up security groups, consider:
- Auditing Rules: Regularly review your NSGs for any unnecessary open ports or rules.
- Logging: Use Azure Network Watcher for monitoring and logging network traffic.
- Linking NSG to Resources: Ensure that your NSG is associated with the correct resources such as Virtual Machines or subnets.
Closing Remarks
Securing your Java application on Azure with Terraform is a crucial step in a robust cloud infrastructure strategy. With Network Security Groups, you can effectively manage and control inbound and outbound traffic, reducing your application’s vulnerability to threats.
For more detailed steps on creating Azure Security Groups with Terraform, you can refer to the existing article Creating Azure Security Group with Terraform, which complements the guidance provided in this post.
By following the steps outlined in this blog post, you can ensure that your Java applications are well-protected on Azure, paving the way for scalable and secure cloud deployments.
Happy coding and stay secure!
Checkout our other articles