Unlocking Private Fields in Java with Reflection: A Guide
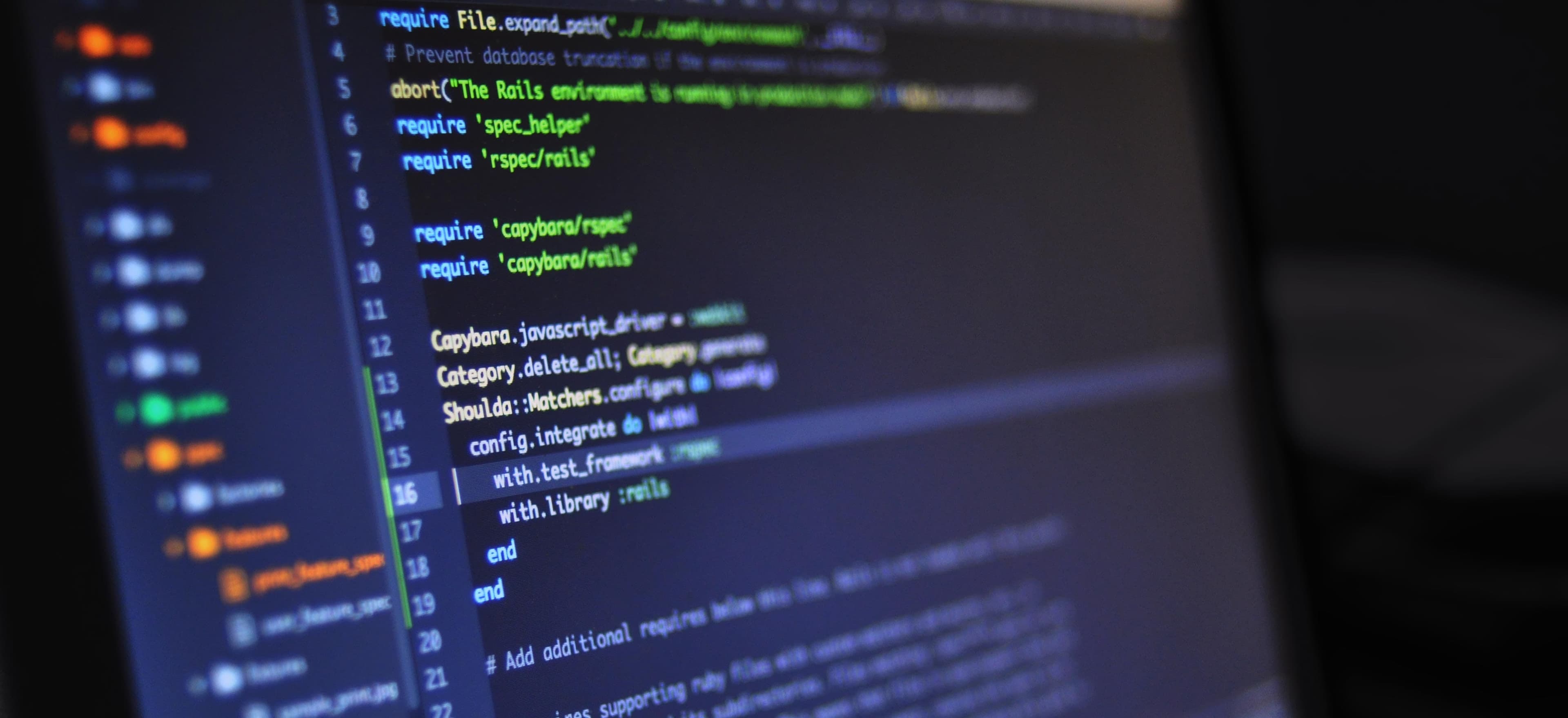
- Published on
Unlocking Private Fields in Java with Reflection: A Guide
Java, known for its strong encapsulation, enforces access control through visibility modifiers like private
. This mechanism not only protects the integrity of the data but also maintains a clear boundary of access for different classes. However, there are scenarios, especially during testing or when working with legacy code, where you might need to access private fields. To this end, Java provides a powerful mechanism called Reflection.
In this blog post, we will explore how to unlock private fields in Java using Reflection, along with insights into why you might need it, how it works, and some best practices to consider.
Understanding Reflection in Java
Reflection is a feature in Java that allows the program to inspect and manipulate classes, interfaces, fields, and methods at runtime. This capability comes in handy in several scenarios such as:
- Testing: Accessing private fields in unit tests.
- Frameworks: Many frameworks (like Spring and Hibernate) utilize Reflection for dependency injection and ORM.
- Serialization: Libraries that serialize and deserialize objects may need access to private fields.
How Does Reflection Work?
At its core, Reflection enables you to investigate and manipulate the properties of classes without knowing their details at compile time. The principal classes used in Java Reflection include:
Class
: Represents the class definition.Field
: Represents the fields (variables) of the class.Method
: Represents the methods of the class.
Accessing Private Fields
To access private fields, you will typically follow these steps:
- Get the
Class
object of the target class. - Retrieve the
Field
object for the private variable. - Set the field accessible using the
setAccessible(true)
method. - Read or modify the field value as needed.
Example: Accessing a Private Field
Let's say we have a simple class with a private field.
public class User {
private String name;
public User(String name) {
this.name = name;
}
// Getter to demonstrate accessing private fields
public String getName() {
return name;
}
}
Now, we can use Reflection to access the name
field of User
.
import java.lang.reflect.Field;
public class ReflectionExample {
public static void main(String[] args) {
try {
// Step 1: Create an instance of User
User user = new User("Alice");
// Step 2: Get the Class object
Class<?> userClass = user.getClass();
// Step 3: Access the private field
Field nameField = userClass.getDeclaredField("name");
nameField.setAccessible(true); // Bypass private access
// Step 4: Get the value of the private field
String nameValue = (String) nameField.get(user);
System.out.println("Name: " + nameValue); // Outputs: Name: Alice
// Optionally, modify the private field
nameField.set(user, "Bob");
System.out.println("Updated Name: " + user.getName()); // Outputs: Updated Name: Bob
} catch (NoSuchFieldException | IllegalAccessException e) {
e.printStackTrace();
}
}
}
Code Explanation
- Create an Instance: We first instantiate the
User
class. - Get Class Object:
user.getClass()
returns the Class object associated withUser
. - Access the Field:
getDeclaredField("name")
fetches the private field. - Bypass Access Control:
setAccessible(true)
allows access to the private field. - Get and Modify Field Value: We retrieve the field value and also demonstrate how to set a new value.
Why Use Reflection?
While Reflection can be a powerful tool, it should be used judiciously. Here are some reasons and scenarios where Reflection is beneficial:
- Testing: In unit tests, you may often want to verify internal state without exposing those variables through public getters for the sake of encapsulation.
- Frameworks and Libraries: Advanced frameworks use Reflection to work with classes dynamically, enabling functionality like Annotation processing, DI (Dependency Injection), etc.
- Serialization: Libraries like Jackson or Gson use Reflection to serialize/deserialize objects easily.
Best Practices and Cautions
While Reflection can be powerful, it isn't without its pitfalls:
1. Performance Overhead
Reflection is inherently slower than standard property access. If performance is critical, use Reflection sparingly.
2. Break Encapsulation
Using Reflection can lead to tighter coupling and diminished encapsulation, which can make your code harder to maintain.
3. Security and Permissions
Setting a field accessible can lead to security vulnerabilities if not handled carefully. Applications should restrict Reflection usage when handling sensitive data.
4. Unsupported Class Change
If you modify private fields based on assumptions about the class implementation and it changes in future versions, you could break your code.
Alternatives to Reflection
Whenever possible, consider other alternatives to Reflection:
- Getters/Setters: Provide public methods to access and modify private fields.
- Dependency Injection: Utilize frameworks like Spring which manage object creation and dependencies without resorting to Reflection.
Key Takeaways
Using Reflection to unlock private fields in Java can be a powerful technique when employed in the right circumstances. It's important to tread carefully and understand the implications of bypassing standard access controls. By being informed about both the risks and benefits, you can leverage this feature when absolutely necessary, ensuring that you maintain code quality and integrity.
For further reading on Java Reflection, you can check the official Java documentation on Reflection, or explore other advanced topics like Annotations in Java.
Remember, while Reflection can open doors that might otherwise be closed, the best designs often avoid needing to resort to it at all. Happy coding!
Checkout our other articles