Preventing Memory Leaks in Java ExecutorService Usage
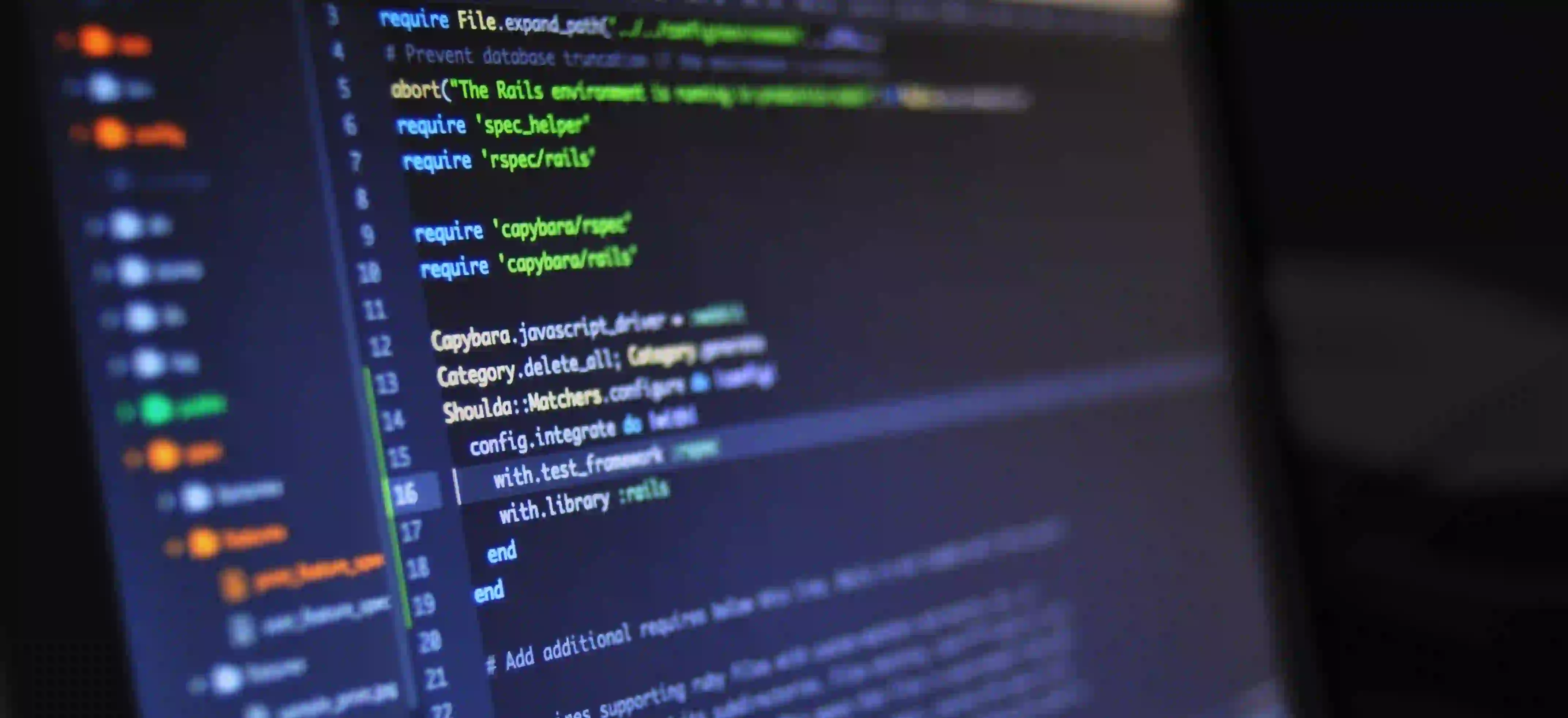
Preventing Memory Leaks in Java ExecutorService Usage
In the realm of Java development, efficient resource management is paramount. One of the most significant pitfalls developers can encounter is memory leaks, particularly when utilizing ExecutorService. This blog post delves into the strenuous overviews of ExecutorService, common scenarios that lead to memory leaks, and practical strategies to prevent them. Let's explore this crucial topic.
Understanding ExecutorService
ExecutorService is a part of the Java Concurrency framework, which simplifies the management of thread pools. It allows developers to submit tasks for asynchronous execution, helping to offload the burden of thread management. Key benefits include:
- Automatic management of multiple tasks.
- Reusability of threads, enhancing performance.
- Simplified code structure for asynchronous operations.
However, improper usage can lead to memory leaks, which can cripple application performance.
Common Scenarios Leading to Memory Leaks
1. Unmanaged Thread Pools
When threads are not properly shut down, resources remain allocated, leading to memory leaks. Consider the following code:
ExecutorService executorService = Executors.newFixedThreadPool(10);
executorService.submit(() -> {
// Implement task
});
// Forgetting to shut down the executor
In this example, the executor is never shut down, which means the threads will linger in memory even after the application has completed its execution. To mitigate this, always ensure to call shutdown()
or shutdownNow()
.
2. Long-lived References
Maintaining references to the tasks submitted to the executor can cause memory leaks. These references defer garbage collection, keeping the tasks alive. The simplest fix is as follows:
List<Future<?>> futures = new ArrayList<>();
for (int i = 0; i < 100; i++) {
Future<?> future = executorService.submit(() -> {
// Task
});
futures.add(future);
}
// Clean up references
futures.clear();
In this scenario, the futures.clear()
method ensures no lingering references exist, aiding garbage collection.
3. Retaining Scalability
Continuously submitting tasks without regard for the state of your executor can lead to unintended memory spikes. For instance:
while (!Thread.currentThread().isInterrupted()) {
executorService.submit(() -> {
// Task processing
});
}
If this code doesn’t properly manage threads, it will lead to a massive accumulation of tasks, causing memory exhaustion. Instead, you should monitor your system resources and limit the number of active submissions.
Best Practices to Prevent Memory Leaks
Preventing memory leaks in ExecutorService hinges on understanding resource management. Here are strategies to follow:
1. Use Appropriate Executor Types
Choose the executor type that best matches the use case. Here are a couple of common types:
- FixedThreadPool: For a limited number of threads, suitable for short-lived tasks.
- CachedThreadPool: For flexible task management but can be memory-intensive for long-running tasks.
Example:
ExecutorService executorService = Executors.newCachedThreadPool();
2. Graceful Shutdown of Executers
Always shut down your executor service. Preferably, use:
executorService.shutdown();
This allows previously submitted tasks to complete execution before shutting down. In scenarios needing immediate response, shutdownNow()
can be utilized, but be cautious as it interrupts ongoing tasks.
3. Implement a Monitoring Mechanism
In production systems, it's crucial to monitor performance metrics to detect any anomalies. Consider tools such as:
- Java Management Extensions (JMX): For monitoring JVM resources.
- Metrics Libraries (e.g., Dropwizard Metrics): To track ExecutorService performance.
4. Leverage Callable and Future judiciously
Utilizing Callable<T>
with Future<T>
provides error handling and result retrieval mechanisms. This balances task execution while ensuring you manage exceptions appropriately:
Callable<String> task = () -> {
// Task logic that might throw an exception
return "Task Completed!";
};
Future<String> result = executorService.submit(task);
try {
String output = result.get();
} catch (InterruptedException | ExecutionException e) {
// Handle exceptions appropriately
}
5. Utilize Weak References
In special use cases where objects managed by the executor service are not critical, consider using weak references to avoid memory retention:
WeakReference<MyObject> weakObj = new WeakReference<>(new MyObject());
Runnable task = () -> {
MyObject obj = weakObj.get();
if (obj != null) {
// Perform task
}
};
executorService.submit(task);
This allows garbage collection to reclaim memory when no strong references exist.
Example Implementation
Here’s a comprehensive example that encapsulates the practices discussed:
import java.util.concurrent.*;
public class MemoryLeakPrevention {
private final ExecutorService executorService;
public MemoryLeakPrevention() {
this.executorService = Executors.newFixedThreadPool(5);
}
public void submitTasks() {
for (int i = 0; i < 100; i++) {
final int taskId = i;
executorService.submit(() -> {
try {
Thread.sleep(1000);
System.out.println("Task " + taskId + " completed");
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
});
}
}
public void shutdownExecutor() {
executorService.shutdown();
try {
if (!executorService.awaitTermination(60, TimeUnit.SECONDS)) {
executorService.shutdownNow();
}
} catch (InterruptedException e) {
executorService.shutdownNow();
}
}
public static void main(String[] args) {
MemoryLeakPrevention mlp = new MemoryLeakPrevention();
mlp.submitTasks();
mlp.shutdownExecutor();
}
}
In this example, the ExecutorService is properly utilized, and tasks are managed with structured shutdown behavior, minimizing the risk of memory leaks.
The Bottom Line
Memory leaks can silently cripple application performance, especially when using ExecutorService for concurrent task execution in Java. By adopting best practices like appropriate executor types, graceful shutdowns, and careful task management, you can successfully mitigate these risks.
Always remain vigilant in resource management, as it leads to robust and sustainable applications. For further reading on concurrency in Java, refer to Java Concurrency in Practice or explore the Java Documentation.
With these insights in mind, may your Java journey be free from memory leaks!