Mastering NumberFormatException in Java: Common Pitfalls
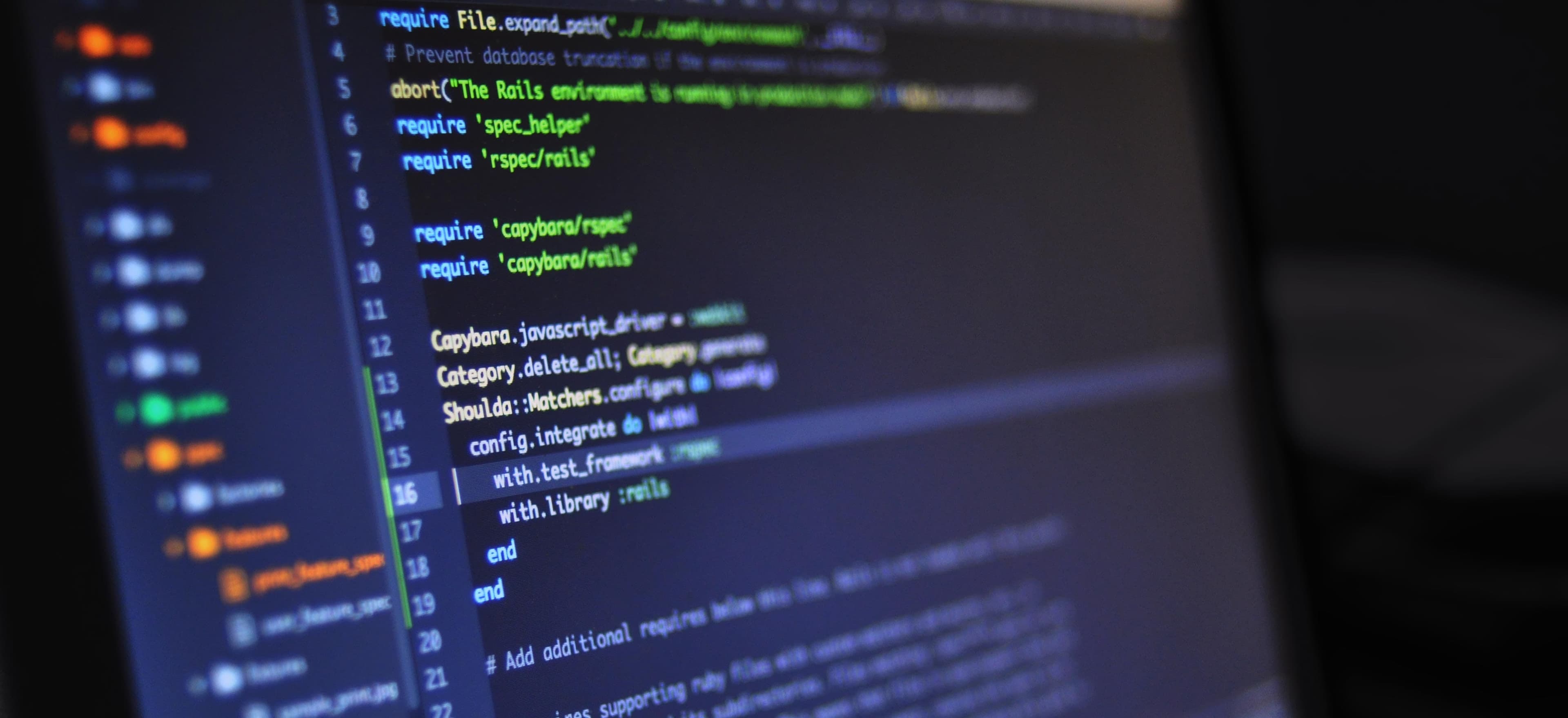
- Published on
Mastering NumberFormatException in Java: Common Pitfalls
Java is a robust programming language that empowers developers with a wide range of functionalities. Among the many features are the elegant ways to handle input and numeric representations. However, one of the common challenges developers face while dealing with numeric data in Java is the NumberFormatException
. This exception can surface under various circumstances, mostly related to the parsing of strings to numeric values, leading to frustrating runtime errors. This blog post aims to delve into NumberFormatException
, illustrating common pitfalls and offering insights on how to manage these errors effectively.
What is NumberFormatException?
In Java, NumberFormatException
is a subclass of IllegalArgumentException
. It is thrown when an application attempts to convert a string that does not have an appropriate format for a numeric type (like int, double, etc.). This problem often occurs in scenarios such as user input validation, data reading from files, or even when data is sourced from APIs.
Key Reasons for NumberFormatException
- Input Format Errors: Users may enter unexpected or invalid characters. For instance, letters in a number field.
- Regional Differences: When cultures format numbers differently, for instance, using commas or periods.
- Null or Empty Strings: Attempting to parse a null or empty string will also lead to this exception.
Example 1: Basic Usage of NumberFormatException
Let’s look at a simple example of how NumberFormatException
can occur.
public class NumberFormatExample {
public static void main(String[] args) {
String numberString = "123a"; // Invalid numeric value
try {
int number = Integer.parseInt(numberString);
} catch (NumberFormatException e) {
System.out.println("Error: " + e.getMessage());
}
}
}
In this code snippet, the string numberString
contains a character that makes it impossible for the parseInt
method to convert it into an integer. The catch
block captures the NumberFormatException
, allowing for graceful error handling.
Why This Matters:
Error handling enables the program to continue running without crashing, thus improving the user experience. When you encounter this exception, consider verifying or validating input before attempting to parse integers or other numeric types.
Common Scenarios Leading to NumberFormatException
Scenario 1: User Input
A frequent source of NumberFormatException
is user input. If you’re building a console application or a GUI, input from users can be unpredictable.
Code Snippet for User Input
import java.util.Scanner;
public class UserInputExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter a number: ");
String userInput = scanner.nextLine(); // Taking user input
try {
int number = Integer.parseInt(userInput);
System.out.println("You entered: " + number);
} catch (NumberFormatException e) {
System.out.println("Invalid number format. Please enter a valid integer.");
}
}
}
In this snippet, if the user enters any non-numeric character, the program catches the NumberFormatException
and prompts them to enter a valid integer.
Scenario 2: Reading From Files
When reading numeric data from files, the data retrieved may not always conform to the expected format.
Code Snippet for File Reading
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReadingExample {
public static void main(String[] args) {
String fileName = "data.txt"; // Assume this file contains numeric data
try (BufferedReader br = new BufferedReader(new FileReader(fileName))) {
String line;
while ((line = br.readLine()) != null) {
try {
int number = Integer.parseInt(line.trim()); // Trimming input to prevent exceptions
System.out.println("Number read: " + number);
} catch (NumberFormatException e) {
System.out.println("Invalid format in line: '" + line + "'");
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Here, we read each line from a file and attempt to parse it as an integer. Any invalid line raises a NumberFormatException
, which is handled appropriately.
Why Use Try-Catch for Input Validation?
Using try-catch blocks around input parsing prevents the entire application from crashing due to a single erroneous input.
Handling Regional Differences
When dealing with numeric formats, be mindful of cultural differences. In some countries, commas are used for decimal points, while in others, periods serve the same purpose.
Code Snippet for Handling Decimal Formats
import java.text.NumberFormat;
import java.text.ParseException;
import java.util.Locale;
public class LocaleNumberFormat {
public static void main(String[] args) {
String numberString = "1,234.56"; // Example for US format
NumberFormat format = NumberFormat.getInstance(Locale.US);
try {
Number number = format.parse(numberString);
System.out.println("Parsed Number: " + number.doubleValue());
} catch (ParseException e) {
System.out.println("Parse exception: " + e.getMessage());
}
}
}
In this instance, the code uses NumberFormat
to consider the locale when parsing numbers, thus minimizing the chances of a format exception. Read more about handling internationalization in Java here.
Closing Remarks
The NumberFormatException
can be a common pitfall for Java developers, but with proper error handling and validation techniques, it can be managed effectively. By understanding and anticipating potential sources of this exception—such as user input, file reading, and cultural differences—you’re better equipped to handle numeric values in Java robustly.
When dealing with numeric conversions, always apply the following best practices:
- Validate user input before parsing.
- Utilize try-catch blocks to handle exceptions seamlessly.
- Consider regional formatting when parsing or displaying numeric values.
By following these strategies, you not only improve the reliability of your Java applications but also enhance the overall user experience. Keep pushing forward in mastering Java; the world of programming awaits you!
Checkout our other articles