Resolving Circular Dependencies in Java Packages Made Easy
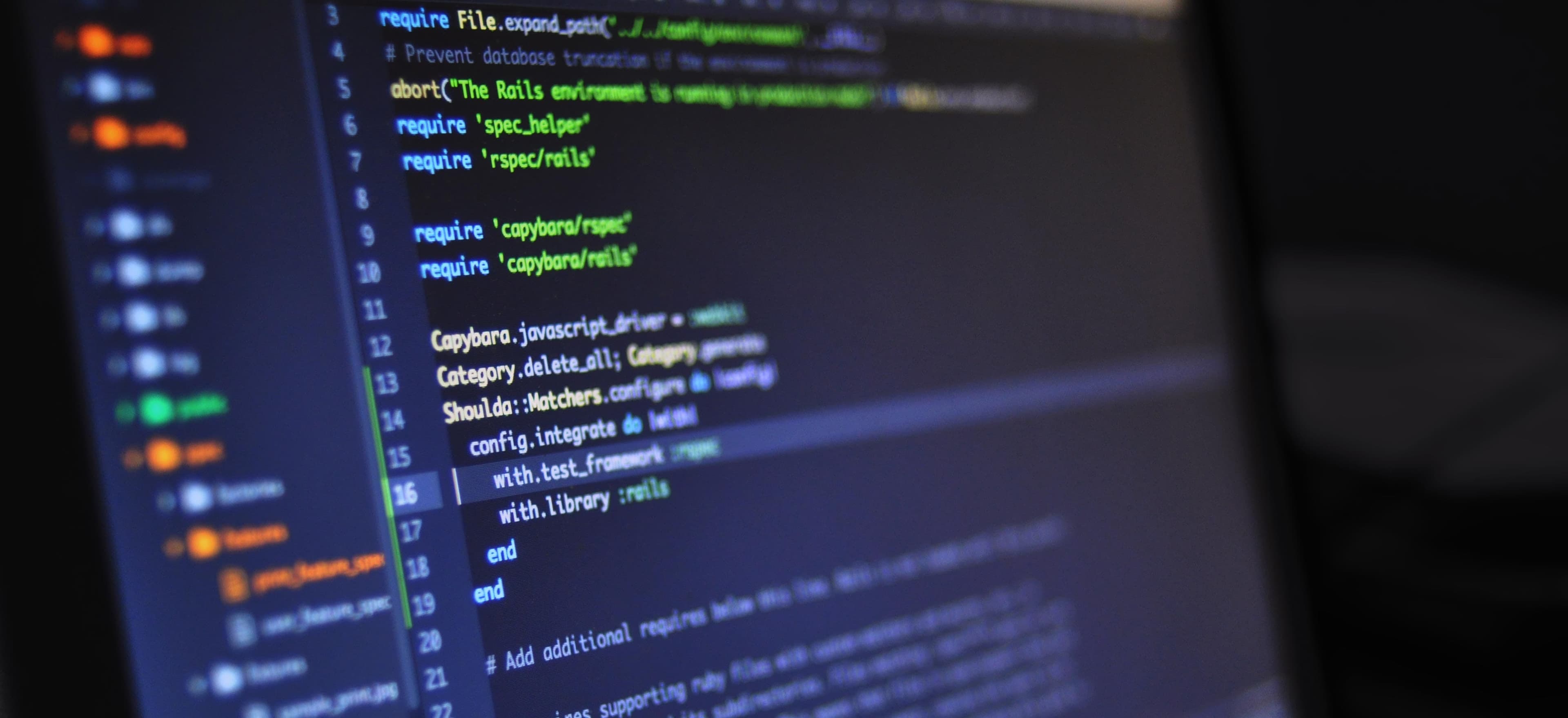
- Published on
Resolving Circular Dependencies in Java Packages Made Easy
Circular dependencies are a common headache in software development, particularly in the realm of Java. This issue arises when two or more packages depend on each other directly or indirectly, leading to a range of complications such as increased complexity, challenging testing, and potentially disastrous performance hits. In this blog post, we will delve into the intricacies of circular dependencies in Java, their causes, and various strategies to resolve them effectively.
Understanding Circular Dependencies
A circular dependency occurs when package A depends on package B while package B, in turn, depends on package A. This circular relationship can also appear indirectly, such as when package A depends on package B, package B depends on package C, and package C depends back on package A.
For instance, consider the following relationships:
- Package A → Package B
- Package B → Package C
- Package C → Package A
The Consequences of Circular Dependencies
Circular dependencies can lead to:
- Tight Coupling: Classes become tightly coupled, making changes more difficult.
- Reduced Readability: Understanding the flow of the code becomes challenging.
- Difficulties in Testing: Isolating components for unit tests becomes complicated.
- Increased Complexity: The system can become more intricate and harder to maintain.
To demonstrate these issues, consider a simple Java project structure:
// Package A
package a;
import b.B;
public class A {
private B b;
public A() {
b = new B();
}
public void display() {
System.out.println("Class A");
}
}
// Package B
package b;
import a.A;
public class B {
private A a;
public B() {
a = new A();
}
public void display() {
System.out.println("Class B");
}
}
In this example, both classes in packages A and B reference each other, creating a circular dependency. This code will not compile due to the cyclic nature of the references.
How to Resolve Circular Dependencies
1. Refactoring Your Code
One of the most effective ways to eliminate circular dependencies is to refactor your code. Refactoring involves rethinking the design and structure of your classes and packages.
- Introduce an Interface: Extract the common functionality into a new interface. Both packages can then depend on this interface instead of each other.
// Interface
package common;
public interface Displayable {
void display();
}
// Package A
package a;
import common.Displayable;
public class A implements Displayable {
public void display() {
System.out.println("Class A");
}
}
// Package B
package b;
import common.Displayable;
public class B implements Displayable {
public void display() {
System.out.println("Class B");
}
}
In this scenario, both class A and class B implement the Displayable
interface, allowing you to remove the direct dependencies between the two packages.
2. Utilizing Dependency Injection
Dependency Injection (DI) is a design pattern that can help alleviate circular dependencies by decoupling the instantiation of classes from their usage. The DI approach allows you to inject dependencies at runtime instead of creating them within each class.
Here’s a basic example of using a DI framework such as Spring:
// Class A
package a;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class A {
private B b;
@Autowired
public A(B b) {
this.b = b;
}
public void display() {
System.out.println("Class A");
}
}
// Class B
package b;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
@Component
public class B {
private A a;
@Autowired
public B(A a) {
this.a = a;
}
public void display() {
System.out.println("Class B");
}
}
Here, Spring manages the object creation for you, allowing both classes to reference each other without directly instantiating the other class. This pattern reduces coupling and makes your code cleaner and more maintainable.
3. Embracing Event-Driven Architectures
In situations where tight coupling is unavoidable, consider shifting towards an event-driven architecture. By using an event bus, two classes can communicate without directly calling each other. This decouples the interaction entirely.
// EventBus
import java.util.HashMap;
import java.util.Map;
public class EventBus {
private static Map<Class<?>, javafx.event.EventHandler<?>> handlers = new HashMap<>();
public static <T> void subscribe(Class<T> eventType, javafx.event.EventHandler<T> handler) {
handlers.put(eventType, handler);
}
public static <T> void publish(T event) {
javafx.event.EventHandler<T> handler = (javafx.event.EventHandler<T>) handlers.get(event.getClass());
if (handler != null) {
handler.handle(event);
}
}
}
// Events
class AEvent {}
class BEvent {}
// Package A
package a;
public class A {
public A() {
EventBus.subscribe(AEvent.class, event -> display());
}
public void display() {
System.out.println("Class A");
}
}
// Package B
package b;
public class B {
public B() {
EventBus.subscribe(BEvent.class, event -> display());
}
public void display() {
System.out.println("Class B");
}
}
// Somewhere in your application
EventBus.publish(new AEvent());
EventBus.publish(new BEvent());
This implementation allows classes A and B to communicate via events, significantly reducing direct dependencies.
4. Using a Mediator Pattern
The Mediator pattern introduces a third-party mediator class that handles the interactions between the two dependent classes. This promotes loose coupling and is particularly useful in complex systems.
// Mediator
public class Mediator {
private A a;
private B b;
public Mediator(A a, B b) {
this.a = a;
this.b = b;
}
public void notify(String message) {
if (message.equals("A to B")) {
b.display();
} else {
a.display();
}
}
}
// Package A
package a;
public class A {
private Mediator mediator;
public A(Mediator mediator) {
this.mediator = mediator;
}
public void doSomething() {
mediator.notify("A to B");
}
public void display() {
System.out.println("Class A");
}
}
// Package B
package b;
public class B {
private Mediator mediator;
public B(Mediator mediator) {
this.mediator = mediator;
}
public void doSomething() {
mediator.notify("B to A");
}
public void display() {
System.out.println("Class B");
}
}
// Usage
Mediator mediator = new Mediator(new A(mediator), new B(mediator));
mediator.notify("A to B");
In this pattern, both classes communicate through a mediator, which reduces the interactions and dependencies between the two directly.
The Closing Argument
Resolving circular dependencies in Java packages can at first appear daunting, but through the conscious application of thoughtful design patterns, interfaces, and DI frameworks, you can successfully navigate around these obstacles. Understanding the underlying causes of circular dependencies allows you to implement effective strategies for resolution, ultimately leading to cleaner, more maintainable code.
By focusing on decoupling classes and utilizing robust architectural patterns like Dependency Injection, Event-Driven Architecture, and the Mediator pattern, you can enhance the readability, maintainability, and testability of your Java projects.
For more information on handling dependencies in Java, you can check out the Java Concurrency in Practice and related resources online.
Remember, success in programming often lies in clean, well-structured code. Avoid circular dependencies, and create a more robust architecture!
Checkout our other articles