Secure Your Java Application: Integrating Azure with Terraform
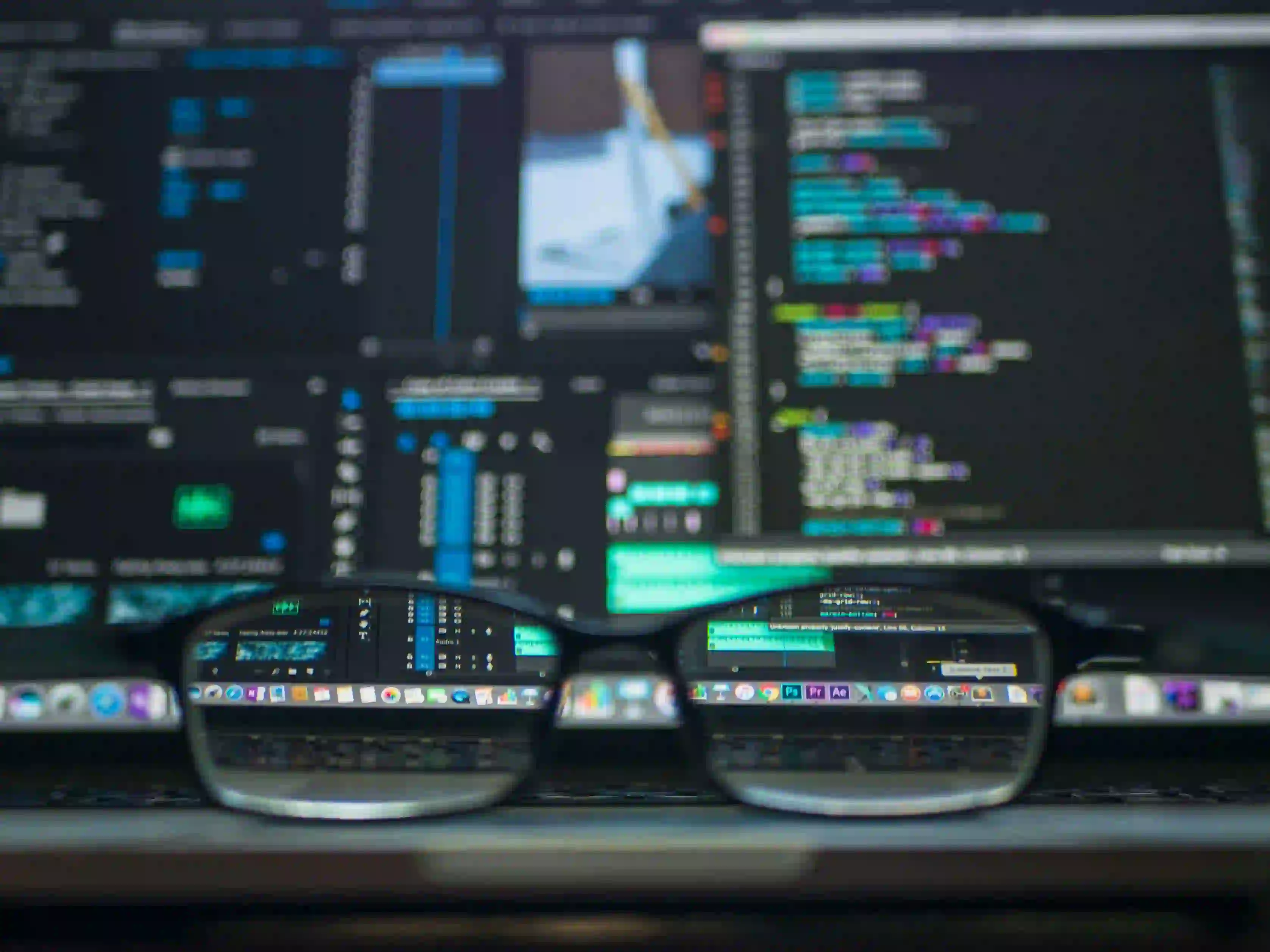
Secure Your Java Application: Integrating Azure with Terraform
In today's fast-paced world of software development, security is paramount. With the increasing reliance on cloud services, securing your applications has become more critical. One powerful method of enforcing security measures is by using Infrastructure as Code (IaC) tools, and Terraform is one of the most popular choices for this purpose.
In this post, we will discuss how to secure a Java application running in Azure by integrating it with Terraform. We will explore the various aspects of using Terraform to create Azure resources such as Azure Security Groups and will also provide practical code snippets to illustrate the concepts discussed.
Why Use Terraform?
Terraform is an open-source tool that allows developers to define and provision their infrastructure using a declarative configuration language. The benefits of using Terraform include:
- Integration with Multiple Providers: Terraform can manage resources across several cloud providers including Azure, AWS, and Google Cloud Platform.
- Version Control: Configuration files can be version-controlled, allowing track changes and rollback capabilities.
- Automation: Infrastructure can be created, modified, and destroyed automatically, minimizing human error.
- Consistency: Ensures that the same infrastructure is deployed every time, reducing the potential for bugs related to configuration drift.
For enhanced security in Azure, an important step is to use Azure Security Groups. You can read more about creating Azure Security Groups with Terraform in the existing article titled "Creating Azure Security Group with Terraform."
Prerequisites
Before diving into the integration of Azure with Terraform for securing your Java application, ensure the following prerequisites are in place:
- An Azure account with a subscription.
- Installed Terraform on your local development machine.
- Basic knowledge of Java application development.
Setting Up Azure Resources
Step 1: Define Your Terraform Configuration
First, letβs create a main configuration file for our Azure resources. Create a file named main.tf
.
provider "azurerm" {
features {}
}
resource "azurerm_resource_group" "my_rg" {
name = "my-java-app-rg"
location = "East US"
}
resource "azurerm_virtual_network" "my_vnet" {
name = "myJavaVNet"
address_space = ["10.0.0.0/16"]
location = azurerm_resource_group.my_rg.location
resource_group_name = azurerm_resource_group.my_rg.name
}
resource "azurerm_subnet" "my_subnet" {
name = "mySubnet"
resource_group_name = azurerm_resource_group.my_rg.name
virtual_network_name = azurerm_virtual_network.my_vnet.name
address_prefixes = ["10.0.1.0/24"]
}
The above code does the following:
- Provider Block: Defines the provider (Azure in this case) to manage resources.
- Resource Group: Creates a new resource group named
my-java-app-rg
. - Virtual Network: Sets up a virtual network to host the resources.
- Subnet: Defines a subnet within the virtual network.
Why This Matters
By properly defining these resources, you are essentially creating an isolated network environment for your Java application, which limits exposure to potential attacks.
Step 2: Create an Azure Security Group
Next, we want to create an Azure Security Group to define inbound and outbound traffic rules. Expand your main.tf
file with the following snippet.
resource "azurerm_network_security_group" "my_nsg" {
name = "myJavaNSG"
location = azurerm_resource_group.my_rg.location
resource_group_name = azurerm_resource_group.my_rg.name
security_rule {
name = "allow-ssh"
priority = 1000
direction = "Inbound"
access = "Allow"
protocol = "Tcp"
source_port_range = "*"
destination_port_range = "22"
source_address_prefix = "*"
destination_address_prefix = "*"
}
security_rule {
name = "allow-http"
priority = 1001
direction = "Inbound"
access = "Allow"
protocol = "Tcp"
source_port_range = "*"
destination_port_range = "80"
source_address_prefix = "*"
destination_address_prefix = "*"
}
security_rule {
name = "allow-https"
priority = 1002
direction = "Inbound"
access = "Allow"
protocol = "Tcp"
source_port_range = "*"
destination_port_range = "443"
source_address_prefix = "*"
destination_address_prefix = "*"
}
}
Why Security Rules Are Important
In this piece of code, we establish three security rules for SSH, HTTP, and HTTPS traffic. This is vital as it:
- Restricts Access: Only allows specific ports to be accessible, minimizing the attack surface.
- Establishes Priorities: Each rule has a unique priority ensuring the correct application of rules in order.
Step 3: Associate the Security Group with the Subnet
After defining the security group, you need to associate it with the subnet created earlier. Add this snippet to your main.tf
.
resource "azurerm_subnet_network_security_group_association" "my_subnet_nsg_association" {
subnet_id = azurerm_subnet.my_subnet.id
network_security_group_id = azurerm_network_security_group.my_nsg.id
}
This code links the previously created network security group with your subnet, enforcing the security rules you defined.
Step 4: Deploying Your Java Application
Once your Azure resources are set up and secured, you can deploy your Java application. Assuming you are using Azure App Services or Azure Kubernetes Service, ensure that your deployment pipelines utilize the VNet and NSG configured.
Java Application Security Practices
For an additional layer of security, consider these best practices for your Java application:
- Use Secure Connections: Always use HTTPS and validate SSL certificates.
- Application Secrets: Use Azure Key Vault to manage application secrets instead of hardcoding them.
- Input Validation: Always validate user inputs to prevent SQL Injection and Cross-Site Scripting attacks.
Closing Remarks
In conclusion, using Terraform to set up Azure resources for your Java application enhances security by streamlining and enforcing network configurations. By integrating Azure Security Groups and following best practices for security in your Java application, you can significantly reduce vulnerabilities.
For further reading on creating Azure Security Groups with Terraform, check out the article "Creating Azure Security Group with Terraform." Stay proactive in securing your applications as they grow, and leverage the tools available to you.
References
With these insights and practices, your journey towards a more secure Java application in Azure will be smoother and more effective. Happy coding!