Handling Edge Cases in Java's ConcurrentLinkedQueue
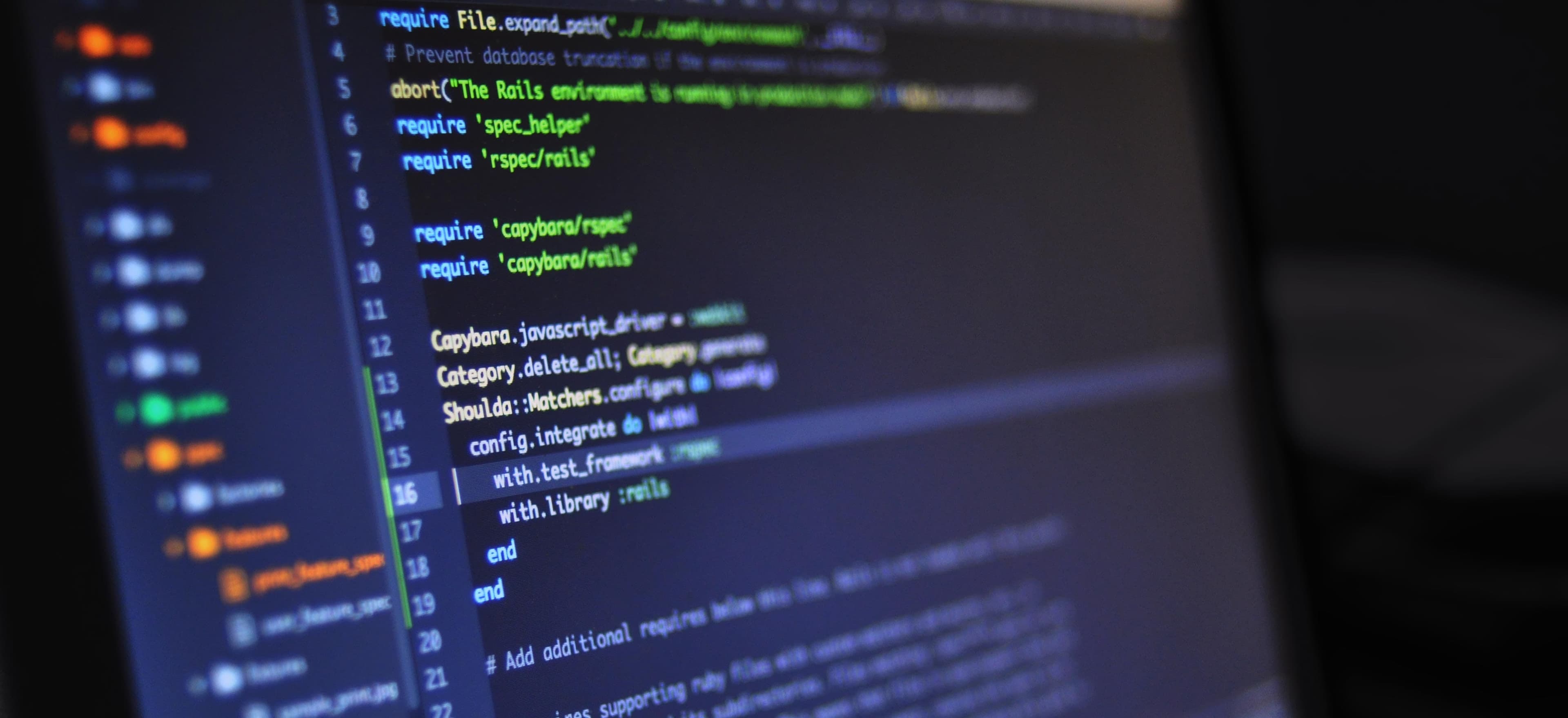
- Published on
Handling Edge Cases in Java's ConcurrentLinkedQueue
Java's ConcurrentLinkedQueue
is a non-blocking, thread-safe queue that enables concurrent access by multiple threads. Designed to perform efficiently under high contention, it is particularly useful in scenarios like producer-consumer models, where multiple threads are adding and removing tasks.
In this blog post, we'll dive into the edge cases you might encounter when using ConcurrentLinkedQueue
and how to handle them effectively. We will cover the queue's strengths, common pitfalls, and provide code examples to illustrate our points.
Understanding ConcurrentLinkedQueue
What is ConcurrentLinkedQueue?
ConcurrentLinkedQueue
is a part of the Java Collections Framework found in the java.util.concurrent
package. It implements the Queue
interface and uses a linked node structure to store its elements. This design allows for efficient insertion and removal operations, ideal for highly concurrent environments.
Key Features
- Non-blocking Operations: All operations are non-blocking, ensuring that threads are not put into a waiting state during insertion or removal.
- Thread Safety: The queue safely supports multiple threads performing operations simultaneously without requiring explicit synchronization.
Common Edge Cases
Although ConcurrentLinkedQueue
is robust, understanding potential edge cases can help you use it more effectively. Let's cover some of these edge cases and how to handle them.
1. Empty Queue
One fundamental case is when the queue is empty. Attempting to remove an element from an empty queue does not throw an exception but returns null
, which could lead to unexpected behavior if not handled correctly.
Example Code
import java.util.concurrent.ConcurrentLinkedQueue;
public class QueueExample {
public static void main(String[] args) {
ConcurrentLinkedQueue<String> queue = new ConcurrentLinkedQueue<>();
// Attempting to dequeue from an empty queue
String result = queue.poll(); // returns null if the queue is empty
if (result == null) {
System.out.println("The queue is empty, nothing to dequeue.");
}
}
}
In this code, we check for null
after calling poll()
, demonstrating the safe way to handle an empty queue.
2. Out-of-Order Processing
When dealing with multiple threads, there is a possibility of out-of-order processing of elements due to concurrent operations. You may assume that items will be processed in the order they were added, but with concurrent modifications, this can lead to issues.
Example Code
import java.util.concurrent.ConcurrentLinkedQueue;
public class OutOfOrderExample {
public static void main(String[] args) {
ConcurrentLinkedQueue<Integer> queue = new ConcurrentLinkedQueue<>();
// Adding elements to the queue
for (int i = 1; i <= 5; i++) {
queue.add(i);
}
// Processing elements in a separate thread
new Thread(() -> {
while (!queue.isEmpty()) {
Integer value = queue.poll();
System.out.println("Processed: " + value);
}
}).start();
// Another processing thread simulating out-of-order execution
new Thread(() -> {
while (!queue.isEmpty()) {
Integer value = queue.poll();
if (value != null) {
System.out.println("Thread 2 processed: " + (value + 10));
}
}
}).start();
}
}
In this example, we have two threads working off the same queue. They may process elements out of the order in which they were added. You should be aware of this if the order of processing is vital for your application logic.
3. Memory Consumption
Although ConcurrentLinkedQueue
does not tie up threads, the memory footprint can increase with high contention. Every entry consumes a node object, and if you have large numbers of threads performing concurrent add/remove operations, memory usage can spike.
Mitigation Strategy
- Regularly monitor the queue size and ensure that your program can handle the increased memory consumption.
- If extremely high memory usage becomes a concern, consider using bounded queues (like
ArrayBlockingQueue
).
4. Traversing the Queue
If you want to traverse the queue while others may be modifying it, you need to note that you cannot simply iterate it while removing elements. If you need to ensure that the elements processed are actually valid at that point, consider using a local copy for processing.
Example Code
import java.util.concurrent.ConcurrentLinkedQueue;
public class TraversingExample {
public static void main(String[] args) {
ConcurrentLinkedQueue<String> queue = new ConcurrentLinkedQueue<>();
queue.add("A");
queue.add("B");
queue.add("C");
// Process a snapshot of the queue
for (String item : queue.toArray(new String[0])) {
System.out.println("Processing: " + item);
// Simulate removal in the original queue
queue.poll(); // It's safe, but will affect next iterations
}
}
}
Using toArray()
creates a snapshot that allows safe traversal without worrying about concurrent modifications.
Troubleshooting ConcurrentLinkedQueue
Should you run into problems when using ConcurrentLinkedQueue
, here are generic steps you can take:
-
Log Queue States: Always log the queue contents before and after critical operations to diagnose issues.
-
Use Debugging Tools: Use Java Debugging tools to track thread execution and possible locks or delays.
-
Review Thread Usage: Confirm that your thread-handling logic is designed to respect the nature of
ConcurrentLinkedQueue
.
In Conclusion, Here is What Matters
The ConcurrentLinkedQueue
class is a powerful tool for concurrent programming in Java. However, understanding its edge cases can help you avoid pitfalls that may lead to incorrect assumptions about its behavior.
By handling cases like empty queues, out-of-order processing, and appropriate ways to traverse or monitor memory consumption, you can leverage ConcurrentLinkedQueue
safely.
For further reading, consider exploring the following links:
- Java Documentation: ConcurrentLinkedQueue
- Java Concurrency in Practice - This book provides a deeper dive into concurrent programming in Java.
With the right knowledge in your toolkit, you can navigate the complexities of concurrency in Java effectively. Happy coding!
Checkout our other articles