Avoiding Common Pitfalls in Java JMX Monitoring
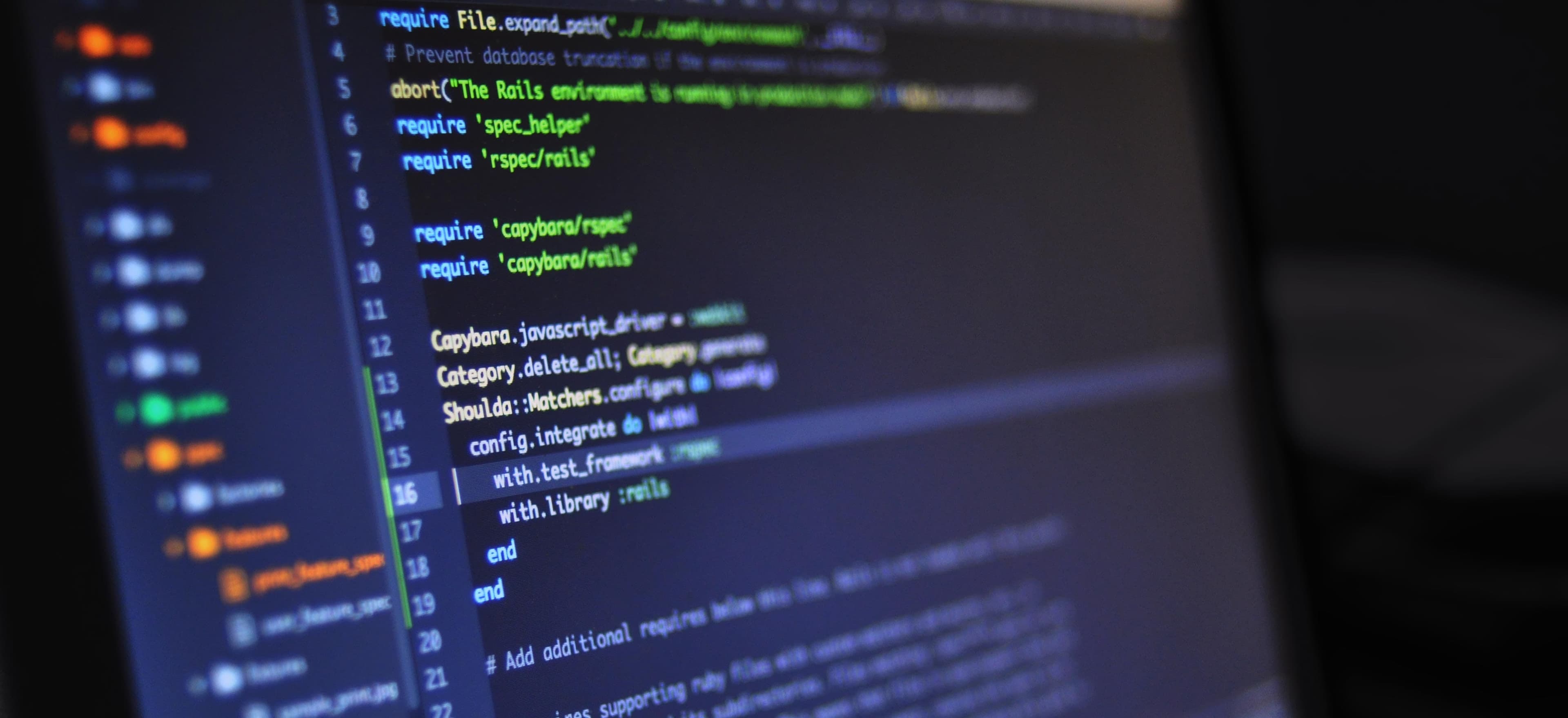
- Published on
Avoiding Common Pitfalls in Java JMX Monitoring
Java Management Extensions (JMX) provides a powerful way to manage and monitor Java applications. It allows developers to track system performance, manage resources, and control operational state via MBeans (Managed Beans). Despite its benefits, many developers encounter pitfalls that can hinder effective monitoring. In this blog post, we will explore common pitfalls in Java JMX monitoring and how to avoid them.
Understanding JMX
Before diving into pitfalls, let's establish a brief understanding of JMX. JMX allows you to represent your application's resources in a standardized way, enabling you to manage them via a set of interfaces. MBeans expose the application’s attributes and operations, facilitating remote management and monitoring.
Why Use JMX?
Some key reasons for employing JMX include:
- Real-time Monitoring: Observe your application’s health in real time.
- Dynamic Management: Modify attribute values or change configuration on the fly.
- Standardized Interface: Use standard protocols like RMI, which ensures flexibility.
For more detailed information about JMX, you can refer to Oracle's JMX Documentation.
Common Pitfalls in JMX Monitoring
1. Not Defining MBeans Properly
One of the most common pitfalls in JMX monitoring is the improper definition and registration of MBeans.
Example:
public class SystemConfig implements SystemConfigMBean {
private String dbUrl;
public SystemConfig(String dbUrl) {
this.dbUrl = dbUrl;
}
public String getDbUrl() {
return dbUrl;
}
public void setDbUrl(String dbUrl) {
this.dbUrl = dbUrl;
}
}
Why It Matters: If an MBean attribute is not defined correctly, then it can lead to issues when trying to access or update those attributes.
Solution: Make sure to enforce proper naming conventions and ensure that MBeans implement the corresponding interfaces.
2. Ignoring Object Naming Constraints
Java JMX uses the ObjectName class to uniquely identify MBeans within an application. Many forget to follow the naming convention, leading to conflicts.
Example:
MBeanServer mbs = ManagementFactory.getPlatformMBeanServer();
ObjectName objectName = new ObjectName("com.example:type=SystemConfig,name=Config1");
mbs.registerMBean(new SystemConfig(), objectName);
Why It Matters: Incorrect naming can cause InstanceAlreadyExistsException
. It’s crucial that names are unique to avoid overwriting existing MBeans.
Solution: Follow a systematic naming pattern for MBeans and always check if an MBean already exists before registration.
3. Overloading MBeans
While it may seem efficient to combine multiple resource types into a single MBean, this can lead to unmanageable code.
Example:
public class AppMetrics implements AppMetricsMBean {
public int getActiveSessions() { /*...*/ }
public void setSessionTimeout(int timeout) { /*...*/ }
public long getMemoryUsage() { /*...*/ } // Mixed metrics
}
Why It Matters: Overloaded MBeans can result in confusion when monitoring. It becomes difficult to manage several metrics related to different functionalities.
Solution: Create separate MBeans for distinct resource types. This not only enhances code readability but also simplifies the monitoring process.
4. Not Securing JMX
Another common oversight is neglecting security measures while exposing JMX beans. By default, JMX might allow unauthorized access to your MBeans.
Solution: Implement security measures by configuring SSL/TLS, authentication, and authorization mechanisms. Here's how you might set up a secured JMX connection:
Example: Configure Java agent to secure JMX
-Dcom.sun.management.jmxremote
-Dcom.sun.management.jmxremote.port=12345
-Dcom.sun.management.jmxremote.authenticate=true
-Dcom.sun.management.jmxremote.password.file=/path/to/password.file
-Dcom.sun.management.jmxremote.access.file=/path/to/access.file
5. Lack of Monitoring Tools
Some developers may not utilize monitoring tools that can help in understanding JMX metrics and performance. JConsole and VisualVM are popular Java monitoring tools, but many do not take full advantage of them.
Why It Matters: Not using monitoring tools means missing out on valuable insights regarding application performance.
Solution: Familiarize yourself with popular Java monitoring tools like JConsole and VisualVM. Use them to visualize and gain insights from JMX metrics.
6. Failing to Implement Notification Mechanisms
JMX allows for event notifications, yet many developers overlook this feature.
Example:
public void sendNotification() {
Notification notification = new Notification("com.example.notification.type",
this, notificationSequence++);
sendNotification(notification);
}
Why It Matters: Notifications keep developers informed regarding critical events such as changes in application status or unexpected errors.
Solution: Implement notifications within your MBeans to alert relevant parties regarding key events.
7. Not Handling Data Types Properly
Data type handling in JMX should be meticulous, otherwise incorrect data manipulation can occur.
Example:
public void setActiveSessionCount(int count) {
// Ensure count is positive
if (count < 0) {
throw new IllegalArgumentException("Count cannot be negative");
}
this.activeSessionCount = count;
}
Why It Matters: Incorrect data types can lead to unexpected exceptions and undermine your monitoring system.
Solution: Use proper data sanitization and validation strategies within your MBeans to prevent invalid state.
8. Ignoring Performance Impact
Lastly, many developers fail to foresee the impact of JMX on application performance. Accessing heavy data frequently can degrade system performance.
Solution: Tune your MBeans to only expose essential attributes or implement caching strategies for data that don’t change often.
Key Takeaways
Navigating the landscape of Java JMX monitoring can be challenging, but by avoiding these common pitfalls, you can establish a more effective and efficient monitoring strategy. Start by focusing on clear and concise MBean design, ensuring security, utilizing tools, and overseeing performance implications.
Monitoring your Java application correctly can lead to significant operational efficiencies and improved performance. Consider implementing the solutions discussed in this post, and keep refining and enhancing your monitoring strategy!
For further exploration into JMX monitoring and management, check out JavaDocs on JMX. Happy coding!
Checkout our other articles