Securing Your Java App: Automating Azure Group Creation
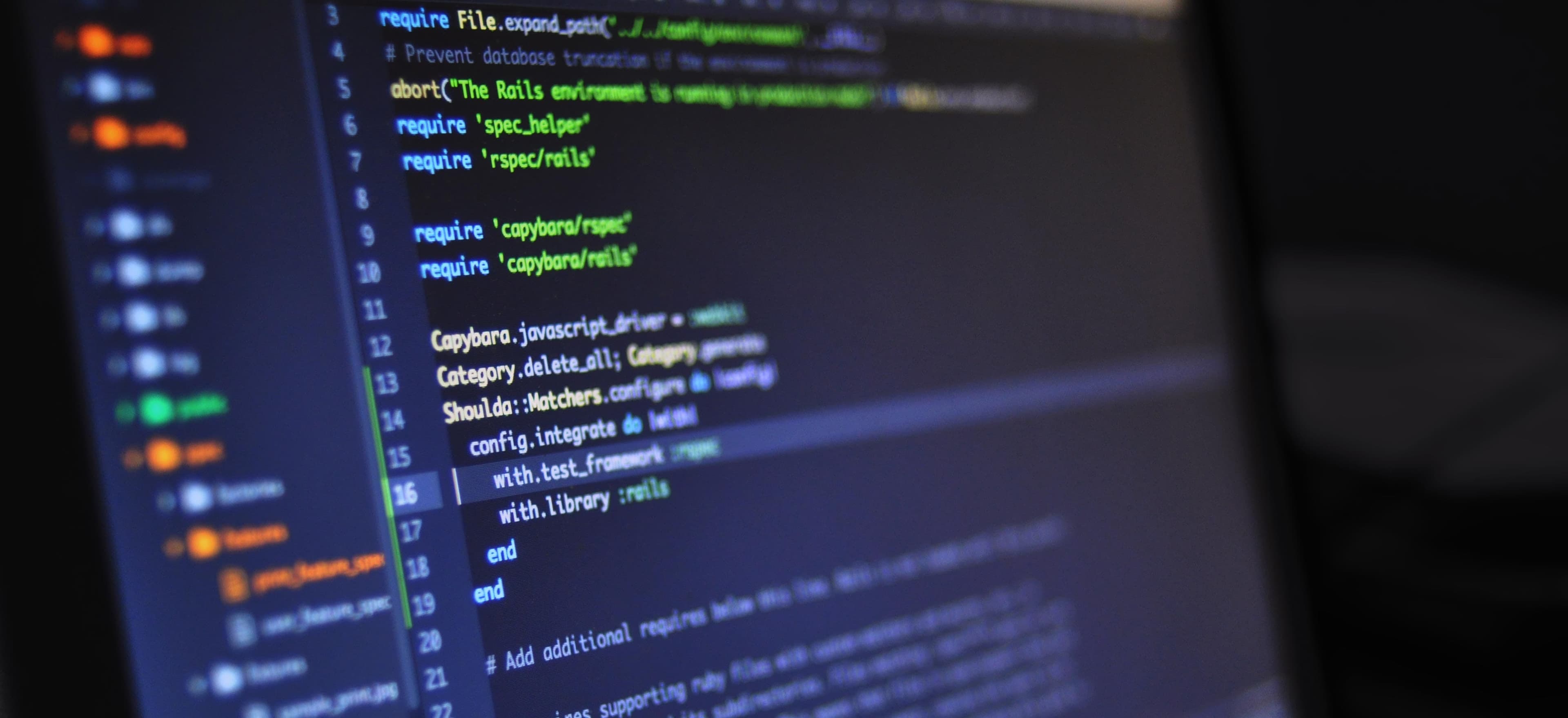
- Published on
Securing Your Java App: Automating Azure Group Creation
In today's technology landscape, securing applications is paramount. Java developers often face challenges when it comes to maintaining high-security standards for their applications. One effective method of enhancing security is by leveraging Azure Security Groups to control access to resources.
In this blog post, we will explore how to automate the creation of Azure Security Groups using Terraform in the context of a Java application. We're going to walk through the necessary concepts, provide examples, and show how you can streamline security management in your development workflow.
Understanding Azure Security Groups
Azure Security Groups (NSGs) are essential for controlling network traffic to and from Azure resources. They act as virtual firewalls, allowing you to specify rules for inbound and outbound traffic. Using NSGs, you can restrict access to your application, thereby enhancing its security posture.
Before we dive into automating the creation of security groups, it's important to establish how they fit into the overall architecture. Consider the following:
- Access Control: NSGs provide fine-grained control over the networking layer by allowing or denying traffic based on rules.
- Isolation: You can segment different components of your application (e.g., web tier, application tier, database tier) to prevent access from unauthorized users.
- Compliance: Many organizations have strict regulatory requirements regarding data access. Automating NSG management helps in compliance adherence.
For reference on creating Azure security groups using Terraform, feel free to visit the existing article, "Creating Azure Security Group with Terraform".
Prerequisites
To follow along with this blog post, you need:
- An Azure account
- Terraform installed on your machine
- Basic knowledge of Java and network security concepts
Setting Up Your Environment
Before we can automate the security group creation, we need to set up our environment.
Step 1: Installing Terraform
First, ensure Terraform is installed. You can verify by running the command in your terminal:
terraform version
If it's not installed, check out the official Terraform installation guide.
Step 2: Setting up Azure CLI
You’ll also want the Azure CLI installed to interact with Azure resources. Similar to Terraform, verify by typing:
az --version
If it’s not installed, you can find the installation instructions on the Azure CLI documentation page.
Automating Security Group Creation with Terraform
Now that your environment is set up, let's create a simple Terraform configuration to automate the creation of an Azure Security Group.
Step 1: Create a New Directory
Create a new directory for your Terraform configuration:
mkdir azure-security-group
cd azure-security-group
Step 2: Initialize Terraform
Create a file called main.tf
in your directory. This will contain the main configuration.
Step 3: Write the Terraform Configuration
Here’s a simplistic example of how to define an Azure Security Group in Terraform:
provider "azurerm" {
features {}
}
resource "azurerm_resource_group" "example" {
name = "example-resources"
location = "West Europe"
}
resource "azurerm_network_security_group" "example" {
name = "example-nsg"
location = azurerm_resource_group.example.location
resource_group_name = azurerm_resource_group.example.name
security_rule {
name = "example-rule"
priority = 100
direction = "Inbound"
access = "Allow"
protocol = "*"
source_port_range = "*"
destination_port_range = "80"
source_address_prefix = "*"
destination_address_prefix = "*"
}
}
Explanation of Code:
-
provider "azurerm": This block initializes the Azure provider which allows Terraform to interact with Azure resources.
-
azurerm_resource_group: This resource creates a resource group in Azure named "example-resources". Resource groups are logical containers for Azure resources.
-
azurerm_network_security_group: This resource defines our Azure Security Group. Here, we add a security rule allowing inbound traffic on port 80 from any source.
This configuration grants HTTP access, but production applications would typically require fine-tuned rules based on specific needs.
Step 4: Execute Terraform Commands
After defining your resources, run the following commands:
- Initialize Terraform:
terraform init
This prepares your directory for deployment, downloading necessary provider plugins.
- Plan Your Deployment:
terraform plan
This command provides a preview of what Terraform will create.
- Apply Configuration:
terraform apply
Terraform will set up your resources based on your configuration. You'll be prompted to confirm before proceeding.
Integration with Java Applications
Now that we have our security group defined, how does this tie back to our Java application?
Using Azure SDK for Java
To leverage Azure’s security features directly in your Java application, you can use the Azure SDK for Java. This SDK allows you to manage Azure resources through Java code.
Sample Code to Access Azure Resources
First, add the Azure SDK dependency to your Maven pom.xml
:
<dependency>
<groupId>com.azure</groupId>
<artifactId>azure-resourcemanager</artifactId>
<version>2.7.0</version>
</dependency>
Next, you can create a simple Java class to manage Azure resources:
import com.azure.resourcemanager.AzureResourceManager;
import com.azure.resourcemanager.AzureEnvironment;
import com.azure.identity.DefaultAzureCredentialBuilder;
public class AzureManager {
private static AzureResourceManager azureResourceManager;
public static void main(String[] args) {
azureResourceManager = AzureResourceManager.configure()
.withLogLevel(LogLevel.BASIC)
.authenticate(new DefaultAzureCredentialBuilder().build(), "<your-tenant-id>")
.withDefaultSubscription();
manageSecurityGroups();
}
private static void manageSecurityGroups() {
// Logic to retrieve, create or update Azure Security Groups
}
}
Why Integrate with SDK?
Integrating your Java application with Azure SDK allows for programmatic management of Azure resources directly from your code, fostering seamless automation. The benefits include:
- Dynamic Security Management: This enables your application to react quickly to security needs.
- Version Control: By coding your infrastructure, it's easier to track changes.
- Consistency: Automated configurations reduce manual errors.
The Bottom Line
Having outlined how to automate Azure Security Group creation with Terraform and integrate it into your Java applications, you now have a functional method of maintaining secure applications on Azure. As developers, it is essential to prioritize security and adopt best practices in application development.
By utilizing tools like Terraform for infrastructure automation and the Azure SDK for direct management, you can effectively safeguard your application landscape.
For further reading on how to create Azure Security Groups with Terraform, check out the detailed guide here.
With security in mind, remember that every application is unique. Tailor your network security setup to meet your specific requirements, thereby providing robust protection for your Java applications.