Integrating Java with HTML: Avoiding Common Mistakes
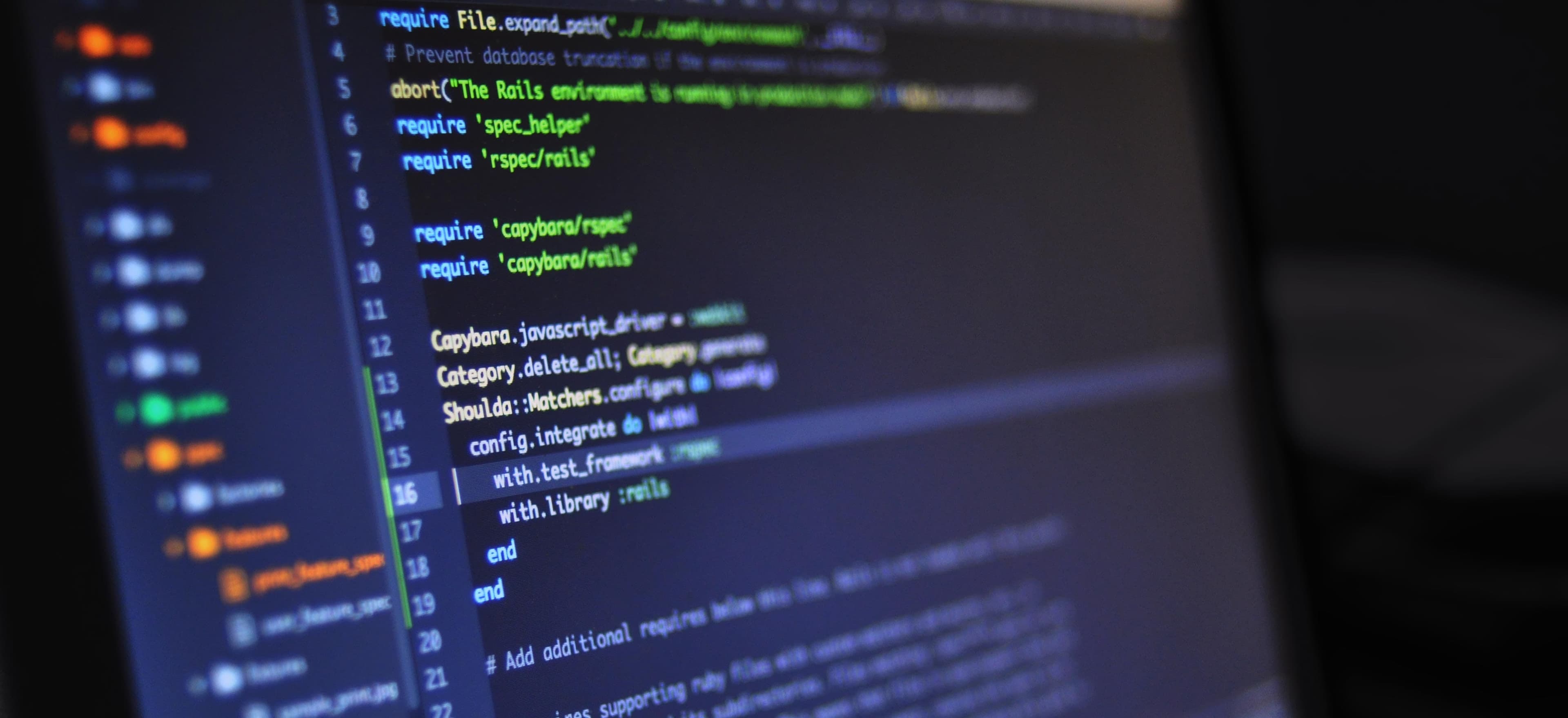
- Published on
Integrating Java with HTML: Avoiding Common Mistakes
In the realm of web development, the integration of Java with HTML is a common yet often puzzling endeavor. Java is a powerful programming language that enables dynamic web applications, while HTML is the backbone of web content structure. Integrating these two can lead to efficient and effective web applications, although it isn't without its pitfalls.
By the end of this article, you'll feel more confident about weaving Java into your HTML projects and steering clear of frequent mistakes. We'll explore best practices, code snippets, and provide resources to enhance your understanding.
Understanding the Integration Process
Before we dive into the code, it's essential to comprehend how Java can interact with HTML. Most often, this is mediated by Java Servlets or JSP (JavaServer Pages). Servlets are Java programs that run on a server and generate dynamic content, while JSP allows for the embedding of Java code directly into HTML, making it easier to generate dynamic web pages.
Java Servlets: The Basics
Java Servlets serve as a bridge between client requests and server responses. When a client sends a request, the servlet processes that request, communicates with the database or backend logic as necessary, and generates a response.
Here's a basic example of a Java Servlet:
import java.io.IOException;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.PrintWriter;
public class HelloWorldServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<html><body>");
out.println("<h1>Hello, World!</h1>");
out.println("</body></html>");
}
}
Code Breakdown
- Content Type: Setting the content type ensures that the server tells the browser what type of content to expect. Here, we set it to
text/html
. - PrintWriter: This allows us to send character text as a response to the client request.
Avoiding Common Pitfalls with Java Servlets
Integrating Java with HTML does come with challenges. For instance, one common mistake is failing to manage session states correctly.
Managing Sessions
Improper session management can lead to user frustration or security vulnerabilities. A common practice when using servlets involves leveraging the HttpSession object. Here is a snippet:
HttpSession session = request.getSession(true);
session.setAttribute("user", username);
Why Is This Important?
Sessions allow the server to recognize returning users, enhancing personalization and user experience. Neglecting to manage sessions may result in loss of user data or account security breaches.
Embracing JSP for Easier Integration
JavaServer Pages (JSP) simplify the process of mixing HTML with Java code more straightforward than servlets. It allows you to craft dynamic pages without extensive boilerplate code.
Here's a simple example of JSP code:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<title>Welcome Page</title>
</head>
<body>
<h1>Welcome, <%= request.getAttribute("user") %></h1>
</body>
</html>
JSP Code Breakdown
- Expression Language: The
<%= %>
syntax allows you to embed Java expressions within the HTML. Here, we reflect a user’s name dynamically. - Simplicity: JSP reduces the volume of Java code required and streamlines data presentation.
Common Mistakes in JSP
While JSP offers convenience, developers often make mistakes, such as embedding too much Java code into pages, which can clutter the overall structure. This can lead to reduced readability and maintainability.
Best Practice
Keep Java logic separate from HTML structure. Use JavaBeans and custom tags to promote clean separation of concerns. This will make your JSP pages more manageable and your web applications more scalable.
An Important Consideration: Security
Security is a critical aspect of any web application. When integrating Java with HTML, developers should be wary of Injection Attacks. Here’s how to mitigate these risks:
Input Validation
Input must always be sanitized or validated before being processed. Here's a generic example:
String userInput = request.getParameter("input");
if (userInput != null && userInput.matches("^[a-zA-Z0-9]+$")) {
// Process input
} else {
// Handle invalid input
}
Why Does This Matter?
Not validating user input can lead to SQL Injection attacks, where attackers manipulate user input to execute arbitrary SQL queries. By validating input upfront, you create a robust defense against numerous web vulnerabilities.
The Closing Argument
Integrating Java with HTML can drastically improve the functionality of your web applications. However, it is paramount to navigate potential pitfalls carefully. Whether you are using Servlets or JSP, maintaining clean code structure, managing sessions properly, and prioritizing security remain vital endeavors.
If you're interested in further reading about PHP and HTML integrations, specifically common pitfalls to watch for, check out the article titled Blending PHP & HTML in Echo: A Common Pitfall.
For an array of effective development strategies and tips, keep these best practices in mind. Mistakes can be learning opportunities; however, by adhering to careful coding standards and security practices, you can prevent them before they even occur. Happy coding!
Checkout our other articles