Avoiding Common Pitfalls in Java Web Frameworks
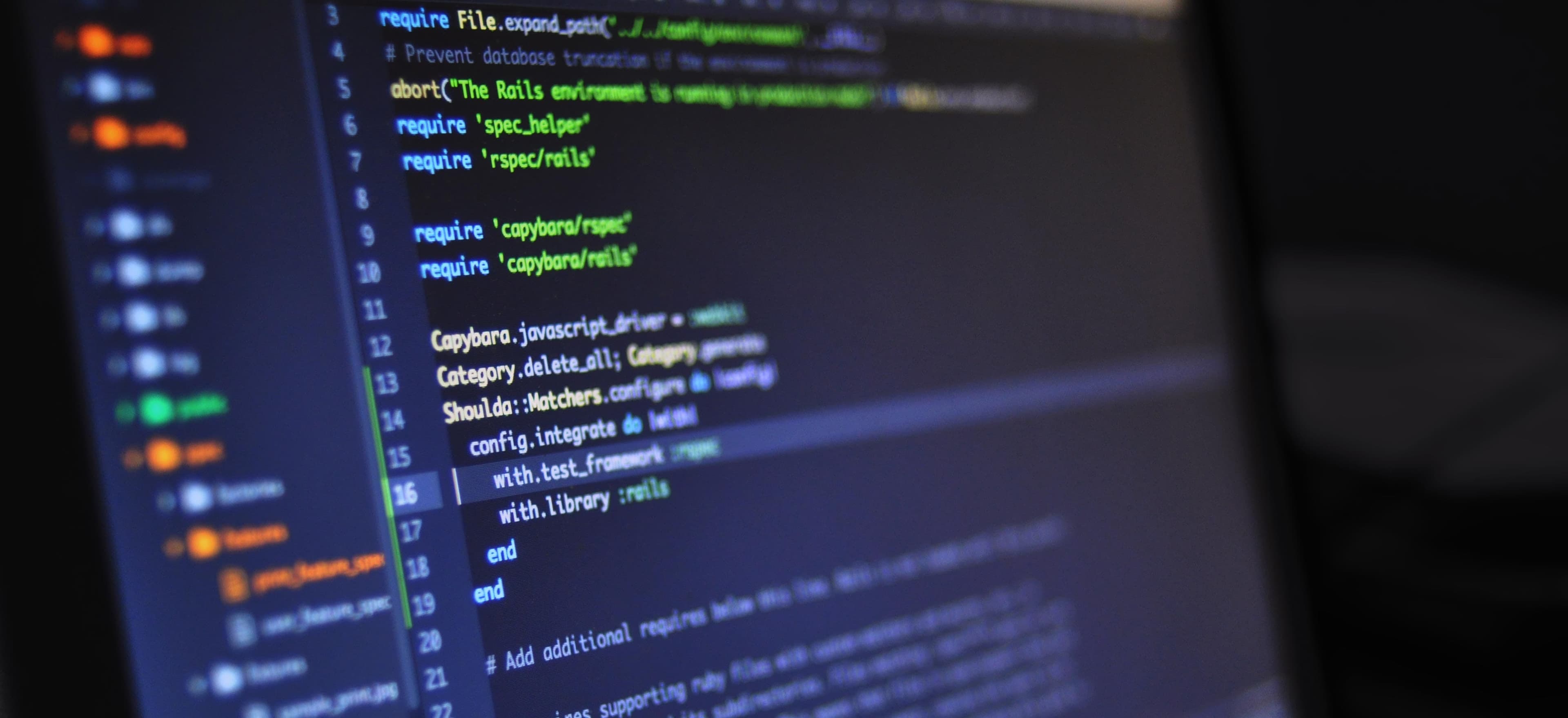
- Published on
Avoiding Common Pitfalls in Java Web Frameworks
Java web frameworks have revolutionized how developers create dynamic web applications. While they offer powerful tools and functionalities, pitfalls can arise that may lead to buggy applications or inefficient coding practices. In this blog post, we will explore some common pitfalls in Java web frameworks and how to avoid them.
Understanding Java Web Frameworks
Before delving into pitfalls, let's clarify what Java web frameworks are. They serve as pre-defined structures to facilitate the development of web applications. Some popular frameworks include:
- Spring: A comprehensive framework offering various modules for different functionalities.
- JavaServer Faces (JSF): A component-based framework for building user interfaces.
- Apache Struts: A framework more suited for MVC architecture.
Each has its strengths, but they also share common challenges. Let's explore these pitfalls and how to navigate them, ensuring you build robust applications.
Pitfall 1: Over-Engineering
One of the most frequent missteps developers make when using Java web frameworks is over-engineering solutions.
Why It Happens
In an effort to make code extensible and maintainable, developers often add unnecessary complexity. For instance, you might be tempted to modularize every single piece of functionality, which can lead to a convoluted architecture.
How to Avoid It
- Keep it Simple: Adopt the KISS principle (Keep It Simple, Stupid). Refactor and streamline code wherever possible.
- Use Built-in Features: Most frameworks have features intended to streamline basic functionality. For example, use Spring's built-in dependency injection instead of creating custom instances for every class.
Code Example
// Over-Engineering: Custom Dependency Injection
public class CustomInjector {
public static MyService getMyService() {
return new MyServiceImpl();
}
}
// Simpler approach using Spring's built-in DI
@Component
public class MyController {
private final MyService myService;
@Autowired
public MyController(MyService myService) {
this.myService = myService;
}
}
In this example, using Spring's built-in dependency injection framework prevents unnecessary boilerplate code. Always remember that existing tools can simplify your tasks.
Pitfall 2: Ignoring Exception Handling
Proper exception handling is often overlooked, leading to applications abruptly shutting down or exposing sensitive information.
Why It Happens
Some developers assume that simply catching an exception will suffice. However, failing to treat exceptions correctly can lead to poor user experience and unexpected application behavior.
How to Avoid It
- Centralized Exception Handling: Use a centralized exception handling approach to catch and log exceptions globally. In Spring, for instance, you can utilize the
@ControllerAdvice
annotation.
Code Example
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<Object> handleResourceNotFound(ResourceNotFoundException ex) {
// Log the exception
return new ResponseEntity<>(ex.getMessage(), HttpStatus.NOT_FOUND);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<Object> handleAllExceptions(Exception ex) {
// Log the exception
return new ResponseEntity<>("An error occurred", HttpStatus.INTERNAL_SERVER_ERROR);
}
}
In this example, handling exceptions globally creates a consistent experience for users and simplifies error management across your application.
Pitfall 3: Neglecting Security
Web applications built on Java frameworks are often exposed to security vulnerabilities like SQL injection and Cross-Site Scripting (XSS).
Why It Happens
With numerous features to focus on, security can fall off the radar, especially when developers prioritize functionality over safety.
How to Avoid It
- Input Validation: Always validate user input to avoid malicious data. Utilize built-in validation in your framework.
- Use Security Features: Frameworks like Spring Security offer essential security features such as authentication and authorization.
Code Example
// Input Validation using Spring’s @Valid annotation
@PostMapping("/register")
public ResponseEntity<String> registerUser(@Valid @RequestBody UserDto userDto) {
userService.register(userDto);
return ResponseEntity.ok("Registration successful");
}
By incorporating input validation, you block the entry of unwanted data into your application. Security should never be an afterthought.
Pitfall 4: Misusing the Framework’s Features
Frameworks provide many powerful tools, but misusing these capabilities can lead to performance issues and fragile codebases.
Why It Happens
Familiarity with the tools may lead developers to use them incorrectly. A common example is improper ORM (Object Relational Mapping) usage resulting in n+1 query problems.
How to Avoid It
- Understand Framework Limitations: Read the documentation. Don’t just scratch the surface; dig deep into performance implications and best practices laid out by the framework maintainers.
Code Example
// Inefficient: Loading data one-by-one (n+1 query issue)
List<User> users = userRepository.findAll();
for (User user : users) {
System.out.println(user.getOrders()); // Triggers additional queries
}
// Using Fetch Joins
@Query("SELECT u FROM User u JOIN FETCH u.orders")
List<User> findUsersWithOrders();
In this example, using a fetch join reduces the number of database calls significantly, improving performance. Always analyze your design decisions in the context of performance.
Pitfall 5: Not Writing Tests
Finally, neglecting to write tests can be a significant omission. Code without tests can lead to regression issues and unreliable builds.
Why It Happens
Gathering requirements, writing code, and deploying can seem overwhelming, and testing often takes a backseat.
How to Avoid It
- Test-Driven Development (TDD): Adopt TDD practices. Writing your test cases before the actual code leads to better-structured code.
- Leverage Framework Testing Support: Frameworks commonly provide testing libraries. Utilize JUnit or Spring Test for unit and integration tests.
Code Example
@RunWith(SpringRunner.class)
@SpringBootTest
public class UserServiceTests {
@Autowired
private UserService userService;
@Test
public void testUserRegistration() {
UserDto dto = new UserDto("testUser", "testPassword");
userService.register(dto);
Assertions.assertNotNull(userService.findUserByUsername("testUser"));
}
}
In this example, using Spring's testing framework allows you to verify the behavior of your application easily. Tests not only safeguard your existing code but also help in future development.
Final Thoughts
Java web frameworks unlock endless possibilities for developers but come with their share of pitfalls. By avoiding over-engineering, properly managing exceptions, ensuring security, utilizing framework features wisely, and writing solid tests, you can create robust, maintainable applications.
For more insights into how web technologies can intersect and lead to potential pitfalls, check out Blending PHP & HTML in Echo: A Common Pitfall.
Java frameworks can simplify and enhance your development experience; understanding and avoiding common pitfalls is key to success. Happy coding!
Checkout our other articles