Managing High Cardinality in Java Time Series Applications
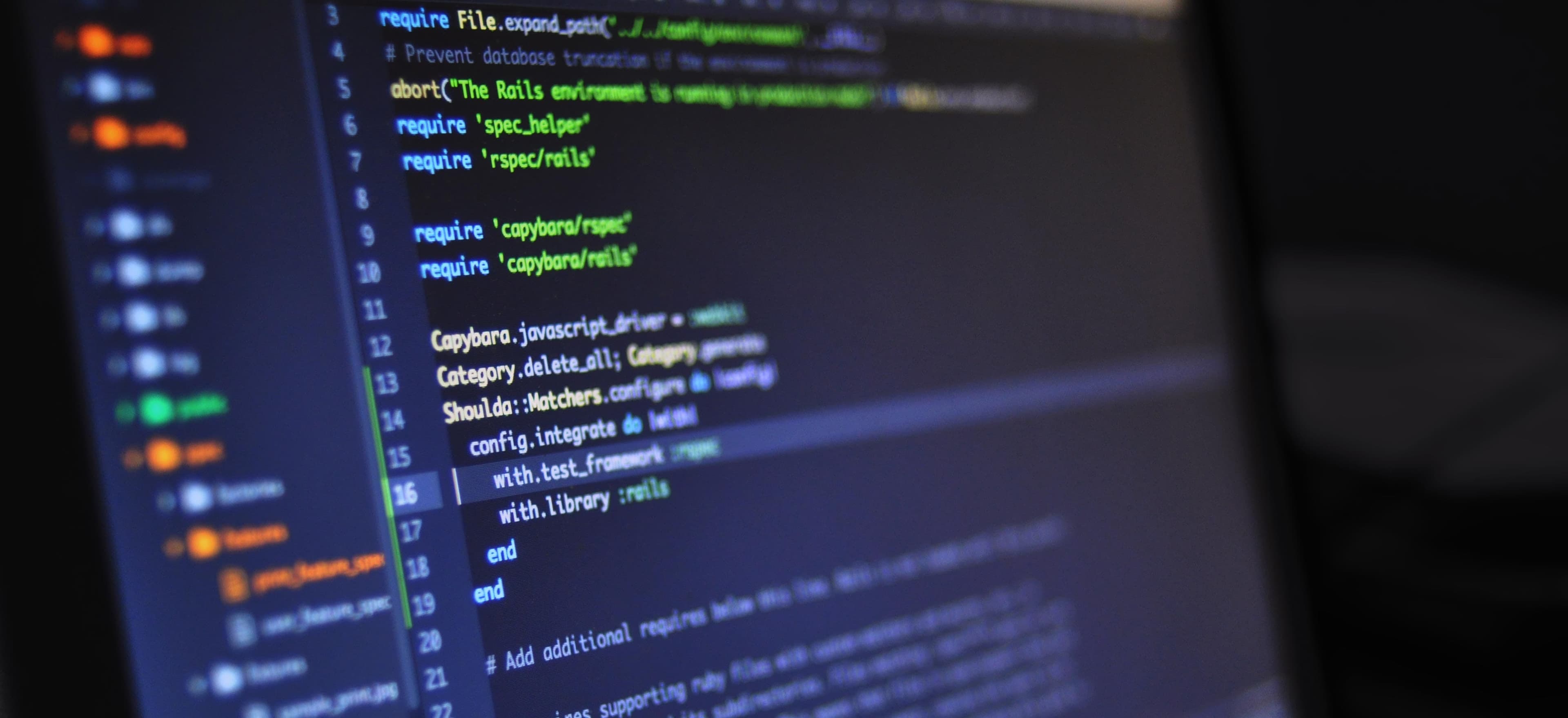
- Published on
Managing High Cardinality in Java Time Series Applications
In today's data-driven world, managing high cardinality data is no trivial feat, especially when it comes to time series databases. High cardinality refers to the situation where a dataset contains a vast number of unique values for a given field. For instance, metrics collected from millions of IoT devices can result in high cardinality, which can complicate data storage, retrieval, and even analytics.
This blog post will explore efficient strategies for handling high cardinality data in Java-based time series applications. We will look at various techniques and best practices that will streamline your workflow and maintain performance. For additional context, make sure to check the article titled Comparison: Handling High Cardinality Data in Time Series Databases.
Understanding High Cardinality
High Cardinality is significant when dealing with time series data. Consider metrics like user activity logs, device statistics, or sensor readings. Each unique user, device, or sensor contributes to high cardinality. The primary challenges associated with it include:
- Storage: Larger volumes of unique values require more space.
- Query performance: Retrieving information becomes slower as more unique metrics are involved.
- Indexing: Traditional indexing techniques may not work efficiently with high cardinality.
Moreover, effectively managing high cardinality data can lead to enhanced performance, faster queries, and ultimately better insights from your data.
Strategies for Managing High Cardinality in Java
1. Use Appropriate Data Structures
Java offers a variety of data structures that can be used to manage high cardinality efficiently. HashMap
is particularly useful for scenarios where you need to maintain a collection of key-value pairs. If you only need to keep track of unique values, a HashSet
might be your best bet.
Example: Storing Unique Event Types
import java.util.HashSet;
public class EventTracker {
private HashSet<String> eventTypes;
public EventTracker() {
eventTypes = new HashSet<>();
}
public void trackEvent(String eventType) {
eventTypes.add(eventType);
}
public int getUniqueEventCount() {
return eventTypes.size();
}
}
Why Use HashSet
?
- It manages unique items efficiently, allowing O(1) time complexity for add and check operations.
- This is ideal for a scenario where tracking unique event types or user interactions is crucial.
2. Compounding Metrics
Consider using compound metrics in your application to reduce cardinality. Instead of storing individual metrics for every unique event or device separately, you can aggregate them into a single metric.
Example: Compounding User Activity
import java.time.LocalDateTime;
import java.util.HashMap;
public class UserActivity {
private HashMap<String, Integer> userActivityCount;
public UserActivity() {
userActivityCount = new HashMap<>();
}
public void recordActivity(String userId) {
userActivityCount.merge(userId, 1, Integer::sum);
}
public void displayActivityCounts() {
for (String userId : userActivityCount.keySet()) {
System.out.println("User: " + userId + " Activity Count: " + userActivityCount.get(userId));
}
}
}
Why Use HashMap for Compounding?
- The
merge
function allows you to aggregate results intuitively. - This approach handles high cardinality gracefully, as it helps avoid multiple entries for the same user.
3. Time Bucketing
Time bucketing helps in reducing the granularity of time series data. Instead of storing every individual timestamp, you can bucket data into larger intervals (e.g., minute, hour).
Example: Bucketing Data
import java.time.Instant;
import java.time.temporal.ChronoUnit;
import java.util.HashMap;
public class TimeBucketing {
private HashMap<Long, Integer> bucketedData; // Storing counts by bucket timestamp
public TimeBucketing() {
bucketedData = new HashMap<>();
}
public void addDataPoint(Instant timestamp) {
long bucketKey = timestamp.truncatedTo(ChronoUnit.HOURS).toEpochMilli(); // Bucketing to the hour
bucketedData.merge(bucketKey, 1, Integer::sum);
}
public void displayBucketedData() {
for (Long key : bucketedData.keySet()) {
System.out.println("Bucket: " + Instant.ofEpochMilli(key) + " Count: " + bucketedData.get(key));
}
}
}
Why Use Time Bucketing?
- It reduces the volume of data by aggregating points over time.
- Query operations become more efficient, as the data size is significantly less.
4. The Role of Compression
Applying compression techniques helps manage the size of high cardinality datasets. Java provides libraries like Java Data Compression (java.util.zip) to facilitate this.
Example: Compressing Data
import java.util.zip.Deflater;
import java.util.zip.Inflater;
public class DataCompressor {
public byte[] compressData(String data) {
Deflater deflater = new Deflater();
deflater.setInput(data.getBytes());
deflater.finish();
byte[] compressedData = new byte[1024];
int compressedDataLength = deflater.deflate(compressedData);
deflater.end();
byte[] result = new byte[compressedDataLength];
System.arraycopy(compressedData, 0, result, 0, compressedDataLength);
return result;
}
}
Why Compress Data?
- It saves storage, making it crucial for high cardinality scenarios.
- Compressed data consumes less space, which translates to improved I/O performance during retrieval.
Lessons Learned
Managing high cardinality in Java time series applications involves a combination of the right data structures, aggregation techniques, time bucketing, and compression strategies. By applying these practices, you can optimize your applications to effectively handle vast amounts of unique data while maintaining superior performance.
For additional insight on handling high cardinality data specifically in time series databases, explore the intricate methodologies discussed in the article Comparison: Handling High Cardinality Data in Time Series Databases. By keeping abreast of these strategies, developers can build more robust time series applications capable of delivering critical business insights despite the complexities of high cardinality data.
Tips for Future Development
- Monitor cardinality levels regularly and adjust strategies as necessary.
- Consider database-specific solutions, like using time series databases that inherently manage high cardinality more efficiently.
By adopting these best practices, you position yourself to meet the challenges of modern data technologies more effectively, paving the way for enhanced data insights and decision-making capabilities in your Java applications.