Mastering Codebase Validation: A Java Developer's Guide
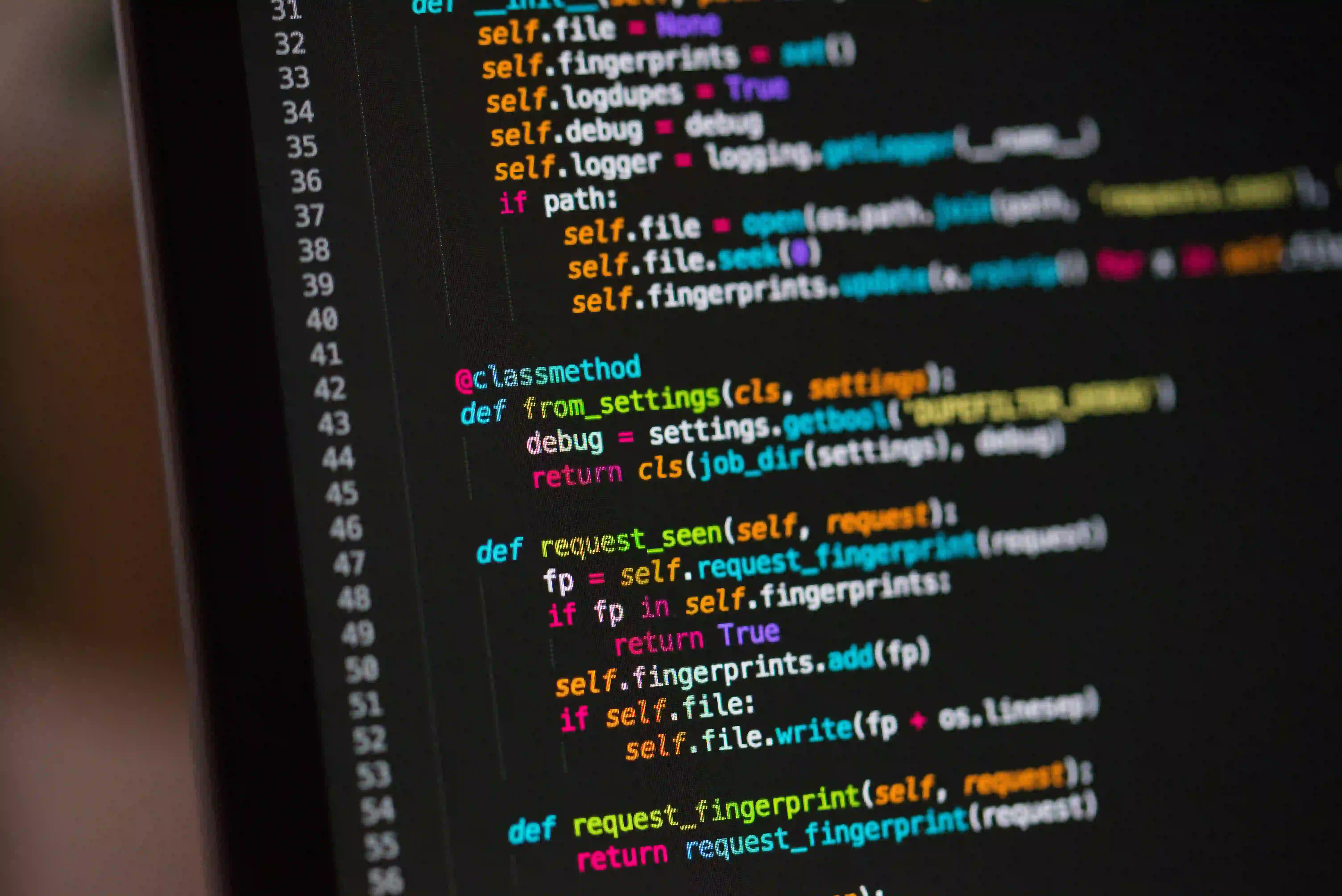
Mastering Codebase Validation: A Java Developer's Guide
In the realm of software development, codebase validation stands as a crucial practice in maintaining code quality and ensuring stability. As Java developers, we often find ourselves updating files, which can lead to introducing bugs if proper validation isn't conducted. In this article, we will explore effective strategies for validating changes within your Java codebase. We'll delve into tools, best practices, and provide relevant code snippets to illustrate our discussion.
Why Codebase Validation Matters
When working on a codebase, changes can have unintended consequences. Without validation, a developer might overlook errors that could cascade into larger issues. Codebase validation helps to mitigate risks associated with these changes. It allows us to:
-
Identify Bugs Early: Detecting issues at the stage of code changes is significantly cheaper compared to post-deployment debugging.
-
Enhance Code Quality: Regular validation encourages adherence to coding standards and best practices.
-
Facilitate Collaboration: Teams can work together more effectively when the codebase is validated, resulting in fewer integration conflicts.
-
Improve Deployment Confidence: You can deploy changes with peace of mind when you know your code has been validated.
To explore how to effectively validate changed files in your codebase, I recommend checking out How to Effectively Validate Changed Files in Your Codebase.
Tools for Codebase Validation
Several tools can aid us in validating our Java codebase:
1. Maven
Maven is not just a build tool but also provides a robust framework for managing project dependencies and plugins, which can be leveraged for validations.
Example: Maven Checkstyle Plugin
Checkstyle helps to maintain consistent coding standards. You can add the Checkstyle plugin to your Maven project as follows:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-checkstyle-plugin</artifactId>
<version>3.1.2</version>
<configuration>
<configLocation>checkstyle.xml</configLocation>
<outputDirectory>${project.build.directory}/checkstyle-output</outputDirectory>
</configuration>
<executions>
<execution>
<phase>validate</phase>
<goals>
<goal>check</goal>
</goals>
</execution>
</executions>
</plugin>
Why this matters: This configuration enforces your project's coding style during the validation phase. Any violation will be reported before the build process continues, ensuring that your team adheres to coding standards.
2. JUnit
Unit testing is an essential component of validating your code changes. JUnit allows us to write repeatable tests for our Java applications.
Example: Simple JUnit Test
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class MathUtilsTest {
@Test
public void testAdd() {
MathUtils mathUtils = new MathUtils();
assertEquals(5, mathUtils.add(2, 3), "Optional assertion message; suggests a bug!");
}
}
Why this matters: The test above verifies the correctness of the add
method in the MathUtils
class. If changes are made to add
, running this test will immediately highlight any issues introduced by the modifications.
3. SonarQube
SonarQube is an excellent tool for continuously checking the quality of your code, keeping track of code smells, bugs, and security vulnerabilities.
Example: Integrating SonarQube with Maven
You can set up SonarQube easily with this configuration in your pom.xml
:
<properties>
<sonar.projectKey>your_project_key</sonar.projectKey>
<sonar.host.url>http://localhost:9000</sonar.host.url>
<sonar.login>your_sonar_login</sonar.login>
</properties>
<build>
<plugins>
<plugin>
<groupId>org.sonarsource.scanner.maven</groupId>
<artifactId>sonar-maven-plugin</artifactId>
<version>3.9.0.1746</version>
</plugin>
</plugins>
</build>
Why this matters: This setup allows you to run SonarQube analysis as part of your Maven build, giving you insights into the quality of your code after each change.
Best Practices for Codebase Validation
While we've looked at tools, our approach to validation should also involve best practices. Here are a few to consider:
1. Validate Before Each Commit
Always run your validation tools and unit tests before committing changes. This ensures that you're not introducing any broken code into the shared codebase.
2. Automate Your Validation Process
Integrating tools like Travis CI or GitHub Actions can automate your validation process. You can set up continuous integration (CI) pipelines to run your tests and linters whenever a pull request is created.
Example of GitHub Actions Workflow
name: Java CI
on: [push, pull_request]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK 11
uses: actions/setup-java@v2
with:
java-version: '11'
- name: Build with Maven
run: mvn clean install
- name: Run Tests
run: mvn test
Why this matters: This YAML configuration automates the build and test process, ensuring that any change is immediately validated before merging into the main branch.
3. Conduct Code Reviews
Pairing up with peers for code reviews can help spot issues that automated tools might overlook. Reviews foster knowledge sharing and collective responsibility for code quality.
Closing the Chapter
Mastering codebase validation in Java is essential for any developer looking to deliver quality software. By utilizing tools like Maven, JUnit, and SonarQube, as well as following best practices, we can significantly reduce the likelihood of bugs and maintain a clean codebase.
For further reading on validating file changes in your codebase, be sure to check out How to Effectively Validate Changed Files in Your Codebase.
Implementing validation strategies within your Java development process is not an overnight endeavor, but with consistent practice, it will become an integral part of your workflow, elevating the quality and reliability of your software.
With this knowledge, you are now equipped to master codebase validation in your Java projects. Start today, and take your code quality to new heights!