Streamlining Validation of Java Code Changes for Better Quality
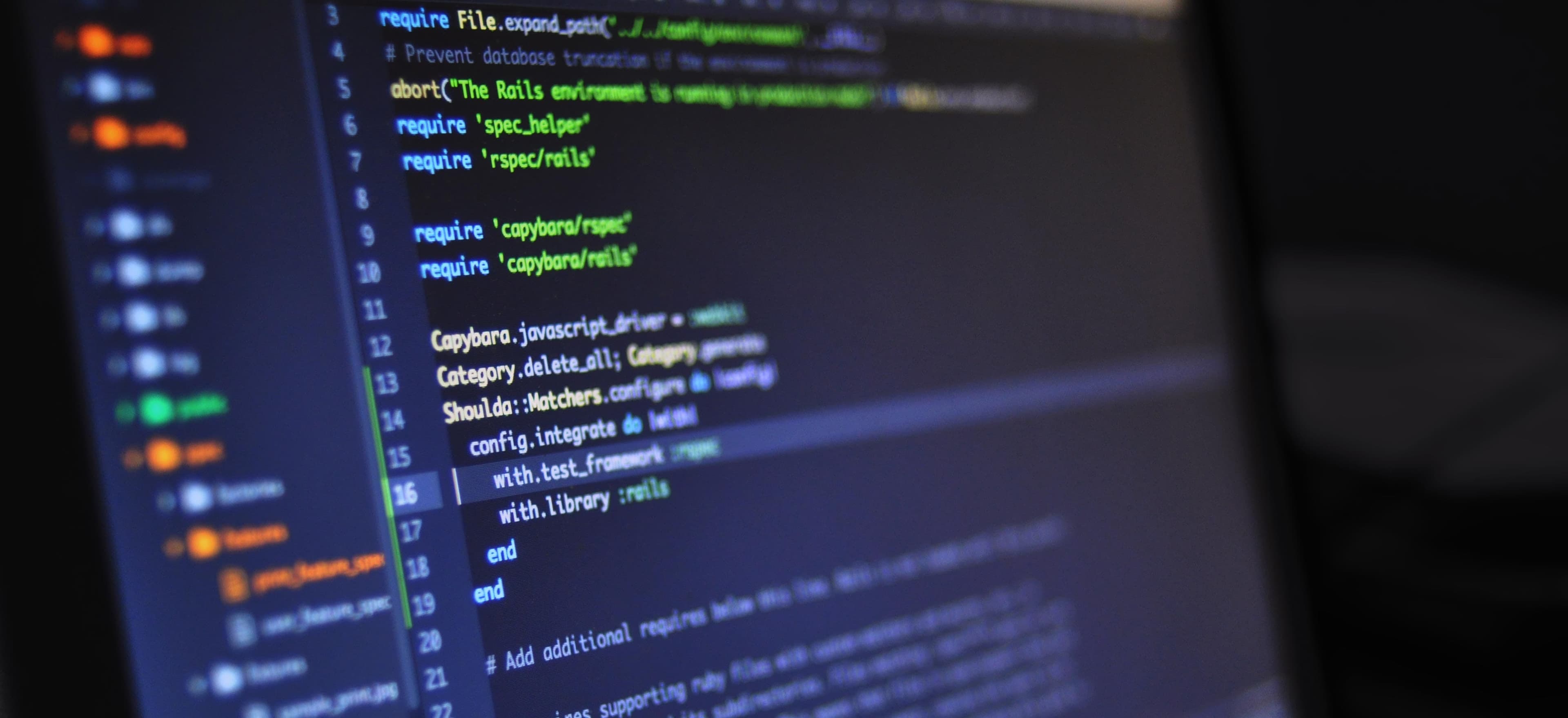
- Published on
Streamlining Validation of Java Code Changes for Better Quality
In today's fast-paced software development environment, the integrity and quality of code are paramount. When changes are made, whether minor or significant, validating those changes becomes critical. In this blog post, we'll explore best practices to streamline the validation process for Java code changes. We'll discuss various techniques, tools, and strategies to enhance the quality of your code and reduce the likelihood of introducing bugs.
The Importance of Code Validation
Validation refers to the process of ensuring that your code meets certain standards and behaves as expected after changes are made. Failing to validate your code can lead to:
- Unexpected Bugs: Unintended behavior or errors that affect the application's functionality.
- Increased Technical Debt: As code quality decreases, maintaining the software becomes increasingly difficult.
- Lowered Team Morale: Frequent issues can lead to frustrations and impact productivity.
As noted in the article "How to Effectively Validate Changed Files in Your Codebase", establishing a robust validation process is essential for maintaining code quality and achieving project goals.
Effective Strategies for Code Validation
- Automated Testing
Automated testing is one of the foundational pillars of a good validation strategy. It enables teams to run a suite of tests anytime code changes occur. This ensures that regressions are identified early and do not slip into production.
Unit Testing
Unit tests verify the functionality of individual components of your application. In Java, popular libraries like JUnit can be employed for this purpose.
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result, "2 + 3 should equal 5");
}
}
Why Use Unit Tests?
Unit tests provide a safety net; if they fail, you know exactly which part of your code is broken. This pinpoints issues related to recent changes and reduces debugging time.
Integration Testing
Once unit tests are in place, integration tests can be used to assess how components interact with one another:
import static org.junit.jupiter.api.Assertions.assertNotNull;
import org.junit.jupiter.api.Test;
public class UserServiceTest {
@Test
public void testUserRegistration() {
UserService userService = new UserService();
User user = userService.register("testUser", "password");
assertNotNull(user, "User should not be null after registration");
}
}
Why Integration Tests?
Integration tests ensure that different parts of your system work together seamlessly. Testing individual components in isolation is important, but we must also verify the entire system operates as expected.
- Code Review Practices
A solid code review system involves team members assessing each other's code changes. This not only helps spot errors but also facilitates knowledge sharing.
Best Practices for Code Reviews:
- Use Pull Requests: This allows discussion and collaborative review before merging to the main branch.
- Enforce Review Guidelines: Specify what should be looked at in each review — readability, performance, and adherence to coding standards.
- Limit Review Size: Smaller pull requests are easier to review, leading to more thorough checks.
- Static Code Analysis
Static analysis tools scan your codebase for issues without executing the code. They can identify potential bugs, security vulnerabilities, and violations of coding standards.
Tools to Consider:
- SonarQube: Provides repository-wide insights into code quality.
- Checkstyle: Enforces coding standards by highlighting violations.
Integrating these tools into your existing CI/CD pipeline can save time and ensure your code adheres to established guidelines before it even reaches QA.
Continuous Integration/Continuous Deployment (CI/CD)
Having a CI/CD setup allows code changes to be validated automatically, ensuring that the integration of new features happens smoothly.
-
Setup Automated Build Process: A CI/CD tool like Jenkins, GitHub Actions, or Travis CI can trigger builds whenever code changes are made.
-
Run Tests Automatically: Ensure that your testing suite is executed during the CI/CD pipeline. Any failures should trigger notifications so that issues can be addressed immediately.
Monitoring Post-Deployment
While pre-deployment validation is crucial, it's equally important to monitor applications after deployment. Observing how changes behave in production can reveal unforeseen issues.
- Logging and Error Tracking: Use frameworks like SLF4J and Logback for logging, and tools like Sentry for tracking errors in real-time.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyService {
private static final Logger logger = LoggerFactory.getLogger(MyService.class);
public void process() {
try {
// Processing logic
} catch (Exception e) {
logger.error("An error occurred while processing", e);
}
}
}
Why Logging Matters?
Effective logging aids in diagnosing issues after they occur and provides context to the problems that users may encounter.
- Performance Monitoring: Tools like New Relic or Prometheus provide insights into how your application performs in a live environment, enabling you to take corrective action swiftly.
My Closing Thoughts on the Matter
Streamlining the validation of Java code changes is essential for delivering high-quality software. Embracing automated testing, adopting good code review practices, utilizing static analysis tools, and establishing a robust CI/CD pipeline will significantly reduce bugs and improve overall code quality.
Bringing these strategies into your development workflow may require some upfront investment, but the payoff in terms of productivity, morale, and code quality cannot be overstated. By following these best practices, you can ensure your Java projects remain robust and maintainable as they evolve.
For a deeper understanding of how to effectively validate files in your codebase, be sure to check out this article. Happy coding!